Python List Methods: Guide (With Examples)
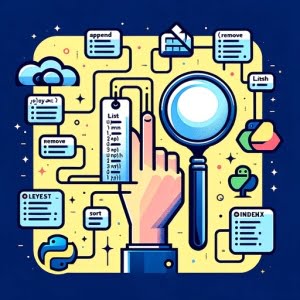
As we work on improving our data processing scripts at IOFLOOD, we have utilized various Python list methods as they allow for various operations such as appending, removing, and searching items. Python list methods offer a comprehensive set of tools to handle list operations efficiently and should be known by any Python Programmer. Today’s article explores what Python list methods are and how to use them, providing insights and examples to help our dedicated server customers.
In this guide, we’ll walk you through all the Python list methods, from the basics to more advanced techniques. We’ll cover everything from the append()
, extend()
, to more complex methods like index()
, reverse()
, and clear()
.
Let’s get started!
TL;DR: What are the Python List Methods?
Python lists come with several built-in methods that allow you to manipulate and work with your data effectively. These methods include
append()
,extend()
,insert()
,remove()
,pop()
,index()
,count()
,sort()
,reverse()
, andclear()
. Each of these methods serves a specific purpose and use.
Here’s a simple example of using some of these methods:
my_list = [1, 2, 3, 4]
my_list.append(5)
my_list.insert(0, 0)
my_list.remove(3)
last_item = my_list.pop()
print(my_list)
print(last_item)
# Output:
# [0, 1, 2, 4]
# 5
In this example, we first create a list my_list
with four elements. We then use the append()
method to add the number 5 to the end of the list, and the insert()
method to add the number 0 at the beginning. We remove the number 3 using the remove()
method, and finally, we use the pop()
method to remove and return the last item in the list. The final list is [0, 1, 2, 4]
, and the last item popped is 5
.
This is just a basic introduction to Python list methods. Continue reading for more detailed explanations and examples of each method.
Table of Contents
Mastering Basic Python List Methods
Let’s start with the basics. Python lists come with several built-in methods that are fundamental to data manipulation. We’ll discuss five of these methods: append()
, extend()
, insert()
, remove()
, and pop()
.
The Append Method
The append()
method allows you to add an item to the end of a list.
numbers = [1, 2, 3]
numbers.append(4)
print(numbers)
# Output:
# [1, 2, 3, 4]
In this example, we’ve appended the integer 4
to the list numbers
. The updated list now includes 4
at the end.
The Extend Method
The extend()
method is used to add multiple items to a list.
numbers = [1, 2, 3]
numbers.extend([4, 5, 6])
print(numbers)
# Output:
# [1, 2, 3, 4, 5, 6]
Here, we’ve extended numbers
by adding a list of three integers. The numbers
list now includes these additional elements.
The Insert Method
The insert()
method allows you to add an item at a specific position in the list.
numbers = [1, 3, 4]
numbers.insert(1, 2) # Insert number 2 at index 1
print(numbers)
# Output:
# [1, 2, 3, 4]
In this example, we’ve inserted the integer 2
at index 1
of the numbers
list. The list now includes 2
in the correct numerical sequence.
The Remove Method
The remove()
method is used to remove the first occurrence of a specified item from the list.
numbers = [1, 2, 3, 2, 4]
numbers.remove(2) # Remove the first occurrence of 2
print(numbers)
# Output:
# [1, 3, 2, 4]
Here, we’ve removed the first occurrence of 2
from the numbers
list. The list now only includes the second occurrence of 2
.
The Pop Method
Finally, the pop()
method removes and returns the item at a given position in the list. If no index is specified, it removes and returns the last item in the list.
numbers = [1, 2, 3, 4]
last_item = numbers.pop()
print(last_item)
print(numbers)
# Output:
# 4
# [1, 2, 3]
In this example, we’ve popped the last item from the numbers
list. The pop()
method returns 4
, and the updated list no longer includes this number.
Advanced Python List Methods
Now that we’ve covered the basics, let’s dive into some more advanced Python list methods. We’ll explore index()
, count()
, sort()
, reverse()
, and clear()
.
The Index Method
The index()
method returns the position of the first occurrence of a specified value in the list.
numbers = [1, 2, 3, 2, 4]
index = numbers.index(2)
print(index)
# Output:
# 1
In this example, we find the index of the first occurrence of 2
in the numbers
list. The index()
method returns 1
, indicating that 2
appears first at index 1
.
The Count Method
The count()
method returns the number of times a specified value appears in the list.
numbers = [1, 2, 3, 2, 4]
count = numbers.count(2)
print(count)
# Output:
# 2
Here, we count the number of occurrences of 2
in the numbers
list. The count()
method returns 2
, indicating that 2
appears twice in the list.
