Java Threads: Understanding Threading in Java
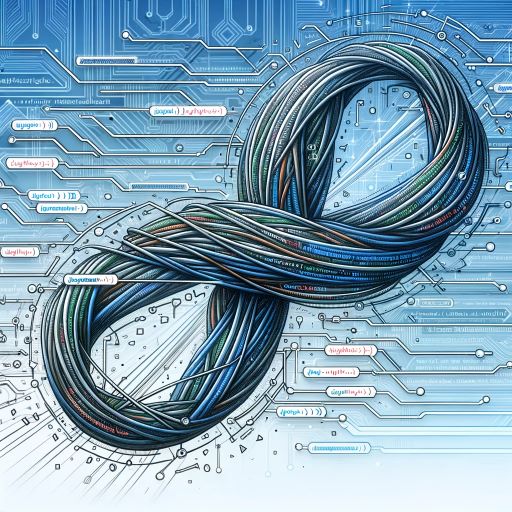
Are you finding it challenging to understand Java threads? You’re not alone. Many developers find themselves puzzled when it comes to handling multithreading in Java, but we’re here to help.
Think of a Java thread as a worker in a factory – performing tasks concurrently to enhance efficiency. It’s a lightweight subprocess that allows your Java applications to do multiple things at once, improving their performance and responsiveness.
In this guide, we’ll walk you through the process of working with Java threads, from their creation, manipulation, and usage. We’ll cover everything from the basics of multithreading to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: What is a Java Thread and How Do I Use It?
A Java thread is a lightweight subprocess that allows concurrent execution of tasks. We can run threads using the
Thread
class:class MyThread extends Thread
It’s a fundamental part of Java’s multithreading capability, enabling you to write code that performs several operations simultaneously. Here’s a simple example of creating and starting a thread in Java:
class MyThread extends Thread {
public void run(){
System.out.println('Thread is running.');
}
}
public class ThreadExample{
public static void main(String args[]){
MyThread t1=new MyThread();
t1.start();
}
}
# Output:
# 'Thread is running.'
In this example, we’ve created a new class MyThread
that extends the Thread
class. The run()
method is overridden to define the task that this thread will execute when it’s started. In the ThreadExample
class, we create an instance of MyThread
and start it using the start()
method, which triggers the run()
method.
This is a basic way to create and start a Java thread, but there’s much more to learn about managing threads, synchronizing them, and using them effectively in your Java applications. Continue reading for a more detailed guide on Java threads.
Table of Contents
- Creating, Starting, and Managing Java Threads: A Beginner’s Guide
- Understanding Thread Synchronization
- Inter-Thread Communication
- Exploring Thread Pooling
- Exploring java.util.concurrent: Advanced Thread Management
- Navigating Common Pitfalls: Deadlock, Thread Starvation, and Race Conditions
- Delving into Multithreading
- Multithreading in Action: Real-World Applications
- Exploring Related Topics
- Wrapping Up: Mastering Java Threads for Concurrent Programming
Creating, Starting, and Managing Java Threads: A Beginner’s Guide
Java threads are created by either extending the Thread
class or implementing the Runnable
interface. Let’s start with the most basic way to create a thread – by extending the Thread
class.
class NumberThread extends Thread {
public void run(){
for(int i=1; i<=5; i++){
System.out.println(i);
try{
Thread.sleep(1000);
} catch(InterruptedException e){
System.out.println("Thread interrupted: " + e);
}
}
}
}
public class ThreadExample {
public static void main(String[] args){
NumberThread t1 = new NumberThread();
t1.start();
}
}
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, NumberThread
extends Thread
to create a custom thread. The run()
method is overridden to define the task of the thread, which in this case, is to print numbers from 1 to 5 with a pause of one second between each print. An InterruptedException
is caught and handled whenever the thread is interrupted during the sleep. The NumberThread
is then instantiated and started in the main()
method of ThreadExample
.
Now, let’s look at another way to create a thread – by implementing the Runnable
interface.
class MyRunnable implements Runnable {
public void run(){
System.out.println('Runnable is running.');
}
}
public class RunnableExample{
public static void main(String args[]){
Thread t1=new Thread(new MyRunnable());
t1.start();
}
}
# Output:
# 'Runnable is running.'
In this example, we’ve created a new class MyRunnable
that implements the Runnable
interface. The run()
method is overridden to define the task that this thread will execute when it’s started. In the RunnableExample
class, we create an instance of Thread
and pass a new MyRunnable
object to the constructor. We then start it using the start()
method, which triggers the run()
method.
Managing threads involves starting them with the start()
method and controlling their execution with methods like sleep()
, join()
, and others. We’ll dive deeper into these methods and more advanced thread management techniques in the next sections.
