Java Polymorphism: Class and Object Manipulation Guide
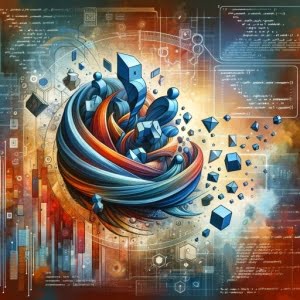
Ever felt like you’re wrestling with understanding polymorphism in Java? You’re not alone. Many developers find the concept of polymorphism a bit daunting. Think of Java’s polymorphism as a chameleon – it allows objects to take on many forms, providing a versatile and handy tool for various tasks.
Polymorphism is a powerful way to extend the functionality of your Java code, making it extremely popular for creating flexible and reusable code.
In this guide, we’ll walk you through the process of understanding and implementing polymorphism in Java, from the basics to more advanced techniques. We’ll cover everything from making simple polymorphic references, handling different types of polymorphism (overloading, overriding, interface polymorphism), to dealing with common issues and even troubleshooting.
Let’s kick things off and learn to master polymorphism in Java!
TL;DR: What is Polymorphism in Java?
Polymorphism in Java is a concept where an object can take on many forms. The most common use of polymorphism in Object-Oriented Programming (OOP) occurs when a parent class reference is used to refer to a child class object, such as in the line of code
Animal myPig = new Pig();
Here’s a simple example:
class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
}
class Pig extends Animal {
void sound() {
System.out.println('The pig says: wee wee');
}
}
Animal myPig = new Pig();
myPig.sound();
// Output:
// 'The pig says: wee wee'
In this example, we have a base class Animal
with a method sound()
. We then create a Pig
class that extends Animal
and overrides the sound()
method. When we create a Pig
object and assign it to an Animal
reference, the overridden sound()
method in the Pig
class is called, demonstrating polymorphism.
This is just a basic example of polymorphism in Java. Continue reading for a more detailed understanding of polymorphism, including its various types and how to use it effectively in your Java programs.
Table of Contents
- Exploring Polymorphism in Java: The Basics
- Diving Deeper: Advanced Polymorphism in Java
- Exploring Alternatives: Abstract Classes and Interfaces
- Troubleshooting Polymorphism in Java: Common Issues and Solutions
- Unpacking OOP: The Backbone of Polymorphism
- Polymorphism in the Bigger Picture: Design Patterns and Frameworks
- Exploring Related Concepts: Abstraction and Encapsulation
- Further Resources for Mastering Polymorphism in Java
- Wrapping Up: Navigating Polymorphism in Java
Exploring Polymorphism in Java: The Basics
Polymorphism, a key pillar of Object-Oriented Programming (OOP), is a Greek word that means ‘many shapes’. In Java, polymorphism allows objects to behave in multiple ways depending on their actual implemented classes.
Polymorphism in Action: A Simple Example
Let’s start with an easy-to-understand example:
// Base class
class Bird {
void fly() {
System.out.println('The bird is flying.');
}
}
// Subclass
class Sparrow extends Bird {
void fly() {
System.out.println('The sparrow flies low.');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Bird myBird = new Sparrow();
myBird.fly();
}
}
// Output:
// 'The sparrow flies low.'
In this example, we have a base class Bird
with a method fly()
. We then create a Sparrow
class that extends Bird
and overrides the fly()
method. When we create a Sparrow
object and assign it to a Bird
reference, the overridden fly()
method in the Sparrow
class is called. This is a basic demonstration of polymorphism in Java.
Advantages of Using Polymorphism
Polymorphism can make your code more flexible and maintainable. It allows you to write code that does not need to be changed when new objects are added, making it easier to develop and upgrade your software.
Potential Pitfalls
While polymorphism is powerful, it can lead to confusion if not used carefully. Overriding methods can lead to unexpected behavior if you’re not aware that a method has been overridden. Also, you can’t use subclass-specific methods and variables when referring to an object with a superclass reference, which can limit functionality.
Diving Deeper: Advanced Polymorphism in Java
As you become more comfortable with polymorphism, you can start exploring its advanced uses. Let’s discuss three more complex forms of polymorphism in Java: method overriding, method overloading, and interface polymorphism.
Method Overriding
Method overriding is a key aspect of polymorphism where a subclass provides a specific implementation of a method that is already defined in its parent class.
// Base class
class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
}
// Subclass
class Dog extends Animal {
void sound() {
System.out.println('The dog says: woof woof');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound();
}
}
// Output:
// 'The dog says: woof woof'
In this example, the Dog
class overrides the sound()
method defined in the Animal
class. When we create a Dog
object and assign it to an Animal
reference, the overridden sound()
method in the Dog
class is called.
Method Overloading
Method overloading is another form of polymorphism where a class can have multiple methods with the same name but different parameters.
// Overloaded methods
class Display {
void show(int num) {
System.out.println('Displaying an integer: ' + num);
}
void show(String str) {
System.out.println('Displaying a string: ' + str);
}
}
// Main class
public class Main {
public static void main(String[] args) {
Display display = new Display();
display.show(10);
display.show('Hello');
}
}
// Output:
// 'Displaying an integer: 10'
// 'Displaying a string: Hello'
In this example, we’ve overloaded the show()
method in the Display
class. When called with an integer, it prints an integer. When called with a string, it prints a string.
