For-each Loops in Java | An Enhanced For-Loop Guide
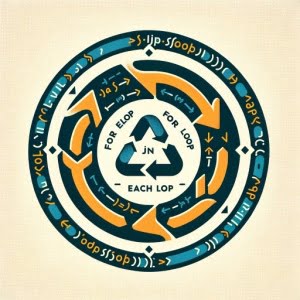
Are you finding it challenging to work with the for-each loops in Java? You’re not alone. Many developers find themselves grappling with this task, but there’s a tool that can make this process simpler and more efficient.
Think of the for-each loop in Java as a well-oiled machine – it can streamline your code and make your collections easier to work with. It’s a powerful tool that can make your coding life significantly easier once you’ve mastered it.
This guide will walk you through everything you need to know about using the for-each loop in Java. We’ll cover everything from the basics of using the for-each loop to more advanced techniques, as well as alternative approaches. We’ll also discuss common issues you may encounter and their solutions.
So, let’s dive in and start mastering the for-each loop in Java!
TL;DR: How Do I Use the For-Each Loop in Java?
The
for-each
loop syntax in Java is as follows:for (dataType variableName : collectionName)
. You write a loop that specifies a variable type and name, followed by a colon, and then the collection you’re iterating over. This loop is also known as an'enhanced for loop'
, as it simplifiesfor
loop syntax.
Here’s a simple example:
ArrayList<String> collection = new ArrayList<>(Arrays.asList("Apple", "Banana", "Cherry"));
for (String item : collection) {
System.out.println(item);
}
# Output:
# Apple
# Banana
# Cherry
In this example, we have an ArrayList named collection
that contains three items. The for-each loop iterates over each item in the collection and prints it to the console. The variable item
takes on the value of each element in the collection, one by one, and the System.out.println(item);
statement prints the value of item
during each iteration.
This is a basic way to use the for-each loop in Java, but there’s much more to learn about this powerful tool. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the For-Each Loop in Java
- Complex Uses of the For-Each Loop in Java
- Exploring Alternatives to the For-Each Loop in Java
- Troubleshooting Common Issues with For-Each Loop in Java
- Fundamentals: Loops and Iteration in Java
- The For-Each Loop in Real-World Applications
- Wrapping Up: Mastering the For-Each Loop in Java
Understanding the For-Each Loop in Java
The 'for-each'
loop, also known as the 'enhanced for loop'
, is a simplified way of iterating over collections in Java, such as arrays and ArrayLists. It eliminates the need to use counters or indices, making your code more readable and less prone to errors.
Here’s a basic example:
ArrayList<String> groceries = new ArrayList<>(Arrays.asList("Milk", "Eggs", "Bread"));
for (String item : groceries) {
System.out.println(item);
}
# Output:
# Milk
# Eggs
# Bread
In this example, we have an ArrayList named groceries
that contains three items. The for-each loop iterates over each item in the groceries
list and prints it to the console. The variable item
takes on the value of each element in the groceries
list, one by one, and the System.out.println(item);
statement prints the value of item
during each iteration.
Advantages of Using the For-Each Loop
The for-each loop in Java has several advantages:
- Readability: The for-each loop is easier to read and write, which can make your code cleaner and more understandable.
- Error Reduction: By eliminating the need for a counter or index, the for-each loop minimizes the risk of off-by-one errors, which are common in traditional for loops.
- Efficiency: The for-each loop automatically handles the traversal of the collection, making it more efficient and less prone to errors.
Potential Pitfalls
While the for-each loop is powerful and useful, it does have some limitations:
- Unmodifiable: You cannot modify the collection while iterating through it with a for-each loop. Attempting to do so will result in a
ConcurrentModificationException
. - Single-Direction Iteration: The for-each loop can only iterate forward, not backward, through a collection.
- No Access to Index: The for-each loop does not provide access to the index of the current element. If you need the index, you may need to use a traditional for loop or an iterator.
Complex Uses of the For-Each Loop in Java
As you become more comfortable with the for-each loop, you may find yourself needing to use it in more complex scenarios. Two such scenarios include iterating over multidimensional arrays and custom objects.