Understanding Thread Synchronization
When you’re working with multiple threads, it’s crucial to ensure that they don’t interfere with each other while accessing shared resources. This is achieved through thread synchronization.
Java provides several mechanisms for thread synchronization, including synchronized
blocks/methods and volatile
variables. Let’s look at an example using a synchronized
method.
class Counter{
int count;
public synchronized void increment(){
count++;
}
}
public class SyncExample{
public static void main(String args[]) throws InterruptedException {
Counter c = new Counter();
Thread t1 = new Thread(new Runnable(){
public void run(){
for(int i=0; i<1000; i++){
c.increment();
}
}
});
Thread t2 = new Thread(new Runnable(){
public void run(){
for(int i=0; i<1000; i++){
c.increment();
}
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println('Count is ' + c.count);
}
}
# Output:
# 'Count is 2000'
In this example, we have a Counter
class with a count
variable and a synchronized
method increment()
. We create two threads that increment the count
1000 times each. Because the increment()
method is synchronized, only one thread can access it at a time, ensuring that the count
is correctly incremented to 2000.
Inter-Thread Communication
Java threads can communicate with each other using methods like wait()
, notify()
, and notifyAll()
. These methods are used in situations where a thread needs to wait for one or more threads before it can proceed.
Exploring Thread Pooling
Thread pooling is a technique where a pool of worker threads is created to perform a queue of tasks. This is more efficient than creating a new thread for each task, especially for applications that perform a large number of short-lived tasks.
Java provides built-in support for thread pools through the ExecutorService
and ThreadPoolExecutor
classes. Here’s an example of creating a fixed thread pool with ExecutorService
.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
Runnable worker = new MyRunnable('' + i);
executor.execute(worker);
}
executor.shutdown();
while (!executor.isTerminated()) {
}
System.out.println('Finished all threads');
}
}
class MyRunnable implements Runnable {
private String command;
public MyRunnable(String s){
this.command=s;
}
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+' Start. Command = '+command);
processCommand();
System.out.println(Thread.currentThread().getName()+' End.');
}
private void processCommand() {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# 'pool-1-thread-1 Start. Command = 0'
# 'pool-1-thread-2 Start. Command = 1'
# 'pool-1-thread-3 Start. Command = 2'
# 'pool-1-thread-4 Start. Command = 3'
# 'pool-1-thread-5 Start. Command = 4'
# 'pool-1-thread-1 End.'
# 'pool-1-thread-1 Start. Command = 5'
# ...
# 'Finished all threads'
In this example, we create a thread pool of 5 worker threads. We then submit 10 tasks to this thread pool. As each task is completed, the worker thread is returned to the pool and can be reused for the next task. This reduces the overhead of thread creation for each task, improving the performance of your Java applications.
Exploring java.util.concurrent: Advanced Thread Management
While the basic thread management techniques are powerful, Java provides a more advanced toolkit for thread management in the java.util.concurrent
package. This package includes several classes that offer high-level concurrency features, simplifying the creation and management of multiple threads.
Executor Framework
The Executor framework is a more modern alternative to managing threads manually. It provides a pool of threads, handling the creation, scheduling, and management of threads for you.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ExecutorsExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
Runnable worker = new MyRunnable('' + i);
executor.execute(worker);
}
executor.shutdown();
while (!executor.isTerminated()) {
}
System.out.println('Finished all threads');
}
}
class MyRunnable implements Runnable {
private String command;
public MyRunnable(String s){
this.command=s;
}
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+' Start. Command = '+command);
processCommand();
System.out.println(Thread.currentThread().getName()+' End.');
}
private void processCommand() {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
# Output:
# 'pool-1-thread-1 Start. Command = 0'
# 'pool-1-thread-2 Start. Command = 1'
# 'pool-1-thread-3 Start. Command = 2'
# 'pool-1-thread-4 Start. Command = 3'
# 'pool-1-thread-5 Start. Command = 4'
# 'pool-1-thread-1 End.'
# 'pool-1-thread-1 Start. Command = 5'
# ...
# 'Finished all threads'
In this example, we create a thread pool of 5 worker threads using the Executors.newFixedThreadPool()
method. We then submit 10 tasks to this thread pool. As each task is completed, the worker thread is returned to the pool and can be reused for the next task. This reduces the overhead of thread creation for each task, improving the performance of your Java applications.
Future and Callable
The Future
and Callable
interfaces provide a way to handle tasks that return a result. Unlike Runnable
, which does not return a result, Callable
can return a value and can throw an exception.
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
public class CallableExample {
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5);
Future<Integer> future = executor.submit(new Callable<Integer>() {
@Override
public Integer call() throws Exception {
return 1 + 1;
}
});
try {
System.out.println('Result: ' + future.get());
} catch (InterruptedException | ExecutionException e) {
e.printStackTrace();
}
executor.shutdown();
}
}
# Output:
# 'Result: 2'
In this example, we use an ExecutorService
to run a Callable
task. The submit()
method returns a Future
object that we can use to retrieve the result of the Callable
task once it’s completed. We use the get()
method of the Future
object to retrieve the result, which blocks until the task is completed.
