Java int: A Guide to Integer Primitive Data Type
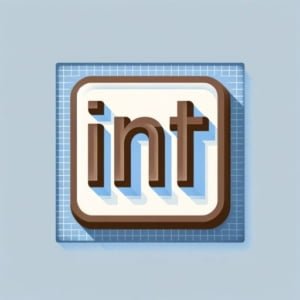
Are you finding it challenging to understand 'int'
in Java? You’re not alone. Many developers, especially beginners, grapple with understanding this fundamental data type. Think of 'int'
in Java as a building block – it’s a basic element that holds up more complex structures in your code.
This guide will walk you through everything you need to know about using ‘int’ in Java, from declaration to advanced usage. We’ll explore the core functionality of ‘int’, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering ‘int’ in Java!
TL;DR: What is ‘int’ in Java and How Do I Use It?
'int'
is a primitive data type in Java used to store integer values, instantiated with the syntax,int number = [numericalValue];
. It’s a fundamental part of Java that you’ll use frequently when dealing with numerical data.
Here’s a simple example of declaring and using an ‘int’:
int number = 10;
System.out.println(number);
# Output:
# 10
In this example, we declare an integer variable named ‘number’ and assign it the value 10. We then print the value of ‘number’ to the console, which outputs ’10’.
This is just the basics of using ‘int’ in Java. There’s much more to learn about ‘int’, including advanced usage and common issues. Continue reading for a more detailed explanation and advanced usage of ‘int’.
Table of Contents
Declaring and Initializing ‘int’ Variables in Java
In Java, declaring and initializing an ‘int’ variable is straightforward. You start with the keyword ‘int’, followed by the variable name, and then assign a value using the ‘=’ operator. Here’s an example:
int myNumber = 25;
System.out.println(myNumber);
# Output:
# 25
In this code block, we declare an integer variable named ‘myNumber’ and assign it the value 25. We then print the value of ‘myNumber’ to the console, which outputs ’25’.
Range of Values for ‘int’
An ‘int’ in Java can hold a wide range of values. It’s a 32-bit signed two’s complement integer, which means it can store values from -2,147,483,648 (-2^31) to 2,147,483,647 (2^31 -1). This wide range makes ‘int’ suitable for a variety of numerical data in your programs. However, for larger values, you’ll need to use other data types, which we’ll discuss later in this guide.
Arithmetic Operations with ‘int’
Java allows you to perform various arithmetic operations with ‘int’ variables. Let’s look at an example:
int a = 10;
int b = 5;
int sum = a + b;
int difference = a - b;
int product = a * b;
int quotient = a / b;
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Quotient: " + quotient);
# Output:
# Sum: 15
# Difference: 5
# Product: 50
# Quotient: 2
In this example, we perform addition, subtraction, multiplication, and division using ‘int’ variables.
Type Conversion with ‘int’
In Java, you can convert an ‘int’ to other data types. This is known as type conversion. Here’s an example of converting an ‘int’ to a ‘double’:
int myInt = 10;
double myDouble = myInt;
System.out.println(myDouble);
# Output:
# 10.0
In this code block, we declare an integer variable ‘myInt’ and assign it the value 10. We then convert ‘myInt’ to a double and store it in ‘myDouble’. The output is ‘10.0’, which is the double representation of the integer 10.
Bitwise Operations with ‘int’
Bitwise operations are advanced operations that you can perform with ‘int’ variables in Java. These operations work on the binary representations of integers. Here’s an example of a bitwise AND operation:
int x = 12; // Binary: 1100
int y = 10; // Binary: 1010
int result = x & y;
System.out.println(result);
# Output:
# 8
In this example, we perform a bitwise AND operation on ‘x’ and ‘y’. The result is ‘8’, which is the decimal representation of the binary number ‘1000’.
Alternatives to ‘int’ in Java
While ‘int’ is commonly used to store integer values in Java, there are alternative data types you can use, depending on your specific needs. Let’s explore some of these alternatives: ‘short’, ‘long’, and ‘BigInteger’.
Using ‘short’ Instead of ‘int’
The ‘short’ data type in Java is a 16-bit signed two’s complement integer. It’s used when memory is a concern, and it can hold values from -32,768 to 32,767.
short myShort = 5000;
System.out.println(myShort);
# Output:
# 5000
In this example, we declare a ‘short’ variable ‘myShort’ and assign it the value 5000. We then print the value of ‘myShort’, which outputs ‘5000’.
Using ‘long’ for Larger Integer Values
The ‘long’ data type in Java is a 64-bit signed two’s complement integer. It’s used when you need to store large integer values that exceed the capacity of ‘int’.
long myLong = 10000000000L;
System.out.println(myLong);
# Output:
# 10000000000
In this code block, we declare a ‘long’ variable ‘myLong’ and assign it the value 10000000000. We then print the value of ‘myLong’, which outputs ‘10000000000’.
Using ‘BigInteger’ for Very Large Integer Values
The ‘BigInteger’ class in Java is used to store very large integer values that exceed the capacity of all primitive integer types.
import java.math.BigInteger;
BigInteger myBigInt = new BigInteger("12345678901234567890");
System.out.println(myBigInt);
# Output:
# 12345678901234567890
In this example, we create a ‘BigInteger’ object ‘myBigInt’ and assign it a very large value. We then print the value of ‘myBigInt’, which outputs ‘12345678901234567890’.
As with any programming element, using ‘int’ in Java comes with its own set of potential problems. One of the most common issues you might encounter is arithmetic overflow. Let’s discuss this issue and how to address it.
