Using JDoodle: Your Online Compiler and Editor
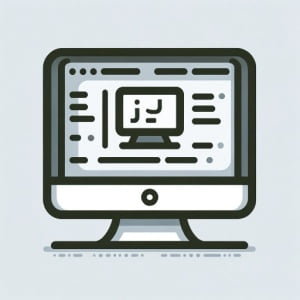
Ever found yourself needing to compile and run a code snippet quickly, but dread the idea of setting up a local development environment? You’re not alone. Many developers find themselves in this situation, but there’s a tool that can make this process a breeze.
Think of JDoodle as your online compiler and editor – a versatile platform that allows you to code in multiple languages without the need for a local setup. It’s like having a development environment at your fingertips, anytime, anywhere.
This guide will walk you through how to use JDoodle, its features, and its pros and cons. We’ll explore JDoodle’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering JDoodle!
TL;DR: What is JDoodle and How Do I Use It?
JDoodle
is an online compiler and editor that supports multiple programming languages, accessed atwww.jdoodle.com
. To use it, you simply select your language, enter your code in the editor, and click'Execute'
.
Here’s a simple example:
# Select Python as your language in JDoodle
print('Hello, JDoodle!')
# Output:
# 'Hello, JDoodle!'
In this example, we’ve selected Python as our language in JDoodle and entered a simple print statement. After clicking ‘Execute’, JDoodle compiles and runs the code, and we see the output ‘Hello, JDoodle!’.
This is a basic way to use JDoodle, but there’s much more to learn about this versatile online compiler and editor. Continue reading for more detailed instructions and advanced features.
Table of Contents
Getting Started with JDoodle
JDoodle is incredibly intuitive, making it easy for beginners to get started. Here’s a step-by-step guide to using JDoodle for the first time.
Selecting a Programming Language
The first step is to select your preferred programming language. JDoodle supports a wide range of languages, from Python and JavaScript to C++ and Java. You can find the list of supported languages in a dropdown menu at the top of the JDoodle interface.
Entering and Executing Code
Once you’ve selected your language, you can start coding. Type your code into the editor panel. When you’re ready to run your code, simply click the ‘Execute’ button.
Here’s an example using Python:
# Select Python as your language in JDoodle
print('Hello, World!')
# Click 'Execute'
# Output:
# 'Hello, World!'
In this example, we’ve selected Python as our language, written a simple print statement, and clicked ‘Execute’. JDoodle compiles and runs the code, and we see the output ‘Hello, World!’.
Interpreting the Results
The output panel displays the results of your code. It’s located below the editor panel. Any print statements, return values, or errors will appear here. This immediate feedback is what makes JDoodle such a powerful tool for learning and testing code.
In our example, the output ‘Hello, JDoodle!’ appears in the output panel. This tells us that our print statement executed successfully. If there were any issues with our code, the output panel would display an error message instead, helping us to troubleshoot the problem.
JDoodle’s Advanced Features
Once you’re comfortable with the basics of JDoodle, it’s time to explore some of its advanced features. JDoodle comes packed with additional functionality that can elevate your coding experience, such as using stdin, working with files, and using command-line arguments.
Using stdin
Standard input, or stdin, is a way to provide input to your programs. In JDoodle, you can use the stdin panel to enter input for your code.
Here’s an example using Python:
# Select Python as your language in JDoodle
name = input('Enter your name: ')
print(f'Hello, {name}!')
# In the stdin panel, enter 'JDoodle'
# Click 'Execute'
# Output:
# 'Enter your name: '
# 'Hello, JDoodle!'
In this example, we’ve written a Python program that asks for your name and then greets you. We entered ‘JDoodle’ in the stdin panel. After clicking ‘Execute’, JDoodle runs the code and we see the output ‘Hello, JDoodle!’.
Working with Files
JDoodle allows you to work with files. You can create, read, write, and delete files as you would in a local environment. Here’s an example of creating and writing to a file in Python:
# Select Python as your language in JDoodle
with open('example.txt', 'w') as file:
file.write('Hello, JDoodle!')
# Click 'Execute'
# Output:
# 'File created: example.txt'
# 'File contents: Hello, JDoodle!'
In this example, we’ve created a file named ‘example.txt’ and written ‘Hello, JDoodle!’ to it.
