Python join() Function | Usage Guide (With Examples)
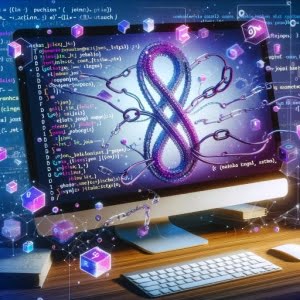
Ever felt like you’re wrestling with your code to join strings in Python? Fret not. The Python join()
function is your skilled craftsman, meticulously and seamlessly connecting pieces of strings together.
This comprehensive guide aims to be your beacon, illuminating the path through the labyrinth of Python’s join()
function. We’ll start from the basic usage, gradually escalating to advanced techniques.
By the end of this journey, you’ll be wielding the join()
function like a seasoned Pythonista, no string joining task would be too daunting!
TL;DR: How Can I Join Strings in Python?
Python’s
join()
function is your go-to tool for joining strings. Here’s a quick demonstration:
words = ['Hello', 'World']
sentence = ' '.join(words)
print(sentence)
# Output:
# 'Hello World'
In this example, we have a list named ‘words’ containing two strings: ‘Hello’ and ‘World’. We use the join()
function with a space (‘ ‘) as the separator to join these strings together into a single string ‘Hello World’. This is the basic usage of Python’s join()
function. But there’s so much more to it! Stay with us for a deep dive into more detailed explanations and advanced usage scenarios.
Table of Contents
- Starting Simple: Basic Use of Python’s join() Function
- Diving Deeper: Complex Scenarios with Python’s join() Function
- Exploring Alternatives: Other Ways to Join Strings in Python
- Navigating Pitfalls: Troubleshooting Common Issues with join()
- Grasping the Basics: Understanding Python’s String Data Type
- Broadening Horizons: The Power of String Joining
- Python’s join(): A Recap and Comparison
Starting Simple: Basic Use of Python’s join()
Function
Python’s join()
function is a string method that concatenates (joins) the elements of an iterable (like a list or tuple) into a single string. The separator between the elements is the string providing this method.
Let’s break it down with a simple example:
fruits = ['apple', 'banana', 'cherry']
my_fruits = ', '.join(fruits)
print(my_fruits)
# Output:
# 'apple, banana, cherry'
In this example, we have a list named ‘fruits’ containing three strings: ‘apple’, ‘banana’, and ‘cherry’. We use the join()
function with a comma and a space (‘, ‘) as the separator to join these strings together into a single string ‘apple, banana, cherry’.
One major advantage of the join()
function is its efficiency. It’s faster and consumes less memory compared to other methods like string concatenation using the +
operator. However, a potential pitfall is that it only works with iterables containing strings. Trying to join an iterable containing non-string types will result in a TypeError.
Diving Deeper: Complex Scenarios with Python’s join()
Function
The join()
function in Python is not just limited to simple string joining. It can handle more complex scenarios, such as joining strings with different separators.
Let’s take a look at an example:
data = ['John', 'Doe', '[email protected]']
name = ' '.join(data[:2])
contact = ': '.join([name, data[2]])
print(contact)
# Output:
# 'John Doe: [email protected]'
In this case, we first join the first two strings in the list ‘data’ using a space as a separator. The result, ‘John Doe’, is then joined with the last string in the list using a colon and space (‘: ‘) as the separator. The final output is ‘John Doe: [email protected]’.
This example demonstrates the flexibility of the join()
function. You can use different separators for different parts of your string, allowing you to format your output string precisely. However, remember that the join()
function only works with iterables containing strings. If you try to join an iterable containing non-string types, you’ll need to convert those elements to strings first.
Exploring Alternatives: Other Ways to Join Strings in Python
While Python’s join()
function is a powerful tool, it’s not the only way to join strings. Let’s explore two alternative approaches: using the +
operator and the format()
function.
Joining Strings Using the +
Operator
The +
operator can be used to concatenate strings. Here’s an example:
first_name = 'John'
last_name = 'Doe'
full_name = first_name + ' ' + last_name
print(full_name)
# Output:
# 'John Doe'
In this example, we’re using the +
operator to combine ‘John’, a space, and ‘Doe’ into a single string ‘John Doe’. While this method is straightforward and easy to understand, it can be inefficient when dealing with large numbers of strings due to the way Python handles string memory.
Joining Strings Using the format()
Function
The format()
function is another method for joining strings, especially when you want to insert variables into a string. Here’s how it works:
first_name = 'John'
last_name = 'Doe'
full_name = '{} {}'.format(first_name, last_name)
print(full_name)
# Output:
# 'John Doe'
In this example, we’re using the format()
function to insert the strings ‘John’ and ‘Doe’ into the string ‘{} {}’. The {}
are placeholders that get replaced by the variables in the format()
function. This method is very flexible and allows for complex string formatting. However, it can be a bit verbose for simple string joining tasks.