Iterating Over Multidimensional Arrays
A multidimensional array is an array of arrays. Here’s how you can use the for-each loop to iterate over a multidimensional array:
int[][] numbers = {{1, 2}, {3, 4}, {5, 6}};
for (int[] innerArray : numbers) {
for (int number : innerArray) {
System.out.println(number);
}
}
# Output:
# 1
# 2
# 3
# 4
# 5
# 6
In this example, we have a 2D array called numbers
. We use a nested for-each loop to iterate over each element in the 2D array. The outer loop iterates over the inner arrays, and the inner loop iterates over the elements of each inner array.
Iterating Over Custom Objects
You can also use the for-each loop to iterate over collections of custom objects. Here’s an example where we have a class Person
and a list of Person
objects:
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return name + " (age: " + age + ")";
}
}
List<Person> people = Arrays.asList(new Person("Alice", 30), new Person("Bob", 35));
for (Person person : people) {
System.out.println(person);
}
# Output:
# Alice (age: 30)
# Bob (age: 35)
In this example, we have a Person
class with a name
and age
field, and a toString()
method that returns a string representation of the object. We then create a list of Person
objects and use a for-each loop to iterate over the list and print each Person
object.
Exploring Alternatives to the For-Each Loop in Java
While the for-each loop is a powerful tool for iterating over collections in Java, it’s not the only tool available. Let’s explore two alternatives: the traditional for loop and the iterator method.
Using the Traditional For Loop
The traditional for loop gives you more control than the enhanced for loop, as it allows you to access the index of the current element and modify the collection while iterating.
Here’s an example of how you can use the traditional for loop to iterate over an ArrayList:
ArrayList<String> groceries = new ArrayList<>(Arrays.asList("Milk", "Eggs", "Bread"));
for (int i = 0; i < groceries.size(); i++) {
System.out.println(groceries.get(i));
}
# Output:
# Milk
# Eggs
# Bread
In this example, we use a counter i
to access each element in the groceries
list by its index. This gives us the ability to modify the list while iterating, which is something you can’t do with a for-each loop.
Using the Iterator Method
The Iterator interface provides methods to iterate over any Collection. It is more flexible than the for-each loop because it allows you to remove elements from the collection during the iteration.
Here’s an example of using an Iterator to iterate over an ArrayList:
ArrayList<String> groceries = new ArrayList<>(Arrays.asList("Milk", "Eggs", "Bread"));
Iterator<String> iterator = groceries.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
System.out.println(item);
}
# Output:
# Milk
# Eggs
# Bread
In this example, we create an Iterator for the groceries
list. The hasNext()
method checks if there’s another element in the list, and the next()
method retrieves the next element.
Choosing the Right Method
The for-each loop, traditional for loop, and Iterator method each have their strengths and weaknesses. The for-each loop is the most readable but the least flexible. The traditional for loop and Iterator method are less readable but more flexible.
The best method to use depends on your specific needs. If you need to modify the collection during the iteration or need access to the index of the current element, you might want to use a traditional for loop or an Iterator. If you simply need to iterate over a collection without modifying it, the for-each loop is a great choice.
Troubleshooting Common Issues with For-Each Loop in Java
Like any programming tool, the for-each loop in Java isn’t without its potential issues. Let’s discuss some common problems you might encounter, along with their solutions.
Dealing with ConcurrentModificationException
A common problem when working with the for-each loop is the ConcurrentModificationException
. This exception is thrown when you try to modify the collection while iterating through it with a for-each loop.
Here’s an example of a situation that would cause a ConcurrentModificationException
:
ArrayList<String> groceries = new ArrayList<>(Arrays.asList("Milk", "Eggs", "Bread"));
for (String item : groceries) {
if (item.equals("Eggs")) {
groceries.remove(item);
}
}
# Output:
# Exception in thread "main" java.util.ConcurrentModificationException
In this example, we’re trying to remove an item from the groceries
list while iterating over it, which causes a ConcurrentModificationException
.
To avoid this exception, you can use an Iterator, which allows you to safely remove elements from the collection during the iteration:
ArrayList<String> groceries = new ArrayList<>(Arrays.asList("Milk", "Eggs", "Bread"));
Iterator<String> iterator = groceries.iterator();
while (iterator.hasNext()) {
String item = iterator.next();
if (item.equals("Eggs")) {
iterator.remove();
}
}
for (String item : groceries) {
System.out.println(item);
}
# Output:
# Milk
# Bread
In this revised example, we use an Iterator to iterate over the groceries
list. When we encounter the item “Eggs”, we use the remove()
method of the Iterator to safely remove it from the list. This prevents the ConcurrentModificationException
from occurring.
