Mastering Java Arrays: An In-depth Guide
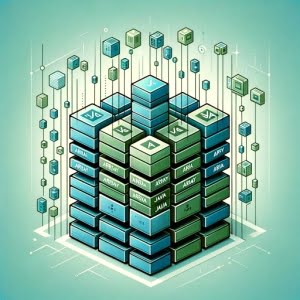
Ever find yourself grappling with arrays in Java? You’re not alone. Many developers find arrays in Java a bit challenging, but consider arrays as a well-organized bookshelf – they help store and manage your data efficiently.
Arrays are a fundamental data structure in Java, crucial for storing and processing data in any Java program. They can store multiple values of the same type and are especially useful when you want to work with a fixed number of elements.
This guide will walk you through the process of creating, initializing, and manipulating arrays in Java. We’ll cover everything from declaring and initializing arrays, accessing array elements, to more advanced topics like multi-dimensional arrays and array manipulation techniques. We’ll also explore alternative data structures to arrays and discuss common issues and their solutions.
So, let’s dive in and start mastering arrays in Java!
TL;DR: What is an Array in Java and How Do I Use It?
An array in Java is a data structure that can store multiple values of the same data type, declared with ‘[]’:
int[] myArray
. It’s like a container that holds a fixed number of values of a single type. Here is a full example:
int[] myArray = new int[]{1, 2, 3, 4, 5};
In this example, we’ve declared an array named myArray
and initialized it with the values 1, 2, 3, 4, and 5. The int[]
before the array name indicates that this array will hold integers.
This is a basic way to use arrays in Java, but there’s much more to learn about creating, manipulating, and using arrays effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Declaring, Initializing, and Accessing Java Arrays: A Beginner’s Guide
- Mastering Multi-Dimensional Arrays and Array Manipulation in Java
- Exploring Alternatives to Java Arrays
- Navigating Common Errors and Best Practices with Java Arrays
- Unraveling the Fundamentals of Java Arrays
- Expanding Your Java Array Knowledge: Applications and Related Topics
- Wrapping Up: Arrays in Java
Declaring, Initializing, and Accessing Java Arrays: A Beginner’s Guide
Java arrays are simple yet powerful tools for storing and managing data. Let’s break down the process into three basic steps: declaring, initializing, and accessing elements in a Java array.
Declaring an Array in Java
The first step in using an array is declaring it. To declare an array in Java, you specify the type of elements it will hold followed by square brackets and the array’s name. Here’s an example:
int[] myArray;
In this line of code, we’ve declared an array named myArray
that will hold integers.
Initializing an Array in Java
After declaring an array, the next step is initializing it, which means assigning values to it. Here’s how you can initialize the myArray
we declared earlier:
myArray = new int[]{6, 7, 8, 9, 10};
In this example, we’ve initialized myArray
with the values 6, 7, 8, 9, and 10.
Accessing Elements in a Java Array
Once an array is declared and initialized, you can access its elements using their index. Array indices in Java start at 0, so the first element is at index 0, the second element is at index 1, and so on. Here’s how you can access the first element of myArray
:
int firstElement = myArray[0];
System.out.println(firstElement);
# Output:
# 1
In this example, we’ve accessed and printed the first element of myArray
, which is 1.
By mastering these basic steps, you’ll have a solid foundation for working with arrays in Java. In the next section, we’ll explore more advanced uses of Java arrays.
Mastering Multi-Dimensional Arrays and Array Manipulation in Java
As you become more comfortable with basic arrays, it’s time to explore more advanced concepts. In this section, we’ll discuss multi-dimensional arrays and array manipulation techniques in Java.
Multi-Dimensional Arrays in Java
A multi-dimensional array is essentially an array of arrays. A two-dimensional array, for instance, is like a table with rows and columns. Here’s how you can declare, initialize, and access a two-dimensional array in Java:
int[][] twoDArray = new int[][]{{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
System.out.println(twoDArray[1][2]);
# Output:
# 6
In this example, we’ve created a two-dimensional array named twoDArray
with three rows and three columns. We then printed the element at row 1, column 2, which is 6.
Array Manipulation Techniques in Java
Manipulating arrays involves tasks like adding, deleting, or modifying array elements. Here’s an example where we change the value of an array element:
int[] myArray = new int[]{1, 2, 3, 4, 5};
myArray[2] = 10;
System.out.println(myArray[2]);
# Output:
# 10
In this example, we’ve changed the third element (at index 2) of myArray
from 3 to 10, then printed the new value.
