Java’s Continue Keyword: A Usage Guide with Examples
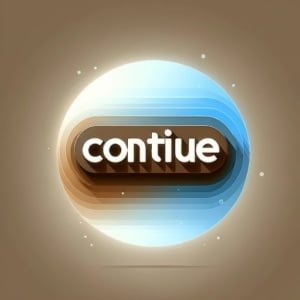
Ever found yourself stuck in a loop in Java, wishing you could skip to the next iteration? You’re not alone. Many developers find themselves in this loop of confusion, but there’s a keyword that can help you break free.
Think of Java’s ‘continue’ keyword as a ‘skip’ button on your remote control. It allows you to jump over the current iteration and move directly to the next one, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of using the ‘continue’ keyword in Java, from its basic usage to more advanced techniques. We’ll cover everything from the fundamentals of control flow in Java, to the relevance of ‘continue’ in larger Java projects and its impact on code readability and performance.
So, let’s dive in and start mastering the ‘continue’ keyword in Java!
TL;DR: How Do I Use the ‘Continue’ Keyword in Java?
The
continue
keyword in Java is used to skip the current iteration of a loop and move directly to the next one, with the syntaxcontinue;
. It’s a powerful tool that can help streamline your code and make your loops more efficient.
Here’s a simple example:
for(int i = 0; i < 10; i++) {
if(i % 2 != 0) {
continue;
}
System.out.println(i);
}
# Output:
# 0
# 2
# 4
# 6
# 8
In this example, we’ve used the ‘continue’ keyword within a for loop. The loop iterates from 0 to 9, but the ‘continue’ keyword is used to skip any iteration where ‘i’ is an odd number. As a result, the code only prints the even numbers between 0 and 9.
This is just a basic way to use the ‘continue’ keyword in Java, but there’s much more to learn about controlling the flow of your loops. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Exploring the ‘Continue’ Keyword: Basic Use in Java
- ‘Continue’ in Nested Loops and with Labels
- Exploring Alternatives to ‘Continue’ in Java
- Troubleshooting ‘Continue’ in Java and Important Considerations
- Understanding Control Flow in Java
- The ‘Continue’ Keyword in Larger Java Projects
- Wrapping Up: The ‘Continue’ Keyword in Java
Exploring the ‘Continue’ Keyword: Basic Use in Java
The ‘continue’ keyword in Java is primarily used within loops. It’s a control statement that tells the loop to stop what it’s doing and start the next iteration. Let’s dive into this concept with a basic example.
for(int i = 0; i <= 10; i++) {
if(i % 2 == 0) {
continue;
}
System.out.println(i);
}
# Output:
# 1
# 3
# 5
# 7
# 9
In this example, the for loop iterates through numbers 0 to 10. Inside the loop, we have an if statement that checks if the current number is even. If it is, the ‘continue’ statement is executed. This immediately stops the current iteration and jumps to the next one, skipping the print statement for even numbers. As a result, only odd numbers between 0 and 10 are printed.
Advantages and Potential Pitfalls
The ‘continue’ keyword can be a powerful tool in your Java toolkit. It can help streamline your loops and make your code more readable by eliminating the need for complex nested if statements.
However, like any tool, it’s important to use it correctly. Overuse of ‘continue’ can lead to code that’s hard to follow, making it difficult for others (or even future you) to understand. It’s also worth noting that ‘continue’ only affects the loop it’s directly inside – if you’re working with nested loops, ‘continue’ will only skip to the next iteration of the innermost loop.
‘Continue’ in Nested Loops and with Labels
As you become more comfortable with the ‘continue’ keyword, you’ll find that its utility extends beyond simple loops. It can be particularly useful in more complex scenarios such as nested loops or when used with labels.
‘Continue’ in Nested Loops
In nested loops, the ‘continue’ keyword operates on the innermost loop. Let’s explore this with an example:
for(int i = 1; i <= 3; i++) {
for(int j = 1; j <= 3; j++) {
if(i == 2) {
continue;
}
System.out.println("i = " + i + ", j = " + j);
}
}
# Output:
# i = 1, j = 1
# i = 1, j = 2
# i = 1, j = 3
# i = 3, j = 1
# i = 3, j = 2
# i = 3, j = 3
In this example, we have a nested loop where ‘i’ and ‘j’ iterate from 1 to 3. If ‘i’ equals 2, the ‘continue’ statement is executed. This skips the current iteration of the inner loop, resulting in no output for ‘i’ equals 2.
