3 Python Methods to Remove Duplicates From a List
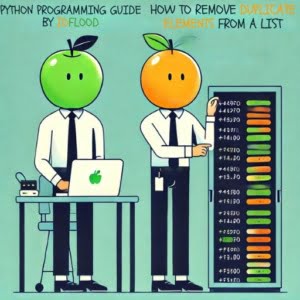
Data processing is a daily task when developing scripts at IOFLOOD, and to ensure the data sets are clean, we often need to remove duplicate elements. This operation ensures that data sets are unique and reliable, which is an important step. This article details the advantages and methods of removing duplicates from lists in Python, sharing our tips and processes to benefit our customers and improve data cleaning practices on their dedicated cloud services.
This comprehensive guide is designed to equip you with various methods to remove duplicates from a Python list. From basic techniques to more advanced methods, we aim to ensure you’re well-prepared to tackle this frequent task.
So, let’s embark on this journey to achieve duplicate-free Python lists!
TL;DR: How do I remove duplicates from a list in Python?
The simplest method to remove duplicates from a Python list is by using a
loop
. This method involves creating a new list and adding items to it only if they are not already present. The synta for this would be,if item not in list_without_duplicates: list_without_duplicates.append(item)
. However, for more advanced methods, background, tips and tricks, continue reading the article.
Example:
# Here is our list with duplicates
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5, 6, 6, 7]
# We create a new list without duplicates
list_without_duplicates = []
for item in list_with_duplicates:
if item not in list_without_duplicates:
list_without_duplicates.append(item)
print(list_without_duplicates)
# Output: [1, 2, 3, 4, 5, 6, 7]
This method, while simple and easy to understand, has its limitations. The most significant one is how slow it is. The “time complexity” of this method is O(n^2), which means it can be quite slow for large lists.
Table of Contents
Basic Method: The Loop
The loop method is a fundamental approach to removing duplicates from a Python list.
This technique involves creating a new list and adding items to it only if they are not already present. We already went over a “for” loop in our “TL;DR” example, so let’s do something a bit different here.
You can also use a while
loop with a counter to achieve the same goal. It’s not as efficient as the for
loop method, but it’s good to know multiple ways of achieving the same result:
# Here is our list with duplicates
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5, 6, 6, 7]
# We create a new list without duplicates
list_without_duplicates = []
# We use a while loop with a counter
i = 0
while i < len(list_with_duplicates):
if list_with_duplicates[i] not in list_without_duplicates:
list_without_duplicates.append(list_with_duplicates[i])
i += 1
print(list_without_duplicates)
# Output: [1, 2, 3, 4, 5, 6, 7]
In this example, we iterate over the list using a counter i
, which increases after each iteration. We achieve the same result as the for
loop example but in a slightly different way. This method could be beneficial for those trying to get familiar with multiple ways of creating loops.
However, another factor to consider is the preservation of the order of elements. Like the for loop, this while loop also preserves the order, which is a significant advantage for some use cases.
Advanced Duplicate Removal Methods
While the loop method provides a basic solution to remove duplicates from a list, Python offers more advanced techniques that accomplish this task with greater efficiency.
Let’s explore two of these methods: the set data type and list comprehension.
Set Data Type
A set, a built-in data type in Python, has unique characteristics that make it ideal for our task. A set automatically eliminates any duplicate elements!
Here’s how you can use it to remove duplicates from a list:
# Here is our list with duplicates
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5, 6, 6, 7]
# We convert the list to a set, which removes duplicates
list_without_duplicates = list(set(list_with_duplicates))
print(list_without_duplicates)
# Output: [1, 2, 3, 4, 5, 6, 7]
This method is very efficient, with a “time complexity” of O(n), making it significantly faster than the loop method for larger lists. However, a crucial drawback of using sets is their inability to preserve the order of elements.
List Comprehension
List comprehension offers a concise way to create lists based on existing lists.
Here’s how you can use list comprehension to remove duplicates:
# Here is our list with duplicates
list_with_duplicates = [1, 2, 2, 3, 4, 4, 5, 6, 6, 7]
# We use list comprehension to remove duplicates
list_without_duplicates = [i for n, i in enumerate(list_with_duplicates) if i not in list_with_duplicates[:n]]
print(list_without_duplicates)
# Output: [1, 2, 3, 4, 5, 6, 7]
This method retains the order of elements and is faster than the loop method. However, it’s not nearly as efficient as the set method, as, like the loop method, it has a “time complexity” of O(n^2).
Troubleshooting and Considerations
While the process of removing duplicates from Python lists can be straightforward, you might stumble upon some common errors or considerations. Let’s dive into these and offer some solutions and workarounds.
TypeError
A common error you might encounter when removing duplicates is a TypeError
. This error typically surfaces when you attempt to remove duplicates from a list containing unhashable items, such as lists or dictionaries. Python sets require their items to be hashable, and trying to convert a list with unhashable items to a set will result in a TypeError
.
Example of a TypeError:
# Here is our list with unhashable items
list_with_unhashables = [[1, 2], [1, 2], [3, 4]]
# Attempting to convert this to a set will result in a TypeError
list_without_duplicates = list(set(list_with_unhashables))
print(list_without_duplicates)
# Output: TypeError: unhashable type: 'list'
To troubleshoot this, ensure all items in your list are hashable before attempting to remove duplicates using the set method.
