Abstraction in Java: A Java Concepts Guide
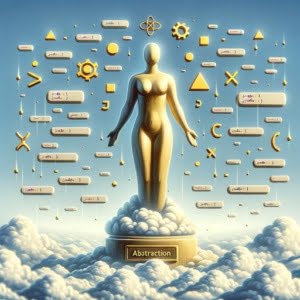
Are you finding it difficult to understand the concept of abstraction in Java? You’re not alone. Many developers find themselves puzzled when it comes to grasping this fundamental object-oriented programming (OOP) concept. Think of Java’s abstraction as a magician’s trick – it hides the complexity of the code behind simple interfaces, allowing you to focus on what the code does, not how it does it.
Abstraction is a powerful tool in Java, enabling us to hide the complex details of how code works and present only the necessary features to the user. It’s a crucial part of Java’s appeal, making code easier to read, write, and maintain.
In this guide, we’ll walk you through the concept of abstraction in Java, from basic understanding to advanced usage. We’ll cover everything from the basics of abstraction, how it works in Java, why it’s important, to more complex uses of abstraction, and even alternative approaches. So, let’s dive in and start mastering abstraction in Java!
TL;DR: What is Abstraction in Java?
Abstraction in Java is a fundamental concept of Object-Oriented Programming (OOP) that hides the complexity of the code and shows only the essential features to the user. It is achieved using abstract classes and interfaces, such as
abstract class Animal { abstract void makeSound(); }
.
Here’s a simple example:
abstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
public void makeSound() {
System.out.println("Bark");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound();
}
}
// Output:
// Bark
In this example, we’ve created an abstract class Animal
with an abstract method makeSound()
. The Dog
class extends Animal
and provides an implementation for the makeSound()
method. In the main
method, we create a Dog
object through the Animal
reference type and call the makeSound()
method, which prints ‘Bark’ to the console.
This is just a basic example of how abstraction works in Java. But there’s much more to learn about abstraction, including more complex uses and alternative approaches. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Grasping the Basics of Abstraction in Java
- Advanced Abstraction: Interfaces and Abstract Methods
- Alternative Approaches to Achieve Abstraction in Java
- Troubleshooting Common Issues with Abstraction in Java
- The Role of Abstraction in Object-Oriented Programming
- Abstraction in Java: The Bigger Picture
- Wrapping Up: Mastering Abstraction in Java
Grasping the Basics of Abstraction in Java
Abstraction, in the context of Java, is an essential principle of Object-Oriented Programming (OOP). Its primary role is to hide the complexity of the code, revealing only the necessary features to the user. This encapsulation of details promotes code modularity and reusability, making your code easier to read and maintain.
One of the primary ways we achieve abstraction in Java is through the use of abstract classes. An abstract class is a class that cannot be instantiated, meaning you cannot create an object of an abstract class. It often contains one or more abstract methods—methods declared without an implementation. Subclasses of the abstract class provide implementations for these abstract methods, thus achieving abstraction.
Let’s take a look at a simple example of an abstract class in Java:
abstract class Vehicle {
abstract void run();
}
class Car extends Vehicle {
public void run() {
System.out.println("The car is running");
}
}
public class Main {
public static void main(String[] args) {
Vehicle myCar = new Car();
myCar.run();
}
}
// Output:
// The car is running
In the code above, we have an abstract class Vehicle
with an abstract method run()
. We then have a Car
class that extends Vehicle
and provides an implementation for the run()
method. In the main
method, we create a Car
object through the Vehicle
reference type and call the run()
method. The output is ‘The car is running’.
This example demonstrates the power of abstraction. The Vehicle
class doesn’t need to know how each type of vehicle runs; it just needs to know that all vehicles can run. This way, the complexity of the code is hidden, and only the essential features are shown to the user.
Advanced Abstraction: Interfaces and Abstract Methods
As you become more comfortable with the basics of abstraction in Java, you can start to explore more complex uses. One such use is the implementation of interfaces and abstract methods.
An interface in Java is a completely abstract class that can only contain abstract methods. It’s another way to achieve abstraction. By using interfaces, you can define what a class should do, but not how it should do it. This is left to the classes that implement the interface.
Let’s dive into a code example to illustrate this concept:
interface Animal {
void makeSound();
}
class Dog implements Animal {
public void makeSound() {
System.out.println("Bark");
}
}
class Cat implements Animal {
public void makeSound() {
System.out.println("Meow");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.makeSound();
Animal myCat = new Cat();
myCat.makeSound();
}
}
// Output:
// Bark
// Meow
In this example, we’ve created an interface Animal
with an abstract method makeSound()
. The Dog
and Cat
classes implement the Animal
interface and provide their own implementations for the makeSound()
method. In the main
method, we create a Dog
and a Cat
object through the Animal
reference type and call the makeSound()
method. The output is ‘Bark’ for the dog and ‘Meow’ for the cat.