‘Continue’ with Labels
Java allows you to label loops, and you can use ‘continue’ with a label to skip to the next iteration of the labeled loop. Let’s see how this works:
outerLoop:
for(int i = 1; i <= 3; i++) {
for(int j = 1; j <= 3; j++) {
if(i == 2) {
continue outerLoop;
}
System.out.println("i = " + i + ", j = " + j);
}
}
# Output:
# i = 1, j = 1
# i = 1, j = 2
# i = 1, j = 3
# i = 3, j = 1
# i = 3, j = 2
# i = 3, j = 3
In this revised example, we’ve added a label ‘outerLoop’ to the outer loop. Now, when ‘i’ equals 2, ‘continue outerLoop’ is executed. This skips the current iteration of the outer loop, not the inner loop, resulting in the same output as before.
Best Practices
While the ‘continue’ keyword can be powerful, it’s important to use it sparingly and thoughtfully. Overuse can lead to code that’s hard to follow and debug. Always consider if there’s a simpler way to achieve the same result without using ‘continue’.
Exploring Alternatives to ‘Continue’ in Java
While the ‘continue’ keyword is a powerful tool in Java, it’s not always the best or only solution. There are several alternatives that can sometimes be more suitable depending on the situation. Let’s explore these alternatives and their advantages.
Using ‘Break’
The ‘break’ keyword, like ‘continue’, is used to control the flow of loops. However, instead of skipping the current iteration, ‘break’ terminates the loop entirely.
for(int i = 0; i < 10; i++) {
if(i == 5) {
break;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the loop terminates as soon as ‘i’ equals 5, and the numbers 0 through 4 are printed.
Redesigning the Loop
Sometimes, a loop can be redesigned to avoid the need for ‘continue’. This can often result in cleaner, more readable code.
for(int i = 0; i < 10; i++) {
if(i % 2 != 0) {
System.out.println(i);
}
}
# Output:
# 1
# 3
# 5
# 7
# 9
In this example, we’ve redesigned the loop to only print the number if ‘i’ is odd, eliminating the need for ‘continue’.
Using a Different Data Structure
In some cases, using a different data structure, like a LinkedList or a HashMap, can make certain operations more efficient, reducing or eliminating the need for ‘continue’.
While these alternatives can be useful, it’s important to remember that each has its own trade-offs. ‘Break’ can make your code harder to follow by abruptly ending loops, redesigning your loop can be time-consuming or not possible in complex scenarios, and using a different data structure may increase memory usage. As always, the best approach depends on your specific situation.
Troubleshooting ‘Continue’ in Java and Important Considerations
While the ‘continue’ keyword is a handy tool in Java, it’s not without its quirks and potential pitfalls. Let’s discuss some common issues you may encounter when using ‘continue’, and provide solutions and workarounds for each issue.
Confusion with ‘Break’
One common issue developers face is confusion between ‘continue’ and ‘break’. While both are used to control the flow of loops, they function differently. ‘Continue’ skips the current iteration and moves to the next, while ‘break’ terminates the loop entirely.
Misuse in Nested Loops
In nested loops, ‘continue’ only affects the innermost loop. If you want to skip to the next iteration of an outer loop, you’ll need to use a labeled ‘continue’ statement. Without the label, ‘continue’ may not behave as expected.
outerloop:
for(int i = 0; i < 5; i++) {
for(int j = 0; j < 5; j++) {
if(j == 2) {
continue outerloop;
}
System.out.println("i = " + i + ", j = " + j);
}
}
# Output:
# i = 0, j = 0
# i = 0, j = 1
# i = 1, j = 0
# i = 1, j = 1
# i = 2, j = 0
# i = 2, j = 1
# i = 3, j = 0
# i = 3, j = 1
# i = 4, j = 0
# i = 4, j = 1
In this example, ‘continue outerloop’ is used to skip to the next iteration of the outer loop whenever ‘j’ equals 2. This results in only two iterations of the inner loop for each iteration of the outer loop.
Remember, understanding the flow of your loops and how ‘continue’ interacts with them is crucial for writing efficient and bug-free code.
Understanding Control Flow in Java
To fully appreciate the role and utility of the ‘continue’ keyword in Java, it’s essential to grasp the concept of control flow. Control flow refers to the order in which the instructions, statements, or function calls of an imperative or a declarative program are executed or evaluated.
