Java Break Statement: Exiting Loop Control Structures
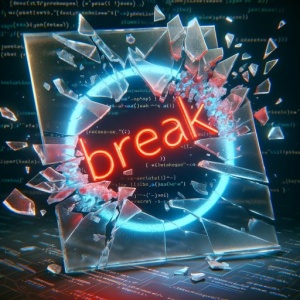
Are you finding it challenging to manage your loops in Java? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a seasoned conductor, the break statement can help you orchestrate your code’s flow. These statements can effectively control loops in Java, making them an essential tool for any Java developer.
This guide will walk you through the ins and outs of the break statement in Java, from basic use to advanced techniques. We’ll explore the break statement’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering the break statement in Java!
TL;DR: How Do I Use the Break Statement in Java?
The break statement in Java is used to exit a loop or a switch statement prematurely with the syntax,
break;
usually placed after a condition. It allows you to control the flow of your code by breaking out of a loop when a certain condition is met.
Here’s a simple example:
for(int i = 0; i < 10; i++) {
if(i == 3) {
break;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
In this example, we have a for loop that is supposed to print numbers from 0 to 9. However, we’ve used the break statement to exit the loop when i
equals 3. As a result, the loop only prints numbers from 0 to 2.
This is a basic way to use the break statement in Java, but there’s much more to learn about controlling the flow of loops. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Break Statement: The Basics
The break
statement in Java is primarily used to terminate the loop or switch statement in which it is present. As soon as the break statement is encountered within a loop, the loop is immediately terminated, and the program control resumes at the next statement following the loop.
Let’s look at a basic example of how the break
statement works in a for loop:
for(int i = 0; i < 10; i++) {
if(i == 5) {
break;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the break
statement is used to exit the loop when i
equals 5. The loop was supposed to print numbers from 0 to 9, but due to the break
statement, it only prints numbers from 0 to 4.
The break
statement can also be used in switch statements. In a switch statement, break
is used to exit the switch case and resume the next line of code after the switch statement. If a break
statement was not used in a switch case, all cases after the correct one would be executed until a break
is encountered.
Here is an example of how break
is used in a switch statement:
int day = 3;
switch(day) {
case 1:
System.out.println("Monday");
break;
case 2:
System.out.println("Tuesday");
break;
case 3:
System.out.println("Wednesday");
break;
default:
System.out.println("Invalid day");
}
# Output:
# Wednesday
In this example, the break
statement is used to exit the switch statement after the correct case is executed. Without the break
statement, if the day was 2, the output would be “Tuesday”, “Wednesday”, “Invalid day”.
While the break
statement can be quite useful, it’s crucial to use it judiciously. Overuse of the break
statement can make your code less readable and harder to debug. It’s generally recommended to use the break
statement sparingly and only when necessary.
Break Statement in Nested Loops
When it comes to nested loops, the break
statement can behave a bit differently. In nested loops, a break
statement will only break out of the innermost loop it is placed in, not all of the loops.
Let’s dive into an example to illustrate this:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
if(j == 2) {
break;
}
System.out.println("i: " + i + " j: " + j);
}
}
# Output:
# i: 0 j: 0
# i: 0 j: 1
# i: 1 j: 0
# i: 1 j: 1
# i: 2 j: 0
# i: 2 j: 1
In this example, we have two nested for
loops. The inner for
loop contains a break
statement that exits the loop when j
equals 2. However, this break
statement only affects the inner loop. The outer loop continues to iterate as normal.
This is a crucial detail to understand when working with nested loops. If you want to break from all loops, not just the innermost one, you may need to use a different approach, such as implementing a flag variable or using labeled break
statements.
While the break
statement is a powerful tool, it’s essential to understand its behavior in different contexts to use it effectively. By understanding how the break
statement works in nested loops, you can write more efficient and readable code.
Exploring Alternatives to Java Break
While the break
statement is a powerful tool for controlling loop execution, Java offers other methods that can provide more nuanced control. Let’s delve into two of these alternatives: the continue
statement and the labeled break
statement.
The Continue Statement
The continue
statement skips the current iteration of a loop and jumps to the next one. It can be particularly useful when you want to skip a specific iteration rather than entirely breaking out of the loop.
Here’s an example of how continue
works:
for(int i = 0; i < 10; i++) {
if(i == 5) {
continue;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 3
# 4
# 6
# 7
# 8
# 9
In this example, instead of breaking out of the loop when i
equals 5, we skip the iteration. Thus, the number 5 is not printed, but the loop continues to print the remaining numbers.
Labeled Break Statement
Java allows you to label your loops and then use those labels as targets for break
statements. This can be beneficial when dealing with nested loops, as a break
statement with a label will exit the specified loop, not just the innermost one.
Let’s look at an example:
outerloop:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
if(i == j) {
break outerloop;
}
System.out.println("i: " + i + " j: " + j);
}
}
# Output:
# i: 1 j: 0
In this example, when i
equals j
, the break
statement exits the outerloop
, not just the inner loop. This allows you to have more control over which loop you want to break out of.
These alternative methods provide more flexibility in controlling loop execution. However, they also require careful handling to avoid confusion and ensure code readability. Whether you choose to use break
, continue
, or labeled break
should depend on the specific needs of your code and what you find most readable and understandable.