Interface Polymorphism
Interface polymorphism is a powerful feature in Java that allows an object to take on multiple forms. This is achieved by implementing multiple interfaces.
// Interfaces
interface Eater {
void eat();
}
interface Sleeper {
void sleep();
}
// Class implementing interfaces
class Human implements Eater, Sleeper {
public void eat() {
System.out.println('The human is eating.');
}
public void sleep() {
System.out.println('The human is sleeping.');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Human human = new Human();
human.eat();
human.sleep();
}
}
// Output:
// 'The human is eating.'
// 'The human is sleeping.'
In this example, the Human
class implements both the Eater
and Sleeper
interfaces. This allows a Human
object to take on the form of an Eater
and a Sleeper
, demonstrating interface polymorphism.
Exploring Alternatives: Abstract Classes and Interfaces
While polymorphism is a powerful tool in Java, there are alternative approaches to achieve similar outcomes. Two such alternatives are abstract classes and interfaces.
Abstract Classes
Abstract classes in Java are classes that contain one or more abstract methods – methods declared without an implementation. Abstract classes cannot be instantiated, but they can be subclassed.
// Abstract class
abstract class Animal {
abstract void sound();
}
// Subclass
class Cat extends Animal {
void sound() {
System.out.println('The cat says: meow meow');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Animal myCat = new Cat();
myCat.sound();
}
}
// Output:
// 'The cat says: meow meow'
In this example, Animal
is an abstract class with an abstract method sound()
. The Cat
class extends Animal
and provides an implementation for sound()
. When a Cat
object is created and assigned to an Animal
reference, the sound()
method in Cat
is called.
Interfaces
An interface in Java is a completely abstract class that can only contain abstract methods. It can be used to achieve full abstraction and multiple inheritance in Java.
// Interface
interface Runner {
void run();
}
// Class implementing interface
class Athlete implements Runner {
public void run() {
System.out.println('The athlete runs fast.');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Runner athlete = new Athlete();
athlete.run();
}
}
// Output:
// 'The athlete runs fast.'
In this example, Runner
is an interface with a method run()
. The Athlete
class implements Runner
and provides an implementation for run()
. When an Athlete
object is created and assigned to a Runner
reference, the run()
method in Athlete
is called.
Making the Right Choice
Choosing between polymorphism, abstract classes, and interfaces depends on your specific needs. If you need to create objects that share a common behavior but also have their unique behaviors, polymorphism is the way to go. If you need to define a template for a group of classes, consider using abstract classes. If you want to define a contract for classes or achieve multiple inheritance, interfaces are your best bet.
Troubleshooting Polymorphism in Java: Common Issues and Solutions
While polymorphism can streamline your Java code, you might encounter some hurdles along the way. Let’s explore some common issues related to polymorphism and how to tackle them.
Type Casting Errors
A common issue when dealing with polymorphism is type casting errors. These usually occur when you attempt to cast an object of one type to another incompatible type.
// Incorrect casting
class Animal {}
class Dog extends Animal {}
// Main class
public class Main {
public static void main(String[] args) {
Animal animal = new Animal();
Dog dog = (Dog) animal; // This will throw a ClassCastException
}
}
In this example, we’re trying to cast an Animal
object to a Dog
object, which throws a ClassCastException
. To avoid this, ensure that the object being cast is actually an instance of the class you’re trying to cast to.
// Correct casting
class Animal {}
class Dog extends Animal {}
// Main class
public class Main {
public static void main(String[] args) {
Animal animal = new Dog(); // This is a Dog object referred to by an Animal reference
Dog dog = (Dog) animal; // This is correct and won't throw an exception
}
}
In this corrected example, we’re assigning a Dog
object to an Animal
reference, then casting it back to a Dog
object. Since the original object was a Dog
, this doesn’t throw an exception.
Method Resolution Problems
Another common issue is method resolution problems. These usually occur when the method to be invoked is determined by the JVM at runtime based on the actual object, not the reference type.
// Method resolution issue
class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
void eat() {
System.out.println('The animal is eating');
}
}
class Dog extends Animal {
void sound() {
System.out.println('The dog says: woof woof');
}
void wagTail() {
System.out.println('The dog is wagging its tail');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.wagTail(); // This will throw a compile error
}
}
In this example, we’re trying to invoke the wagTail()
method on a Dog
object referred to by an Animal
reference. Since wagTail()
is not a method in the Animal
class, this throws a compile error. To avoid this, ensure that the method you’re trying to invoke exists in the reference type’s class.
Remember, understanding the concept of polymorphism and its potential issues is key to effectively using it in your Java programs. With careful implementation and the right troubleshooting techniques, you can harness the full power of polymorphism in Java.
Unpacking OOP: The Backbone of Polymorphism
To fully grasp polymorphism in Java, we need to delve into the principles of Object-Oriented Programming (OOP) that underpin it. OOP is a programming paradigm based on the concept of ‘objects’, which can contain data and code: data in the form of fields (often known as attributes), and code, in the form of procedures (often known as methods).
