Python Pandas iloc: Guide to Integer-location Indexing
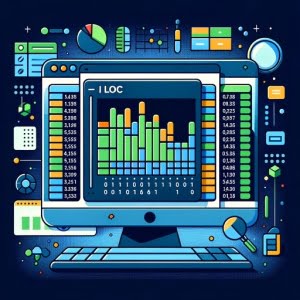
Struggling with data selection in pandas? You’re not alone. Data selection is a crucial part of data analysis, and it can often be a stumbling block, especially for beginners. But don’t worry, help is at hand.
Like a precise GPS, the pandas iloc function lets you navigate your data by integer-location. It’s a powerful tool that can make your data selection tasks a breeze.
This article will guide you through the ins and outs of using iloc in pandas, from basic usage to advanced techniques. So, buckle up and get ready to master data selection in pandas with iloc!
TL;DR: How Do I Use iloc in Pandas?
The pandas
iloc
function allows you to select data by its integer location, used with the syntaxdf.iloc[indexLocation]
. Here’s a simple example:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.iloc[1])
# Output:
# A 2
# B 5
# Name: 1, dtype: int64
In the above example, we first import the pandas library and create a DataFrame ‘df’ with two columns ‘A’ and ‘B’. We then use the iloc function to select the second row (index 1) of the DataFrame. The output shows the values of ‘A’ and ‘B’ in the selected row.
Continue reading for a more detailed understanding and advanced usage scenarios of pandas iloc.
Table of Contents
Understanding the Basics of ‘.iloc[]’
The pandas iloc function is a versatile tool that allows you to select data by its integer location in a DataFrame. iloc stands for integer location so that should help with remembering what it does.
Let’s start by understanding its basic usage with a simple example.
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df.iloc[1])
# Output:
# A 2
# B 5
# Name: 1, dtype: int64
In this example, we first create a pandas DataFrame ‘df’ with two columns ‘A’ and ‘B’. We then use the iloc function to select the second row (index 1) of the DataFrame. The output shows the values of ‘A’ and ‘B’ in the selected row.
Advantages of Using iloc
Pandas iloc is efficient and versatile. It allows you to select data based on its position in the DataFrame, regardless of the index labels. This makes it a powerful tool for data selection, especially when dealing with large datasets.
Potential Pitfalls of Using iloc
While iloc is a powerful tool, it’s important to remember that it works based on the integer location. This means that if your DataFrame’s index labels are not integers, or if they are not in a sequential order, you might end up selecting the wrong data. It’s always a good idea to double-check your index labels before using iloc.
Leveraging .iloc[] for Larger Datasets
As you become more comfortable with pandas iloc, you’ll find that it’s an incredibly powerful tool when dealing with larger datasets. Let’s delve into some of the advanced techniques that can make your data selection tasks even easier.
Slicing Data with iloc
One of the key features of iloc is the ability to slice the data. This allows you to select multiple rows or columns at once. Here’s how you can do it:
import pandas as pd
df = pd.DataFrame({'A': range(1, 6), 'B': range(6, 11), 'C': range(11, 16)})
print(df.iloc[1:4, 0:2])
# Output:
# A B
# 1 2 7
# 2 3 8
# 3 4 9
In this example, we’re slicing the DataFrame to select the second to fourth rows and the first two columns. The output shows the selected rows and columns.
Using Conditional Statements with iloc
Pandas iloc also allows you to use conditional statements to select data. This can be particularly useful when you want to select data based on certain conditions. Here’s an example:
import pandas as pd
df = pd.DataFrame({'A': range(1, 6), 'B': range(6, 11), 'C': range(11, 16)})
print(df.iloc[df['A'] > 3])
# Output:
# A B C
# 3 4 9 14
# 4 5 10 15
In this example, we’re using a conditional statement to select the rows where the value in column ‘A’ is greater than 3. The output shows the selected rows.
Best Practices for Using iloc
When using iloc, it’s important to remember that it’s based on the integer location. Always double-check your indexes and make sure they are in the correct order. Also, remember that Python uses zero-based indexing, so the first row or column is at index 0.