Remember, arrays in Java are fixed in size. To add or delete elements, you’ll need to create a new array or use a dynamic data structure like an ArrayList, which we’ll discuss in a later section.
By understanding multi-dimensional arrays and array manipulation techniques, you can handle more complex data structures and solve a wider range of problems in Java.
Exploring Alternatives to Java Arrays
While arrays are an essential data structure in Java, there are alternative data structures that offer more flexibility and functionality. In this section, we’ll explore two popular alternatives: ArrayLists and LinkedLists.
Unleashing the Power of ArrayLists
ArrayLists are dynamic data structures that can grow and shrink at runtime, making them a flexible alternative to arrays. Let’s look at how to declare, initialize, and manipulate an ArrayList:
import java.util.ArrayList;
ArrayList<Integer> myList = new ArrayList<Integer>();
myList.add(1);
myList.add(2);
myList.add(3);
System.out.println(myList.get(1));
# Output:
# 2
In this example, we’ve created an ArrayList named myList
and added three elements to it. We then printed the second element, which is 2. Notice how we used the add
method to add elements and the get
method to access elements.
Leveraging LinkedLists in Java
LinkedLists, like ArrayLists, are dynamic. However, they offer efficient insertion and removal of elements at the beginning and end. Here’s an example of declaring, initializing, and manipulating a LinkedList:
import java.util.LinkedList;
LinkedList<Integer> myLinkedList = new LinkedList<Integer>();
myLinkedList.add(1);
myLinkedList.add(2);
myLinkedList.add(3);
System.out.println(myLinkedList.get(1));
# Output:
# 2
In this example, we’ve created a LinkedList named myLinkedList
and added three elements to it. We then printed the second element, which is 2. The syntax is similar to ArrayList, but LinkedList has different performance characteristics.
Both ArrayLists and LinkedLists have their strengths and weaknesses. ArrayLists provide fast random access but slow insertions and deletions in the middle, while LinkedLists offer fast insertions and deletions but slow random access. Your choice between arrays, ArrayLists, and LinkedLists should depend on your specific needs and the trade-offs you’re willing to make.
Even with an understanding of arrays in Java, it’s common to encounter errors. In this section, we’ll discuss some common issues while working with arrays and their solutions, along with tips for optimization.
Array Index Out of Bounds
One of the most common errors when working with arrays is trying to access an index that does not exist. This will throw an ArrayIndexOutOfBoundsException
. Here’s an example:
int[] myArray = new int[]{1, 2, 3, 4, 5};
System.out.println(myArray[5]);
# Output:
# Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
In this example, we’ve tried to access the element at index 5 of myArray
, which does not exist. To avoid this error, always ensure that the index you’re trying to access is within the array’s bounds.
Null Array Reference
Another common error is trying to access an array that has not been initialized, which will throw a NullPointerException
. Here’s an example:
int[] myArray;
System.out.println(myArray[0]);
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve tried to access an element of myArray
before initializing it. To avoid this error, always initialize your arrays before trying to access their elements.
Best Practices and Optimization
When working with arrays in Java, it’s important to follow best practices for efficiency and readability. Here are a few tips:
- Always initialize your arrays before using them to avoid
NullPointerException
- Check that an index is within the array’s bounds before accessing it to avoid
ArrayIndexOutOfBoundsException
- Use enhanced for-loops (also known as for-each loops) for easier and safer iteration over arrays
- Consider using alternative data structures like ArrayLists or LinkedLists for more flexibility and functionality
By understanding these common errors and best practices, you’ll be well-equipped to handle arrays in Java effectively and efficiently.
Unraveling the Fundamentals of Java Arrays
To truly master arrays in Java, we need to delve into some fundamental concepts and understand why arrays are so essential in programming. We’ll also discuss memory allocation for arrays and the difference between static and dynamic arrays.
The Importance of Arrays in Programming
Arrays are a cornerstone of many programming languages, not just Java. They provide a way to store multiple values of the same type under a single identifier, making data management more efficient. Whether you’re working with a list of student grades, a collection of employee names, or a sequence of numbers, arrays can make your job easier.
Memory Allocation for Java Arrays
When you declare an array in Java, the system allocates a block of memory large enough to hold the array’s elements. The size of this block depends on the array’s type and length. For example, if you declare an array of integers (which are 4 bytes each) with a length of 10, the system will allocate a block of 40 bytes.
int[] myArray = new int[10];
In this example, myArray
takes up 40 bytes of memory.
