Multithreading in Java: A Need-to-Know Guide
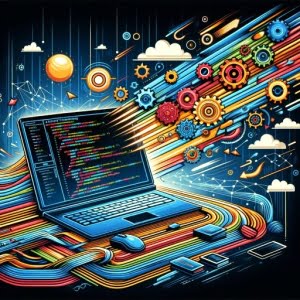
Are you finding it challenging to manage multiple threads in Java? You’re not alone. Many developers find multithreading in Java a bit daunting, but with the right guide, it can be as harmonious as a well-conducted orchestra.
Like a skilled conductor, Java has the ability to orchestrate multiple threads to work in harmony, providing a powerful tool to enhance your applications’ performance and responsiveness.
In this guide, we will walk you through the process of mastering multithreading in Java, from the basics to more advanced techniques. We’ll cover everything from creating and starting threads, to thread synchronization, inter-thread communication, and even alternative approaches to traditional threading.
So, let’s dive in and start mastering multithreading in Java!
TL;DR: How Do I Use Multithreading in Java?
Java provides the Thread class and the Runnable interface for creating and managing threads. You can create a new thread by extending the Thread class with:
class MyThread extends Thread
and overriding its run() method with:public void run()
, or by implementing the Runnable interface and passing it to a Thread instance.
Here’s a simple example:
class MyThread extends Thread {
public void run(){
System.out.println('Thread is running.');
}
}
MyThread t1=new MyThread();
t1.start();
# Output:
# 'Thread is running.'
In this example, we create a new class MyThread
that extends the Thread
class and overrides its run()
method. The run()
method contains the code that will be executed when the thread is started. We then create an instance of MyThread
and call its start()
method to begin the execution of the thread.
This is a basic way to use multithreading in Java, but there’s much more to learn about managing multiple threads, thread synchronization, and advanced threading techniques. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Creating and Starting Threads in Java
- Thread Synchronization in Java
- Inter-Thread Communication in Java
- Thread Pooling in Java
- Exploring the Executor and Fork/Join Frameworks
- Navigating Common Multithreading Issues in Java
- Understanding Multithreading in Java
- The Impact of Multithreading on Java Applications
- Wrapping Up: Mastering Multithreading in Java
Creating and Starting Threads in Java
In Java, there are two main ways to create a new thread: using the Thread
class and using the Runnable
interface. Let’s explore both methods and understand their pros and cons.
Using the Thread Class
The simplest way to create a new thread in Java is by extending the Thread
class. In this approach, you create a new class that extends Thread
and override its run()
method. The run()
method contains the code that will be executed in the new thread.
Here’s a basic example:
class MyThread extends Thread {
public void run(){
System.out.println('Thread is running.');
}
}
MyThread t1=new MyThread();
t1.start();
# Output:
# 'Thread is running.'
In this example, we create a new class MyThread
that extends the Thread
class and overrides its run()
method. The run()
method contains the code that will be executed when the thread is started. We then create an instance of MyThread
and call its start()
method to begin the execution of the thread.
The advantage of this approach is its simplicity. However, the main downside is that Java does not support multiple inheritance, which means your class cannot extend any other class if it already extends Thread
.
Using the Runnable Interface
An alternative way to create a new thread in Java is by implementing the Runnable
interface. In this approach, you create a new class that implements Runnable
and override its run()
method. Then, you pass an instance of this class to a new Thread
instance.
Here’s a basic example:
class MyRunnable implements Runnable {
public void run(){
System.out.println('Runnable is running.');
}
}
Thread t2=new Thread(new MyRunnable());
t2.start();
# Output:
# 'Runnable is running.'
In this example, we create a new class MyRunnable
that implements the Runnable
interface and overrides its run()
method. We then create a new Thread
instance and pass an instance of MyRunnable
to its constructor. Finally, we call the start()
method to begin the execution of the thread.
The advantage of this approach is that it avoids the limitations of multiple inheritance because your class can still extend another class while implementing Runnable
. However, it can be a bit more complex to set up than the Thread
class approach.
Thread Synchronization in Java
Thread synchronization is defined as a mechanism which ensures that two or more concurrent threads do not simultaneously execute some particular program segment known as a critical section.
Concurrent accesses to shared resources can lead to race conditions. In Java, we can use synchronized
keyword to control the access of multiple threads to the shared resource.
Here’s a basic example:
class Counter{
int count;
public synchronized void increment(){
count++;
}
}
Counter c = new Counter();
Thread t1=new Thread(new Runnable(){
public void run(){
for(int i=1; i<=1000; i++){
c.increment();
}
}
});
Thread t2=new Thread(new Runnable(){
public void run(){
for(int i=1; i<=1000; i++){
c.increment();
}
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println('Count is ' + c.count);
# Output:
# 'Count is 2000'
In this example, we have a Counter
class with a count
variable and a increment()
method which increases the count
. We have two threads t1
and t2
which call the increment()
method 1000 times each. Without synchronization, we could have a race condition where the two threads interleave in a way that leads to an incorrect result. But with the synchronized
keyword, we ensure that only one thread can access the increment()
method at a time, leading to the correct result of 2000.