Alternative Data Selection Methods
While pandas iloc is a powerful tool for data selection, it’s not the only method available. Let’s explore a couple of alternative approaches that you might find useful.
Using loc for Label-Based Indexing
The pandas loc function allows you to select data based on its label. This can be particularly useful when your DataFrame’s index labels are meaningful and not just sequential integers.
Here’s an example of how you can use loc:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]}, index=['x', 'y', 'z'])
print(df.loc['y'])
# Output:
# A 2
# B 5
# Name: y, dtype: int64
In this example, we first create a DataFrame with two columns ‘A’ and ‘B’, and the index labels are ‘x’, ‘y’, and ‘z’. We then use the loc function to select the row labeled ‘y’.
Boolean Indexing for Condition-Based Selection
Boolean indexing allows you to select data based on certain conditions. This can be particularly useful when you want to filter your data.
Here’s an example of how you can use boolean indexing:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df[df['A'] > 1])
# Output:
# A B
# 1 2 5
# 2 3 6
In this example, we’re using boolean indexing to select the rows where the value in column ‘A’ is greater than 1.
Comparison Table of Data Selection Methods
Method | Advantages | Disadvantages |
---|---|---|
iloc | Efficient for large datasets, Allows slicing and conditional statements | Works only with integer-location |
loc | Works with label-location, Can be more intuitive if index labels are meaningful | Can be confusing if index labels are integers |
Boolean Indexing | Powerful for condition-based selection, Works with both labels and integer-locations | Can be more complex to implement |
While each method has its own strengths and weaknesses, they all provide powerful ways to select data in pandas. It’s recommended to choose the method that best suits your needs and the structure of your data.
While pandas iloc is a powerful tool for data selection, it’s not without its quirks. Let’s discuss some common issues you might encounter and how to work around them.
Dealing with ‘IndexError’
One common issue when using iloc is the ‘IndexError’. This usually happens when you try to select data at an index that doesn’t exist.
Here’s an example:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
try:
print(df.iloc[3])
except IndexError as e:
print(f'Error: {e}')
# Output:
# Error: single positional indexer is out-of-bounds
In this example, we’re trying to select data at an index that doesn’t exist in the DataFrame. Python raises an ‘IndexError’ with a message ‘single positional indexer is out-of-bounds’.
To avoid this error, always make sure that the index exists before trying to select data at that index.
Handling Non-Integer Indexes
Another common issue is trying to use iloc with non-integer indexes. Remember, iloc works based on the integer-location, not the index labels.
Here’s an example:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]}, index=['x', 'y', 'z'])
try:
print(df.iloc['x'])
except TypeError as e:
print(f'Error: {e}')
# Output:
# Error: cannot do label indexing on <class 'pandas.core.indexes.base.Index'> with these indexers [x] of <class 'str'>
In this example, we’re trying to use iloc with a non-integer indexer ‘x’. Python raises a ‘TypeError’ with a message indicating that we cannot use label indexing with iloc.
To avoid this error, always use integer-location with iloc, regardless of your DataFrame’s index labels.
Understanding the Concepts in Pandas
Before we dive deeper into the use of pandas iloc, it’s important to understand the fundamental concepts that underpin it. These are pandas DataFrame, Series, and indexing.
pandas DataFrame: The Foundation
A pandas DataFrame is a two-dimensional, size-mutable, heterogeneous tabular data structure that also contains axes labels (rows and columns). Think of it as a spreadsheet or SQL table, or a dictionary of Series objects. It’s generally the most commonly used pandas object.
Here’s a simple example of a DataFrame:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df)
# Output:
# A B
# 0 1 4
# 1 2 5
# 2 3 6
In this example, we create a DataFrame ‘df’ with two columns ‘A’ and ‘B’. The output shows the DataFrame with its default integer-location based index.
pandas Series: Single Column Data
A pandas Series is a one-dimensional labeled array capable of holding any data type. It’s essentially a single column in a DataFrame. Here’s how you can select a Series from a DataFrame:
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
print(df['A'])
# Output:
# 0 1
# 1 2
# 2 3
# Name: A, dtype: int64
In this example, we select the ‘A’ Series from the DataFrame. The output shows the Series with its values and the corresponding index.
