Python Reverse List: Complete Guide (With Examples)
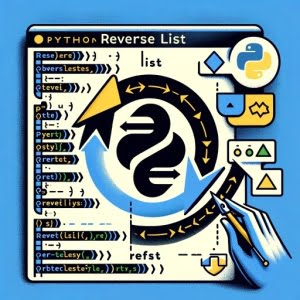
While working to automate our data analysis programs at IOFLOOD, we had the pleasure of becoming familiar with the methods of reversing lists in Python. Although it is a basic task, list reversal plays a significant role in data processing as it ensures that data is organized in the desired sequence. This article details the methods we utilize for reversing lists in Python, sharing our tips and processes to assist our customers looking to utilize dependable data manipulation practices on their dedicated cloud servers.
This guide will walk you through the process of reversing a list in Python, from the basics to more advanced techniques. We’ll cover everything from the reverse()
method, slicing technique, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Reverse a List in Python?
You can reverse a list in Python using the
reverse()
method, with the syntaxmy_list.reverse().
You can also use the[::-1]
slicing technique. These are built-in features of Python that make list reversal a breeze.
Here’s a simple example:
my_list = [1, 2, 3]
my_list.reverse()
print(my_list)
# Output:
# [3, 2, 1]
In this example, we have a list named my_list
with the elements 1, 2, and 3. We use the reverse()
method on my_list
, which reverses the order of the elements in the list. When we print my_list
, we see that the elements are now in reverse order.
This is just the tip of the iceberg when it comes to reversing lists in Python. Continue reading for more detailed explanations, advanced usage scenarios, and alternative approaches.
Table of Contents
The Basics: Reversing a List in Python
Python provides several ways to reverse a list. Here, we’ll discuss two of the most straightforward methods: the reverse()
method and the slicing technique.
The reverse()
Method
The reverse()
method is a built-in Python method that directly modifies the original list. It doesn’t return any value (or more technically, it returns None
). Here’s how it works:
my_list = ['apple', 'banana', 'cherry']
my_list.reverse()
print(my_list)
# Output:
# ['cherry', 'banana', 'apple']
In this example, we have a list of fruits. The reverse()
method reverses the order of the elements in the original list. When we print the list, we see the fruits in reverse order.
One thing to note about the reverse()
method is that it alters the original list. If you need to keep the original list unchanged, this method might not be the best choice.
The Slicing Technique
Python’s slicing technique can also be used to reverse a list. This method does not modify the original list, but instead returns a new list that is a reversed version of the original list.
Here’s an example of how to reverse a list using slicing:
my_list = ['apple', 'banana', 'cherry']
reversed_list = my_list[::-1]
print(reversed_list)
# Output:
# ['cherry', 'banana', 'apple']
In this example, [::-1]
is a slice that starts at the end of the string, ends at position 0, and moves with the step -1 (which means one step backwards).
Unlike the reverse()
method, slicing doesn’t alter the original list. This can be particularly useful when you want to keep the original list intact.
Advanced List Reversal Methods
Python’s flexibility allows lists to contain various data types and even nested lists. Let’s explore how we can reverse these complex lists.
Reversing Lists with Different Data Types
In Python, a list can contain different data types, such as integers, strings, and even other lists. Both the reverse()
method and the slicing technique can handle these mixed data type lists.
Here’s an example:
mixed_list = [1, 'apple', 3.14, ['a', 'b', 'c']]
mixed_list.reverse()
print(mixed_list)
# Output:
# [['a', 'b', 'c'], 3.14, 'apple', 1]
In this example, mixed_list
contains a float, a string, an integer, and a nested list. After applying the reverse()
method, we get the elements in reverse order, regardless of their data type.
Reversing Nested Lists
A nested list is a list within a list. When you reverse a list that contains nested lists, Python treats the nested lists as single elements. To reverse the elements within the nested lists, you’ll need to apply the reversal method to each nested list separately.
