Python Stack Guide: Setup, Usage, Examples
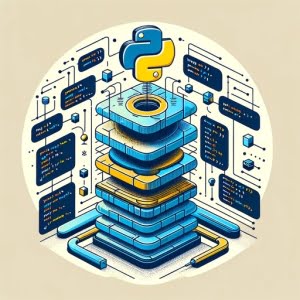
Ever found yourself struggling with implementing stacks in Python? You’re not alone. Many developers find themselves puzzled when it comes to understanding and implementing stacks in Python. But, think of a Python stack as a pile of books – you can only add or remove a book from the top.
Whether you’re trying to manage data in a specific order, reverse a sequence, or navigate through a series of web pages, understanding how to create and manipulate stacks in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of implementing stacks in Python, from the basics to more advanced techniques. We’ll cover everything from using Python’s list data structure, creating a stack using a class, to alternative approaches like using the collections.deque or queue.LifoQueue.
Let’s dive in and start mastering Python Stack!
TL;DR: How Do I Implement a Stack in Python?
In Python, a stack can be implemented using a list. You can use the append() method to add elements and the pop() method to remove elements from the stack.
Here’s a simple example:
stack = []
stack.append('a')
stack.append('b')
stack.append('c')
print(stack)
# Output:
# ['a', 'b', 'c']
In this example, we’ve created an empty list named ‘stack’. We then use the append() method to add elements ‘a’, ‘b’, and ‘c’ to the stack. The print() function is used to display the contents of the stack, which are [‘a’, ‘b’, ‘c’].
This is a basic way to implement a stack in Python, but there’s much more to learn about creating and manipulating stacks. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Python Stack Basics: Using Lists
- Implementing Python Stack Using a Class
- Diving Deeper: Alternative Approaches to Implement Python Stack
- Troubleshooting Python Stacks: Common Issues and Solutions
- Understanding Stack Data Structure
- Python Stacks in Real-World Applications
- Exploring Related Concepts
- Wrapping Up: Mastering Python Stacks
Python Stack Basics: Using Lists
Python’s built-in list data structure is a simple and effective way to implement a stack. The key operations in a stack, namely ‘push’ (add an element to the top of the stack) and ‘pop’ (remove an element from the top of the stack), can be easily achieved using the append() and pop() methods of a list.
Adding Elements to the Stack
To add an element to the stack (push operation), you can use the append() method. This method adds an element to the end of the list, which corresponds to the top of the stack in our analogy.
Here’s an example:
stack = []
stack.append('a')
stack.append('b')
stack.append('c')
print(stack)
# Output:
# ['a', 'b', 'c']
In this code block, we first create an empty list named ‘stack’. We then add the elements ‘a’, ‘b’, and ‘c’ to the stack using the append() method. The print() function displays the contents of the stack, showing the elements in the order they were added.
Removing Elements from the Stack
To remove an element from the stack (pop operation), you can use the pop() method. This method removes the last element from the list, which corresponds to the top of the stack.
Here’s how you can do it:
last_element = stack.pop()
print(f'Popped Element: {last_element}')
print(f'Stack after Pop: {stack}')
# Output:
# Popped Element: c
# Stack after Pop: ['a', 'b']
In this example, we use the pop() method to remove the last element (‘c’) from the stack. The removed element is stored in the variable ‘last_element’, and we then print this value. We also print the contents of the stack after the pop operation, showing that the ‘c’ element has been removed.
Using Python’s list data structure and these two methods, you can easily implement a basic stack and perform the fundamental ‘push’ and ‘pop’ operations.
Implementing Python Stack Using a Class
As you become more comfortable with Python and stacks, you might find it useful to implement a stack using a class. This approach provides a cleaner, more organized way to handle stacks and can make your code more readable and maintainable.