Troubleshooting Java Break Statement
While the break
statement in Java is quite straightforward, it can sometimes lead to unexpected results, especially when used in nested loops or complex control structures. Let’s discuss some common issues you might encounter and how to resolve them.
Breaking from Nested Loops
One of the most common issues with the break
statement is its behavior in nested loops. As we discussed earlier, a break
statement will only break out of the innermost loop. If you want to break out of an outer loop, you’ll need to use a labeled break
statement.
Here’s an example of a common mistake and how to correct it using a labeled break
statement:
// Incorrect usage
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
if(i == j) {
break;
}
System.out.println("i: " + i + " j: " + j);
}
}
// Correct usage
outerloop:
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
if(i == j) {
break outerloop;
}
System.out.println("i: " + i + " j: " + j);
}
}
# Output:
# i: 1 j: 0
In the incorrect usage, the break
statement only breaks out of the inner loop, not the outer one. By using a labeled break
statement, we can break out of the outerloop
when i
equals j
.
Overuse of Break Statements
Another common issue is the overuse of break
statements. While break
can be a powerful tool for controlling flow, overusing it can make your code harder to read and debug. Instead, consider whether you can achieve the same result with a well-crafted conditional statement or by using the continue
statement to skip certain iterations.
The break
statement is a powerful tool in Java, but like all tools, it should be used judiciously and appropriately. By understanding its behavior and potential issues, you can use the break
statement effectively to control your code’s flow.
Understanding Java Loop Control
To fully grasp the break
statement’s functionality, it’s essential to understand the fundamental concepts of loop control in Java. Loop control statements are used to handle the flow of control in loops and switch cases.
Loop Control in Java
In Java, loops are used to repeatedly execute a block of code until a specific condition is met. The control flow of loops is managed by three key statements: break
, continue
, and return
.
- The
break
statement, as we’ve discussed, is used to exit the loop prematurely. - The
continue
statement skips the current iteration and jumps to the next one. - The
return
statement exits not just the loop but the entire method.
Here’s a simple example illustrating these statements:
for(int i = 0; i < 10; i++) {
if(i == 3) {
continue;
}
if(i == 5) {
break;
}
System.out.println(i);
}
# Output:
# 0
# 1
# 2
# 4
In this example, the continue
statement skips the loop iteration when i
equals 3, so 3 is not printed. The break
statement exits the loop when i
equals 5, so the numbers after 4 aren’t printed.
Understanding these fundamental concepts of loop control is key to mastering the break
statement and controlling your code’s flow effectively.
Break Statement in Larger Java Projects
The break
statement is not just for simple loops in small programs; it’s equally relevant in larger, more complex Java projects. In fact, as projects grow and become more complex, the need for loop control and flow management becomes even more critical.
In a large project, you might have multiple nested loops, complex switch cases, and intricate control flows. The break
statement can help manage these complex structures, making your code more readable and maintainable.
Exploring Related Concepts
While mastering the break
statement is an important step, there’s a lot more to controlling flow in Java. Exception handling, for example, is a crucial concept that allows you to manage errors and exceptional conditions in your code.
Multithreading is another advanced concept in Java that involves running multiple threads in a single program. The break
statement and other loop control mechanisms play a key role in managing the flow of these threads.
Further Resources for Mastering Java Break
To deepen your understanding of the break
statement and related concepts, here are some resources you might find useful:
- Tips and Techniques for Using Loops in Java – Explore real-world examples of loop usage in Java applications.
If Statement in Java – Learn about the if statement in Java for conditional execution of code blocks.
Continue Statement in Java – Master the continue statement for fine-grained control over loop execution in Java
Java Break and Continue tutorial from W3Schools provides a comprehensive overview of the
break
andcontinue
statements in Java.Java Control Flow Statements provides an in-depth look at control flow statements, including
break
.Java Multithreading guide delves into the concept of multithreading in Java.
Wrapping Up: Java Break Statement
In this comprehensive guide, we’ve delved into the intricacies of the break
statement in Java. We’ve explored its basic usage, advanced application, and even discussed alternative approaches to handle loop control.
We began with the basics, understanding how to use the break
statement in loops and switch statements. We then moved on to more advanced usage, discussing its behavior in nested loops and how to use labeled break
statements for greater control. Along the way, we also explored common issues and their solutions, ensuring you’re well-equipped to handle any challenges that come your way.
We’ve also discussed alternative approaches to control loop execution. Specifically, we explored the continue
statement and labeled break
statement, both of which offer more nuanced control over your loops.
Here’s a quick comparison of these methods:
Method | Use Case | Pros | Cons |
---|---|---|---|
Break | To exit a loop prematurely | Simple and straightforward | Only breaks the innermost loop |
Continue | To skip a particular iteration | Provides more control over loop execution | Can lead to confusion if overused |
Labeled Break | To break a specific loop in nested loops | Provides precise control in complex structures | Requires careful handling to avoid confusion |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up your skills, we hope this guide has provided you with a deeper understanding of the break
statement and its alternatives. The ability to control the flow of your loops is a powerful tool in Java, and now you’re well-equipped to use it effectively. Happy coding!