Python String Interpolation | Learn 3 Efficient Methods
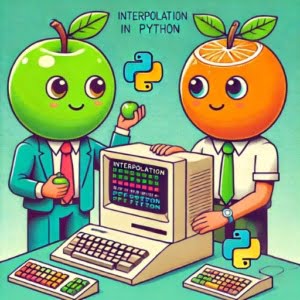
Formatting strings within a script is important to us at IOFLOOD, to ensure consistent results when automating our internal processes. Python string interpolation is our preferred method as it allows for readable string formatting, resulting in clear and concise outputs. Drawing from our notes and experiences, we have crafted today’s article to help our bare metal cloud server customers, and other programmers, master python interpolate string techniques.
In this guide you will find valuable examples and insights on string interpolation in Python, including its syntax, use cases, and best practices.
So, let’s dive in and explore the power of Python with string interpolation!
TL;DR: What is String Interpolation in Python?
String interpolation is a method in Python that allows you to insert variables directly into strings. It can be used with
f-string
formatting, performed with syntax such as,f"Substitute the value {here}"
.
For example:
name = "John"
print(f"Hello, {name}!")
This will output: Hello, John!
.
For more advanced methods, background, tips and tricks on string interpolation, continue reading the article.
Table of Contents
Basics of Python String Interpolation
In the simplest terms, string interpolation is a method of substituting values into placeholders in a string. In Python, this is achieved using specific syntax that allows you to insert variables directly into strings. This feature is incredibly useful as it enables the dynamic generation of string values.
Python offers several ways to format strings, but the f-string
formatting is the most common method. Let’s understand this with a simple example:
age = 25
print(f"John is {age} years old!")
In this example, age
is a variable that holds the integer 25
. The f-string
formatting is used yet again print
function. When the code is run, it will display: John is 25 years old!
Why Use String Interpolation in Python?
You might wonder why to use string interpolation when you can simply concatenate strings? The answer lies in readability and efficiency.
String interpolation results in cleaner, more readable code. It also allows for more complex expressions, like calculations, to be embedded directly into strings.
Advanced String Interpolation Python
Having understood the basics of string interpolation, let’s delve into some advanced use cases. String interpolation extends beyond inserting single variables into strings. It can also be used to insert complex expressions and even call functions. For instance:
x = 10
y = 20
print(f"The sum of {x} and {y} is {x + y}.")
In this example, we’re not merely inserting the variables x
and y
into the string — we’re also calculating their sum directly within the string. The output will be: The sum of 10 and 20 is 30.
Python Interpolate String Examples
String interpolation can accommodate more complex expressions, such as function calls:
def greet(name):
return "Hello, " + name
name = "John"
print(f"{greet(name)}, nice to meet you!")
Here, we’re invoking the greet
function directly within the string interpolation syntax. The output will be: Hello, John, nice to meet you!
To find out more on the different functions built-in to Python, we have an in-depth Cheat Sheet to Python here!
Best Practices: Python Interpolation
While string interpolation is a powerful feature, it’s essential to be aware of potential pitfalls. Here we go over two of them:
Don’t “F” Around
A common mistake is forgetting to prefix the string with f
when using f-string
formatting.
Example of a common pitfall:
name = 'John'
# Incorrect usage (missing f prefix)
print('Hello, {name}!')
In the incorrect usage example above, the output will be Hello, {name}!
instead of Hello, John!
because the f
prefix was missing. Without the f
prefix, Python will interpret the string as a normal string and won’t interpolate the variables.
Syntax and a deep understanding of methods is vital to avoid errors in Python, there are numerous free Python tutorials online that can be used to bolster your knowledge!
NameError for Undefined Variables
Another frequent issue arises when trying to interpolate an undefined variable. Python will raise a NameError
in such cases.
Look at the following example of a common pitfall:
# Incorrect usage (undefined variable 'name')
print(f'Hello, {name}!')
In this example, because name
was not defined before the print statement, Python cannot interpolate it and throws a NameError
with the message: NameError: name 'name' is not defined
.
To avoid this, always make sure that all variables used in your f-string are defined before the string interpolation occurs.
Comparing Python Interpolation Tools
How does string interpolation compare to traditional string formatting in Python?
Method | Readability | Flexibility |
---|---|---|
f-string formatting | High | High |
% operator | Medium | Medium |
str.format() method | Medium | High |
The table above provides a quick comparison of the different string formatting methods in Python. Both methods can be used to insert variables into strings, but string interpolation is generally more readable and concise.
Traditional string formatting, such as using the
%
operator or thestr.format()
method, can become cumbersome with complex strings and multiple variables.
Example of complex string manipulation:
import datetime
name = 'John'
date = datetime.date.today()
print(f'Hello, {name}! Today is {date:%A, %B %d, %Y}.')
The code block above shows a complex example of string manipulation, including a python datetime function call to datetime.date.today()
and a complex expression within the string interpolation.
Beyond String Interpolation in Python
While string interpolation is a key tool in Python, it’s part of a larger toolbox. Other important concepts related to string interpolation include escape sequences (like \n
for a newline), raw strings (which ignore escape sequences), and Unicode strings (which accommodate a wider range of characters).
Example of using escape sequences, raw strings, and Unicode strings:
# Using escape sequence
print('Hello,\nJohn!')
# Using raw string
print(r'Hello,\nJohn!')
# Using Unicode string
print(u'Hello, \u4E16\u754C!')
The code block above shows how to use an escape sequence for a newline, a raw string to ignore the escape sequence, and a Unicode string to print ‘Hello, World!’ in Chinese.
Further Resources for Python Strings
If you’re interested in learning more ways to handle strings in Python, here are a few resources that you might find helpful:
- Streamlining Text Operations with Python String Methods: Streamline your text operations in Python projects by effectively employing various string methods for better results.
Python String Format: Formatting Strings in Python: This tutorial provides an overview of different ways to format strings in Python using the string format() method, with examples and explanations of formatting options.
Python String Replace: Replacing Substrings in a String: Learn how to use the replace() method in Python to replace specific substrings in a string, with examples and explanations on how to use different options for replacement.
String Interpolation in Python: Programiz provides a tutorial on string interpolation in Python, explaining different approaches to embed variables within strings.
PEP 498: Literal String Interpolation: The official Python Enhancement Proposal (PEP) 498 document that introduces literal string interpolation, also known as f-strings, in Python.
Python String Interpolation: GeeksforGeeks offers explanations and examples of string interpolation in Python, covering traditional string formatting and f-strings.
Recap: Python Interpolate String
In Python programming, string interpolation is a key feature. It streamlines the process of inserting variables into strings, enhancing the readability and efficiency of your code.
The applications of string interpolation are extensive, from simplifying code to managing complex expressions and function calls. It’s a tool that is as flexible as it is potent.
Like any tool, it’s vital to use it correctly to avoid common issues, such as forgetting the f
prefix or trying to interpolate undefined variables.
String interpolation is merely one method of string manipulation in Python. Other methods, like the +
operator, %
operator, and str.format()
method, also play a significant role in a Python programmer’s toolkit.
In conclusion, whether you’re a seasoned Python developer or embarking on your programming journey, mastering string interpolation and other string manipulation techniques can elevate your Python skills. So, continue to learn, practice, and code!