Booleans in Java: From Basics to Advanced
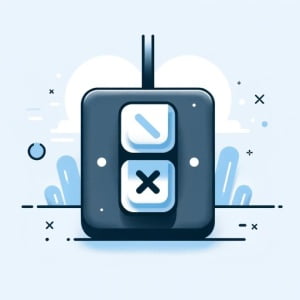
Are you finding it challenging to work with boolean in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling boolean data types in Java, but we’re here to help.
Think of Java’s boolean as a light switch – it can only be in one of two states, true or false. This simple yet powerful feature provides a versatile and handy tool for various tasks, especially in control structures.
In this guide, we’ll walk you through the process of working with boolean in Java, from declaration and initialization to more advanced uses in control structures. We’ll cover everything from the basics of boolean data types to more advanced techniques, as well as alternative approaches.
Let’s get started and master boolean in Java!
TL;DR: How Do I Use Boolean in Java?
In Java, you can declare a boolean variable and assign it a value of true or false:
boolean isJavaDifficult = false
This is a fundamental concept in Java that is used in various control structures.
Here’s a simple example:
boolean isJavaFun = true;
System.out.println(isJavaFun);
// Output:
// true
In this example, we declare a boolean variable isJavaFun
and assign it a value of true
. We then print the value of isJavaFun
, which outputs true
.
This is just a basic way to use boolean in Java, but there’s much more to learn about using boolean in control structures and other advanced scenarios. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Declaring and Initializing Boolean Variables in Java
- Leveraging Boolean in Control Structures
- Exploring the Java Boolean Class
- Common Pitfalls and Solutions with Java Boolean
- Understanding Java Boolean Fundamentals
- The Relevance of Boolean in Larger Java Programs
- Exploring Related Concepts
- Wrapping Up: Mastering Boolean in Java
Declaring and Initializing Boolean Variables in Java
In Java, declaring a boolean variable is straightforward. You use the boolean
keyword followed by the variable name. Let’s dive into a simple example:
boolean isLearningJava;
In this example, we’ve declared a boolean variable isLearningJava
but haven’t assigned a value yet. By default, a boolean variable is false
if you don’t assign it a value.
To initialize a boolean variable, you assign it a value of true
or false
.
boolean isLearningJava = true;
Now, isLearningJava
is not just declared, but also initialized with the value true
.
The Power of Boolean
Booleans are simple, yet powerful. They are primarily used in control flow statements like if
, while
, and for
loops. Booleans can help your program make decisions based on certain conditions.
Potential Pitfalls with Boolean
While booleans are easy to use, they can lead to confusing code if not used properly. For instance, using boolean variables that have not been initialized can lead to unexpected results. Always ensure your boolean variables are properly initialized before using them in your code.
Leveraging Boolean in Control Structures
In Java, boolean expressions play a critical role in control structures such as if-else statements and while loops. Let’s explore how to use boolean expressions in these structures.
If-Else Statements with Boolean
In an if-else statement, a boolean condition determines the flow of execution. If the condition is true
, the code within the if
block executes. If the condition is false
, the else
block executes (if present).
Here’s an example:
boolean isLearningJava = true;
if (isLearningJava) {
System.out.println("Keep up the good work!");
} else {
System.out.println("Let's start learning Java.");
}
// Output:
// Keep up the good work!
In this example, since isLearningJava
is true
, the message “Keep up the good work!” is printed.
While Loops with Boolean
A while loop continues to execute as long as the boolean condition remains true
. Here’s an example:
int count = 0;
while (count < 5) {
System.out.println("Count is: " + count);
count++;
}
// Output:
// Count is: 0
// Count is: 1
// Count is: 2
// Count is: 3
// Count is: 4
In this example, the while loop continues to print and increment the count as long as count < 5
is true
. Once count
reaches 5, the condition becomes false
, and the loop terminates.
Exploring the Java Boolean Class
Java provides a Boolean class, which is a part of java.lang package. It wraps the boolean
primitive data type into an object. The Boolean class offers a range of methods to help you manipulate boolean values.
Using Boolean Class Methods
Let’s look at a few examples of these methods. The Boolean.valueOf()
method returns the Boolean value of the specified string. If the string argument is not null
and is equal, ignoring case, to the string “true”, it returns true
. Otherwise, it returns false
.
Boolean bool1 = Boolean.valueOf("True");
Boolean bool2 = Boolean.valueOf("False");
System.out.println(bool1);
System.out.println(bool2);
// Output:
// true
// false
In this example, Boolean.valueOf("True")
returns true
, and Boolean.valueOf("False")
returns false
.
Advantages of Using Boolean Class
The Boolean class has several advantages. It provides useful constants and methods for dealing with boolean values. It also allows null values, unlike the primitive boolean type.
Disadvantages of Using Boolean Class
However, it’s important to remember that objects of the Boolean class are immutable. Once a Boolean object is created, it cannot change its value. This is unlike the primitive boolean type, which can be reassigned freely. Also, comparing Boolean objects using '=='
can lead to unexpected results, as it compares object references, not their values.
Common Pitfalls and Solutions with Java Boolean
Working with boolean in Java can sometimes lead to unexpected results. Let’s discuss some common issues and their solutions.