Remember, understanding potential problems and how to troubleshoot them is an essential part of mastering the for-each loop in Java.
Fundamentals: Loops and Iteration in Java
Before we delve deeper into the for-each loop, let’s take a step back and understand the concept of loops and iteration in Java.
What are Loops in Java?
Loops are a fundamental concept in programming. They allow you to execute a block of code repeatedly until a certain condition is met. In Java, there are three main types of loops: the for
loop, the while
loop, and the do-while
loop.
Here’s an example of a basic for
loop that prints the numbers 1 to 5:
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the loop starts with i
equal to 1. It repeats as long as i
is less than or equal to 5, and for each iteration, it increments i
by 1.
What is Iteration?
Iteration is the process of repeating a sequence of operations until a specific condition is met. In the context of loops, each repetition of the loop’s code block is called an iteration.
Understanding the For-Each Loop
The for-each loop, also known as the enhanced for loop, is a simpler, more readable way to iterate over arrays and collections in Java. Unlike traditional for loops, which use counters and indices, the for-each loop automatically iterates over each element in the collection, making your code more readable and less prone to errors.
The for-each loop is essentially a simpler way to write a specific type of for loop, known as an iterator for loop. It’s specifically designed for iterating over collections and arrays, which is a very common task in Java programming.
By understanding these fundamentals, you’ll have a better grasp of how the for-each loop works in Java and why it’s such a powerful tool for handling collections.
The For-Each Loop in Real-World Applications
The for-each loop is not just a theoretical construct, but a practical tool that finds extensive use in real-world applications. It’s particularly relevant when handling data in large software projects.
In large-scale applications, data handling is a common task. You often need to iterate over large collections of data, such as user lists, product catalogs, or data records. The for-each loop makes this process simpler and more efficient, allowing you to write cleaner and more readable code.
Exploring Related Concepts
Once you’ve mastered the for-each loop, you might want to explore related concepts in Java, such as streams and lambda expressions.
- Streams: Introduced in Java 8, streams provide a powerful and flexible way to process collections. They allow you to perform complex data processing tasks, such as filtering, mapping, or aggregating data, in a declarative way.
Lambda Expressions: Also introduced in Java 8, lambda expressions are a way to write anonymous functions that you can pass to methods. They are often used in conjunction with streams to perform operations on collections.
These concepts are beyond the scope of this guide, but they are worth exploring if you want to deepen your understanding of Java and improve your ability to work with collections.
Further Resources for Mastering Java Iteration
To continue your learning journey, here are some additional resources that provide more in-depth information about the for-each loop and related concepts in Java:
- Advanced Techniques for Using Loops in Java – Explore loop-based iteration techniques for parsing and processing data in Java.
Java While Loop – Understand how to use while loops to repeatedly execute code blocks.
Using Do-While Loop in Java – Master the do-while loop for scenarios requiring initial execution before condition evaluation.
Oracle Java Documentation covers all aspects of Java, including the for-each loop.
Baeldung’s Guide to Java Loops: provides a detailed overview of all types of loops in Java, including the for-each loop.
For-Each Loop in Java by GeeksforGeeks provides an in-depth explanation of the ‘for-each’ loop in Java.
Remember, mastering a programming language is a journey. Keep learning, keep practicing, and don’t be afraid to dive deep!
Wrapping Up: Mastering the For-Each Loop in Java
In this comprehensive guide, we’ve delved into the intricacies of the for-each loop in Java, a powerful tool for iterating over collections and arrays.
We started with the basics, explaining how to use the for-each loop in simple scenarios. We then explored more complex uses, such as iterating over multidimensional arrays and custom objects. We also discussed alternative approaches to iteration, including the traditional for loop and the iterator method.
Along the way, we addressed common issues that you might encounter when using the for-each loop, such as the ConcurrentModificationException, and provided solutions to help you navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Readability | Can Modify Collection During Iteration |
---|---|---|---|
For-Each Loop | Low | High | No |
Traditional For Loop | High | Moderate | Yes |
Iterator Method | High | Moderate | Yes |
Whether you’re new to Java or an experienced developer looking to deepen your understanding of the language, we hope this guide has given you a thorough understanding of the for-each loop and its alternatives.
The ability to iterate over collections is fundamental to many programming tasks, and the for-each loop is a powerful tool for this purpose. Now that you’ve mastered it, you’re well-equipped to handle a wide range of programming challenges. Happy coding!