Java REPL: Guide to Interactive Command Line Coding
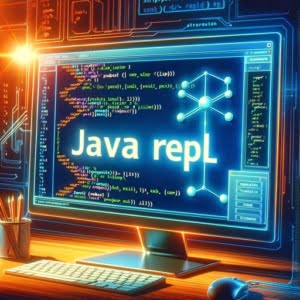
Are you finding it challenging to grasp Java REPL? You’re not alone. Many developers, especially those new to Java, find this tool a bit intimidating. But think of Java REPL as a personal tutor, always ready to help you learn Java interactively.
This guide will walk you through the ins and outs of Java REPL, from basic usage to advanced techniques. We’ll explore how to start using Java REPL, delve into its advanced features, and even discuss common issues and their solutions. So, let’s dive in and start mastering Java REPL!
TL;DR: What is Java REPL and How Do I Use It?
Java REPL, or Read-Eval-Print Loop, is a tool introduced in Java 9 that allows you to execute Java code interactively. It is used by prepending the
jshell
keyword before a command on the java command line, for examplejshell> System.out.println("Hello, World!");
. It’s like a playground for testing your Java code snippets.
Here’s a simple example:
jshell> System.out.println("Hello, World!");
# Output:
# Hello, World!
In this example, we’ve used the Java REPL (jshell) to execute a simple print statement. The System.out.println("Hello, World!");
command is entered directly into the jshell prompt, and the output is immediately displayed.
This is just a basic way to use Java REPL, but there’s much more to learn about this powerful tool. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with Java REPL
- Exploring Advanced Features of Java REPL
- Exploring Alternatives to Java REPL
- Navigating Common Issues with Java REPL
- Understanding REPL and Its Significance in Programming
- The Evolution of Java and the Introduction of REPL
- Java REPL in Real-World Programming
- Wrapping Up: Mastering Java REPL
Getting Started with Java REPL
Java REPL, also known as JShell, is a command-line tool that was introduced in Java 9. It provides an environment for you to test Java code snippets without needing to compile or run an entire program. This makes it a fantastic tool for beginners to learn Java interactively and get immediate feedback.
To start using Java REPL, you first need to open it. If you have Java 9 or later installed, simply type jshell
in your command line and hit enter. You will be greeted with a prompt that looks like this:
jshell>
This is where you can start typing in Java expressions. Let’s try a simple arithmetic operation:
jshell> 5 + 7
$1 ==> 12
In this example, we’ve asked Java REPL to add 5 and 7. The $1 ==> 12
output indicates that the result of our operation is 12. The $1
is a temporary variable that Java REPL automatically assigns to hold the result of our operation.
Advantages of Using Java REPL
One of the biggest advantages of using Java REPL is its interactivity. It provides immediate feedback, which is especially useful when learning a new concept or debugging a piece of code.
Another advantage is that it allows you to test small code snippets without having to write a full program. This can significantly speed up your learning process and increase your productivity.
Pitfalls to Avoid
While Java REPL is a powerful tool, it’s important to remember that it’s not a replacement for a full-fledged Java development environment. It’s best used for learning, experimenting with new concepts, and debugging small code snippets.
Also, keep in mind that Java REPL doesn’t save your work automatically. If you exit the tool, you’ll lose any code you’ve written. To save your work, you can use the /save
command followed by the filename, like this:
jshell> /save mywork.jsh
This will save your work into a file named mywork.jsh
.
Exploring Advanced Features of Java REPL
Java REPL isn’t just a tool for beginners. It also offers advanced features that can be beneficial to intermediate-level developers. Let’s delve into some of these features.
Testing Code Snippets
One of the most valuable aspects of Java REPL is its ability to test code snippets. This can be particularly useful when you’re trying to understand a new concept or troubleshoot a piece of code. For instance, let’s say you’re working with Java streams and want to test a filter operation:
jshell> var list = java.util.Arrays.asList(1, 2, 3, 4, 5)
list ==> [1, 2, 3, 4, 5]
jshell> list.stream().filter(n -> n%2 == 0).collect(java.util.stream.Collectors.toList())
$2 ==> [2, 4]
In this example, we’ve created a list of integers and used a stream to filter out the even numbers. The output $2 ==> [2, 4]
shows the result of our operation.
Debugging
Java REPL can also be a powerful tool for debugging. You can execute pieces of your code and inspect the results immediately. This can help you pinpoint the source of an issue more quickly than running an entire program.
