Java For Loop: A Detailed Usage Guide
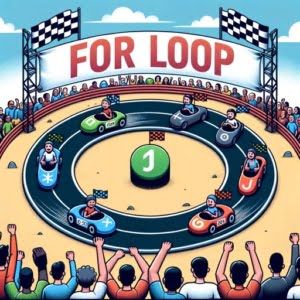
Are you finding it challenging to comprehend for loops in Java? You’re not alone. Many developers find themselves puzzled when it comes to understanding and implementing for loops in Java. Think of a for loop as a factory assembly line, repeating a task for a set number of times, providing a versatile and handy tool for various tasks.
This guide will walk you through the basics to advanced usage of for loops in Java. We’ll cover everything from the structure of a for loop, including initialization, condition, and increment/decrement, to more complex uses such as nested for loops and for-each loops. We’ll also discuss alternative looping constructs in Java, such as while and do-while loops, and when to use them over for loops.
So, let’s dive in and start mastering for loops in Java!
TL;DR: How Do I Use a For Loop in Java?
A basic for loop in Java is structured as follows:
for(initialization; condition; increment/decrement){ //code }
. This structure allows you to repeat a block of code a certain number of times, making it a powerful tool in any Java programmer’s toolkit.
Here’s a simple example:
for(int i = 5; i > 0; i--){
System.out.println(i);
}
// Output:
// 5
// 4
// 3
// 2
// 1
In this example, we initialize a variable i
to 5. The loop continues to execute as long as i
is greater than 0, printing the value of i
each time. After each iteration, i
is decremented by 1. When i
reaches 0, the condition is met.
This is a basic way to use a for loop in Java, but there’s much more to learn about for loops and their various use cases. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
Understanding the Basics of For Loops in Java
A for
loop in Java has a very specific structure. It’s composed of three main parts: initialization, condition, and increment/decrement. Let’s break down each part:
- Initialization: This is where you initialize your loop variable. This part only runs once when the loop starts.
- Condition: This is a Boolean expression that the program checks before each iteration. If the condition is
true
, the loop continues; if it’sfalse
, the loop ends. - Increment/Decrement: This part updates the loop variable after each iteration.
Here’s a basic example of a for loop in Java:
for(int i = 0; i < 5; i++) {
System.out.println(i);
}
// Output:
// 0
// 1
// 2
// 3
// 4
In this example:
- We initialize
i
to 0. - The condition is
i < 5
, which means the loop will continue as long asi
is less than 5. - After each iteration, we increment
i
by 1 (i++
).
So, on each iteration of the loop, the program prints the current value of i
, then increments i
by 1. The loop stops once i
is no longer less than 5.
Exploring Advanced For Loop Concepts in Java
As you become more comfortable with for loops, you’ll find they can be used in more complex ways to handle a variety of tasks. Let’s explore two such concepts: nested for loops and for-each loops.
Nested For Loops
A nested for loop is a loop within a loop. It’s particularly useful when you need to iterate over multi-dimensional arrays or when you want to perform a certain action multiple times for each iteration of the outer loop.
Here’s an example of a nested for loop:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 2; j++) {
System.out.println("i: " + i + ", j: " + j);
}
}
// Output:
// i: 0, j: 0
// i: 0, j: 1
// i: 1, j: 0
// i: 1, j: 1
// i: 2, j: 0
// i: 2, j: 1
In this example, for each iteration of the outer loop (i
), the inner loop (j
) executes twice. So, we end up with six print statements in total.
For-Each Loops
Java also includes a for-each loop, which is used to iterate over elements in arrays or collections. It’s simpler and more readable than a traditional for loop, especially when you don’t need to know the index of the current element.
Here’s an example of a for-each loop:
int[] numbers = {1, 2, 3, 4, 5};
for(int number : numbers) {
System.out.println(number);
}
// Output:
// 1
// 2
// 3
// 4
// 5
In this example, the for-each loop iterates over the numbers
array, and for each iteration, it assigns the current element to the number
variable and then prints it. This makes for-each loops a concise and powerful tool when working with collections in Java.
Exploring Alternative Looping Constructs in Java
While the for
loop is a powerful tool, Java offers other looping constructs that might be more suitable for certain scenarios. Let’s delve into while
and do-while
loops and understand when you might want to use them over for
loops.
While Loops
A while
loop continues executing as long as its condition remains true. Unlike a for
loop, a while
loop doesn’t have a built-in initialization or increment/decrement statement. This makes while
loops ideal for situations where you don’t know how many times you need to loop.
Here’s an example of a while
loop:
int i = 0;
while(i < 5) {
System.out.println(i);
i++;
}
// Output:
// 0
// 1
// 2
// 3
// 4
In this example, we start by initializing i
to 0. The while
loop continues to execute as long as i
is less than 5, printing the value of i
each time. After each iteration, i
is incremented by 1. When i
reaches 5, the loop terminates.
Do-While Loops
A do-while
loop is similar to a while
loop, but with one key difference: the do-while
loop will always execute at least once, because it checks the condition after the loop body.
Here’s an example of a do-while
loop:
int i = 0;
do {
System.out.println(i);
i++;
} while(i < 5);
// Output:
// 0
// 1
// 2
// 3
// 4
Just like the while
loop example, this do-while
loop prints the numbers 0 through 4. However, if the condition were false from the start (for example, if we initialized i
to 5), the do-while
loop would still execute once, while the while
loop would not execute at all.
Choosing between a for
loop, a while
loop, and a do-while
loop depends on your specific needs. If you know exactly how many times you need to loop, a for
loop is usually the best choice. If you don’t know how many times you need to loop, but you know you need to loop at least once, a do-while
loop is the way to go. And if you don’t know how many times you need to loop, and you might not need to loop at all, a while
loop is your best bet.
