Java Byte: A Memory-Efficient Primitive Data Type
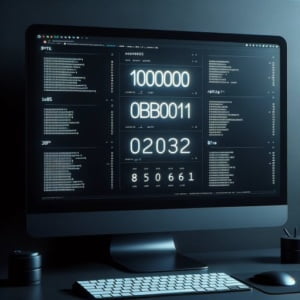
Are you finding it difficult to understand Java byte? You’re not alone. Many developers find themselves puzzled when it comes to handling bytes in Java, but we’re here to help.
Think of a byte in Java as a tiny container that can hold a small amount of data. It’s a fundamental part of Java’s primitive data types, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through what a byte in Java is, how to use it, and when it’s most effective. We’ll cover everything from the basics of declaring and using a byte variable to more advanced techniques, as well as alternative approaches.
Let’s dive in and start mastering Java byte!
TL;DR: What is a Byte in Java?
In Java, a byte is a primitive data type that can hold an 8-bit signed two’s complement integer, instantiated by using the
byte
data type before a variable name, ie:byte myByte = 100;
. It has a minimum value of -128 and a maximum value of 127 (inclusive). Here’s a simple declaration and initialization of a byte in Java:
byte myByte = 100;
System.out.println(myByte);
// Output:
// 100
In this example, we declare a byte variable named myByte
and assign it the value 100. When we print the value of myByte
, the output is 100.
This is just the tip of the iceberg when it comes to understanding and using bytes in Java. Continue reading for more detailed information and examples.
Table of Contents
- Declaring and Using Byte Variables in Java
- Byte Operations and Conversion in Java
- Alternatives to Byte: Short, Int, and Long
- Troubleshooting Common Byte Issues
- Java’s Primitive Data Types and the Two’s Complement Concept
- The Relevance of Byte in Memory-Efficient Programming
- Byte’s Role in Network Programming
- Further Resources for Mastering Java Byte
- Wrapping Up: Mastering Java Byte Data Type
Declaring and Using Byte Variables in Java
In Java, declaring and using a byte variable is straightforward. Let’s start with a simple example:
byte myByte = 10;
System.out.println(myByte);
// Output:
// 10
In this example, we declare a byte variable called myByte
and assign it the value 10. When we print myByte
, it outputs 10 as expected.
The Importance of Byte in Java
The byte data type in Java is very useful when you’re working with a stream of data from a network or file. It allows you to read or write data byte by byte.
Advantages and Pitfalls of Java Byte
One of the main advantages of using byte in Java is its small size. A byte takes up only 8 bits of memory, making it a memory-efficient choice when dealing with large amounts of data.
However, one potential pitfall of using byte is its limited range of values (-128 to 127). If you try to store a value outside of this range, you’ll encounter an error. For example:
byte bigByte = 130;
// Output:
// Error: incompatible types: possible lossy conversion from int to byte
In this case, we tried to assign the value 130 to a byte variable, which is outside the valid range. As a result, Java throws an error. Always be cautious when using byte to ensure the values you’re working with fall within the valid range.
Byte Operations and Conversion in Java
As we dive deeper into the use of bytes in Java, we encounter more complex operations such as bitwise operations and type conversions.
Bitwise Operations with Bytes
Java provides several bitwise operators that can be used with byte data type. These operators perform operations on individual bits of a byte. Let’s take a look at an example:
byte byte1 = 0b0011; // decimal 3
byte byte2 = 0b0101; // decimal 5
byte result = (byte) (byte1 & byte2);
System.out.println(result);
// Output:
// 1
In the above example, we perform a bitwise AND operation on byte1
and byte2
. The result is 1, which is the decimal equivalent of the binary number obtained by performing the AND operation on the individual bits of byte1
and byte2
.
Byte Conversion in Java
Java allows us to convert bytes to other primitive data types. This is known as type casting. Let’s look at an example of converting a byte to an int:
byte myByte = 100;
int myInt = myByte;
System.out.println(myInt);
// Output:
// 100
Here, we have a byte variable myByte
with a value of 100. We can convert this byte to an integer simply by assigning it to an int variable myInt
. The output of the program is 100, the integer equivalent of the byte value.
Remember, while converting byte to larger data types is straightforward, converting larger types to byte should be done with caution to avoid data loss. For example, if an integer value is larger than 127, converting it to byte will result in data loss.
Alternatives to Byte: Short, Int, and Long
While byte is a useful data type in Java, it’s not always the best choice. Depending on your needs, you might find other data types like short, int, or long more suitable. Let’s explore these alternatives.
Short: The Middle Ground
Short is a 16-bit signed two’s complement integer. It has a minimum value of -32,768 and a maximum value of 32,767. It’s twice as large as byte and can be a better choice when you need to store values that can’t fit into a byte.
short myShort = 32000;
System.out.println(myShort);
// Output:
// 32000
In this example, we use a short to store the value 32000, which is outside the range of byte.
Int: The Go-To Choice
Int is a 32-bit signed two’s complement integer. It’s the most commonly used integer type in Java, and it can hold a much larger range of values than byte or short.
int myInt = 1000000;
System.out.println(myInt);
// Output:
// 1000000
Here, we use an int to store the value 1000000, which is far outside the range of both byte and short.
Long: When You Need More
Long is a 64-bit signed two’s complement integer. It’s used when int is not large enough to hold the required value.
long myLong = 5000000000L;
System.out.println(myLong);
// Output:
// 5000000000
In this example, we use a long to store the value 5000000000, which is outside the range of int.
