Python Data Structures | Reference Guide
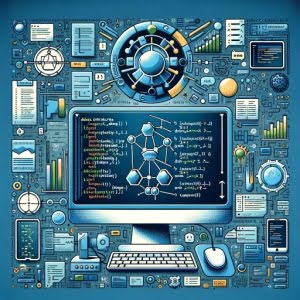
Do you find yourself grappling with Python data structures? You’re not alone. Python, akin to a well-stocked toolbox, offers a diverse range of data structures. Each of these structures is purpose-built for specific tasks, and understanding when and how to use them is key to becoming proficient in Python.
This guide is your companion on this journey. It will introduce you to Python’s built-in data structures, show you how they’re used, and share best practices. By the end of this guide, you’ll have a solid grasp of Python data structures, and you’ll be able to use them effectively in your coding projects.
TL;DR: What are the main data structures in Python?
Python’s power lies in its four built-in data structures: Lists, Tuples, Sets, and Dictionaries. Each of these structures has unique properties and specific use-cases. Here’s a quick example of what they might look like in code:
# List
my_list = [1, 2, 3, 'apple', 'banana']
# Tuple
my_tuple = (1, 2, 3, 'apple', 'banana')
# Set
my_set = {1, 2, 3, 'apple', 'banana'}
# Dictionary
my_dict = {'one': 1, 'two': 2, 'three': 3, 'fruit': ['apple', 'banana']}
# Output:
# No output is expected as we're just declaring the variables
In the above example, we’ve created four different Python data structures. The list and tuple can contain multiple data types, the set will only hold unique values, and the dictionary pairs keys with values. Each of these data structures is used differently depending on what you need to accomplish in your code.
Dive into the following sections for a detailed explanation and examples of each Python data structure.
Table of Contents
- Python Data Structures: The Basics
- Python Data Structures: The Next Level
- Advanced Python Data Structures: Beyond the Basics
- Navigating Python Data Structures: Common Errors and Solutions
- Python Data Structures: Under the Hood
- Python Data Structures: Real-World Applications
- Going Deeper: Algorithms and Memory Management
- Python Data Structures: A Recap
Python Data Structures: The Basics
Python Lists: Your Flexible Friend
Python lists are one of the most used data structures. They are mutable, meaning you can change their content without changing their identity. You can store different types of items in a list.
Here’s a basic example of a Python list:
fruits = ['apple', 'banana', 'cherry']
print(fruits)
# Output:
# ['apple', 'banana', 'cherry']
In the above example, we’ve created a list called ‘fruits’ and filled it with three strings. Lists are perfect for when you need a collection of items that can change over time.
Python Tuples: The Constant Companions
Tuples, unlike lists, are immutable. This means once a tuple is created, you cannot change its content. Tuples are used when you want a list of items that never changes.
Here’s an example of a Python tuple:
days_of_week = ('Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday')
print(days_of_week)
# Output:
# ('Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday')
In this example, we’ve created a tuple called ‘days_of_week’. As the days of the week don’t change, a tuple is the perfect data structure for this use-case.
Python Sets: The Unique Collectors
A set in Python is an unordered collection of unique items. They are mutable, but they can only contain immutable (and hashable) objects. Sets are a great tool when you want to store multiple items in a single variable and ensure that each item is unique.
Here’s an example of a Python set:
fruit_set = {'apple', 'banana', 'cherry', 'apple'}
print(fruit_set)
# Output:
# {'apple', 'banana', 'cherry'}
In the above example, we’ve created a set called ‘fruit_set’. Even though we added ‘apple’ twice, it only appears once in the output because sets only allow unique items.
Python Dictionaries: The Key-Value Keepers
A dictionary is a mutable, unordered collection of key-value pairs. When you need a logical association between a key and a value, the dictionary is your go-to data structure.
Here’s an example of a Python dictionary:
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}
print(fruit_colors)
# Output:
# {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}
In this example, we’ve created a dictionary called ‘fruit_colors’. Each fruit (the key) is associated with a color (the value). Dictionaries are perfect for when you need to associate values with keys.
Python Data Structures: The Next Level
Nested Data Structures in Python
Python allows you to nest its data structures, i.e., you can have data structures within data structures. This is particularly useful when you need to store more complex data.
Here’s an example of nested data structures:
nested_dict = {'fruits': ['apple', 'banana', 'cherry'], 'colors': {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}}
print(nested_dict)
# Output:
# {'fruits': ['apple', 'banana', 'cherry'], 'colors': {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}}
In this example, we’ve created a dictionary with two keys: ‘fruits’ and ‘colors’. The ‘fruits’ key points to a list of fruits, and the ‘colors’ key points to another dictionary that associates each fruit with a color.
Comprehension Syntax in Python
Comprehensions are a unique feature in Python that allows you to create lists, dictionaries, and sets from existing iterables in a clear and concise way.
