Do-While Loop in Java: Usage Guide with Examples
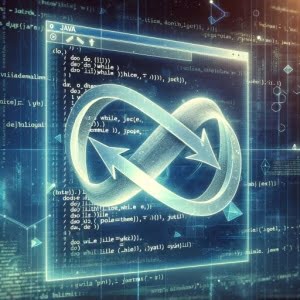
Are you finding it challenging to work with the do-while loop in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this looping construct, but we’re here to help.
Think of Java’s do-while loop as a persistent robot – tirelessly executing a block of code until a certain condition is met. It’s a powerful tool in your Java toolkit, providing a versatile and handy solution for various tasks.
In this guide, we’ll walk you through the process of working with the do-while loop in Java, from basic usage to advanced techniques. We’ll cover everything from the syntax of the loop, its operation, to more complex scenarios and even alternative approaches.
Let’s get started and master the do-while loop in Java!
TL;DR: How Do I Use a Do-While Loop in Java?
The do-while loop in Java is a control flow statement that allows code to be executed at least once, and then repeatedly as long as a certain condition is true. Here’s a simple way to use it:
do { /* code to be executed */ } while (condition);
.
Here’s a simple example:
int count = 0;
do {
System.out.println("Count is: " + count);
count++;
} while (count < 5);
// Output:
// Count is: 0
// Count is: 1
// Count is: 2
// Count is: 3
// Count is: 4
In this example, we initialize a variable count
to 0. The do-while loop then prints the value of count
and increments it. This continues until count
is no longer less than 5, at which point the loop terminates.
This is a basic way to use a do-while loop in Java, but there’s much more to learn about this powerful looping construct. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with the Do-While Loop in Java
The do-while loop in Java is a control flow statement that allows a block of code to be executed at least once and then repeatedly as long as a certain condition remains true. The loop will continue until the condition becomes false. The syntax for a do-while loop is as follows:
do {
// code to be executed
} while (condition);
The ‘do’ keyword signals the start of the loop. The code within the curly braces {} is the block of code that will be executed. The ‘while’ keyword is followed by the condition in parentheses (). The loop will continue to execute as long as this condition is true.
Here’s a simple example of a do-while loop in action:
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, we’ve set up a do-while loop that prints the numbers 0 through 4. The loop continues until the variable i
is no longer less than 5.
Advantages and Pitfalls of the Do-While Loop
One of the main advantages of the do-while loop is that it ensures the code block is executed at least once, regardless of the condition. This is because the condition is checked after the code block has been executed. This can be particularly useful in scenarios where you need to execute a block of code at least once and then continue execution based on a condition.
However, this can also be a pitfall if not handled correctly. If the condition is never met, the do-while loop can result in an infinite loop, causing the program to crash or behave unexpectedly. Therefore, it’s crucial to ensure that your condition will eventually be met to avoid infinite loops.
Advanced Use of Do-While Loop in Java
As you become more comfortable with the do-while loop in Java, you can start to explore more complex uses. This includes scenarios like nested do-while loops and using break and continue statements within your loops.
Nested Do-While Loops
A nested do-while loop is a loop within a loop. The inner loop will complete all its iterations for each single iteration of the outer loop. Here’s an example:
int i = 0, j = 0;
do {
do {
System.out.println(i + ", " + j);
j++;
} while (j < 2);
j = 0;
i++;
} while (i < 2);
// Output:
// 0, 0
// 0, 1
// 1, 0
// 1, 1
In this example, we have an outer loop that iterates over i
and an inner loop that iterates over j
. For each iteration of i
, j
goes from 0 to 1, resulting in four prints to the console.
Using Break and Continue Statements
The break
and continue
statements provide additional control over your do-while loops. The break
statement allows you to exit the loop prematurely, while the continue
statement skips to the next iteration of the loop. Here’s an example that demonstrates both:
int i = 0;
do {
if (i == 2) {
i++;
continue;
}
if (i == 4) {
break;
}
System.out.println(i);
i++;
} while (i < 5);
// Output:
// 0
// 1
// 3
In this example, when i
equals 2, the continue
statement causes the loop to skip the print statement and proceed to the next iteration. When i
equals 4, the break
statement causes the loop to terminate prematurely. As a result, the numbers 0, 1, and 3 are printed to the console.
Alternative Looping Constructs in Java
While the do-while loop is a powerful tool, it’s not the only looping construct in Java. Two other common loop types are the while
loop and the for
loop. Let’s explore these alternatives and how they compare to the do-while loop.
The While Loop
The while
loop is similar to the do-while loop, but with a key difference: the condition is checked before the loop’s code block is executed. This means that if the condition is false to start with, the code block may not run at all.
Here’s a simple while
loop example:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, the output is the same as our do-while loop example. However, if i
was initially set to 5, nothing would be printed because the condition i < 5
would be false from the start.
The For Loop
The for
loop is a bit more complex. It includes initialization, condition, and iteration all in one line. Here’s a simple for
loop example:
for (int i = 0; i < 5; i++) {
System.out.println(i);
}
// Output:
// 0
// 1
// 2
// 3
// 4
Again, the output is the same as the previous examples. However, the for
loop allows us to define the variable i
, set the condition i -1
is always true, so the loop never ends. To avoid infinite loops, always ensure that your condition will eventually become false.
