Selenium Java: Web Application Testing Guide
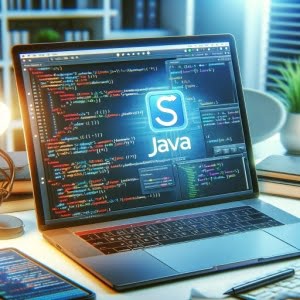
Are you wrestling with web application testing? You’re not alone. Many developers find themselves in a bind when it comes to effective and efficient testing. But there’s a tool that can make this process a breeze.
Like a skilled puppeteer, Selenium with Java can help you automate and control your testing process. These tools can help you create robust, browser-based regression automation suites and tests, scale and distribute scripts across many environments, and more.
This guide will walk you through the steps of using Selenium with Java for effective web application testing. We’ll explore Selenium’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Selenium with Java!
TL;DR: How Do I Set Up Selenium with Java for Web Application Testing?
To set up
Selenium
with Java for web application testing, you first need to install Java and Selenium WebDriver, which can be found here:www.selenium.dev/downloads
. Once installed you will need to add the location to your system’s PATH, for example:export PATH=$PATH:/path/to/webdriver
. Then, you need to configure your IDE (like Eclipse or IntelliJ) to write and run your test scripts.
Here’s a simple example of how you might set up Selenium WebDriver with Java:
// Import the WebDriver package
import org.openqa.selenium.WebDriver;
// Import the FirefoxDriver class
import org.openqa.selenium.firefox.FirefoxDriver;
public class MyClass {
public static void main(String[] args) {
// Set the path to the geckodriver executable
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
// Use the driver to visit a website
driver.get("http://www.google.com");
// Close the browser
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.google.com, and then closes.
In this example, we import the necessary Selenium WebDriver classes, set the path to the geckodriver executable (which is necessary for Firefox), create a new instance of the Firefox driver, use it to visit Google, and then close the browser.
This is just the beginning of what you can do with Selenium and Java. Continue reading for a more detailed step-by-step process, from installation to writing advanced test scripts.
Table of Contents
- Setting Up Selenium Java: A Beginner’s Guide
- Advanced Selenium Java: Beyond Basics
- Selenium Java: Exploring Alternative Tools and Libraries
- Troubleshooting Selenium Java: Common Issues and Solutions
- Understanding Selenium Java: The Fundamentals
- Selenium Java in the Real World: CI/CD and Agile Development
- Wrapping Up: Selenium Java for Web Application Testing
Setting Up Selenium Java: A Beginner’s Guide
Before we can start writing our test scripts, we need to set up our environment. This involves installing Java and Selenium WebDriver, and configuring our IDE. Here’s a step-by-step guide on how to do it.
Installing Java
First, we need to install Java. This can be done by downloading the latest Java Development Kit (JDK) from Oracle’s official website and following the installation instructions.
Installing Selenium WebDriver
Next, we need to set up Selenium WebDriver. This can be done by downloading the WebDriver language binding of your choice from Selenium’s official website and adding its location to your system’s PATH.
Here’s an example of how you might do this on a Unix-based system:
export PATH=$PATH:/path/to/webdriver
Configuring Your IDE
Finally, we need to configure our IDE to use Selenium WebDriver. Most popular IDEs like Eclipse and IntelliJ have built-in support for Selenium WebDriver. This usually involves adding the WebDriver JAR files to your project’s build path.
Now that we have our environment set up, we can start writing our first simple test script.
Writing Your First Selenium Java Test Script
Here’s an example of a simple Selenium Java test script that opens a web page and checks its title:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class MyFirstTest {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("http://www.google.com");
String pageTitle = driver.getTitle();
System.out.println("Page title is: " + pageTitle);
driver.quit();
}
}
# Output:
# 'Page title is: Google'
In this script, we first set the system property for the Firefox driver, create a new FirefoxDriver instance, and use it to open Google’s homepage. We then get the page title and print it to the console. Finally, we close the driver.
This simple script demonstrates the power of Selenium Java. With just a few lines of code, we can automate a common web browsing task. However, it’s just the tip of the iceberg. Selenium Java offers much more advanced functionality, which we’ll explore in the next sections.
Advanced Selenium Java: Beyond Basics
Once you’ve mastered the basics of Selenium Java, it’s time to dive into more complex scenarios. This includes testing form inputs, handling pop-up windows, and testing AJAX-based applications. Let’s explore each of these in detail.