These are just a few examples of the advanced features provided by the java.util.concurrent
package. The package also includes classes for thread-safe collections, locks, atomic variables, and more. By using these advanced features, you can write more efficient, scalable, and reliable multithreaded Java applications.
When working with Java threads, you may encounter several common issues. Understanding these pitfalls and how to avoid them is crucial in developing efficient and bug-free multithreaded applications.
Deadlock
Deadlock is a situation where two or more threads are blocked forever, each waiting for the other to release a lock. It usually occurs in the context of multiple threads holding locks and waiting for locks held by other threads.
public class DeadlockExample {
public static void main(String[] args) {
final String resource1 = 'resource1';
final String resource2 = 'resource2';
Thread t1 = new Thread(() -> {
synchronized (resource1) {
System.out.println('Thread 1: locked resource 1');
try { Thread.sleep(100);} catch (Exception e) {}
synchronized (resource2) {
System.out.println('Thread 1: locked resource 2');
}
}
});
Thread t2 = new Thread(() -> {
synchronized (resource2) {
System.out.println('Thread 2: locked resource 2');
try { Thread.sleep(100);} catch (Exception e) {}
synchronized (resource1) {
System.out.println('Thread 2: locked resource 1');
}
}
});
t1.start();
t2.start();
}
}
# Output:
# 'Thread 1: locked resource 1'
# 'Thread 2: locked resource 2'
In this example, Thread 1
locks resource1
and Thread 2
locks resource2
. They then try to lock the other resource, resulting in a deadlock as each thread waits for the other to release its lock.
Thread Starvation
Thread starvation occurs when a thread is continually denied access to resources and is unable to make progress. This happens when low-priority threads are not given CPU time because high-priority threads are consuming all CPU time.
Race Conditions
A race condition occurs when the behavior of a program depends on the relative timing of threads. They are often caused by threads sharing mutable data.
public class RaceConditionExample {
public static void main(String[] args) {
Counter counter = new Counter();
Thread t1 = new Thread(counter, 'Thread 1');
Thread t2 = new Thread(counter, 'Thread 2');
t1.start();
t2.start();
}
}
class Counter implements Runnable {
private int count = 0;
@Override
public void run() {
for (int i = 0; i < 5; i++) {
incrementCount();
System.out.println(Thread.currentThread().getName() + ' - ' + getCount());
}
}
public void incrementCount() {
count++;
}
public int getCount() {
return count;
}
}
# Output:
# 'Thread 1 - 1'
# 'Thread 2 - 2'
# 'Thread 1 - 3'
# 'Thread 2 - 4'
# 'Thread 1 - 5'
# 'Thread 2 - 6'
# 'Thread 1 - 7'
# 'Thread 2 - 8'
# 'Thread 1 - 9'
# 'Thread 2 - 10'
In this example, Thread 1
and Thread 2
are incrementing a shared count. Because they’re running concurrently, the final value of the count can vary depending on the relative timing of the threads. This is a race condition.
Best Practices to Avoid These Issues
- Avoid Nested Locks: Don’t lock one resource while holding a lock on another. If you must do so, always acquire the locks in the same order.
- Use Thread Pools: Java’s built-in thread pools can automatically manage the number of threads, helping to prevent resource exhaustion that can lead to thread starvation.
- Synchronize Methods and Blocks: Use Java’s built-in
synchronized
keyword to ensure that only one thread can access a method or block at a time. - Use Atomic Variables and Concurrent Collections: The
java.util.concurrent
package provides several thread-safe and atomic classes that can help prevent race conditions.
By following these best practices, you can avoid common threading issues and write safer, more efficient multithreaded Java applications.
Delving into Multithreading
Multithreading is a core concept in Java, and understanding it is crucial for writing efficient and responsive applications. But what exactly is multithreading, and why is it so important?
