JVM Java Virtual Machine | Executing Code on Any System
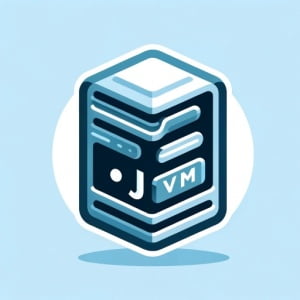
Have you ever pondered over what gives Java its unique characteristic of being platform-independent? You’re not alone. Many developers find themselves intrigued by this feature. The secret sauce behind this is the Java Virtual Machine (JVM). Think of JVM as a universal translator that enables Java programs to run on any device, irrespective of the operating system.
This guide will delve into the world of JVM, its architecture, and its pivotal role in Java programming. We’ll explore the core functionality of JVM, discuss its advanced features, and even tackle common issues and their solutions.
So, let’s dive in and start unraveling the mysteries of JVM!
TL;DR: What is JVM in Java?
JVM
, orJava Virtual Machine
, is anabstract machine that enables your computer to run a Java program
. When you compile your Java code, it’s turned into bytecode, which JVM interprets and runs it on your machine. For example,System.out.println("Hello, World!");
can be written on one Operating system, and then cam be ran on a JVM regardless of any other operating system.
Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve written a simple Java program that prints ‘Hello, World!’. When this code is compiled, it’s turned into bytecode. The JVM then interprets this bytecode and runs it on your machine, printing ‘Hello, World!’ to the console.
This is just a basic example of how JVM works in Java, but there’s much more to learn about JVM’s architecture, components, and its role in Java programming. Continue reading for a more detailed understanding.
Table of Contents
Exploring JVM Architecture: The Basics
The Java Virtual Machine (JVM) has a unique architecture that allows Java programs to run uniformly across different platforms. Let’s break down the key components of JVM’s architecture:
Class Loader
The class loader is the component of JVM that loads class files. When a Java program is executed, the class loader reads the .class files and loads them into the memory.
Here’s an example of how a class loader works:
public class Main {
public static void main(String[] args) {
ClassLoader classLoader = Main.class.getClassLoader();
System.out.println(classLoader);
}
}
# Output:
# sun.misc.Launcher$AppClassLoader@73d16e93
In this example, we’re getting the class loader that loaded our Main
class and printing it out. The output is the reference to the AppClassLoader, which is the class loader for our application.
Runtime Data Areas
JVM has various runtime data areas used for different purposes during the execution of a program. These include the method area, heap area, stack area, PC registers, and native method stacks. Each of these areas plays a critical role in the execution of Java programs.
Execution Engine
The execution engine is the component of JVM that executes the bytecode which has been loaded into the memory by the class loader. The execution engine reads the bytecode line by line, interprets it, and then executes it.
Native Method Interface (JNI)
The JVM uses the Java Native Interface (JNI) to interact with the Native Method Libraries and provides the native libraries required for the execution of the program.
By understanding these core components of JVM, we can see how JVM enables platform independence in Java. It does this by acting as a ‘middle-man’ between the Java code and the underlying operating system. This means that Java code can be written once and run anywhere – a fundamental philosophy of Java.
Diving Deeper: JVM Components and Their Roles
Now that we’ve covered the basics of JVM and its architecture, let’s delve deeper into the advanced components and their roles.
Understanding Bytecode
Bytecode is the intermediate representation of Java code, which the JVM interprets to execute the program. It’s the product of compiling your Java code, and it’s what makes Java platform-independent.
Here’s an example of how bytecode works:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
When this code is compiled, it’s turned into bytecode. This bytecode can then be interpreted by the JVM on any platform, producing the same output.
Just-In-Time (JIT) Compilation
JIT compilation is an optimization technique used by JVM to improve the performance of Java applications. It involves compiling bytecode into native machine code at runtime, which helps to speed up the program’s execution.
Garbage Collection
Garbage collection is a critical aspect of JVM’s memory management. It automatically reclaims memory that is no longer in use, which helps to prevent memory leaks and improve the performance of Java applications.