The Sort Method
The sort()
method sorts the items in the list in ascending order by default. You can also sort items in descending order by passing reverse=True
as an argument to the sort()
method.
numbers = [4, 2, 3, 1, 5]
numbers.sort()
print(numbers)
# Output:
# [1, 2, 3, 4, 5]
In this example, we sort the numbers
list in ascending order. The sort()
method rearranges the list so that the numbers are in ascending order from 1
to 5
.
The Reverse Method
The reverse()
method reverses the order of the items in the list.
numbers = [1, 2, 3, 4]
numbers.reverse()
print(numbers)
# Output:
# [4, 3, 2, 1]
Here, we reverse the order of the numbers
list. The reverse()
method modifies the list so that the numbers are in descending order from 4
to 1
.
The Clear Method
Finally, the clear()
method removes all items from the list.
numbers = [1, 2, 3, 4]
numbers.clear()
print(numbers)
# Output:
# []
In this example, we clear all items from the numbers
list. The clear()
method empties the list, resulting in an empty list []
.
Other Methods for List Manipulation
While Python list methods offer a direct way to manipulate lists, Python also provides alternative techniques that can be more efficient or suitable in certain scenarios. These include list comprehensions, lambda functions, and the use of third-party libraries like NumPy.
List Comprehensions
List comprehensions provide a concise way to create lists based on existing lists. Here’s an example where we create a new list containing the squares of all numbers in an existing list:
numbers = [1, 2, 3, 4]
squares = [number**2 for number in numbers]
print(squares)
# Output:
# [1, 4, 9, 16]
In this example, we create a new list squares
where each element is the square of the corresponding element in numbers
. The resulting list squares
contains the squares of all numbers from numbers
.
Lambda Functions
Lambda functions can be used along with Python’s built-in map()
function to apply a function to all items in a list. Here’s an example where we use a lambda function to square all numbers in a list:
numbers = [1, 2, 3, 4]
squares = list(map(lambda number: number**2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16]
In this example, we use a lambda function to square each number in numbers
. The map()
function applies this lambda function to all items in numbers
, resulting in a new list squares
containing the squares of all numbers.
NumPy Library
NumPy is a powerful third-party library for numerical operations in Python. It provides an array object that is more efficient and convenient for numerical operations than Python lists. Here’s an example where we use a NumPy array to perform element-wise multiplication:
import numpy as np
numbers = np.array([1, 2, 3, 4])
doubles = numbers * 2
print(doubles)
# Output:
# array([2, 4, 6, 8])
In this example, we create a NumPy array numbers
and multiply it by 2
. NumPy performs element-wise multiplication, resulting in a new array doubles
where each element is twice the corresponding element in numbers
.
Solving Errors: Python List Methods
While Python list methods are powerful tools, they can sometimes lead to common errors if not used correctly. Let’s discuss some of these issues and how to resolve them.
Type Errors
A TypeError
often occurs when you try to use a list method on a non-list object. Here’s an example:
non_list = 'I am not a list'
non_list.append('But I try to behave like one')
# Output:
# AttributeError: 'str' object has no attribute 'append'
In this example, we try to use the append()
list method on a string, which results in an AttributeError
because strings do not have an append()
method. To fix this, make sure to use list methods only on list objects.
Index Errors
An IndexError
occurs when you try to access a list item at an index that does not exist. Here’s an example:
numbers = [1, 2, 3, 4]
print(numbers[4])
# Output:
# IndexError: list index out of range
In this example, we try to access the item at index 4
in a list that only has four items (indices 0
to 3
). This results in an IndexError
. To fix this, always ensure that the index you’re trying to access exists in the list.
Value Errors
A ValueError
occurs when you try to remove a value from a list that does not exist. Here’s an example:
numbers = [1, 2, 3, 4]
numbers.remove(5)
# Output:
# ValueError: list.remove(x): x not in list
In this example, we try to remove the number 5
from a list that does not contain 5
. This results in a ValueError
. To fix this, always ensure that the value you’re trying to remove exists in the list.
Understanding these common issues can help you avoid errors and use Python list methods more effectively.
The Significance of Python Lists
Before we delve into the more complex aspects of Python list methods, it’s essential to understand what Python lists are, how they work, and why they are an important part of Python programming.
What are Python Lists?
In Python, a list is a built-in data structure that can hold a collection of items. These items can be of various types, including integers, strings, and even other lists. Here’s an example of a Python list:
my_list = ['apple', 'banana', 'cherry']
print(my_list)
# Output:
# ['apple', 'banana', 'cherry']
In this example, my_list
is a Python list that contains three strings. The list is defined by square brackets []
, and its items are separated by commas.
How Do Python Lists Work?
Python lists are ordered, which means that the items in a list have a defined order that will not change unless you do so explicitly. Each item in a list is assigned a unique index based on its position, starting from 0
for the first item.