Off-By-One Errors
An off-by-one error occurs when the loop iterates one time too many or one time too few. This often happens due to errors in defining the loop’s condition. Here’s an example:
int i = 0;
do {
System.out.println(i);
i++;
} while (i <= 5);
// Output:
// 0
// 1
// 2
// 3
// 4
// 5
In this example, the loop was supposed to print numbers from 0 to 4, but it went up to 5 because the condition was i <= 5
instead of i < 5
. To avoid off-by-one errors, pay close attention to the loop’s condition and how it relates to the loop’s iterations.
Remember, understanding these common issues and how to avoid them is crucial when working with do-while loops in Java. Always test your loops carefully to ensure they behave as expected.
Understanding Loops and Control Flow in Java
To fully grasp the do-while loop in Java, it’s important to understand the broader concepts of loops and control flow in Java programming.
What are Loops in Java?
Loops are one of the fundamental concepts in programming. They allow a block of code to be repeated multiple times, which can drastically reduce the amount of code you need to write and make your programs more efficient.
In Java, there are three main types of loops: for
, while
, and do-while
. Each type of loop has its own use cases and syntax, but they all serve the same basic purpose: to repeat a block of code.
Understanding Control Flow
Control flow refers to the order in which the statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated. In simpler terms, it’s the order in which your code runs.
In Java, control flow is determined by conditional statements (if
, else if
, else
), loops (for
, while
, do-while
), and function calls. These constructs allow you to control when and how certain parts of your code are executed.
The Role of the Do-While Loop
The do-while loop plays a unique role in Java’s control flow. Unlike the for
and while
loops, which test the loop condition before executing the loop’s code block, the do-while loop checks the condition after the code block has been executed. This means that the code block in a do-while loop is always executed at least once.
Here’s a basic example of a do-while loop in Java:
int i = 0;
do {
System.out.println(i);
i++;
} while (i < 5);
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, the loop prints the numbers 0 through 4. If i
was initially set to 5, the loop would still execute once, printing the number 5, because the condition is checked after the loop’s code block is executed.
Understanding these fundamental concepts will help you better understand the do-while loop and its role in Java programming.
Expanding Your Java Skills: The Do-While Loop and Beyond
While mastering the do-while loop is a significant step in your Java journey, it’s only a part of the larger picture. This looping construct can play a crucial role in more complex programs and projects.
Do-While Loop in Larger Projects
In larger Java projects, the do-while loop can be particularly useful for tasks that need to be executed at least once. This could include initializing a part of your program, processing user input, or running a particular algorithm. The key is to identify situations where a task must be performed at least once and then potentially repeated based on a condition.
Exploring Related Topics: Recursion and Multi-Threading
As you continue to expand your Java knowledge, you might want to explore related topics such as recursion and multi-threading. Recursion involves a function calling itself in its definition, while multi-threading refers to a program executing multiple threads concurrently. Both concepts can be combined with do-while loops and other control flow constructs to create more complex and efficient Java programs.
Further Resources for Mastering Java Loops
To continue your journey towards mastering loops in Java, you might find the following resources helpful:
- Mastering Loops in Java: A Comprehensive Guide – Learn about the various loop structures in Java.
For-Each Loop in Java – Explore the for-each loop in Java for iterating over collections and arrays.
For Loop in Java – Learn about the for loop in Java for iterating over a range of values.
Oracle’s official Java Tutorials cover all aspects of the language, including loops.
Java Loop Control by Tutorials Point goes in-depth into the different control structures in Java.
Java Programming Basics by Udacity covers the basics of Java programming, including control flow statements like the do-while loop.
Remember, mastering any programming concept takes practice. Don’t be afraid to write code, make mistakes, and learn from them. Happy coding!
Wrapping Up: Do-While Loops
In this comprehensive guide, we’ve delved into the do-while loop in Java, a powerful control flow statement that allows a block of code to be executed at least once, and then repeatedly as long as a certain condition remains true.
We started with the basics, learning how to use the do-while loop in its simplest form. We then progressed to more complex uses, including nested do-while loops and the use of break and continue statements within our loops. We also discussed common issues you might encounter when using do-while loops, such as infinite loops and off-by-one errors, and provided solutions to help you avoid these pitfalls.
In addition, we explored alternative looping constructs in Java, such as the while loop and the for loop. We compared their usage, advantages, and disadvantages, giving you a broader understanding of loops in Java. Here’s a quick comparison of these loop types:
Loop Type | Pros | Cons |
---|---|---|
Do-While Loop | Ensures code block is executed at least once | Potential for infinite loops if condition is not carefully controlled |
While Loop | Checks condition before executing code block | Code block may not run at all if condition is initially false |
For Loop | Compact syntax, ideal when number of iterations is known in advance | More complex syntax, may not be suitable for all scenarios |
Whether you’re just starting out with Java or you’re looking to level up your control flow skills, we hope this guide has given you a deeper understanding of the do-while loop and its place within Java’s control flow constructs.
Mastering the do-while loop and other control flow statements is a crucial step in becoming a proficient Java programmer. With practice and understanding, you’ll be able to write more efficient and effective Java code. Happy coding!