Understanding Arithmetic Overflow
Arithmetic overflow occurs when the result of an arithmetic operation exceeds the maximum value that the ‘int’ data type can hold (2,147,483,647).
int maxInt = Integer.MAX_VALUE;
System.out.println("Max int: " + maxInt);
int result = maxInt + 1;
System.out.println("Result: " + result);
# Output:
# Max int: 2147483647
# Result: -2147483648
In this example, we first print the maximum ‘int’ value, which is 2,147,483,647. Then, we try to add 1 to this maximum value. Instead of getting a larger number, we get -2,147,483,648. This is because the ‘int’ value has overflowed and wrapped around to the minimum ‘int’ value.
Solutions to Arithmetic Overflow
To prevent arithmetic overflow, you can use the ‘long’ data type for operations that might result in large values. Alternatively, you can use the ‘BigInteger’ class for operations that might result in very large values.
Remember, understanding the limitations of the ‘int’ data type and planning your code accordingly is key to avoiding common issues like arithmetic overflow.
Understanding Data Types in Java
In Java, every variable has a data type, which determines the size and layout of the variable’s memory, the range of values that can be stored within that memory, and the set of operations that can be applied to the variable.
There are two categories of data types in Java: primitive types and reference types.
Primitive Data Types
Primitive data types are the most basic data types in Java. They include ‘byte’, ‘short’, ‘int’, ‘long’, ‘float’, ‘double’, ‘boolean’, and ‘char’. Each of these types has a predefined size and a specific range of values they can represent.
For instance, ‘int’ is a 32-bit signed two’s complement integer that can hold values from -2,147,483,648 to 2,147,483,647.
int myInt = 100;
System.out.println(myInt);
# Output:
# 100
In this example, we declare an ‘int’ variable ‘myInt’ and assign it the value 100. We then print the value of ‘myInt’, which outputs ‘100’.
Reference Data Types
Reference data types, on the other hand, are used to store references to objects. The reference types can be classes, interfaces, arrays, or enumerations. Unlike primitive types, reference types can be null, which means they don’t currently refer to an object.
Here’s an example of a reference type:
String myString = "Hello, world!";
System.out.println(myString);
# Output:
# Hello, world!
In this code block, we declare a ‘String’ variable ‘myString’ and assign it the value “Hello, world!”. We then print the value of ‘myString’, which outputs ‘Hello, world!’.
Understanding the difference between primitive types and reference types is fundamental to programming in Java, as it influences how you declare variables, how you use memory, and how you structure your code.
Expanding Your Use of ‘int’ in Java
The ‘int’ data type is not just for simple operations or small programs. It’s a fundamental part of Java that you’ll use in larger, more complex programs. Whether you’re creating an array of integers, using ‘int’ in loop counters, or incorporating ‘int’ in object-oriented programming, understanding ‘int’ is crucial to your success as a Java developer.
Implementing ‘int’ in Arrays
Arrays in Java can store multiple values of the same type, including ‘int’. Here’s a simple example:
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
# Output:
# 1
# 2
# 3
# 4
# 5
In this code block, we declare an array of integers and then use a for-each loop to print each number.
Using ‘int’ in Loops
‘int’ variables are often used as loop counters in Java. Here’s an example of using ‘int’ in a for loop:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, we declare an ‘int’ variable ‘i’ and use it as a counter in a for loop. We then print the value of ‘i’ in each iteration of the loop.
‘int’ in Object-Oriented Programming
In object-oriented programming, ‘int’ can be used as an attribute in a class. Here’s an example:
public class MyClass {
int myInt = 10;
}
In this code block, we declare a class ‘MyClass’ with an ‘int’ attribute ‘myInt’.
Further Resources for Mastering ‘int’ in Java
For additional learning, here are some valuable resources:
- Best Practices for Using Primitive Data Types in Java – Learn about the proper usage of Java’s primitive types.
Java Boolean – Understand the Java boolean data type for representing true or false values.
Java Byte – Learn about the Java byte data type for representing small integer values.
Oracle’s Java Tutorials provides a set of tutorials that cover all aspects of Java programming.
Java Programming Notes provide a great overview of Java programming, including the use of ‘int’.
GeeksforGeeks’ Java Programming Language resource offers a wealth of information on Java programming.
Wrapping Up: Mastering ‘int’ in Java
In this comprehensive guide, we’ve journeyed through the world of ‘int’ in Java, a fundamental data type for storing integer values.
We started with the basics, learning how to declare and initialize ‘int’ variables. We then explored more advanced topics, such as arithmetic operations, type conversion, and bitwise operations with ‘int’. We also discussed common issues you might encounter when using ‘int’, such as arithmetic overflow, and provided solutions to these problems.
Along the way, we looked at alternative data types that can be used in place of ‘int’, including ‘short’, ‘long’, and ‘BigInteger’. Here’s a quick comparison of these data types:
Data Type | Size | Range |
---|---|---|
int | 32-bit | -2,147,483,648 to 2,147,483,647 |
short | 16-bit | -32,768 to 32,767 |
long | 64-bit | -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
BigInteger | Arbitrary-precision | No theoretical limit |
Whether you’re a beginner just starting out with Java or an experienced developer looking to deepen your understanding of ‘int’ and its alternatives, we hope this guide has been a valuable resource.
Understanding ‘int’ and its alternatives is crucial for working with numerical data in Java. With this knowledge, you’re well-equipped to write efficient and effective Java code. Happy coding!