The Power of Multithreading
Multithreading is the ability of a CPU (or a single core in a multi-core processor) to execute multiple processes or threads concurrently, supported by the operating system. This concurrent execution of threads leads to optimal CPU utilization as idle CPU time is minimized.
class MultiThreadDemo implements Runnable {
private String threadName;
MultiThreadDemo(String threadName) {
this.threadName = threadName;
}
@Override
public void run() {
for(int i=0; i<5; i++) {
System.out.println(threadName + ' output: ' + i);
}
}
}
public class Main {
public static void main(String[] args) {
MultiThreadDemo demo1 = new MultiThreadDemo('Thread 1');
MultiThreadDemo demo2 = new MultiThreadDemo('Thread 2');
new Thread(demo1).start();
new Thread(demo2).start();
}
}
# Output:
# 'Thread 1 output: 0'
# 'Thread 2 output: 0'
# 'Thread 1 output: 1'
# 'Thread 2 output: 1'
# 'Thread 1 output: 2'
# 'Thread 2 output: 2'
# 'Thread 1 output: 3'
# 'Thread 2 output: 3'
# 'Thread 1 output: 4'
# 'Thread 2 output: 4'
In this example, we create two threads, ‘Thread 1’ and ‘Thread 2’. Each thread prints out the numbers 0-4. When we run the program, we see that the output from the two threads is interleaved. This is because the threads are running concurrently, with the CPU switching back and forth between them.
How Multithreading Works in Java
In Java, multithreading is primarily supported through the Thread
class and the Runnable
interface. A Java program can create multiple threads that run concurrently, with each thread being a separate path of execution within the program.
Each thread in Java has a priority, and the JVM schedules thread execution based on their priorities. The JVM continues to execute threads until either of the following occurs: the exit
method is invoked, all daemon threads of the process have died, or all threads that are not daemon threads have died.
Understanding multithreading is crucial for any Java developer, as it forms the basis for many advanced features and can significantly improve the performance of your applications.
Multithreading in Action: Real-World Applications
Multithreading is not just a theoretical concept; it’s a practical tool that can significantly enhance the performance and responsiveness of your Java applications. But where is multithreading used in the real world, and how does it benefit these applications?
Multithreading in Web Servers
Web servers are a prime example of where multithreading is used. When a web server receives a request, it often spawns a new thread to handle that request. This allows the server to handle multiple requests simultaneously, improving its throughput and responsiveness.
Multithreading in GUI Applications
In GUI applications, multithreading is used to ensure that the user interface remains responsive. A common pattern is to have a main thread handling the user interface and separate worker threads performing any time-consuming operations. This way, even if a worker thread is blocked or busy, the user interface remains responsive.
Multithreading in Data Processing
Data processing tasks, such as those found in big data and machine learning applications, often involve processing large amounts of data. Multithreading allows these tasks to be broken down and processed in parallel, significantly reducing the overall processing time.
Exploring Related Topics
While this guide covers the basics of multithreading in Java, there’s much more to learn. Related topics that you might find interesting include concurrent collections, atomic variables, and the Fork/Join framework. These topics delve into more advanced concurrency concepts and can help you write more efficient and robust multithreaded applications.
Further Resources for Mastering Java Multithreading
To dive into Java’s standard library and learn about essential classes and methods, Click Here for a Full Java Tutorial!
And for other resources, consider the following:
- Multithreading in Java Overview – explore multithreading concepts in Java and learn how to improve performance.
JVM Overview – Understand the Java Virtual Machine (JVM) and its role in executing Java bytecode on different platforms.
Java Concurrency in Practice covers all aspects of concurrency in Java.
Oracle’s Java Tutorials: Concurrency – Oracle’s official tutorials on Java concurrency.
A Guide to Java Executors – An in-depth guide to the Executor framework in Java.
Wrapping Up: Mastering Java Threads for Concurrent Programming
In this comprehensive guide, we’ve delved into the world of Java threads, a powerful tool for creating concurrent programs in Java. We’ve explored the fundamental concepts of multithreading, from the creation and management of threads to more advanced techniques like synchronization and thread pooling.
We embarked on our journey with the basics, learning how to create, start, and manage threads in Java. We then ventured into more advanced territory, discussing thread synchronization, inter-thread communication, and thread pooling. We also touched on alternative approaches to thread management using the java.util.concurrent
package, providing you with a more advanced toolkit for handling threads.
Along the way, we addressed common challenges you might face when working with threads, such as deadlock, thread starvation, and race conditions, offering solutions and best practices to help you avoid these pitfalls. Here’s a quick comparison of the techniques we’ve discussed:
Technique | Pros | Cons |
---|---|---|
Basic Thread Management | Simple to implement, direct control over threads | Manual management can be error-prone |
Advanced Thread Management (Synchronization, Inter-thread Communication) | Allows safe access to shared resources, better coordination between threads | Requires careful design to avoid issues like deadlock |
Executor Framework (java.util.concurrent ) | Simplifies thread management, provides high-level concurrency features | More complex, requires understanding of advanced concepts |
Whether you’re just starting out with Java threads or looking to deepen your understanding of concurrent programming, we hope this guide has equipped you with the knowledge and skills you need to effectively use threads in your Java applications.
The ability to create and manage threads is a fundamental skill in Java programming, opening the door to writing more efficient, responsive, and powerful applications. Now, you’re well equipped to harness the power of multithreading in your Java programs. Happy coding!