Creating a Stack Class
Let’s create a simple Stack class that encapsulates the functionality of a stack:
class Stack:
def __init__(self):
self.stack = []
def push(self, item):
self.stack.append(item)
def pop(self):
if len(self.stack) < 1:
return None
return self.stack.pop()
def size(self):
return len(self.stack)
my_stack = Stack()
my_stack.push('a')
my_stack.push('b')
my_stack.push('c')
print(f'Stack: {my_stack.stack}')
# Output:
# Stack: ['a', 'b', 'c']
In the above code, we define a Stack class with a constructor that initializes an empty list. This list represents the stack. We then define the push() and pop() methods to add and remove elements from the stack, respectively. The size() method returns the current size of the stack.
We create an instance of the Stack class, my_stack, and add the elements ‘a’, ‘b’, and ‘c’ to it. The print() function displays the contents of the stack, showing the elements in the order they were added.
This approach gives us a clean, organized way to handle stacks, and allows us to easily extend the functionality of our Stack class in the future.
Diving Deeper: Alternative Approaches to Implement Python Stack
While Python lists and classes are common ways to implement a stack, Python also offers some other built-in data structures that can be used to create a stack. Two such data structures are collections.deque and queue.LifoQueue.
Python Stack with collections.deque
The collections module provides a deque (double-ended queue) object that can be used as a stack. Deque is preferred over list in performance wise as it provides an O(1) time complexity for append() and pop() operations as compared to list which provides O(n) time complexity.
Here’s an example of how to use collections.deque to implement a stack:
from collections import deque
stack = deque()
stack.append('a')
stack.append('b')
stack.append('c')
print(f'Stack: {list(stack)}')
# Output:
# Stack: ['a', 'b', 'c']
In this example, we import the deque class from the collections module and create a deque object named ‘stack’. We then use the append() method to add elements to the stack and the print() function to display the contents of the stack.
Python Stack with queue.LifoQueue
The queue module provides a LifoQueue (Last in, First out Queue) which offers stack functionality. Here’s how you can use it:
from queue import LifoQueue
stack = LifoQueue()
stack.put('a')
stack.put('b')
stack.put('c')
while not stack.empty():
print(f'Popped: {stack.get()}')
# Output:
# Popped: c
# Popped: b
# Popped: a
In this example, we import the LifoQueue class from the queue module and create a LifoQueue object named ‘stack’. We then use the put() method to add elements to the stack. We use a while loop to pop and print each element until the stack is empty.
These alternative methods provide additional flexibility and performance benefits. Depending on your specific use case and requirements, you might find one method more suitable than the others.
Troubleshooting Python Stacks: Common Issues and Solutions
While working with stacks in Python, you might encounter some issues. Two of the most common problems are ‘underflow’ and ‘overflow’.
Understanding Stack Underflow
Underflow occurs when you try to pop an element from an empty stack. In Python, this will raise an IndexError
with the message ‘pop from empty list’.
Here’s an example:
stack = []
try:
stack.pop()
except IndexError:
print('Underflow occurred. Stack is empty.')
# Output:
# Underflow occurred. Stack is empty.
In this example, we try to pop an element from an empty stack, which raises an IndexError
. We catch this error with a try/except block and print a message indicating that an underflow occurred.
Handling Stack Overflow
Overflow is less of a concern in Python than in some other languages, as Python’s dynamic list resizing makes it less likely to occur. However, if you’re working with a very large stack or have limited memory, you might encounter a MemoryError
when trying to push an element onto a full stack.
While it’s difficult to provide a code example for a stack overflow (as it depends on your system’s memory), you can generally avoid this issue by ensuring your stack doesn’t grow beyond your system’s memory limits.
Tips for Working with Python Stacks
When working with stacks in Python, you should always check whether the stack is empty before attempting to pop an element. This will prevent underflow errors. Similarly, be mindful of your system’s memory limits to avoid stack overflow errors.
Remember that different stack implementations have different performance characteristics. For example, a list-based stack may perform poorly if you’re frequently inserting or removing elements from the middle of the stack, while a deque-based stack or a LifoQueue-based stack would handle such operations more efficiently.
Understanding Stack Data Structure
Before diving into the depths of implementing stacks in Python, it’s crucial to understand the fundamental concept of a stack data structure. A stack is a linear data structure that follows a particular order in which operations are performed. The order can be summed up as LIFO (Last In First Out), or FILO (First In Last Out).