Using Command-Line Arguments
JDoodle also supports command-line arguments. You can enter these arguments in the args panel. Here’s an example using Python:
# Select Python as your language in JDoodle
import sys
for arg in sys.argv:
print(f'Argument: {arg}')
# In the args panel, enter '1 2 3'
# Click 'Execute'
# Output:
# 'Argument: script'
# 'Argument: 1'
# 'Argument: 2'
# 'Argument: 3'
In this example, we’ve written a Python program that prints each command-line argument. We entered ‘1 2 3’ in the args panel. After clicking ‘Execute’, JDoodle runs the code and we see the output ‘Argument: 1’, ‘Argument: 2’, and ‘Argument: 3’.
Exploring Alternatives to JDoodle
While JDoodle is a powerful tool for online coding, it’s not the only player in the game. There are several other platforms that offer similar functionality, each with its own set of pros and cons. Let’s explore some of these alternatives.
Repl.it: An Online IDE
Repl.it is a full-fledged online integrated development environment (IDE). It supports a wide array of languages and offers a collaborative coding environment.
# To use Repl.it, simply visit repl.it in your web browser
# Select your preferred language and start coding
print('Hello, Repl.it!')
# Click 'Run'
# Output:
# 'Hello, Repl.it!'
Repl.it provides a more comprehensive set of features compared to JDoodle, such as real-time collaboration and hosting of web apps. However, it may be a bit overwhelming for beginners due to its extensive feature set.
CodeChef: A Platform for Competitive Programming
CodeChef is a competitive programming platform that also provides an online compiler. It supports a wide range of languages and is known for its large collection of coding challenges.
# To use CodeChef, visit codechef.com/ide
# Select your language and start coding
printf('Hello, CodeChef!');
# Click 'Run'
# Output:
# 'Hello, CodeChef!'
CodeChef is a great platform for honing your coding skills through challenges. However, its primary focus is competitive programming, so it might not be the best choice for simple code testing.
Local Development Environments: Full Control and Flexibility
Local development environments, such as Visual Studio Code or PyCharm, offer the most control and flexibility. They require setup and maintenance, but in return, provide a wide array of features and customization options.
# To use a local development environment, you need to install it on your machine
# Here's an example of running Python code in a local environment
python3 hello.py
# Output:
# 'Hello, World!'
Local environments are ideal for large projects and offer the best performance. However, they lack the convenience and simplicity of online compilers for quick code snippets or when you’re on the go.
Like any tool, JDoodle isn’t without its quirks. Let’s discuss some common issues you might encounter while using JDoodle, and how to troubleshoot them.
Compilation Errors
Compilation errors occur when your code has syntax errors or undefined references. JDoodle will display these errors in the output panel.
# An example of a compilation error in Python
print('Hello, JDoodle!'
# Click 'Execute'
# Output:
# 'SyntaxError: unexpected EOF while parsing'
In this example, we forgot to close the print statement with a parenthesis, resulting in a SyntaxError. The solution is to correct the syntax by adding the missing parenthesis.
Runtime Errors
Runtime errors are issues that occur while your code is running. These could be logical errors or unhandled exceptions.
# An example of a runtime error in Python
print(5 / 0)
# Click 'Execute'
# Output:
# 'ZeroDivisionError: division by zero'
In this example, we’re trying to divide by zero, which is not allowed in mathematics. This results in a ZeroDivisionError. The solution is to handle this exception or fix the logic that led to the division by zero.
Limitations of the Online Platform
JDoodle, being an online platform, has certain limitations compared to a local development environment. For instance, it might not support certain libraries or have restrictions on execution time and memory usage.
# An example of a limitation in JDoodle
import tensorflow as tf
# Click 'Execute'
# Output:
# 'ModuleNotFoundError: No module named 'tensorflow''
In this example, we’re trying to import the TensorFlow library, which is not supported by JDoodle. The solution would be to use a local environment or a cloud-based platform that supports TensorFlow for this specific task.
The Power of Online Compilers and Editors
In the rapidly evolving world of programming, online compilers and editors such as JDoodle have emerged as a game-changer. Their importance and role in modern programming cannot be understated, especially in the context of cloud computing and remote work.
The Rise of Online Compilers
Online compilers allow you to write, compile, and run code directly in your web browser. This eliminates the need for setting up and maintaining a local development environment, which can be a time-consuming and complex process, especially for beginners.
# An example of using an online compiler
# Select Python as your language in JDoodle
print('Hello, Online Compiler!')