Memory Management
JVM plays a crucial role in managing memory in Java applications. It allocates memory for objects and variables, and it also deallocates memory when it’s no longer needed (through garbage collection).
By understanding these advanced components of JVM, we can see how JVM not only enables platform independence in Java but also helps to improve the performance of Java applications.
Exploring Alternatives to JVM
While JVM is the standard runtime environment for executing Java programs, it’s not the only option. There are alternative Java virtual machines and even different programming languages that you can use.
Other Java Virtual Machines
JRockit
JRockit is a proprietary Java SE implementation developed by BEA Systems (later acquired by Oracle). It’s designed to ensure high performance for enterprise applications and is particularly well-suited for long-running applications.
IBM’s J9
IBM’s J9 is another proprietary Java virtual machine. It’s known for its small memory footprint, making it a good choice for systems with limited resources.
Here’s how you might specify a different Java virtual machine when running a Java program:
java -version:JRockit Main
java -version:J9 Main
# Output:
# (The output will depend on the specific JVM and your program.)
In these examples, we’re using the -version
option to specify which JVM to use when running our Main
class. The output will depend on the specific JVM and your program.
Different Programming Languages
Java is not the only programming language that can run on a virtual machine. Languages like Python, Ruby, and JavaScript also have their own virtual machines (like PyPy, YARV, and V8, respectively). These languages and their virtual machines offer different features and advantages compared to Java and JVM.
While JVM is a powerful tool for executing Java programs, it’s worth exploring these alternative approaches to see if they might be a better fit for your specific needs.
Troubleshooting Common JVM Issues
Even with its robust architecture, JVM sometimes encounters issues that may hinder the smooth execution of your Java programs. Let’s discuss some common JVM-related problems and their solutions.
Out of Memory Errors
One of the most common issues you might encounter when working with JVM is an OutOfMemoryError. This error occurs when the JVM cannot allocate an object because it is out of memory, and no more memory could be made available by the garbage collector.
public class Main {
public static void main(String[] args) {
int[] memoryFillIntVar = new int[Integer.MAX_VALUE];
}
}
# Output:
# Exception in thread "main" java.lang.OutOfMemoryError: Requested array size exceeds VM limit
In this example, we’re trying to allocate a large array that exceeds the maximum size that the JVM can handle, leading to an OutOfMemoryError.
Performance Tuning
Performance tuning in JVM involves optimizing the performance of your Java application by modifying the default JVM settings. JVM performance tuning is a complex task that requires a deep understanding of the Java platform and its behavior.
Garbage Collection Problems
Garbage collection is a critical aspect of JVM’s memory management. However, if not managed properly, it can cause problems like memory leaks or excessive CPU usage.
public class Main {
public static void main(String[] args) {
for (int i = 0; i < 10000000; i++) {
Integer[] array = new Integer[100000];
}
}
}
# Output:
# (The program runs indefinitely, consuming more and more memory.)
In this example, we’re creating a new array in each iteration of the loop, which consumes more and more memory. This can lead to excessive garbage collection, slowing down the program and consuming a lot of CPU resources.
By understanding these common issues and their solutions, you can ensure that your Java applications run smoothly on the JVM.
JVM and the Principles of Virtual Machines
Before diving deeper into the workings of JVM, it’s crucial to understand the fundamental principles of virtual machines and how JVM embodies these principles.
The Concept of Virtual Machines
A virtual machine (VM) is a software emulation of a computer system. It provides the functionality of a physical computer and can run programs just like a real computer. VMs operate based on the architecture of a computer, with its own CPU, memory, and devices.
# An example of creating a virtual machine using VirtualBox
VBoxManage createvm --name "My VM" --ostype "Ubuntu_64" --register
# Output:
# Virtual machine 'My VM' is created and registered.
# UUID: aaaaaaaa-bbbb-cccc-dddd-eeeeeeeeeeee
In this example, we’re using VirtualBox, a popular software for creating and managing virtual machines, to create a new virtual machine named ‘My VM’. The output confirms that the virtual machine is created and registered.