Static vs Dynamic Arrays
In Java, arrays are static, meaning their size is fixed at the time of creation. Once an array is created, you cannot add or remove elements (though you can change their values). This can be limiting when you need a more flexible data structure.
int[] myArray = new int[5];
myArray[5] = 6; // This will throw an ArrayIndexOutOfBoundsException
# Output:
# Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
In this example, we’ve tried to add a sixth element to myArray
, which only has space for five. This throws an ArrayIndexOutOfBoundsException
.
Dynamic arrays, on the other hand, can grow and shrink at runtime. In Java, dynamic arrays are implemented using classes like ArrayList. These classes provide methods to add and remove elements, making them a more flexible alternative to static arrays.
import java.util.ArrayList;
ArrayList<Integer> myList = new ArrayList<Integer>();
myList.add(1);
myList.add(2);
myList.add(3);
myList.add(4);
myList.add(5);
myList.add(6); // This is perfectly fine
# Output:
# No errors
In this example, we’ve added six elements to myList
, which is an ArrayList. Unlike with the static array, this does not throw an error.
By understanding these fundamentals, you’ll have a deeper appreciation for arrays in Java and be better equipped to use them effectively.
Expanding Your Java Array Knowledge: Applications and Related Topics
Arrays in Java are not just standalone entities; they are a part of a larger ecosystem of data structures and algorithms. Understanding the application of arrays in larger projects and algorithms can significantly enhance your programming skills. Let’s explore some related topics that can broaden your knowledge horizon.
Java Array Applications in Larger Projects
In larger projects, arrays are used to store and manipulate data efficiently. They can be used to implement various data structures and algorithms. For example, in a project that involves sorting a list of names, an array can be used to store the names, and a sorting algorithm can be applied to the array to sort the names.
Sorting Algorithms and Java Arrays
Sorting algorithms are fundamental algorithms in computer science, and many of them use arrays. For example, the Bubble Sort algorithm repeatedly steps through the array, compares adjacent elements, and swaps them if they are in the wrong order. The pass through the array is repeated until the array is sorted.
Data Structures and Object-Oriented Programming in Java
Arrays are just one type of data structure in Java. Understanding other data structures, such as linked lists, trees, and graphs, can open up new possibilities for data management and manipulation. Moreover, Java is an object-oriented programming language, so understanding object-oriented programming concepts like classes, objects, inheritance, and polymorphism can help you use arrays more effectively.
Further Resources for Mastering Java Arrays
For those looking to delve deeper into Java arrays and related topics, here are some valuable resources:
- Exploring Java Data Structures – Learn about arrays, lists, sets, maps, and other data structures available in Java.
Declaring Arrays in Java – Learn about array literals, constructors, and dynamic initialization methods.
Java Array Methods – Discover useful methods for manipulating arrays in Java programming.
Oracle’s Java Tutorials offer comprehensive guides on various Java topics, including arrays.
GeeksforGeeks Arrays in Java Articles provides a wealth of info on Java arrays, data structures, and algorithms.
Codecademy’s Java Course includes interactive lessons on Java arrays and other topics.
By exploring these resources and understanding the broader context of arrays in Java, you’ll be well on your way to mastering Java programming.
Wrapping Up: Arrays in Java
In this comprehensive guide, we’ve delved into the world of arrays in Java, a fundamental data structure that can store multiple values of the same type.
We began with the basics, learning how to declare, initialize, and access elements in a Java array. We then ventured into more advanced territory, exploring multi-dimensional arrays and array manipulation techniques. Along the way, we tackled common challenges you might face when working with arrays, such as ArrayIndexOutOfBoundsException
and NullPointerException
, providing you with solutions and best practices for each issue.
We also looked at alternative approaches to data manipulation in Java, comparing arrays with other data structures like ArrayLists and LinkedLists. Here’s a quick comparison of these data structures:
Data Structure | Flexibility | Ease of Use | Memory Efficiency |
---|---|---|---|
Array | Low | High | High |
ArrayList | High | High | Moderate |
LinkedList | High | Moderate | Low |
Whether you’re just starting out with arrays in Java or you’re looking to level up your data manipulation skills, we hope this guide has given you a deeper understanding of arrays and their capabilities.
With its balance of simplicity, memory efficiency, and ease of use, arrays are a powerful tool for data manipulation in Java. Now, you’re well equipped to enjoy those benefits. Happy coding!