Example of handling nested lists:
# Here is our list of lists
list_with_duplicates = [[1, 2], [1, 2], [3, 4]]
# We convert the inner lists to tuples
list_with_tuples = [tuple(i) for i in list_with_duplicates]
# Now we can convert this to a set to remove duplicates
list_without_duplicates = list(set(list_with_tuples))
print(list_without_duplicates)
# Output: [(1, 2), (3, 4)]
Preserving Order
As we’ve previously discussed, some methods of removing duplicates do not preserve the order of elements. If the order of elements in your list is significant, you’ll need to use a method that preserves order, like the loop method or list comprehension.
Nested Lists
Nested lists can also present a challenge when removing duplicates. Since lists are unhashable, trying to remove duplicates from a list of lists using the set method will result in a TypeError
.
To handle this, you can convert your inner lists to tuples, which are hashable, before using the set method.
Here’s how you can remove duplicates from a list of lists by converting them into tuples:
# This is your initial list of lists
list_of_lists = [[1, 2], [2, 3], [1, 2], [3, 4], [2, 3]]
# Convert each inner list to a tuple. set() will now be able to process them, removing duplicates
list_without_duplicates = list(set(tuple(i) for i in list_of_lists))
# If you want to convert your tuples back into lists
final_output = [list(i) for i in list_without_duplicates]
print(final_output) # Output: [[3, 4], [2, 3], [1, 2]]
In this example, we convert each inner list to a tuple to remove duplicates using set() and then convert them back to lists. The final output is a list of lists without duplicates.
Understanding Python Lists
Now that you’ve gotten the low down on removing duplicates from lists, some of you may appreciate some information on Python lists in general.
What are Lists in Python?
A list is a built-in Python data type that stores multiple items in a single variable. Lists, along with Tuple, Set, and Dictionary, are four built-in data types in Python used to store collections of data. Lists are created by enclosing all the items (which can be of different data types) inside square brackets []
, separated by commas.
For more information on Dictionaries, you can view our detailed guide here!
The Principle of List Mutability
One of the defining features of lists in Python is their mutability. This means that we can modify, add, and remove items in a list after its creation. The mutability of lists is what facilitates the removal of duplicates.
Example of list mutability:
# Here is our list
list = [1, 2, 3]
print('Original list:', list)
# Modifying the list
list[0] = 'a'
list.append(4)
print('Modified list:', list)
# Output:
# Original list: [1, 2, 3]
# Modified list: ['a', 2, 3, 4]
We can iterate over the list and expunge any elements that occur more than once.
Going Beyond Python Lists
While mastering the removal of duplicates from lists is a valuable skill, it’s just the beginning of what Python programming has to offer.
Let’s expand our scope and delve into other data types in Python, common operations on lists, and the importance of comprehending Python’s data structures.
Python’s Other Data Types
Python supports a plethora of data types besides lists, which can be utilized to store and manipulate data. These include integers, floats, strings, tuples, sets, and dictionaries. Each of these data types possesses unique properties and applications. For instance, unlike lists, tuples are immutable, meaning they can’t be altered once they’re created. Sets, similar to lists, can be modified, but they only permit unique elements.
Common Operations on Lists
The removal of duplicates is merely one of the many operations you can execute on Python lists. Other common operations encompass adding and removing elements, sorting lists, determining the length of a list, and more. These operations render lists incredibly versatile and useful across a wide spectrum of programming tasks.
Further Resources for Python
If you’re interested in learning more ways to utilize the Python language, here are a few resources that you might find helpful:
- Exploring Python Lists in Depth: Dive deep into the functionality and versatility of Python lists with this comprehensive exploration.
IOFlood’s Python Guide: Looping Through a List: This guide explains how to loop through a list in Python, covering different methods like using a for loop, list comprehension, and the enumerate() function.
IOFlood’s Python Guide: Removing an Item from a List: This guide provides various ways to remove an item from a list in Python, including using the remove() method, list comprehension, and the del keyword.
Python: Ways to Remove Duplicates from a List – GeeksforGeeks: This GeeksforGeeks article provides different approaches and techniques to remove duplicates from a list in Python.
Python How To Remove Duplicates from a List – w3schools.com: w3schools.com explains various methods to remove duplicates from a list in Python, along with examples.
Python How to Remove Duplicates from a List – Guru99: Guru99 provides a tutorial that illustrates different ways to remove duplicates from a list in Python and provides sample code for each method.
Wrapping Up: List Manipulation
This comprehensive guide has equipped you with various methods to remove duplicates from Python lists.
We began with the fundamental loop method, an understandable and effective technique. We then expanded into more advanced techniques, including the set data type and list comprehension, which provide more efficiency and are capable of handling larger lists.
We also navigated through some common errors and considerations, such as the management of unhashable items and the preservation of element order, offering solutions and workarounds for these challenges.
We underlined the importance of selecting the right method based on your specific needs, whether you’re dealing with extensive lists, need to maintain order, or are working with nested lists.
Method | Efficiency | Preserves Order | Handles Nested Lists |
---|---|---|---|
Loop | O(n^2) | Yes | Yes |
Set | O(n) | No | No |
List Comprehension | O(n^2) | Yes | Yes |
In addition, we took a step back to comprehend what lists are in Python, their importance, and how they fit into the broader context of Python’s data structures. We explored other data types in Python, common operations on lists, and the importance of understanding Python’s data structures for effective programming.
Removing duplicates from lists is a prevalent task in Python programming, especially in data cleaning. By mastering these methods, you not only become more proficient in Python but also gain the skills to handle real-world data more effectively. So keep practicing, keep exploring, and soon you’ll be an expert at making your Python lists duplicate-free!