This advanced use of abstraction allows us to define a common behavior for all animals (making a sound) but allows each animal to implement that behavior in its own way. This is the power of abstraction in Java.
Alternative Approaches to Achieve Abstraction in Java
While abstract classes and interfaces are common ways to achieve abstraction in Java, they are not the only methods. Other key principles of Object-Oriented Programming (OOP), such as encapsulation and inheritance, can also be used to achieve abstraction.
Encapsulation for Abstraction
Encapsulation is the technique of making the fields in a class private and providing access to the fields via public methods. It’s another way to hide the internal details of how a class works.
Here’s a simple example of encapsulation in Java:
public class Employee {
private String name;
public String getName() {
return name;
}
public void setName(String newName) {
name = newName;
}
}
public class Main {
public static void main(String[] args) {
Employee emp = new Employee();
emp.setName("John");
System.out.println(emp.getName());
}
}
// Output:
// John
In this example, we’ve created an Employee
class with a private field name
. We provide access to this field through the public methods getName()
and setName()
. This way, we are hiding the details of the Employee
class (i.e., its fields) and showing only the necessary features (i.e., the methods).
Inheritance for Abstraction
Inheritance allows us to derive a class from another class, inheriting its fields and methods. This is another way to achieve abstraction, as you can hide the implementation details in the parent class and expose only the necessary features in the child class.
Here’s a simple example of inheritance in Java:
class Animal {
void eat() {
System.out.println("Eating...");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Barking...");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat();
myDog.bark();
}
}
// Output:
// Eating...
// Barking...
In this example, we’ve created a Dog
class that extends an Animal
class. The Dog
class inherits the eat()
method from the Animal
class and adds its own method bark()
. In the main
method, we create a Dog
object and call both the eat()
and bark()
methods.
These alternative approaches to abstraction in Java allow you to hide the complexity of your code and expose only the necessary features, making your code easier to read, write, and maintain.
Troubleshooting Common Issues with Abstraction in Java
As you apply abstraction in Java, you may encounter some common issues. These issues often arise from multiple inheritance and the use of abstract methods. In this section, we’ll discuss these challenges and provide solutions and best practices to overcome them.
Dealing with Multiple Inheritance
Java does not support multiple inheritance with classes. In other words, a class cannot inherit from more than one class. This can become a problem when you want a class to inherit the properties and behaviors of multiple classes.
However, Java provides a workaround: a class can implement multiple interfaces. This allows you to define the behaviors of multiple classes in separate interfaces and have a single class implement all those interfaces.
Here’s a simple example:
interface Eat {
void eat();
}
interface Sleep {
void sleep();
}
class Human implements Eat, Sleep {
public void eat() {
System.out.println("Eating...");
}
public void sleep() {
System.out.println("Sleeping...");
}
}
public class Main {
public static void main(String[] args) {
Human john = new Human();
john.eat();
john.sleep();
}
}
// Output:
// Eating...
// Sleeping...
In this example, we’ve created two interfaces, Eat
and Sleep
, each with one method. The Human
class implements both interfaces, providing its own implementation for each method. This way, we can achieve a form of multiple inheritance in Java.
Managing Abstract Methods
A common issue when working with abstract classes and interfaces is forgetting to implement all abstract methods in the subclass. If a subclass does not provide implementations for all abstract methods of its superclass or interface, it must also be declared as abstract.
To avoid this issue, always ensure that your subclasses provide implementations for all inherited abstract methods. Your IDE will typically warn you if you’ve missed any.
Implementing abstraction in Java can be a complex process, but with these troubleshooting tips, you should be able to navigate common issues and apply best practices effectively.
The Role of Abstraction in Object-Oriented Programming
To fully appreciate the power of abstraction in Java, we need to understand its place in the broader context of Object-Oriented Programming (OOP). Abstraction is one of the four fundamental principles of OOP, alongside encapsulation, inheritance, and polymorphism. These principles guide the design and structure of OOP languages like Java.
Abstraction, at its core, is about simplifying complex systems by breaking them down into smaller, more manageable parts. In OOP, this means breaking down a complex software system into simpler, reusable objects. Each of these objects hides its internal complexity from the outside world, exposing only what’s necessary through a simple interface.