Inter-Thread Communication in Java
Inter-thread communication is an essential feature of Java which allows threads to communicate with each other. This is mainly used in cases where a thread needs to wait for other threads to complete their tasks.
For example, the wait()
, notify()
, and notifyAll()
methods can be used in Java for inter-thread communication.
Thread Pooling in Java
Thread pooling is a technique where a pool of threads is created and used to execute tasks. This can be more efficient than creating a new thread for each task, especially for many short-lived tasks.
Java provides the ExecutorService
interface for creating and managing thread pools. Here’s a basic example:
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
Runnable worker = new WorkerThread('' + i);
executor.execute(worker);
}
executor.shutdown();
while (!executor.isTerminated()) { }
System.out.println('Finished all threads');
# Output:
# 'Finished all threads'
In this example, we create a thread pool with 5 threads using Executors.newFixedThreadPool(5)
. We then submit 10 tasks to the executor. Each task is a Runnable
object. The executor will execute these tasks with 5 threads. Once all tasks are submitted, we call executor.shutdown()
to stop accepting new tasks and wait for all tasks to finish.
Exploring the Executor and Fork/Join Frameworks
While the Thread class and Runnable interface are the basic tools for multithreading in Java, there are more advanced alternatives that can offer greater control and efficiency. Two such alternatives are the Executor framework and the Fork/Join framework.
The Executor Framework
The Executor framework is a higher-level replacement for working with threads directly. Executors are capable of managing a pool of threads, so we do not have to manually create new threads and run tasks in an asynchronous way.
Here’s a basic example of using an Executor:
ExecutorService executor = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
Runnable worker = new WorkerThread('' + i);
executor.execute(worker);
}
executor.shutdown();
while (!executor.isTerminated()) { }
System.out.println('Finished all threads');
# Output:
# 'Finished all threads'
In this example, we create a thread pool with 5 threads using Executors.newFixedThreadPool(5)
. We then submit 10 tasks to the executor. Each task is a Runnable
object. The executor will execute these tasks with 5 threads. Once all tasks are submitted, we call executor.shutdown()
to stop accepting new tasks and wait for all tasks to finish.
The Fork/Join Framework
The Fork/Join framework is an implementation of the ExecutorService interface that helps you take advantage of multiple processors. It is designed for work that can be broken into smaller pieces recursively. The goal is to use all available processing power to enhance the performance of your application.
Here’s a basic example of using a ForkJoinPool:
ForkJoinPool forkJoinPool = new ForkJoinPool(4);
Long computedResult = forkJoinPool.invoke(new RecursiveTask<Long>() {
@Override
protected Long compute() {
// compute task
return computedResult;
}
});
# Output:
# Computed result: {computedResult}
In this example, we create a ForkJoinPool
with four threads and submit a RecursiveTask
to it. The compute()
method contains the code that can be broken down into smaller tasks and executed in parallel.
Both the Executor and Fork/Join frameworks provide powerful tools for managing multithreading in Java. Choosing between them depends on the specific needs of your project.
While multithreading can significantly enhance your Java applications’ performance, it also introduces a range of potential issues. Let’s discuss some of the most common problems you might encounter when working with multithreading in Java, including race conditions, deadlocks, and thread starvation, and provide solutions and workarounds for each.
Race Conditions
A race condition occurs when two or more threads can access shared data and they try to change it at the same time. As a result, the values of variables may be unpredictable and vary depending on the timings of context switches of the processes.
Here’s a simple example of a race condition:
class Counter {
int count;
public void increment() {
count++;
}
}
Counter c = new Counter();
Thread t1 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
c.increment();
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 1000; i++) {
c.increment();
}
});
t1.start();
t2.start();
t1.join();
t2.join();
System.out.println('Count is ' + c.count);
# Output:
# 'Count is ' + {random number}
In this example, we have two threads t1
and t2
that both increment the count
variable of a Counter
object 1000 times. Because the increment()
method is not synchronized, the two threads can access and modify count
at the same time, leading to a race condition and an unpredictable result.
The solution to this problem is to use the synchronized
keyword to ensure that only one thread can access the increment()
method at a time.
Deadlocks
A deadlock is a situation where two or more threads are blocked forever, waiting for each other. This usually occurs when multiple threads need the same locks but obtain them in different order.
A common solution to avoid deadlocks is to always acquire the locks in the same order.
Thread Starvation
Thread starvation occurs when a thread is unable to gain regular access to shared resources and is unable to make progress. This happens when shared resources are made unavailable for long periods by “greedy” threads. In Java, thread starvation can be caused by setting thread priority.
A common solution to avoid thread starvation is fair locking and thread scheduling.