Here’s an example:
nested_list = [[1, 2, 3], ['apple', 'banana', 'cherry']]
# Reverse the main list
nested_list.reverse()
# Reverse each nested list
for sublist in nested_list:
sublist.reverse()
print(nested_list)
# Output:
# [['cherry', 'banana', 'apple'], [3, 2, 1]]
In this example, nested_list
contains two lists. After reversing the main list and each nested list separately, we get the elements in the main list and the nested lists in reverse order.
Alternate Ways to Reverse Python List
Apart from the reverse()
method and slicing technique, Python offers other ways to reverse a list, such as using the reversed()
function and leveraging third-party libraries. Let’s delve into these alternatives.
The reversed()
Function
Unlike the reverse()
method, the reversed()
function doesn’t modify the original list. Instead, it returns a reverse iterator which we can convert back to a list using the list()
function.
Here’s an example:
my_list = ['apple', 'banana', 'cherry']
reversed_list = list(reversed(my_list))
print(reversed_list)
# Output:
# ['cherry', 'banana', 'apple']
In this example, the reversed()
function returns a reverse iterator of my_list
, and list()
converts this iterator into a list. This approach doesn’t alter the original list, making it a viable option when you need the original list to remain unchanged.
Leveraging Third-Party Libraries
Python’s extensive ecosystem includes numerous third-party libraries that offer additional ways to reverse a list. For example, the NumPy library, commonly used for scientific computing, provides the flip()
function for reversing arrays (which can also handle Python lists).
Here’s an example using NumPy:
import numpy as np
my_list = ['apple', 'banana', 'cherry']
reversed_list = np.flip(my_list).tolist()
print(reversed_list)
# Output:
# ['cherry', 'banana', 'apple']
In this example, we import the NumPy library and use its flip()
function to reverse my_list
. The tolist()
function then converts the NumPy array back to a Python list. This approach can be particularly useful in data science and machine learning applications where NumPy is commonly used.
Troubleshooting Python List Reversal
While reversing a list in Python is typically straightforward, you may encounter some issues, especially when dealing with complex data structures or incompatible data types. Let’s discuss some common problems and their solutions.
Type Errors with Incompatible Data Types
When working with lists that contain different data types, you may encounter a TypeError if you try to perform operations that are not supported by one of the data types in the list.
For example, if you try to use the sort()
method before reversing a list that contains both strings and integers, you’ll encounter a TypeError because Python doesn’t know how to compare these different data types.
Here’s an example:
mixed_list = [1, 'apple', 3.14, 'banana']
mixed_list.sort()
# Output:
# TypeError: '<' not supported between instances of 'str' and 'int'
In this case, you should either ensure that the list contains only compatible data types before sorting or manage the TypeError with a try/except handling block.
Reversing a List in Place
As we discussed earlier, the reverse()
method reverses a list in place, meaning it modifies the original list. If you need the original list to remain unchanged, you should use an alternative method like slicing or the reversed()
function.
Here’s an example of reversing a list without modifying the original list using slicing:
my_list = ['apple', 'banana', 'cherry']
reversed_list = my_list[::-1]
print('Original list:', my_list)
print('Reversed list:', reversed_list)
# Output:
# Original list: ['apple', 'banana', 'cherry']
# Reversed list: ['cherry', 'banana', 'apple']
In this example, the original list remains unchanged, and a new list is created for the reversed version.
By understanding these potential issues and how to handle them, you can reverse lists in Python more effectively and avoid common pitfalls.
Deep Dive: Python Lists and Mutability
Before we delve further into reversing lists, it’s crucial to understand Python’s list data type and its methods. Moreover, the concept of mutability plays a significant role in how we manipulate lists in Python.
Understanding Python Lists
In Python, a list is a collection of items that are ordered and changeable. It allows duplicate members and can contain items of any data type. You can create a list by enclosing a comma-separated sequence of items in square brackets []
.
Here’s a simple example:
my_list = ['apple', 'banana', 'cherry']
print(my_list)
# Output:
# ['apple', 'banana', 'cherry']
In this example, my_list
is a list that contains three strings. When we print my_list
, we see the items in the order they were added.