Here’s an example of list comprehension:
numbers = [1, 2, 3, 4, 5]
squares = [number**2 for number in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In the above example, we’ve created a new list ‘squares’ from the ‘numbers’ list. Each element in the ‘squares’ list is the square of the corresponding element in the ‘numbers’ list.
Common Methods for Python Data Structures
Python provides several built-in methods for manipulating data structures. These methods allow you to add, remove, or change elements in your data structures, among other things.
Here’s an example of some common methods for lists:
fruits = ['apple', 'banana', 'cherry']
fruits.append('date')
print(fruits)
# Output:
# ['apple', 'banana', 'cherry', 'date']
In this example, we’ve used the ‘append’ method to add a new element to the end of the ‘fruits’ list. There are many other methods available for each data structure, and understanding these methods can greatly increase your productivity when working with Python data structures.
Advanced Python Data Structures: Beyond the Basics
Python’s built-in data structures are powerful, but sometimes you need something a bit more specialized. That’s where the collections module comes in. It provides alternatives to the standard data structures that offer additional functionality.
DefaultDict: No More Key Errors
The defaultdict is a dictionary subclass that calls a factory function to supply missing values. In other words, it provides a default value for a nonexistent key.
Here’s an example of defaultdict:
from collections import defaultdict
fruit_colors = defaultdict(lambda: 'Unknown')
fruit_colors['apple'] = 'red'
print(fruit_colors['apple'])
print(fruit_colors['banana'])
# Output:
# red
# Unknown
In this example, we’ve created a defaultdict where the default value for any key that does not exist is ‘Unknown’. So when we try to access the color of ‘banana’ which we have not set, it returns ‘Unknown’.
OrderedDict: Remember the Order
The OrderedDict is a dictionary subclass that remembers the order in which its contents are added.
Here’s an example of OrderedDict:
from collections import OrderedDict
fruit_order = OrderedDict()
fruit_order['apple'] = 1
fruit_order['banana'] = 2
fruit_order['cherry'] = 3
print(fruit_order)
# Output:
# OrderedDict([('apple', 1), ('banana', 2), ('cherry', 3)])
In this example, the OrderedDict ‘fruit_order’ remembers the order in which we added the fruits.
Deque: Double-Ended Queues
The deque (pronounced ‘deck’) is a list-like container with fast appends and pops on either end.
Here’s an example of deque:
from collections import deque
queue = deque(['apple', 'banana', 'cherry'])
queue.appendleft('date')
queue.pop()
print(queue)
# Output:
# deque(['date', 'apple', 'banana'])
In this example, we’ve created a deque ‘queue’ and demonstrated how to add an item to the start of the deque with ‘appendleft’ and remove an item from the end with ‘pop’.
These advanced data structures can be incredibly useful in the right situations. Understanding their benefits and drawbacks will help you make better decisions about which data structure to use in your Python projects.
List Index Out of Range
One of the most common errors when working with Python lists is the ‘IndexError: list index out of range’. This error occurs when you try to access an index that does not exist in the list.
fruits = ['apple', 'banana', 'cherry']
print(fruits[3])
# Output:
# IndexError: list index out of range
In the above example, we’re trying to access the fourth element (index 3) of a list that only has three elements. To avoid this error, always ensure that the index you’re trying to access exists in the list.
Modifying Tuples
Tuples are immutable, which means you cannot change their content once created. Trying to do so results in a ‘TypeError’.
days_of_week = ('Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday', 'Sunday')
days_of_week[0] = 'Newday'
# Output:
# TypeError: 'tuple' object does not support item assignment
In the above example, we’re trying to change the first day of the week to ‘Newday’, which results in a ‘TypeError’. If you find that you need to change the content of a tuple, consider using a list instead.
Adding Elements to a Set
Sets are unordered collections of unique elements. Trying to add a list or another set to a set results in a ‘TypeError’ because sets only accept hashable (immutable) types.
fruit_set = {'apple', 'banana', 'cherry'}
fruit_set.add(['date', 'elderberry'])
# Output:
# TypeError: unhashable type: 'list'
In the above example, we’re trying to add a list to a set, which results in a ‘TypeError’. To add multiple items to a set, you can use the ‘update’ method instead of ‘add’.
Missing Key in Dictionary
Trying to access a key that does not exist in a dictionary results in a ‘KeyError’.
fruit_colors = {'apple': 'red', 'banana': 'yellow', 'cherry': 'red'}
print(fruit_colors['date'])
# Output:
# KeyError: 'date'
In the above example, we’re trying to access the color of ‘date’, which we have not set, so it results in a ‘KeyError’. To avoid this, you can use the dictionary’s ‘get’ method, which allows you to return a default value if the key does not exist.
Understanding these common errors and their solutions will help you avoid pitfalls and write more robust Python code. Remember, practice makes perfect, so don’t be afraid to experiment and learn from any mistakes you make along the way.
Python Data Structures: Under the Hood
The Essence of Data Structures
At its core, a data structure is a particular way of organizing and storing data in a computer so that it can be accessed and modified efficiently. More precisely, a data structure is a collection of data values, the relationships among them, and the functions or operations that can be applied to the data.