Understanding Multithreading in Java
Multithreading is a core concept in Java, and understanding it is crucial for any serious Java developer. But what exactly is multithreading, and why is it so important?
What is Multithreading?
Multithreading is a feature that allows concurrent execution of two or more parts of a program for maximum utilization of CPU. Each part of such a program is called a thread, and each thread defines a separate path of execution.
Here’s a simple example of a multithreaded program in Java:
class MultiThreadDemo {
public static void main(String args[]) {
Thread t1 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
System.out.println('Thread 1: ' + i);
try { Thread.sleep(1000); } catch (Exception e) {}
}
});
Thread t2 = new Thread(() -> {
for (int i = 0; i < 5; i++) {
System.out.println('Thread 2: ' + i);
try { Thread.sleep(1000); } catch (Exception e) {}
}
});
t1.start();
t2.start();
}
}
# Output:
# (The output will interleave prints from Thread 1 and Thread 2)
In this example, we create two threads t1
and t2
that each print numbers from 0 to 4. Because t1
and t2
are separate threads, their outputs can interleave in any order.
Why Use Multithreading?
Multithreading can significantly improve the performance of a program by allowing multiple operations to run in parallel. It can also make a program more responsive by allowing it to continue running while long-running operations are performed in the background.
How Does Java Handle Multithreading?
Java provides built-in support for multithreading through the java.lang.Thread
class and the java.lang.Runnable
interface. These tools allow you to create and manage threads, synchronize their execution, and communicate between threads.
However, working with threads directly can be complex and error-prone. Java also provides higher-level tools for multithreading, such as the java.util.concurrent
package, which includes the Executor framework and the Fork/Join framework.
In the following sections, we will explore these tools and concepts in more detail, and show you how to use them to write efficient and reliable multithreaded programs in Java.
The Impact of Multithreading on Java Applications
Multithreading plays a critical role in building scalable and responsive Java applications. It allows tasks to be executed concurrently, leading to better use of system resources and improved application performance.
Exploring Concurrent Collections
Java provides a set of interfaces and classes in the java.util.concurrent
package that offer thread-safe collection classes. These classes, known as concurrent collections, include ConcurrentHashMap
, CopyOnWriteArrayList
, and BlockingQueue
. They are designed to scale well under high concurrency by using fine-grained locking or lock-free algorithms.
Understanding Atomic Variables
Java also provides classes in the java.util.concurrent.atomic
package that support lock-free, thread-safe programming on single variables. These classes, known as atomic variables, include AtomicInteger
, AtomicLong
, and AtomicReference
. They rely on the compare-and-swap (CAS) operation provided by the hardware to achieve their thread-safety without the need for locks.
Leveraging Locks
In addition to the implicit locking provided by the synchronized
keyword, Java provides explicit locking through the java.util.concurrent.locks
package. This package offers more flexible locking operations than synchronized
, including the ability to interrupt a thread waiting for a lock, to attempt to acquire a lock without waiting indefinitely, and to acquire and release locks in different scopes.
Further Resources for Mastering Java Multithreading
If you’re interested in diving deeper into Java multithreading, here are a few resources that you might find helpful:
- Java Tutorial: Understanding Basics – Discover how to work with dates, times, and calendars in Java applications.
Java Thread Basics – Learn about threads in Java and how they enable concurrent execution of tasks.
Exploring Java REPL Tools – Learn how Java REPL tools enable rapid prototyping and quick code testing.
Java Concurrency in Practice – A comprehensive guide to writing concurrent programs in Java.
Oracle’s Java Tutorials – Concurrency – Oracle’s official tutorials on concurrency in Java.
Guide to the Fork/Join Framework in Java – A detailed guide on using the Fork/Join framework in Java.
Wrapping Up: Mastering Multithreading in Java
In this comprehensive guide, we’ve explored the ins and outs of multithreading in Java. From the basics of creating and starting threads to advanced techniques like thread synchronization and inter-thread communication, we’ve covered a wide array of topics to help you understand and effectively use multithreading in your Java applications.
We began with the basics, learning how to create and start threads using the Thread class and the Runnable interface. We then delved into more advanced topics, such as thread synchronization, inter-thread communication, and thread pooling, providing code examples and detailed explanations for each concept.
We also explored alternative approaches to multithreading, including the Executor framework and the Fork/Join framework, and discussed common issues you might encounter when working with multithreading, such as race conditions, deadlocks, and thread starvation, providing solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Thread class | Simple to use | Limited by multiple inheritance |
Runnable interface | Avoids multiple inheritance limitations | Slightly more complex to set up |
Executor framework | Manages thread pools, more control | Higher complexity |
Fork/Join framework | Utilizes multiple processors, improves performance | Requires tasks that can be broken down |
Whether you’re just starting out with multithreading in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of multithreading and its capabilities in Java. With its balance of simplicity and control, multithreading is a powerful tool for enhancing the performance and responsiveness of your Java applications. Happy coding!