Learning New Java Features
Java REPL is an excellent tool for familiarizing yourself with new features introduced in Java updates. For instance, you can use it to experiment with the new switch expressions introduced in Java 12:
jshell> var day = "MONDAY"
day ==> "MONDAY"
jshell> switch (day) {
...> case "MONDAY", "TUESDAY", "WEDNESDAY", "THURSDAY", "FRIDAY" -> "Weekday"
...> case "SATURDAY", "SUNDAY" -> "Weekend"
...> default -> "Invalid day"
...> }
$3 ==> "Weekday"
In this example, we’ve used a switch expression to determine whether a given day is a weekday or a weekend. The output $3 ==> "Weekday"
shows the result of our operation.
Exploring Alternatives to Java REPL
While Java REPL is a powerful tool, it’s not the only option for interactive Java development. There are several other tools and IDEs that offer similar features. Let’s take a look at some of these alternatives and how they compare to Java REPL.
Eclipse JShell Plugin
Eclipse, a popular Java IDE, offers a JShell plugin. This plugin integrates the functionality of Java REPL directly into the Eclipse environment. You can execute Java code snippets and see the results immediately, just like in Java REPL.
// In Eclipse JShell plugin
System.out.println("Hello, Eclipse!");
// Output:
// Hello, Eclipse!
The advantage of using the Eclipse JShell plugin is that you can enjoy the benefits of Java REPL while staying within your familiar development environment. However, the plugin may not offer all the features of the standalone Java REPL tool.
IntelliJ IDEA
IntelliJ IDEA, another popular Java IDE, also offers interactive Java development features. Its ‘Evaluate Expression’ feature allows you to execute Java code snippets on the fly.
// In IntelliJ IDEA
System.out.println("Hello, IntelliJ!");
// Output:
// Hello, IntelliJ!
The advantage of using IntelliJ IDEA is that it provides a more integrated development experience, with advanced features like code completion and debugging. However, it may be overkill if you’re just looking for a simple tool to experiment with Java code snippets.
Groovy Console
The Groovy Console is an interactive environment for running Groovy scripts, which are similar to Java code snippets. It provides immediate feedback, much like Java REPL.
// In Groovy Console
println("Hello, Groovy!")
// Output:
// Hello, Groovy!
The advantage of using the Groovy Console is that it supports Groovy, a dynamic language that is fully compatible with Java. However, it may not be suitable if you’re specifically looking to work with standard Java code.
In conclusion, while Java REPL is a great tool for interactive Java development, it’s not the only game in town. Depending on your needs and preferences, you might find one of these alternatives more suitable.
Like any tool, Java REPL comes with its own set of challenges. Let’s discuss some of the common issues you may encounter when using Java REPL and how to resolve them.
Issue 1: Unsaved Work
As mentioned earlier, Java REPL doesn’t automatically save your work. If you exit the tool, you’ll lose any code you’ve written. To avoid this, remember to save your work regularly using the /save
command.
jshell> /save mywork.jsh
This command will save your work into a file named mywork.jsh
. You can then reload this file the next time you use Java REPL with the /open
command:
jshell> /open mywork.jsh
Issue 2: Unexpected Results
Sometimes, you might get unexpected results when executing a code snippet in Java REPL. This could be due to a variety of reasons, such as a syntax error or a logical error in your code.
For instance, consider the following code snippet:
jshell> var list = java.util.Arrays.asList(1, 2, 3, 4, 5)
list ==> [1, 2, 3, 4, 5]
jshell> list.stream().filter(n -> n%2 == 0).collect(java.util.stream.Collectors.toList())
$2 ==> [2, 4]
If you expected the output to be [1, 3, 5]
(the odd numbers), you might be confused by the actual output [2, 4]
. The issue here is a logical error in the code: the filter condition n -> n%2 == 0
checks for even numbers, not odd numbers. To get the odd numbers, you should change the condition to n -> n%2 != 0
.
Issue 3: REPL Doesn’t Start
If Java REPL doesn’t start when you type jshell
in your command line, it could be because you don’t have Java 9 or later installed, or your Java installation is not correctly configured. To resolve this issue, check your Java version with the java -version
command and make sure your JAVA_HOME environment variable is set correctly.
In conclusion, while Java REPL is a powerful and convenient tool, it’s important to be aware of its quirks and how to navigate them. With the right knowledge and strategies, you can use Java REPL effectively and avoid common pitfalls.