Misunderstanding '=='
and ‘equals()’
One common mistake when working with the Boolean class is misunderstanding the difference between '=='
and ‘equals()’. The '=='
operator checks if two references point to the same object, whereas ‘equals()’ checks if the two objects have the same value.
Boolean bool1 = new Boolean(true);
Boolean bool2 = new Boolean(true);
System.out.println(bool1 == bool2); // false
System.out.println(bool1.equals(bool2)); // true
In this example, bool1 == bool2
returns false
because bool1
and bool2
are different objects. However, bool1.equals(bool2)
returns true
because both objects have the same value (true
).
Uninitialized Boolean Variables
Another common issue is using a boolean variable that has not been initialized. In Java, local variables are not given a default value.
boolean myBool;
System.out.println(myBool);
// Output:
// Compilation error: variable myBool might not have been initialized
In this example, trying to print myBool
results in a compilation error because myBool
has not been initialized. Always ensure your boolean variables are properly initialized before using them.
Understanding Java Boolean Fundamentals
In Java, a boolean is a primitive data type that can only hold one of two possible values: true
or false
. This binary nature makes boolean data types extremely useful in control structures, where they can dictate the flow of a program based on certain conditions.
boolean isRaining = false;
if (isRaining) {
System.out.println("Better take an umbrella!");
} else {
System.out.println("No need for an umbrella.");
}
// Output:
// No need for an umbrella.
In this example, the boolean variable isRaining
is false
, so the program prints “No need for an umbrella.” If isRaining
were true
, the program would print “Better take an umbrella!”
Primitive Boolean vs Boolean Class
Java provides two ways to work with boolean values: the primitive boolean data type and the Boolean class. The primitive boolean data type is simple and efficient, but it lacks the object-oriented features provided by the Boolean class.
The Boolean class, on the other hand, wraps a value of the primitive type boolean
in an object. An object of type Boolean contains a single field, whose type is boolean
. In addition, this class provides several useful constants and methods for manipulating boolean values.
Boolean isRaining = new Boolean(false);
if (isRaining.equals(true)) {
System.out.println("Better take an umbrella!");
} else {
System.out.println("No need for an umbrella.");
}
// Output:
// No need for an umbrella.
In this example, we use the Boolean class instead of the primitive boolean data type. The result is the same, but notice the use of the equals()
method for comparison.
The Relevance of Boolean in Larger Java Programs
In larger Java programs, boolean plays a significant role in conditional statements, loops, and error handling. The ability of boolean to hold one of two values, true
or false
, makes it an essential tool for controlling program flow.
Boolean in Conditional Statements
In conditional statements like if
, else if
, and else
, boolean expressions determine which block of code executes. For example:
boolean isEligible = checkEligibility(user);
if (isEligible) {
grantAccess(user);
} else {
denyAccess(user);
}
// Output:
// (depends on the result of checkEligibility(user))
Boolean in Loops
Boolean is also used in loops such as while
and for
. A boolean condition controls when the loop starts and ends. For example:
int i = 0;
while (i < 10) {
System.out.println(i);
i++;
}
// Output:
// 0
// 1
// 2
// ...
// 9
Boolean in Error Handling
In error handling, boolean can be used to indicate the success or failure of a method. For example:
boolean isSuccess = saveData(data);
if (!isSuccess) {
System.out.println("Failed to save data.");
}
// Output:
// (depends on the result of saveData(data))
Exploring Related Concepts
While boolean is a fundamental concept in Java, it’s just one piece of the puzzle. To write effective Java programs, you should also understand related concepts like control structures and exception handling in Java.
Further Resources for Mastering Java Boolean
To deepen your understanding of boolean in Java, here are some additional resources:
- IOFlood’s Java Primitive Data Types Guide explores Java’s default values for uninitialized primitive variables.
Java Float – Explore the Java float data type for representing single-precision floating-point numbers.
Int Data Type in Java – Learn about the range and memory allocation of int variables in Java.
Java Boolean Documentation – Official Java documentation for the Boolean class.
Java Tutorials by Oracle – Comprehensive tutorials on various aspects of Java, including boolean and control structures.
Java Programming Basics by Udacity – An online course that covers the basics of Java programming, including boolean.
Wrapping Up: Mastering Boolean in Java
In this comprehensive guide, we’ve embarked on a journey to understand and master the use of boolean in Java, from its declaration and initialization to its role in control structures.
We began with the basics, explaining how to declare and initialize boolean variables in Java. We then delved into more advanced topics, such as using boolean expressions in control structures like if-else statements and while loops. We also explored the Boolean class in Java and its methods, providing a more object-oriented approach to manipulating boolean values.
Along the way, we addressed common issues that you might encounter when working with boolean in Java, such as the difference between '=='
and ‘equals()’ when comparing Boolean objects, and the importance of initializing boolean variables. We provided solutions and workarounds for these issues, helping you to avoid these pitfalls in your coding journey.
Approach | Pros | Cons |
---|---|---|
Primitive boolean | Simple, efficient | No object-oriented features |
Boolean class | Object-oriented, provides useful methods | Immutable, '==' compares references |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of boolean, we hope this guide has shed light on the power and versatility of boolean in Java.
Understanding and using boolean effectively is a key skill in Java programming. With this guide, you’re well on your way to mastering boolean in Java. Happy coding!