Loops and Conditional Statements in Java
In Java, control flow is often managed through loops and conditional statements. Loops allow a block of code to be executed repeatedly, while conditional statements enable the program to choose different paths of execution based on certain conditions.
for(int i = 0; i < 5; i++) {
if(i % 2 == 0) {
System.out.println(i + " is even");
} else {
System.out.println(i + " is odd");
}
}
# Output:
# 0 is even
# 1 is odd
# 2 is even
# 3 is odd
# 4 is even
In this example, the for loop iterates five times. Inside the loop, the if-else conditional statement checks if the current number is even or odd and prints the result.
Related Keywords: ‘Break’ and ‘Return’
In addition to ‘continue’, Java has other keywords that help control the flow of execution. The ‘break’ keyword, like ‘continue’, is used within loops and switch statements. However, instead of skipping to the next iteration, ‘break’ terminates the loop or switch statement entirely.
The ‘return’ keyword is used to finish the execution of a method, and optionally return a value from a method. It can be used to exit a method and return to the caller before the end of the method is reached.
Understanding these fundamentals of control flow in Java will give you a solid foundation to effectively use the ‘continue’ keyword and its counterparts.
The ‘Continue’ Keyword in Larger Java Projects
In larger Java projects, the ‘continue’ keyword can greatly impact code readability and performance. It allows developers to control the flow of loops, making the code more efficient and easier to understand. However, it’s crucial to use ‘continue’ judiciously to avoid creating code that’s difficult to follow or debug.
The Impact on Code Readability
Well-placed ‘continue’ statements can make your code more readable by eliminating the need for nested conditional statements. This can make your code easier to understand and maintain, particularly in larger projects.
The Impact on Performance
In terms of performance, the ‘continue’ keyword can help make your loops more efficient by skipping unnecessary iterations. However, it’s worth noting that the performance gain is usually minimal and should not be the primary reason for using ‘continue’.
Related Topics to Explore
If you’re interested in further enhancing your Java skills, there are several related topics that you may find beneficial. Exception handling in Java, for example, is a crucial aspect of writing robust and error-free code. Multithreading in Java is another advanced topic that can help you write more efficient and responsive applications.
Further Resources for Mastering Java Control Flow
To deepen your understanding of control flow in Java and the use of the ‘continue’ keyword, you may find the following resources helpful:
- Your Solution for Iteration: Loops in Java – Learn about the benefits of using loops over manual iteration in Java.
Java Break Statement – Understand the Java break statement for prematurely exiting loop structures.
Java For Loop – Understand the syntax and usage of for loops for controlled iteration.
Oracle’s Java Tutorials cover all aspects of Java programming, including control flow statements.
Java Code Geeks offers a wealth of articles and tutorials on various Java topics, including control flow.
Baeldung’s Guide on Java Control Flow provides an in-depth look at control flow in Java, including ‘continue’.
Wrapping Up: The ‘Continue’ Keyword in Java
In this comprehensive guide, we’ve dived deep into the world of Java’s ‘continue’ keyword, exploring its usage, benefits, and potential pitfalls.
We began with the basics, learning how to use the ‘continue’ keyword in simple loops. We then advanced to more complex scenarios, such as nested loops and labeled ‘continue’ statements, empowering you to control the flow of your loops with greater precision and flexibility.
Along the way, we tackled common challenges you might encounter when using ‘continue’, such as confusion with ‘break’ and misuse in nested loops. We provided solutions and workarounds for each issue, equipping you with the knowledge to write efficient and bug-free code.
We also explored alternatives to ‘continue’, such as using ‘break’, redesigning the loop, or using a different data structure. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Continue | Skips current iteration, efficient in loops | Can lead to hard-to-follow code if overused |
Break | Terminates the loop, useful when a certain condition is met | Abruptly ends the loop, can lead to unexpected results |
Redesigning the Loop | Can eliminate the need for ‘continue’ | Time-consuming, might not be possible in complex scenarios |
Different Data Structure | Can make certain operations more efficient | Might increase memory usage |
Whether you’re a beginner just starting out with Java or an experienced developer looking to hone your skills, we hope this guide has given you a deeper understanding of the ‘continue’ keyword and its role in controlling the flow of loops in Java.
With its ability to skip unnecessary iterations and streamline your loops, the ‘continue’ keyword is a powerful tool in your Java toolkit. Happy coding!