Understanding REPL and Its Significance in Programming
REPL stands for Read-Eval-Print Loop. It’s a simple, interactive computer programming environment that takes single user inputs, executes them, and returns the result to the user. A REPL environment allows you to execute code and see the results in real-time, which is particularly useful for learning new concepts, debugging code, and performing data analysis.
// In a REPL environment
jshell> String greeting = "Hello, REPL!"
greeting ==> "Hello, REPL!"
jshell> System.out.println(greeting);
Hello, REPL!
In this example, we’ve created a string variable greeting
and printed it. The output Hello, REPL!
is displayed immediately.
The Evolution of Java and the Introduction of REPL
Java, developed by Sun Microsystems (now owned by Oracle), has been a mainstay in the world of computer programming since its inception in 1995. It’s used in various domains, from web applications to mobile apps to enterprise systems.
Despite its widespread use, Java lacked an interactive programming environment for many years. This changed in 2017 with the release of Java 9, which introduced JShell, Java’s own REPL tool.
The introduction of JShell marked a significant milestone in Java’s evolution. It provided Java developers with a new way to run and test code snippets quickly, without the need to write a full program or set up a testing environment. This has made Java more accessible to beginners and more convenient for experienced developers.
// In Java REPL (JShell)
jshell> int x = 10;
x ==> 10
jshell> int y = 20;
y ==> 20
jshell> int sum = x + y;
sum ==> 30
In this example, we’ve used JShell to declare two integer variables x
and y
, add them together, and store the result in a third variable sum
. The output sum ==> 30
is displayed immediately, showing the result of our operation.
Java REPL in Real-World Programming
Java REPL, while primarily a learning and debugging tool, also has relevance in real-world programming. It provides a quick and easy way to test out ideas, explore new Java features, and debug code snippets. This can be particularly useful in a fast-paced development environment where time is of the essence.
Exploring Related Concepts
The introduction of Java REPL in Java 9 is part of a broader trend towards more interactive and user-friendly programming tools. If you’re interested in this topic, you might also want to explore related concepts like other Java development tools, the new features introduced in Java 9 and subsequent releases, and the evolution of Java as a language and platform.
The Impact of Java REPL on Java Development
Java REPL has had a significant impact on the way Java is learned and taught. By providing immediate feedback, it has made the learning process more interactive and engaging. This has the potential to attract more beginners to the language and help them progress faster.
Java REPL has also influenced the way Java code is written and tested. Developers can now quickly try out different approaches and see their effects in real-time. This can lead to more efficient code and faster problem-solving.
Further Resources for Mastering Java REPL
To deepen your understanding of Java REPL and its applications, you might find the following resources helpful:
- Fundamentals Covered: Java Tutorial – Master advanced Java topics like generics, annotations, and reflection.
Multithreading in Java – Explore multithreading techniques, including synchronization and inter-thread communication.
Functions of Java – Learn how to define, invoke, and organize functions in Java classes.
The JShell User Guide – This official guide from Oracle provides an in-depth look at the features and usage of Java REPL.
Java 9: An Introduction to JShell – This article on Baeldung offers a practical introduction to Java REPL.
Java REPL (JShell) Tutorial provides instructions on JShell, which allows you to execute Java code and see the results immediately.
Wrapping Up: Mastering Java REPL
In this comprehensive guide, we’ve journeyed through the world of Java REPL, an interactive tool for learning and experimenting with Java.
We started with the basics, learning how to use Java REPL for simple operations and understanding its advantages and pitfalls. We then ventured into more advanced territory, exploring complex uses of Java REPL, such as testing code snippets, debugging, and learning new Java features.
Along the way, we tackled common challenges you might face when using Java REPL, such as unsaved work, unexpected results, and startup issues, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to interactive Java development, comparing Java REPL with other tools like the Eclipse JShell plugin, IntelliJ IDEA, and the Groovy Console. Here’s a quick comparison of these tools:
Tool | Interactivity | Integration | Language Support |
---|---|---|---|
Java REPL | High | None | Java |
Eclipse JShell plugin | High | Eclipse | Java |
IntelliJ IDEA | High | IntelliJ | Java |
Groovy Console | High | None | Groovy |
Whether you’re just starting out with Java REPL or you’re looking to level up your Java skills, we hope this guide has given you a deeper understanding of Java REPL and its capabilities.
With its balance of interactivity, ease of use, and immediate feedback, Java REPL is a powerful tool for learning and experimenting with Java. Happy coding!