Serialization in Java: A Detailed Guide with Examples
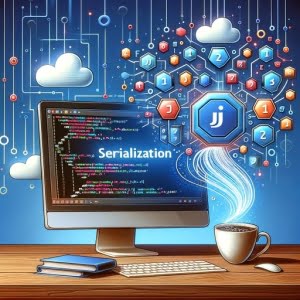
Are you finding it challenging to work with serialization in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling serialization in Java, but we’re here to help.
Think of Java’s serialization as a magic diary – it allows Java to jot down its thoughts (object states) on paper, so it can remember them later. It’s a powerful tool that can help you store and transfer data in a versatile and efficient manner.
In this guide, we’ll walk you through the process of implementing serialization in Java, from the basics to more advanced techniques. We’ll cover everything from how to serialize objects in Java, dealing with potential issues, to exploring alternative approaches and beyond.
So, let’s dive in and start mastering serialization in Java!
TL;DR: What is Serialization in Java?
Serialization in Java is the process of converting an object into a byte stream, which can then be saved to a file or sent over a network. This process is crucial when you need to send objects over a network or store them in files for later retrieval.
Here’s a simple example:
FileOutputStream fileOut = new FileOutputStream('/tmp/employee.ser');
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(e);
out.close();
fileOut.close();
# Output:
# The object 'e' is serialized and stored in '/tmp/employee.ser'
In this example, we create a FileOutputStream
and an ObjectOutputStream
to serialize the object ‘e’. The serialized object is then stored in a file named ’employee.ser’.
This is just a basic introduction to serialization in Java. There’s much more to learn about handling complex objects, dealing with serialization issues, and exploring alternative approaches. Continue reading for a more detailed guide.
Table of Contents
- Basic Serialization in Java: A Step-by-Step Guide
- Advanced Serialization Techniques in Java
- Exploring Alternatives to Java Serialization
- Troubleshooting Common Serialization Issues in Java
- Understanding the Theory Behind Java Serialization
- The Role of Serialization in Larger Java Projects
- Wrapping Up: Mastering Serialization in Java
Basic Serialization in Java: A Step-by-Step Guide
Serialization in Java is a fundamental concept that every developer should grasp. It allows us to convert an object into a byte stream, which can be stored in a file or sent over a network. Let’s dive into a detailed explanation of how to serialize objects in Java.
Step 1: Implement Serializable Interface
The first step in serialization is to implement the Serializable
interface in the class of the object you want to serialize. The Serializable
interface is a marker interface (it has no methods or fields) and serves to inform the JVM that the class can be serialized.
import java.io.Serializable;
class Employee implements Serializable {
String name;
String department;
}
In this example, we have an Employee
class that implements Serializable
. It has two fields: name
and department
.
Step 2: Serialize the Object
Next, we need to serialize the object. This can be done using FileOutputStream
and ObjectOutputStream
.
Employee e = new Employee();
e.name = "John Doe";
e.department = "Engineering";
FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(e);
out.close();
fileOut.close();
# Output:
# The 'e' object is serialized and stored in 'employee.ser'
Here, we create an Employee
object ‘e’, set its fields, and then serialize it. The serialized object is stored in a file named ’employee.ser’.
Benefits and Potential Issues of Serialization
Serialization offers various benefits. It allows you to save the state of an object and retrieve it later. It’s also essential for transferring data over a network, especially in distributed systems.
However, serialization also has potential issues. For instance, it can lead to performance issues if not handled correctly. Serialized objects also need to be deserialized, which can lead to security issues if the source of the serialized object is unknown or untrusted. We’ll delve more into these issues in the ‘Troubleshooting and Considerations’ section.
Advanced Serialization Techniques in Java
As you become more comfortable with basic serialization in Java, it’s time to explore some advanced topics. These include custom serialization, serializing arrays and collections, and dealing with changes to serialized objects.
Custom Serialization in Java
In some cases, you may want to control the serialization process. This is where custom serialization comes into play. You can define the writeObject()
and readObject()
methods in your class to control what data is serialized and how.
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject();
oos.writeObject(encryptedPassword);
}
private void readObject(ObjectInputStream ois) throws ClassNotFoundException, IOException {
ois.defaultReadObject();
this.encryptedPassword = (String) ois.readObject();
}
# Output:
# The 'encryptedPassword' field is now included in the serialization process.
In this example, we’ve included the encryptedPassword
field in the serialization process. This wouldn’t have been possible with default serialization.
Serializing Arrays and Collections
Serialization isn’t limited to single objects. You can also serialize arrays and collections in Java.