Why are Python Lists Important?
Python lists are one of the most commonly used data structures in Python programming due to their versatility. They can hold any type of data, they are mutable (meaning you can change their content), and they come with several built-in methods for easy data manipulation.
The Concept of Mutability in Python Lists
In Python, objects are either mutable or immutable. Mutable objects can change their content without changing their identity, while immutable objects cannot. Python lists are mutable, which means you can modify a list by adding, removing, or changing its items.
Here’s an example of list mutability:
my_list = ['apple', 'banana', 'cherry']
my_list[1] = 'blueberry'
print(my_list)
# Output:
# ['apple', 'blueberry', 'cherry']
In this example, we change the second item of my_list
from 'banana'
to 'blueberry'
. The my_list
object maintains its identity, but its content changes.
Understanding the concept of mutability is important when working with Python lists, as it allows for efficient in-place modifications.
Python List Methods in the Real World
Python list methods are not just theoretical concepts to be learned; they are practical tools that you will use in many real-world applications. Let’s explore how Python list methods can be used in larger projects and discuss some related topics you might want to explore further.
Python List Methods in Data Analysis
In data analysis, Python list methods are often used to clean and prepare data for analysis. For instance, you might use the append()
and extend()
methods to collect data from multiple sources, the sort()
method to order your data for easier analysis, and the count()
method to summarize your data.
# Example: Collecting and summarizing data
# Collect data from multiple sources
data = []
data.append([1, 2, 3]) # Data from source 1
data.append([4, 5, 6]) # Data from source 2
# Flatten the list
flat_data = []
for sublist in data:
flat_data.extend(sublist)
# Summarize the data
count = flat_data.count(1) # Count occurrences of 1
print(count)
# Output:
# 1
In this example, we collect data from two sources into a list of lists, flatten the list using the extend()
method, and then count the occurrences of the number 1
using the count()
method.
Python List Methods in Web Development
In web development, Python list methods can be used to manage user data, manipulate URLs, and more. For example, you might use the insert()
and pop()
methods to add and remove items from a list of user sessions, or the sort()
method to order a list of URLs.
# Example: Managing user sessions
sessions = [] # List of active user sessions
# A new user logs in
sessions.append('User1')
# Another user logs in
sessions.append('User2')
# The first user logs out
sessions.remove('User1')
print(sessions)
# Output:
# ['User2']
In this example, we manage a list of active user sessions using the append()
and remove()
methods. When a user logs in, their username is added to the list, and when they log out, their username is removed.
Further Resources for Python List Mastery
To further your understanding of Python lists and their related concepts, consider exploring the following resources:
- Beginner’s Guide to Python Lists: A comprehensive guide that introduces Python lists and provides essential tips for beginners.
Introduction to Python List Comprehension: This guide provides an introduction to list comprehension in Python, a concise and powerful way to create lists based on existing lists or other iterable objects.
Understanding the Python List extend() Method with Usage and Examples: This tutorial explains how to use the extend() method in Python to add multiple elements to an existing list, providing examples and use cases for a better understanding.
Python’s Official Documentation on Data Structures: This is a comprehensive resource on Python’s built-in data structures, including lists, tuples, sets, and dictionaries.
Real Python’s Guide on Python Lists: This guide provides an in-depth look at Python lists and tuples, with plenty of examples and exercises.
Geeks for Geeks Python Data Types: This article covers all of Python’s built-in data types, including lists, and provides a good foundation for understanding how different data types can be used in Python.
Wrapping Up: Python List Methods
In this comprehensive guide, we’ve journeyed through the world of Python list methods, a crucial part of effective data manipulation in Python.
We began with the basics, understanding how to use simple list methods like append()
, extend()
, insert()
, remove()
, and pop()
. We then advanced to more complex methods such as index()
, count()
, sort()
, reverse()
, and clear()
. Each method was explained with practical code examples, showcasing their functionality and use cases.
Along the way, we tackled common issues you might encounter when using Python list methods, such as TypeError
, IndexError
, and ValueError
, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to list manipulation, such as list comprehensions, lambda functions, and the use of third-party libraries like NumPy. This gave us a broader perspective on the various ways we can manipulate lists in Python.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Python List Methods | Direct, built-in methods for list manipulation | May require troubleshooting for some issues |
List Comprehensions | Concise way to create lists based on existing lists | Can be more complex for beginners |
Lambda Functions | Offers flexibility in applying functions to list items | Requires understanding of functional programming |
NumPy Library | Efficient and convenient for numerical operations | Requires installation of an external library |
Whether you’re a beginner just starting out with Python or an experienced developer looking to deepen your understanding of Python list methods, we hope this guide has been a valuable resource.
With a solid grasp of these methods, you’re now better equipped to handle data manipulation tasks in Python. Happy coding!