Troubleshooting Common For Loop Errors in Java
Even experienced developers can encounter errors when using for loops. Let’s discuss some common issues and their solutions, along with some best practices for optimizing your loops.
Infinite Loops
One of the most common errors is creating an infinite loop. This happens when the loop’s condition always evaluates to true. For example:
for(int i = 0; i >= 0; i++) {
System.out.println(i);
}
In this example, i
will always be greater than or equal to 0 because it’s continuously incremented. This creates an infinite loop that will print numbers indefinitely.
To avoid infinite loops, always ensure that your loop’s condition will eventually become false.
Off-By-One Errors
Another common mistake is the off-by-one error. This happens when the loop runs one time too many or one time too few. For instance:
for(int i = 0; i <= 5; i++) {
System.out.println(i);
}
// Output:
// 0
// 1
// 2
// 3
// 4
// 5
In this example, if you only wanted to print numbers 0 through 4, you’ve gone one step too far by including 5. Always double-check your loop’s condition to avoid off-by-one errors.
Best Practices and Optimization
When writing for loops in Java, here are some tips for best practices and optimization:
- Prefer for-each loops when iterating over collections or arrays. They’re more readable and less error-prone than traditional for loops.
- Avoid unnecessary computations in the loop’s condition. If the condition includes a computation that yields the same result on every iteration, compute it once before the loop and store the result in a variable.
- Use
break
andcontinue
judiciously. These statements can control the flow of your loop, but they can also make your code harder to read if overused.
Understanding Loops and Control Flow in Java
To fully grasp the concept of for loops in Java, it’s crucial to understand the underlying principles of loops and control flow in the language.
Loops in Java
In Java, a loop is a control structure that repeats a block of code until a specific condition is met. It’s a way to perform a task multiple times without having to write the same code over and over. Java provides several types of loops, including for
, while
, and do-while
loops.
Control Flow in Java
Control flow refers to the order in which the program’s code executes. In Java, control flow is determined by conditional statements (if
, else if
, else
), loops (for
, while
, do-while
), and jump statements (break
, continue
, return
).
For example, in a for
loop, the control flow starts with the initialization statement, then checks the condition. If the condition is true
, the program executes the loop’s body, then executes the increment/decrement statement, and finally, checks the condition again. This flow continues until the condition is false
.
The Role of Arrays in Loops
Arrays often work hand-in-hand with loops in Java. An array is a data structure that can store a fixed-size sequence of elements of the same type. You can use a loop to iterate over the elements in an array.
For example, here’s a for
loop that prints all elements in an array:
int[] numbers = {1, 2, 3, 4, 5};
for(int i = 0; i < numbers.length; i++) {
System.out.println(numbers[i]);
}
// Output:
// 1
// 2
// 3
// 4
// 5
In this example, the loop iterates over the numbers
array by using the length
property of the array in the loop’s condition. On each iteration, it prints the current element of the array (numbers[i]
).
For Loops in Java: Beyond the Basics
Understanding for loops is just the beginning. They are fundamental building blocks in Java and are used in a multitude of larger programs and projects. Whether you’re working on a simple script or a complex software application, chances are you’ll need to use a for loop at some point.
For Loops in Data Processing
For loops are often used in data processing tasks. For instance, if you’re working with a large dataset, you might need to iterate over the data to perform computations or transformations. In this case, a for loop could be used to iterate over each data point and apply the necessary operations.
For Loops in Game Development
In game development, for loops can be used to control game logic, such as updating the state of game objects or checking for collisions. For example, a for loop could iterate over a list of game objects and update each one’s position based on its velocity.
For Loops and Multithreading
In multithreaded applications, for loops can be used in conjunction with Java’s concurrency utilities to perform tasks in parallel. For example, a for loop could be used to spawn multiple threads, each performing a portion of a larger task.
Further Resources for Java For Loops
Ready to dive deeper into for loops and related topics? Check out these resources for more in-depth information:
- Building Robust Applications with Loops in Java – Understand loop iteration patterns for processing large datasets in Java.
Do-While Loop in Java – Understand the do-while loop in Java for executing a block of code at least once.
Java Continue Statement – Explore the Java continue statement for skipping iterations within loops.
Oracle’s Java Tutorials – A guide to Java, including detailed tutorials on loops and other control flow statements.
Baeldung’s Guide to Java Loops explains for loops and other looping constructs in Java.
GeeksforGeeks’ Java Programming Language section includes articles on for loops, while loops, and other control structures.
Wrapping Up: Java For Loops
In this comprehensive guide, we’ve navigated the world of for loops in Java, a fundamental tool in any Java programmer’s toolkit.
We began with the basics, understanding the structure of a for loop, including initialization, condition, and increment/decrement. We then ventured into more advanced territory, exploring nested for loops and for-each loops, powerful tools for handling complex tasks. We also discussed the alternative looping constructs in Java, the while
and do-while
loops, and when to use them over for loops.
Along the way, we tackled common errors when using for loops, such as infinite loops and off-by-one errors, equipping you with the knowledge to troubleshoot and optimize your loops. We also delved into the relationship between loops and arrays, a common combination in Java programming.
Here’s a quick comparison of the loop constructs we’ve discussed:
Loop Type | Pros | Cons |
---|---|---|
For Loop | Ideal for known iteration counts | Prone to off-by-one errors |
While Loop | Useful for unknown iteration counts | No built-in initialization or increment/decrement |
Do-While Loop | Executes at least once | Less commonly used |
Whether you’re a beginner just starting out with Java or an intermediate developer looking to solidify your understanding of for loops, we hope this guide has been a helpful resource.
Mastering for loops in Java is a significant step in your journey as a Java developer. With this knowledge, you’re well equipped to write efficient, robust code in Java. Happy coding!