ArrayList<String> list = new ArrayList<String>();
list.add("Element1");
list.add("Element2");
FileOutputStream fos = new FileOutputStream("arraylist.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(list);
oos.close();
fos.close();
# Output:
# The ArrayList 'list' is serialized and stored in 'arraylist.ser'.
In this example, we’ve serialized an ArrayList
of String
objects. The serialized list is then stored in a file named ‘arraylist.ser’.
Dealing with Changes to Serialized Objects
One of the challenges with serialization is dealing with changes to objects. If an object is serialized and then its class definition changes, problems can occur when the object is deserialized. Java provides several mechanisms to handle this, such as the serialVersionUID
field.
private static final long serialVersionUID = 1L;
By setting the serialVersionUID
field, you ensure that the class’s serialized objects are compatible with future versions of the class.
These advanced techniques can help you get the most out of serialization in Java. They allow you to handle complex scenarios and ensure your serialized data is safe and compatible.
Exploring Alternatives to Java Serialization
While serialization in Java is a powerful tool, it’s not the only way to save and restore object states. Let’s explore some alternative methods such as using JSON or XML, and compare them with Java serialization.
JSON Serialization
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy to read and write. It’s a popular choice for data serialization, especially in web development.
Gson gson = new Gson();
String json = gson.toJson(employee);
# Output:
# The Employee object is serialized to a JSON string.
In this example, we’re using the Gson library to serialize an Employee object to a JSON string. This method is straightforward and produces a human-readable output. However, it requires using an external library and might not be suitable for complex Java objects.
XML Serialization
XML (eXtensible Markup Language) is another format for data serialization. It’s more verbose than JSON but can represent complex data structures.
JAXBContext jaxbContext = JAXBContext.newInstance(Employee.class);
Marshaller marshaller = jaxbContext.createMarshaller();
StringWriter sw = new StringWriter();
marshaller.marshal(employee, sw);
String xml = sw.toString();
# Output:
# The Employee object is serialized to an XML string.
Here, we’re using JAXB to serialize an Employee object to an XML string. This method can handle complex objects and doesn’t require an external library. However, its output is less human-readable than JSON.
Comparing Alternatives with Java Serialization
While JSON and XML serialization offer benefits, they also have drawbacks. They require additional processing to convert objects to and from strings, which can impact performance. Moreover, they might not support all Java features, such as handling of transient fields.
In contrast, Java serialization is built into the Java platform and supports all Java features. However, it produces binary data, which is not human-readable and can be larger than equivalent JSON or XML. It also has potential security issues, as we discussed in the ‘Troubleshooting and Considerations’ section.
Choosing the right method depends on your use case. If you need to exchange data with non-Java systems or store data in a human-readable format, JSON or XML might be a better choice. If you’re working within a Java environment and need to serialize complex objects, Java serialization might be the way to go.
Troubleshooting Common Serialization Issues in Java
Serialization in Java is a powerful tool, but like any tool, it can sometimes cause issues. Let’s discuss some common problems you might encounter when working with Java serialization and how to address them.
Dealing with Non-Serializable Objects
One common issue is trying to serialize an object that doesn’t implement the Serializable
interface. If you attempt this, Java will throw a NotSerializableException
.
public class NonSerializableClass {
private int data;
}
public class Main {
public static void main(String[] args) throws IOException {
NonSerializableClass obj = new NonSerializableClass();
ObjectOutputStream out = new ObjectOutputStream(new FileOutputStream("test.ser"));
out.writeObject(obj);
}
}
# Output:
# java.io.NotSerializableException: NonSerializableClass
In this example, we tried to serialize an object of NonSerializableClass
, which does not implement Serializable
. This resulted in a NotSerializableException
.
To fix this issue, you need to ensure that the class of the object you’re trying to serialize implements the Serializable
interface.
Handling Version Changes
Another common issue is handling changes to the class of a serialized object. If you serialize an object and then change the class (for example, by adding a new field), you might not be able to deserialize the object.
To handle this, Java provides a mechanism called a serialVersionUID
. This is a unique identifier for each version of a class. If the serialVersionUID
of the serialized object matches that of the class, Java can deserialize the object.
private static final long serialVersionUID = 1L;
By setting the serialVersionUID
field, you ensure that the class’s serialized objects are compatible with future versions of the class.
These are just a few of the issues you might encounter when working with serialization in Java. By understanding these problems and their solutions, you can use serialization more effectively and avoid common pitfalls.
Understanding the Theory Behind Java Serialization
To fully grasp serialization in Java, it’s crucial to understand the theory behind it. Let’s delve into how serialization works, why it’s used, and its relationship with the Java object model and the Serializable interface.
How Serialization Works in Java
Serialization in Java is the process of converting the state of an object into a byte stream. This byte stream can then be saved to a file or sent over a network. When needed, the byte stream can be converted back into an object, a process known as deserialization.