Each of these data types has its benefits and drawbacks. While short, int, and long can hold larger values, they also take up more memory. When choosing a data type, consider both the range of values you need to store and the memory efficiency of the data type.
Troubleshooting Common Byte Issues
When working with byte in Java, you may encounter a few common issues. Let’s discuss some of these problems and how to resolve them.
Data Overflow
One common issue is data overflow. This happens when you try to store a value in a byte that’s outside its valid range (-128 to 127). Here’s an example:
byte myByte = 130;
// Output:
// Error: incompatible types: possible lossy conversion from int to byte
In this case, we tried to assign the value 130 to a byte variable, which is outside the valid range. As a result, Java throws an error. To resolve this, ensure the values you’re working with fall within the valid range for byte.
Type Casting
Another common issue is related to type casting. When you convert a larger data type to byte, you may lose data if the value is outside the byte’s range. Here’s an example:
int myInt = 130;
byte myByte = (byte) myInt;
System.out.println(myByte);
// Output:
// -126
In this case, we tried to cast an int value of 130 to a byte. Since 130 is outside the byte’s range, we end up with an unexpected result. To avoid such issues, be cautious when casting larger data types to byte.
Java’s Primitive Data Types and the Two’s Complement Concept
To fully grasp the byte data type in Java, it’s essential to understand Java’s primitive data types and the concept of signed two’s complement.
Java’s Primitive Data Types
Java has eight primitive data types: byte, short, int, long, float, double, char, and boolean. Each of these data types has a different size and value range, and they serve different purposes. For instance, byte, short, int, and long are used for storing whole numbers, while float and double are used for fractional numbers.
byte byteNum = 10;
short shortNum = 1000;
int intNum = 100000;
long longNum = 1000000000L;
float floatNum = 1.23f;
double doubleNum = 123.456;
char character = 'A';
boolean flag = true;
In this example, we declare and initialize each of the eight primitive data types in Java.
The Concept of Signed Two’s Complement
The byte data type in Java is a signed two’s complement integer. This means it can represent both positive and negative numbers, making it different from the byte you might be familiar with in other languages, which is unsigned and can only represent positive numbers.
The two’s complement method is a way of representing positive and negative integers in binary. It allows for easy addition and subtraction of numbers and simplifies the hardware design of computers.
For example, the number 10 in binary is 00001010
. The two’s complement of 10 is obtained by inverting the digits (1 becomes 0, 0 becomes 1) and adding 1 to the result. So, the two’s complement of 10 is 11110110
, which represents -10 in two’s complement notation.
Understanding these fundamental concepts will help you make the most of the byte data type in Java.
The Relevance of Byte in Memory-Efficient Programming
The byte data type in Java plays a crucial role in memory-efficient programming. Its small size (8 bits) makes it an ideal choice when working with large amounts of data. For example, when reading data from a file or a network, using byte allows you to process the data byte by byte, reducing the memory footprint of your program.
byte[] buffer = new byte[1024];
int bytesRead = inputStream.read(buffer);
In this example, we create a buffer of bytes to read data from an InputStream. This allows us to read up to 1024 bytes of data at a time, which can significantly improve the efficiency of our program when dealing with large amounts of data.
Byte’s Role in Network Programming
Byte also plays a significant role in network programming. When transmitting data over a network, the data is typically sent as a stream of bytes. Therefore, understanding how to work with bytes is essential for network programming.
byte[] message = "Hello, World!".getBytes();
outputStream.write(message);
In this example, we convert a string to a byte array using the getBytes()
method, and then write the byte array to an OutputStream. This is a common operation in network programming.
Further Resources for Mastering Java Byte
To further deepen your understanding of Java byte and related concepts, here are some resources you might find helpful:
- Fundamentals of Primitive Data Types in Java – Explore the performance benefits of using primitive data types in Java.
Characters in Java – Explore the Java char data type for representing individual characters.
Java Int – Explore the Java int data type for storing integer values.
Oracle’s Java Tutorials: Primitive Data Types – A comprehensive guide to the primitive data types in Java, including byte.
Java Data Types Tutorial by JavaTpoint covers everything you need to know about data types in Java.
Data Types in Java by GeeksforGeeks provides an in-depth overview of primitive and non-primitive data types in Java, detailing .
Wrapping Up: Mastering Java Byte Data Type
In this comprehensive guide, we’ve delved into the world of Java byte, a primitive data type that holds a significant place in Java programming.
We began with the basics, understanding what a byte in Java is and how to use it. We then delved into more advanced territory, exploring complex byte operations, conversions, and even alternative data types for handling small integer values.
Along the way, we tackled common challenges you might encounter when working with byte in Java, such as data overflow and type casting issues, providing you with solutions to these problems. We also discussed the relevance of byte in memory-efficient and network programming, shedding light on its importance beyond basic data storage.
We also compared byte with other data types in Java, such as short, int, and long. Here’s a quick comparison of these data types:
Data Type | Size | Min Value | Max Value |
---|---|---|---|
byte | 8 bits | -128 | 127 |
short | 16 bits | -32,768 | 32,767 |
int | 32 bits | -2,147,483,648 | 2,147,483,647 |
long | 64 bits | -9,223,372,036,854,775,808 | 9,223,372,036,854,775,807 |
Whether you’re just starting out with Java or you’re looking to level up your data handling skills, we hope this guide has given you a deeper understanding of Java byte and its capabilities.
With its balance of size, range, and flexibility, byte is a powerful tool for data handling in Java. Keep experimenting, keep learning, and happy coding!