Java’s ‘Write Once, Run Anywhere’ Philosophy
Java’s philosophy of ‘Write Once, Run Anywhere’ (WORA) is made possible by JVM. With JVM, you can write your Java code once, and it can run on any device that has a JVM, regardless of the underlying hardware and operating system.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve written a simple Java program that prints ‘Hello, World!’. This program can run on any device with a JVM, whether it’s a Windows PC, a Mac, or a Linux server.
By understanding these principles, we can better appreciate how JVM operates and the benefits it brings to Java programming.
The Impact of JVM on Larger Projects and the Java Ecosystem
The JVM’s role extends beyond just running individual Java applications. It plays a pivotal role in larger projects and has a significant impact on the broader Java ecosystem.
JVM in Large-Scale Projects
In large-scale projects, JVM’s platform independence, robust garbage collection, and performance optimization features come to the forefront. It allows developers to focus on the business logic of the application, rather than worrying about platform-specific nuances or memory management.
JVM’s Influence on the Java Ecosystem
JVM’s influence on the Java ecosystem is profound. It has led to the creation of numerous JVM languages like Groovy, Scala, and Kotlin, which offer different features and syntax but can still run on the JVM. This has greatly expanded the capabilities of the Java platform and its community.
// Kotlin code that runs on JVM
fun main(args: Array<String>) {
println("Hello, JVM!")
}
# Output:
# Hello, JVM!
In this example, we have a simple Kotlin program that prints ‘Hello, JVM!’. Despite being written in Kotlin, this program runs on the JVM, just like a Java program would.
Further Resources for Mastering Java Virtual Machine (JVM)
To continue your journey in mastering JVM, consider exploring the following resources:
- IOFlood’s Java Tutorial Guide – Understand multithreading concepts and synchronization in Java.
JDoodle Overview – Learn about JDoodle, an online platform offering a Java compiler and IDE.
Understanding Threading in Java – Explore examples and best practices for working with threads in Java.
Oracle’s Java Tutorials cover a wide range of topics, including explanations of JVM.
Jenkov’s Java JVM Tutorials offers in-depth tutorials on JVM and Java concurrency.
Baeldung’s Guide to JVM Languages provides an overview of different JVM languages and their features.
By understanding JVM’s role in larger projects and its impact on the Java ecosystem, you can better leverage its capabilities in your Java development journey.
Wrapping Up: Java Virtual Machine (JVM)
In this comprehensive guide, we’ve navigated the intricate world of the Java Virtual Machine (JVM), a cornerstone of Java programming. We’ve unraveled its architecture, dissected its components, and understood its role in making Java a versatile and platform-independent language.
We embarked on our journey with the basics, understanding JVM’s architecture, including the class loader, runtime data areas, execution engine, and native method interface. We then delved deeper, exploring advanced aspects like bytecode, Just-In-Time (JIT) compilation, garbage collection, and memory management.
Along the way, we tackled common challenges you might encounter when working with JVM, such as out of memory errors and performance tuning issues. We’ve also provided insights into alternatives to JVM, including other Java virtual machines like JRockit and IBM’s J9, broadening your understanding of the Java ecosystem.
Here’s a quick comparison of different Java virtual machines:
JVM | Pros | Cons |
---|---|---|
Standard JVM | Robust, widely used | May require troubleshooting for some programs |
JRockit | Optimized for long-running applications | Proprietary, not suitable for all applications |
IBM’s J9 | Small memory footprint | Proprietary, may not be suitable for all applications |
Whether you’re just starting out with JVM or you’re looking to enhance your understanding, we hope this guide has provided you with a comprehensive understanding of JVM and its capabilities.
With its platform independence and performance optimization features, JVM is a powerful tool for Java programming. Now, you’re well equipped to leverage its benefits. Happy coding!