Unpacking the LIFO Principle
The LIFO principle is what makes a stack unique. It means that the last element added to the stack will be the first one to be removed. This principle is similar to a real-world stack, such as a stack of dishes or a pile of books, where you can only add or remove items from the top of the stack.
Here’s a simple demonstration of the LIFO principle in action:
stack = []
stack.append('a')
stack.append('b')
stack.append('c')
last_in = stack.pop()
print(f'Last In, First Out: {last_in}')
# Output:
# Last In, First Out: c
In this example, we add the elements ‘a’, ‘b’, and ‘c’ to the stack in that order. When we pop an element from the stack, ‘c’ is the first to be removed, even though it was the last to be added. This behavior is the embodiment of the LIFO principle.
Importance of Stacks
Stacks are incredibly versatile and are used in a variety of applications, including parsing, expression evaluation, and backtracking algorithms. They’re also used extensively in memory organization and function execution within computer systems.
Understanding how to implement and manipulate stacks in Python will not only help you write more efficient code, but it will also give you a better understanding of how data is managed and processed within your programs.
Python Stacks in Real-World Applications
Python stacks are not just theoretical constructs; they have practical applications in various areas of computer science and software development.
Backtracking Algorithms
Backtracking is a method of solving problems where you move forward only if there is no obstacle in the solution path, and if you encounter an obstacle, you move backwards and try another path. Stacks are used extensively in backtracking problems to keep track of the visited paths and to backtrack when no solution is found on the current path.
Parsing
In compilers, stacks are used in the parsing phase to check the correctness of expressions and their syntax. For example, they can be used to check whether the opening and closing parentheses in an expression are balanced.
Memory Management
Stacks play a vital role in memory management. When a function is called, the memory for its variables is allocated on the stack, and when the function returns, this memory is freed up.
Exploring Related Concepts
If you’re interested in data structures and want to expand your knowledge beyond stacks, you might want to explore queues and linked lists. Queues follow the FIFO (First In First Out) principle, which is the opposite of the LIFO principle followed by stacks. Linked lists, on the other hand, are a type of data structure where each element points to the next, forming a chain-like structure.
Further Resources for Python Stack Mastery
To deepen your understanding of stacks and related concepts in Python, consider exploring these resources:
- Mastering Python Data Types: Essentials Unveiled explains Python’s data type system to grasp variable storage.
Understanding Python’s Data Structures – Master Python data structures to optimize your algorithms.
Python Float: Working with Floating-Point Numbers – Understand the usage of floating-point numbers in Python.
Python Data Structures Documentation provides a comprehensive overview of Python’s built-in data structures, can be used to implement stacks.
Real Python offers a variety of tutorials and articles on Python programming, including in-depth guides on data structures.
Python Stack tutorial from GeeksforGeeks provides clear examples of how to use built-in types in Python to create stacks.
Wrapping Up: Mastering Python Stacks
In this comprehensive guide, we’ve navigated the world of Python stacks, from basic to advanced implementations. We’ve dissected the concept of stacks, understood the LIFO principle, and learned how to create and manipulate stacks using Python’s list data structure and classes.
We started with the basics, learning how to implement a stack using Python’s built-in list data structure. We then moved on to more advanced techniques, including creating a stack using a class and alternative methods like collections.deque and queue.LifoQueue. Along the way, we tackled common issues you might encounter when working with Python stacks, such as underflow and overflow, and provided solutions to help you navigate these challenges.
We also explored the real-world applications of Python stacks, such as backtracking algorithms, parsing, and memory management, and pointed you towards further resources to deepen your understanding of stacks and related concepts.
Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Performance | Flexibility |
---|---|---|---|
List-based Stack | High | Moderate | Low |
Class-based Stack | Moderate | High | High |
collections.deque | High | High | Moderate |
queue.LifoQueue | Moderate | High | High |
Whether you’re a beginner just starting out with Python stacks or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to create and manipulate stacks in Python, and the power of this data structure. Happy coding!