# Click 'Execute'
# Output:
# 'Hello, Online Compiler!'
In this example, we’re using JDoodle to run a simple Python print statement. This demonstrates the ease and convenience of using an online compiler. You can execute code in seconds, without any setup.
The Impact of Cloud Computing
Cloud computing has revolutionized the way we store data and run applications. It’s no surprise that it’s also transforming the way we code. Online compilers and editors leverage the power of the cloud, allowing you to code from any device, anywhere, anytime.
# An example of the benefits of cloud computing
# You can access your code on JDoodle from any device
# Just log in to your JDoodle account and your code is there
This example illustrates the flexibility and accessibility provided by cloud-based online compilers. Your code is stored in the cloud, so you can access it from any device with an internet connection.
The Future of Remote Work
With the rise of remote work, having a portable and accessible coding environment is more important than ever. Online compilers and editors like JDoodle meet this need, making them an essential tool for the modern programmer.
# An example of the benefits of remote work
# You can code from home, a coffee shop, or even a beach
# As long as you have an internet connection, you can use JDoodle
This example highlights the freedom and flexibility provided by online compilers in a remote work setting. As long as you have an internet connection, you can code from anywhere.
JDoodle in the Larger Development Workflow
JDoodle is not just an online compiler and editor, it’s a tool that can seamlessly integrate into larger development workflows. This includes continuous integration and continuous deployment (CI/CD) practices, which are now industry standards in software development.
JDoodle and Continuous Integration
Continuous Integration (CI) is a practice where developers integrate their changes back to the main branch as often as possible. This is usually supported by automated build and test processes. JDoodle can be a part of this process, serving as a platform for quick testing and debugging.
# An example of using JDoodle in CI
# You can quickly test a function or a class in JDoodle
# before integrating it into the main branch
The above example demonstrates how JDoodle can fit into a CI workflow. Before integrating a piece of code into the main branch, you can quickly test it in JDoodle to ensure it works as expected.
JDoodle and Continuous Deployment
Continuous Deployment (CD) is a practice where code changes are automatically built, tested, and prepared for release to production. JDoodle can serve as a preliminary testing environment before the code is pushed to the CD pipeline.
# An example of using JDoodle in CD
# You can run your code in JDoodle
# to ensure it's ready for the CD pipeline
In the above example, JDoodle is used as a first line of defense in the CD process. By running the code in JDoodle, you can catch potential issues early, before they enter the CD pipeline.
Further Resources for JDoodle
To delve deeper into JDoodle and related topics, check out the following resources:
- Beginner’s Guide to Java: Tutorial Edition – Explore Java fundamentals, including variables, loops, and conditionals.
Exploring Java IDEs Online – Explore features such as syntax highlighting and debugging support in online Java IDEs.
Exploring JVM Concepts – Explore the benefits and capabilities of the JVM for running Java programs.
JDoodle’s Official Documentation – Access JDoodle’s official documentation for details on the online compiler’s usage.
A Guide to CI/CD – Get a complete understanding of continuous integration and continuous delivery.
JDoodle Online Compiler – How to write programs on your website and redirect them to JDoodle online compiler.
These resources provide a wealth of information on JDoodle, CI/CD practices, and cloud-based development environments, helping you to enhance your skills and knowledge.
Wrapping Up: JDoodle for Online Coding
In this comprehensive guide, we’ve journeyed through the world of JDoodle, an online compiler and editor that supports multiple programming languages.
We began with the basics, learning how to select a programming language, enter code, and execute it using JDoodle. We then ventured into more advanced territory, exploring JDoodle’s advanced features such as using stdin, working with files, and using command-line arguments. Along the way, we tackled common issues that you might encounter when using JDoodle, such as compilation errors and runtime errors, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to online coding, comparing JDoodle with other platforms like Repl.it and CodeChef, as well as local development environments. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
JDoodle | Quick and easy online coding | Limited library support |
Repl.it | Full-fledged online IDE | Can be overwhelming for beginners |
CodeChef | Great for competitive programming | Not ideal for simple code testing |
Local Environments | Full control and flexibility | Requires setup and maintenance |
Whether you’re just starting out with JDoodle or you’re looking to level up your online coding skills, we hope this guide has given you a deeper understanding of JDoodle and its capabilities.
With its balance of simplicity, convenience, and power, JDoodle is a valuable tool for any programmer. Happy coding!