This principle of abstraction makes it easier to manage and maintain complex software systems. It allows developers to focus on one part of the system at a time, without needing to understand the entire system’s complexity.
Here’s a simple example to illustrate this concept:
abstract class Shape {
abstract void draw();
}
class Circle extends Shape {
public void draw() {
System.out.println("Drawing a circle");
}
}
class Square extends Shape {
public void draw() {
System.out.println("Drawing a square");
}
}
public class Main {
public static void main(String[] args) {
Shape myShape = new Circle();
myShape.draw();
myShape = new Square();
myShape.draw();
}
}
// Output:
// Drawing a circle
// Drawing a square
In this example, we’ve created an abstract class Shape
with an abstract method draw()
. The Circle
and Square
classes extend Shape
and provide their own implementations for the draw()
method. In the main
method, we create a Circle
and a Square
object through the Shape
reference type and call the draw()
method. The output is ‘Drawing a circle’ for the circle and ‘Drawing a square’ for the square.
This example demonstrates how abstraction works in OOP. The Shape
class doesn’t need to know how to draw each type of shape; it just needs to know that all shapes can be drawn. This way, the complexity of the code is hidden, and only the essential features are shown to the user.
Abstraction in Java: The Bigger Picture
As you dive deeper into Java and start working on larger projects, you’ll find that abstraction becomes even more crucial. It’s not just about hiding complexity and making code easier to read. Abstraction in Java is also about managing complexity in large software systems and promoting code reusability.
Interplay with Other OOP Concepts
Abstraction in Java doesn’t exist in a vacuum. It’s deeply intertwined with other OOP concepts like encapsulation, inheritance, and polymorphism. These principles often work together to achieve the primary goals of OOP: code reusability and modularity.
For instance, encapsulation—another key OOP concept—also helps to hide the internal state and functionality of an object, similar to abstraction. However, while abstraction focuses on hiding complexity from the user, encapsulation focuses on bundling related data and functionality together within an object.
Inheritance and polymorphism also play a role in abstraction. Inheritance allows a class to inherit properties and methods from another class, promoting code reusability and abstraction. Polymorphism, on the other hand, allows objects of different classes to be treated as objects of a common superclass, providing a level of abstraction for the code that uses these objects.
Further Resources for Mastering Abstraction in Java
To deepen your understanding of abstraction in Java and its interplay with other OOP concepts, here are some resources you may find helpful:
- Fundamentals of Java Object Oriented Programming – Discover the role of constructors in Java object initialization.
Using Serialization in Java – Master serialization for persisting and transmitting object data in Java applications.
Polymorphism in Java – Understand polymorphism in Java for writing flexible and reusable code.
Oracle’s Java Tutorials from the creators of Java, covers everything from the basics to advanced topics, and OOP concepts.
Java Abstraction guide by W3Schools focuses specifically on abstraction in Java, with plenty of examples.
Java Programming Masterclass for Software Developers – This Udemy course covers all aspects of Java programming, and is suitable for programmers of all levels.
Remember, mastering abstraction in Java is not just about understanding the concept itself, but also about understanding how it fits into the broader context of Java and OOP. So keep learning, keep practicing, and keep coding!
Wrapping Up: Mastering Abstraction in Java
In this comprehensive guide, we’ve embarked on a journey to understand the concept of abstraction in Java. We’ve explored how this fundamental principle of Object-Oriented Programming (OOP) can make our code more readable, maintainable, and reusable by hiding its complexity and exposing only the necessary features.
We began with the basics, learning how to use abstract classes in Java to achieve abstraction. We then progressed to more advanced topics, such as using interfaces and abstract methods for more complex abstraction scenarios. We also discussed alternative approaches to achieve abstraction, including encapsulation and inheritance, providing you with a broader perspective on abstraction in Java.
Throughout our journey, we’ve tackled common challenges you might face when implementing abstraction in Java, such as dealing with multiple inheritance and managing abstract methods. We’ve provided solutions and best practices for these issues, helping you navigate the complexities of abstraction.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Abstract Classes | Easy to understand, good for sharing code | Cannot be used for multiple inheritance |
Interfaces | Supports multiple inheritance, good for defining contracts | Cannot hold state |
Encapsulation | Hides internal state, promotes modularity | Not as flexible as interfaces |
Inheritance | Promotes code reusability, easy to understand | Can lead to tightly coupled code |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its core principles, we hope this guide has given you a solid foundation in the concept of abstraction. With this knowledge, you can write cleaner, more efficient Java code and better navigate the complexities of large software projects. Keep practicing, keep learning, and happy coding!