Indexing in pandas: Navigating Your Data
Indexing in pandas is a way to label the rows and columns in your DataFrame. By default, pandas assigns integer labels starting from 0, but you can also set your own custom labels.
When using pandas iloc, we’re using integer-location based indexing. This means that we’re selecting data based on its integer position in the DataFrame, regardless of the index labels. It’s a powerful tool for data selection, but it’s important to remember that it works based on the integer-location, not the index labels.
Practical Uses of iloc with Datasets
Understanding and mastering the pandas iloc function is not just about selecting data in a DataFrame. It’s about opening the door to more advanced data analysis and data cleaning techniques. With iloc, you can efficiently navigate and manipulate your data, making it a fundamental tool in any data scientist’s toolkit.
Data Cleaning with iloc
Data cleaning, which involves correcting or removing errors in datasets, is a critical step in the data analysis process. With iloc, you can easily locate and modify specific data points based on their position, making it an effective tool for data cleaning.
Exploring Related Concepts
Once you’ve mastered data selection with iloc, you can explore related concepts like data visualization with pandas, machine learning, and more. For instance, you can use iloc to select specific data for your plots when visualizing data with pandas. In machine learning, iloc can be used to split your data into training and test sets.
Further Resources for Pandas Library
To further enhance your understanding of pandas and iloc, consider exploring online resources, tutorials, and documentation. Websites like Stack Overflow and the official pandas documentation are excellent places to start. Remember, practice is key when it comes to mastering any new skill, so don’t hesitate to experiment with different datasets and iloc operations.
We have a few resources on the Pandas Library right here on our blog that we hope you find helpful:
- Pandas for Beginners: A Comprehensive Introduction: Get started with Python Pandas with this beginner-friendly guide, covering all the basics you need to know.
Resetting Index in a Pandas DataFrame with reset_index(): This tutorial explains how to use the
reset_index()
function in Pandas to reset the index of a DataFrame in Python, allowing you to reorganize and clean your data for further analysis.Removing Duplicate Rows in a Pandas DataFrame using drop_duplicates(): This guide demonstrates how to utilize the
drop_duplicates()
function in Pandas to efficiently remove duplicate rows from a DataFrame in Python, helping you ensure data quality and integrity.pandas.DataFrame.iloc – pandas API Reference: Official documentation for the iloc attribute in Pandas DataFrame, explaining how to extract rows and columns using integer-based indexing.
Python – Extracting Rows using Pandas iloc[]: A GeeksforGeeks article that illustrates the usage of iloc[] in Pandas for extracting rows based on integer-based indexing.
Pandas DataFrame iloc[] Property: A tutorial on w3schools.com that introduces the iloc property in Pandas DataFrame, showcasing its usage for extracting specific rows or cells using integer-based indexing.
Recap: Mastering pandas iloc
Throughout this guide, we’ve explored the power of pandas iloc, a fundamental tool for data selection based on integer-location. From basic usage to advanced techniques, iloc serves as a precise GPS, helping you navigate your data efficiently.
We’ve also discussed common issues you might encounter while using iloc, such as ‘IndexError’ and problems with non-integer indexes, along with their solutions. Remember, iloc works based on integer-location, so always double-check your indexes and ensure they are in the correct order.
In addition to iloc, we’ve examined alternative data selection methods in pandas, including loc for label-based indexing and boolean indexing for condition-based selection. Each method has its own strengths and weaknesses, and it’s recommended to choose the one that best suits your needs and the structure of your data.
Here’s a quick comparison of the discussed methods:
Method | Advantages | Disadvantages |
---|---|---|
iloc | Efficient for large datasets, Allows slicing and conditional statements | Works only with integer-location |
loc | Works with label-location, Can be more intuitive if index labels are meaningful | Can be confusing if index labels are integers |
Boolean Indexing | Powerful for condition-based selection, Works with both labels and integer-locations | Can be more complex to implement |
Finally, we’ve touched upon the relevance of iloc in data analysis, data cleaning, and beyond, and suggested further resources for deeper understanding. Whether you’re a beginner or an experienced data analyst, mastering pandas iloc is a step forward in your data science journey.