Python provides several built-in methods for manipulating lists, such as append()
to add an item, remove()
to remove an item, and sort()
to sort the items. As we’ve seen, the reverse()
method is another built-in list method that reverses the order of items.
The Concept of Mutability in Python
In Python, objects are either mutable or immutable. Mutable objects can change their state or contents, while immutable objects cannot. Lists in Python are mutable, which means we can change their content without changing their identity.
For example, when we use the reverse()
method, we’re changing the state of the list (i.e., the order of its items), but the list itself remains the same object.
Here’s an example to illustrate this:
my_list = ['apple', 'banana', 'cherry']
print('Before:', my_list, id(my_list))
my_list.reverse()
print('After:', my_list, id(my_list))
# Output:
# Before: ['apple', 'banana', 'cherry'] 140735889619904
# After: ['cherry', 'banana', 'apple'] 140735889619904
In this example, my_list
is reversed using the reverse()
method. We print the list and its id (a unique identifier for the object) before and after reversing. As you can see, the id remains the same, indicating that it’s the same object, even though its state has changed.
Understanding Python’s list data type, its built-in methods, and the concept of mutability is fundamental to effectively reversing lists and manipulating them in other ways.
Use Cases of Reversing Lists
Reversing a list might seem like a simple task, but it’s a fundamental operation that’s deeply connected to other areas of programming and data manipulation. Let’s explore some of these connections.
List Reversal in Sorting Algorithms
Reversing a list is a common step in many sorting algorithms. For example, in the bubble sort algorithm, reversing the order of a list can be used to sort the list in descending order. Understanding how to reverse a list in Python can therefore improve your understanding and implementation of these algorithms.
Data Analysis and List Reversal
In data analysis, you often need to manipulate data stored in lists or similar data structures. Reversing the order of a list can help you analyze trends over time, compare the latest data points with earlier ones, or prepare your data for specific types of visualizations.
Exploring Related Concepts
Once you’ve mastered reversing a list in Python, you can explore related concepts like lambda functions and list comprehension. List comprehension is a concise way to create lists based on existing lists, while lambda functions can help you create anonymous functions on the fly.
For example, you could use list comprehension and a lambda function to create a reversed copy of a list, like this:
my_list = [1, 2, 3]
reversed_list = [x for x in sorted(my_list, key=lambda x: -x)]
print(reversed_list)
# Output:
# [3, 2, 1]
In this example, the sorted()
function, combined with a lambda function, sorts the list in descending order, and list comprehension creates a new list from the sorted list.
Further Resources for Python List Mastery
If you want to delve deeper into Python lists and related topics, here are some resources that you might find helpful:
- Effective Data Management with Python Lists: Learn how to efficiently manage and manipulate data using Python lists, accompanied by practical examples and useful tips.
Calculating the Sum of Elements in a List using Python: Different approaches to calculate the sum of elements in a list with Python.
Finding the Length of a List in Python: A tutorial on the different methods to determine the length of a list in Python.
Python Lists and List Manipulation: A comprehensive guide on Python lists from DataCamp.
Python Lambda Functions: An in-depth tutorial on lambda functions in Python from Real Python.
Python List Comprehension: A detailed explanation of list comprehension in Python from Programiz.
Wrapping Up: Python List Reversal
In this comprehensive guide, we’ve delved into the process of reversing a list in Python, covering everything from the basics to more advanced techniques.
We began with the fundamentals, exploring how to reverse a list using the reverse()
method and the slicing technique. We then ventured into more advanced territory, discussing how to handle lists with different data types and nested lists. Along the way, we tackled common issues you might encounter when reversing a list, providing you with solutions and workarounds for each problem.
We also explored alternative approaches to reversing a list, such as using the reversed()
function and third-party libraries like NumPy. Here’s a quick comparison of these methods:
Method | Changes Original List | Returns New List |
---|---|---|
reverse() method | Yes | No |
Slicing technique | No | Yes |
reversed() function | No | Yes |
NumPy flip() function | No | Yes |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your list manipulation skills, we hope this guide has given you a deeper understanding of how to reverse a list in Python and the power of this operation. Happy coding!