In summary, while the join()
function is a versatile and efficient method for joining strings in Python, don’t forget about the +
operator and the format()
function. They can be more suitable depending on your specific needs.
Like any other function, Python’s join()
function has its quirks. Let’s discuss some common issues you might encounter while joining strings and how to overcome them.
Joining Non-String Types
One common issue is trying to join non-string types. The join()
function only works with iterables containing strings. If you try to join an iterable containing non-string types, you’ll get a TypeError. Here’s an example:
numbers = [1, 2, 3]
numbers_str = ', '.join(numbers)
print(numbers_str)
# Output:
# TypeError: sequence item 0: expected str instance, int found
In this example, we tried to join a list of integers, which results in a TypeError. To fix this, we need to convert the integers to strings first. This can be done using a list comprehension:
numbers = [1, 2, 3]
numbers_str = ', '.join(str(num) for num in numbers)
print(numbers_str)
# Output:
# '1, 2, 3'
In this corrected example, we convert each integer in the list to a string using the str()
function before joining them. The result is a single string ‘1, 2, 3’.
Remember, Python’s join()
function is a powerful tool, but it requires careful handling. Always ensure that the iterable you’re joining contains strings, and be mindful of the separator you’re using.
Grasping the Basics: Understanding Python’s String Data Type
To fully comprehend the power of Python’s join()
function, it’s essential to understand the string data type in Python. A string in Python is a sequence of characters enclosed in either single quotes, double quotes, or triple quotes.
Let’s look at an example of a Python string:
greeting = 'Hello, World!'
print(greeting)
# Output:
# 'Hello, World!'
In this example, ‘Hello, World!’ is a string. It’s a sequence of characters enclosed in single quotes.
But strings in Python are more than just sequences of characters. They are also considered as ‘iterables’, which means you can loop over each character in the string. This property is what allows the join()
function to work its magic.
Here’s an example demonstrating this concept:
greeting = 'Hello, World!'
for char in greeting:
print(char)
# Output:
# H
# e
# l
# l
# o
# ,
# (space)
# W
# o
# r
# l
# d
# !
In this example, we loop over each character in the string ‘Hello, World!’ using a for loop. Each character is printed on a new line. This shows that a string in Python is an iterable of its characters.
Understanding this fundamental concept of strings in Python is crucial for mastering the join()
function. It’s the iterable nature of strings that allows us to join them together using different separators.
Broadening Horizons: The Power of String Joining
The join()
function in Python isn’t just a tool for creating neat sentences or phrases. It plays a vital role in various domains like data processing, file handling, and more.
Consider reading data from a CSV file. The data is usually comma-separated, and when you read the file, you might want to join these pieces of data into a single string for further processing. Here’s where the join()
function comes into play.
csv_data = ['John', 'Doe', '[email protected]']
csv_line = ','.join(csv_data)
print(csv_line)
# Output:
# 'John,Doe,[email protected]'
In this example, we’re simulating a line from a CSV file. Each piece of data is a string in a list. We use the join()
function with a comma (‘,’) as the separator to join these strings together into a single string that represents a line in a CSV file.
The join()
function is also a stepping stone to more advanced string manipulation techniques in Python, such as string formatting and regular expressions. If you’re interested in diving deeper, we recommend exploring Python’s format()
function and the re
module for regular expressions.
Mastering the join()
function and these related concepts will give you a strong foundation in handling strings in Python, opening up new possibilities for your coding projects.
Further Learning and Related Topics
To find out more on how to enhance your Python coding skills further, Click Here for a guide on the print() function.
And for additional resources on Python methods, consider the following resources:
- Quick Guide: Converting to Lowercase – Master Python lowercase conversion for consistent data processing and analysis.
Zfill in Python – Explore Python’s zfill() method for padding numeric strings with leading zeros.
Python String Formatting – Discover real Python’s guide on string formatting.
All About Strings in Python – This article provides an understanding of string handling processes in Python.
Python String Manipulation Handbook – FreeCodeCamp provides a handbook on Python string manipulation.
Python’s join()
: A Recap and Comparison
We’ve journeyed through the realms of Python’s join()
function, discovering its usage, potential issues, and solutions. We started with the basic usage, where we learned how to join a list of strings into a single string using a specified separator.
We then delved into more complex scenarios, using different separators for different parts of the string. We also explored alternative methods for joining strings, such as the +
operator and the format()
function. Each method has its advantages and disadvantages, and the best one to use depends on your specific needs.
Finally, we discussed some common issues that you might encounter when using the join()
function, such as trying to join non-string types, and how to overcome them.
Method | Use Case | Pros | Cons |
---|---|---|---|
join() | Joining a list of strings | Efficient, flexible | Only works with strings |
+ Operator | Simple string concatenation | Easy to understand | Inefficient for large numbers of strings |
format() | Complex string formatting | Highly flexible | Can be verbose for simple tasks |
Mastering Python’s join()
function and understanding its alternatives are crucial skills for any Python programmer. Armed with this knowledge, you’re now ready to take on any string joining task in Python!