Inheritance: The Parent-Child Relationship
Inheritance is one of the core principles of OOP. It allows a class (child) to inherit the properties and methods of another class (parent). This means that the child class can reuse the code from the parent class, with the ability to introduce specific behavior.
// Parent class
class Animal {
void sound() {
System.out.println('The animal makes a sound');
}
}
// Child class
class Dog extends Animal {
void sound() {
System.out.println('The dog says: woof woof');
}
}
// Main class
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound();
}
}
// Output:
// 'The dog says: woof woof'
In this example, the Dog
class inherits from the Animal
class. This means Dog
has access to the sound()
method in Animal
. However, Dog
chooses to override this method to provide its own implementation. This is an example of inheritance in action.
Encapsulation: Bundling Data and Methods
Encapsulation is another fundamental principle of OOP. It’s the mechanism of bundling the data (attributes) and the methods that operate on the data. It also hides the complexity of the operations from the users, providing a simple interface.
// Encapsulation example
class Car {
private String color;
// Getter
public String getColor() {
return color;
}
// Setter
public void setColor(String c) {
this.color = c;
}
}
// Main class
public class Main {
public static void main(String[] args) {
Car myCar = new Car();
myCar.setColor('Red');
System.out.println(myCar.getColor());
}
}
// Output:
// 'Red'
In this example, we encapsulate the color
attribute in the Car
class. We provide a getter method getColor()
to access the value of color
, and a setter method setColor()
to modify it. This is an example of encapsulation in action.
The Role of Polymorphism in OOP
Polymorphism, alongside inheritance and encapsulation, is a fundamental principle of OOP. It allows objects to take on many forms, depending on their data types, class or interface. This makes Java code more flexible and dynamic. As we’ve seen in the examples above, polymorphism allows a child class to provide a specific implementation of a method that is already provided by its parent class.
Polymorphism in the Bigger Picture: Design Patterns and Frameworks
Polymorphism isn’t just a standalone concept. It’s a cornerstone of larger Java projects, playing a critical role in design patterns and frameworks. It allows objects of different types to be processed in a uniform way, making it easier to manage complexity in larger software systems.
Polymorphism in Design Patterns
Many design patterns in Java, such as Strategy, State, and Observer, rely on polymorphism. These patterns use polymorphism to provide loose coupling, making the code more flexible and maintainable.
Polymorphism in Frameworks
Java frameworks like Spring and Hibernate heavily utilize polymorphism. For instance, in Spring, polymorphism is used in dependency injection, where a base class reference can be injected with any subclass object at runtime.
Exploring Related Concepts: Abstraction and Encapsulation
Polymorphism is just one piece of the OOP puzzle. To truly master Java programming, it’s essential to understand related concepts like abstraction and encapsulation.
Abstraction is the process of hiding the implementation details and showing only the functionality to the user. Encapsulation, as we discussed earlier, is the technique of making the fields in a class private and providing access through public methods.
Further Resources for Mastering Polymorphism in Java
Ready to dive deeper into polymorphism in Java? Here are some excellent resources to help you on your journey:
- Demystifying Java OOPs Concepts – Explore the principles of code reusability and maintainability in Java OOP.
Using Abstraction in Java – Master abstraction for simplifying program design and maintenance in Java.
Multiple Inheritance in Java – Explore the concept of multiple inheritance in Java and its limitations.
Oracle’s Java Tutorials provides comprehensive tutorials on all Java concepts, including polymorphism.
GeeksforGeeks’ Java Programming collection offers Java programming articles, including discussions on polymorphism.
Baeldung’s Guide to Polymorphism in Java provides a practical approach to understanding polymorphism in Java.
In this comprehensive guide, we’ve taken a deep dive into the world of polymorphism in Java, a fundamental concept in Object-Oriented Programming (OOP) that allows an object to take on many forms.
We began with the basics, understanding what polymorphism is and how it’s implemented in Java. We then explored more advanced techniques, discussing method overriding, method overloading, and interface polymorphism. We also tackled common issues you might encounter when implementing polymorphism, such as type casting errors and method resolution problems, offering solutions to these challenges.
We didn’t stop there. We looked at alternative approaches to achieve similar outcomes, such as abstract classes and interfaces. We also delved into the principles of OOP that underlie polymorphism, like inheritance and encapsulation. Finally, we discussed the role of polymorphism in larger Java projects, including its application in design patterns and frameworks.
Concept | Description | Use Case |
---|---|---|
Polymorphism | Allows an object to take on many forms | When you want to extend the functionality of your code |
Abstract Classes | Defines a template for a group of classes | When you want to provide common behavior for related classes |
Interfaces | Defines a contract for classes | When you want to ensure certain methods are implemented |
Whether you’re a beginner just starting out with Java or an experienced developer looking to level up your understanding of polymorphism, we hope this guide has been a valuable resource. Polymorphism is a powerful tool in your Java toolkit, enabling you to write more flexible and maintainable code. Now, you’re well equipped to harness the power of polymorphism in your future Java projects. Happy coding!