// Serialization
FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(e);
out.close();
fileOut.close();
// Deserialization
FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Employee e = (Employee) in.readObject();
in.close();
fileIn.close();
# Output:
# The 'e' object is serialized and stored in 'employee.ser'. It is then deserialized back into an Employee object.
In this example, we first serialize an Employee object ‘e’ and store it in a file. Then we deserialize the byte stream from the file back into an Employee object.
Why Serialization is Used
Serialization is used for two main reasons: to persist data for future use and to transfer data between different parts of a system (such as between client and server in a network application).
The Role of the Serializable Interface
In Java, only objects of classes that implement the Serializable
interface can be serialized. This interface is a ‘marker’ interface – it doesn’t contain any methods or fields. It simply tells the Java Virtual Machine (JVM) that objects of this class can be serialized.
public class Employee implements Serializable {
// ...
}
# Output:
# The Employee class implements Serializable, so its objects can be serialized.
In this example, the Employee class implements Serializable
. Therefore, objects of the Employee class can be serialized.
Understanding these fundamentals will give you a solid foundation for working with serialization in Java. It will help you understand why serialization is necessary and how to use it effectively in your programs.
The Role of Serialization in Larger Java Projects
Java serialization is not just a standalone concept, it plays a significant role in larger Java projects, particularly in distributed computing and persistent storage.
Serialization in Distributed Computing
In distributed systems, data needs to be exchanged between different parts of the system, potentially running on different machines. Serialization is essential in this context as it allows objects to be converted into a format that can be easily sent over a network.
// Server side
ServerSocket serverSocket = new ServerSocket(9000);
Socket socket = serverSocket.accept();
ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream());
out.writeObject(employee);
// Client side
Socket socket = new Socket("localhost", 9000);
ObjectInputStream in = new ObjectInputStream(socket.getInputStream());
Employee employee = (Employee) in.readObject();
# Output:
# The 'employee' object is serialized and sent from the server to the client over a network.
In this example, a server serializes an Employee
object and sends it over a network. The client then receives the byte stream and deserializes it back into an Employee
object.
Serialization for Persistent Storage
Serialization also plays a critical role in persistent storage. It allows the state of an object to be saved and retrieved later, which is crucial for applications that need to maintain state across sessions.
// Save state
FileOutputStream fileOut = new FileOutputStream("employee.ser");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(employee);
out.close();
fileOut.close();
// Restore state
FileInputStream fileIn = new FileInputStream("employee.ser");
ObjectInputStream in = new ObjectInputStream(fileIn);
Employee employee = (Employee) in.readObject();
in.close();
fileIn.close();
# Output:
# The state of the 'employee' object is saved to a file and then restored from the file.
In this example, the state of an Employee
object is saved to a file. Later, the state is restored from the file, allowing the application to continue from where it left off.
Further Resources for Mastering Java Serialization
To deepen your understanding of Java serialization, here are some valuable resources:
- Best Practices for Java OOPs Concepts – Learn about the Java Object class and its significance.
Using Lambdas in Java – Master lambda expressions for enhancing readability and efficiency in Java programming.
Abstraction in Java – Explore abstraction in Java for modeling complex systems at a higher level.
Java Object Serialization Specification provides in-depth information on how serialization works in Java.
Java Serialization Tutorial – This tutorial from Baeldung covers both basic and advanced topics in Java serialization.
Java Serialization: A Practical Guide – This guide from JournalDev provides examples of serialization in Java, including handling of arrays and collections.
Wrapping Up: Mastering Serialization in Java
In this comprehensive guide, we’ve delved into the world of serialization in Java, a powerful tool for storing and transferring object states.
We began with the basics, exploring how to implement serialization in Java and serialize simple objects. We then ventured into more advanced territory, covering custom serialization, dealing with changes to serialized objects, and serializing arrays and collections.
Along the way, we confronted common challenges associated with Java serialization, such as handling non-serializable objects and managing version changes. We also examined alternative approaches to saving and restoring object states, such as using JSON or XML, and compared them with Java serialization.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Java Serialization | Built into Java, supports all Java features | Produces binary data, potential security issues |
JSON Serialization | Human-readable, widely used | Requires additional processing, might not support all Java features |
XML Serialization | Can represent complex data structures, doesn’t require an external library | Verbose, requires additional processing |
Whether you’re just starting out with serialization in Java or you’re looking to deepen your understanding, we hope this guide has been a valuable resource.
The ability to store and transfer object states is crucial in many Java applications, from simple programs to complex distributed systems. Now, you’re well equipped to leverage the power of serialization in your Java projects. Happy coding!