Python’s Implementation of Data Structures
Python’s built-in data structures, like lists, tuples, sets, and dictionaries, are powerful tools that can handle most of your data storage and manipulation needs. Python implements these data structures in a way that allows them to be used intuitively and flexibly. They are all mutable (except for tuples), which means you can change their content without changing their identity.
Here’s an example of how Python implements a list:
# List
my_list = [1, 2, 3, 'apple', 'banana']
print(my_list)
# Output:
# [1, 2, 3, 'apple', 'banana']
In this example, we’ve created a list that contains both integers and strings. This kind of flexibility is a hallmark of Python’s data structures.
Comparing Python’s Data Structures to Other Languages
In comparison to other programming languages, Python’s data structures stand out for their ease of use and flexibility. For instance, in languages like Java or C++, you would need to declare the data type of a data structure’s elements upfront, and all elements would need to be of the same type. Python, on the other hand, allows you to mix and match data types in its data structures, as shown in the example above.
Moreover, Python’s data structures come with built-in methods that make data manipulation easy. For example, you can add an element to a list with the ‘append’ method, remove an element with the ‘remove’ method, and so on. These built-in methods make Python’s data structures incredibly user-friendly and powerful.
Understanding the fundamentals of data structures and how they are implemented in Python will give you a solid foundation for using them effectively in your coding projects.
Python Data Structures: Real-World Applications
Data Analysis with Python Data Structures
In the field of data analysis, Python data structures are used extensively. For instance, lists can be used to store and manipulate data sets, while dictionaries can be used to create histograms.
# List
data = [1, 1, 2, 3, 5, 8, 13, 21, 34]
# Dictionary for histogram
histogram = {x: data.count(x) for x in data}
print(histogram)
# Output:
# {1: 2, 2: 1, 3: 1, 5: 1, 8: 1, 13: 1, 21: 1, 34: 1}
In the above example, we’ve created a list of data and then used a dictionary comprehension to create a histogram of the data. The dictionary’s keys are the unique elements in the data, and the values are their frequencies.
Web Development and Python Data Structures
In web development, Python data structures are often used to handle HTTP requests and responses, among other tasks. For example, dictionaries are commonly used to store key-value pairs of data being sent or received over the internet.
# Dictionary
user_data = {'name': 'John Doe', 'email': '[email protected]', 'password': 'secret'}
print(user_data)
# Output:
# {'name': 'John Doe', 'email': '[email protected]', 'password': 'secret'}
In this example, we’ve created a dictionary to store a user’s data. This dictionary could be sent as a JSON payload in an HTTP request or response.
Going Deeper: Algorithms and Memory Management
While Python data structures are a fundamental part of coding in Python, there are other related topics that you might find interesting. Algorithms, for instance, are step-by-step procedures for performing calculations. They often make use of data structures to store and manipulate data.
Memory management is another related topic. Understanding how Python’s data structures are stored in memory can help you write more efficient code. For instance, knowing that lists take up more memory than tuples for the same data can influence your choice of data structure.
Further Resources and Readings
If you’re interested in learning more about these topics, there are many resources available online. We have compiled a handful here:
- Python Data Types: A Comprehensive Guide to Python’s fundamental data types for effective data representation.
Exploring index() Function in Python – Master Python indexing techniques for efficient data traversal and manipulation.
Python Stack: Exploring Stack Data Structure – Explore the concept of stacks in Python and their role in managing data.
Official Python Data Structures – Comprehensive insight into Python’s data structures.
Python.org – Official site for Python programming language resources.
GeeksforGeeks – In-depth Python programming guide from GeeksforGeeks.
By delving into these resources on Python Data Structures, you’ll acquire a more profound understanding and expertise, which can transform you into a proficient Python developer.
Python Data Structures: A Recap
In this guide, we’ve navigated the landscape of Python data structures: lists, tuples, sets, and dictionaries. We’ve explored their usage, delved into common issues, and discovered solutions to these problems.
We’ve also ventured beyond the basics, exploring alternative approaches and advanced data structures available through Python’s collections module, such as defaultdict, OrderedDict, and deque.
To wrap up, let’s look at a comparison of Python’s data structures:
Data Structure | Mutable | Ordered | Allows Duplicates | Use Case |
---|---|---|---|---|
List | Yes | Yes | Yes | Storing a collection of items that can change over time |
Tuple | No | Yes | Yes | Storing a collection of items that never change |
Set | Yes | No | No | Storing multiple unique items in a single variable |
Dictionary | Yes | No (Python < 3.7), Yes (Python >= 3.7) | Yes (for values) | Storing key-value pairs |
In the table above, we’ve compared the mutability, order, allowance of duplicates, and typical use cases of Python’s main data structures. Understanding these characteristics will help you choose the right data structure for your specific needs.
Remember, the key to mastering Python data structures lies in practice. So don’t hesitate to get your hands dirty and start coding!