Testing Form Inputs
Form input testing is a critical aspect of web application testing. Selenium Java provides a simple and intuitive API for interacting with form elements. Here’s an example of a test script that fills out a form and submits it:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
public class FormTest {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("http://www.example.com/form");
WebElement nameField = driver.findElement(By.name("name"));
nameField.sendKeys("John Doe");
WebElement submitButton = driver.findElement(By.name("submit"));
submitButton.click();
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.example.com/form, fills the 'name' field with 'John Doe', clicks the 'submit' button, and then closes.
In this script, we first navigate to the form page. We then locate the name field and submit button using the findElement
method and the By.name
locator. We fill out the name field using the sendKeys
method and submit the form using the click
method.
Handling Pop-Up Windows
Pop-up windows can be a challenge to handle in automated tests. However, Selenium Java provides a way to switch the driver’s context to the pop-up window. Here’s an example:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class PopupTest {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("http://www.example.com/popup");
String mainWindow = driver.getWindowHandle();
driver.findElement(By.name("open-popup")).click();
for (String windowHandle : driver.getWindowHandles()) {
if (!windowHandle.equals(mainWindow)) {
driver.switchTo().window(windowHandle);
System.out.println(driver.getTitle());
driver.close();
}
}
driver.switchTo().window(mainWindow);
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.example.com/popup, opens a new pop-up window, prints the pop-up's title, closes the pop-up, and then closes the main window.
In this script, we first save the main window’s handle. We then click the button that opens the pop-up. We iterate over all window handles, switch to the pop-up window when we find it, print its title, and close it. Finally, we switch back to the main window and close it.
Testing AJAX-Based Applications
Testing AJAX-based applications can be tricky due to the asynchronous nature of AJAX. However, Selenium Java provides explicit and implicit waits to handle this. Here’s an example:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class AjaxTest {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("http://www.example.com/ajax");
driver.findElement(By.name("load-data")).click();
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement dataElement = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("data")));
System.out.println(dataElement.getText());
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.example.com/ajax, clicks the 'load-data' button, waits for the 'data' element to become visible, prints its text, and then closes.
In this script, we first navigate to the AJAX page and click the button that loads data. We then create a WebDriverWait instance and use it to wait for the data element to become visible. Once it’s visible, we print its text and close the driver.
These examples demonstrate the versatility of Selenium Java. Whether you’re testing form inputs, handling pop-up windows, or testing AJAX-based applications, Selenium Java has you covered.
Selenium Java: Exploring Alternative Tools and Libraries
Selenium Java is a powerful tool for web application testing. But did you know that you can augment its functionality with additional tools and libraries? In this section, we’ll explore some of these alternatives, including TestNG, JUnit, and Maven.
Enhancing Your Tests with TestNG
TestNG is a testing framework that can be used alongside Selenium to provide additional functionality, such as flexible test configuration, parallel test execution, and test-driven development.
Here’s an example of how you might use TestNG to structure your Selenium tests:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.testng.Assert;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
public class TestNGExample {
private WebDriver driver;
@BeforeClass
public void setUp() {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
driver = new FirefoxDriver();
}
@Test
public void testPageTitle() {
driver.get("http://www.google.com");
Assert.assertEquals(driver.getTitle(), "Google");
}
@AfterClass
public void tearDown() {
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.google.com, checks that the page title is 'Google', and then closes.
In this script, we use TestNG’s @BeforeClass
and @AfterClass
annotations to set up and tear down our test environment. We use the @Test
annotation to mark our test method, and the Assert
class to verify that the page title is correct.
Structuring Your Tests with JUnit
JUnit is another testing framework that can be used with Selenium. It provides a set of annotations and assertions to help you structure and write your tests.
Here’s an example of how you might use JUnit to structure your Selenium tests:
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class JUnitExample {
private WebDriver driver;
@Before
public void setUp() {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
driver = new FirefoxDriver();
}
@Test
public void testPageTitle() {
driver.get("http://www.google.com");
org.junit.Assert.assertEquals("Google", driver.getTitle());
}
@After
public void tearDown() {
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.google.com, checks that the page title is 'Google', and then closes.
In this script, we use JUnit’s @Before
and @After
annotations to set up and tear down our test environment. We use the @Test
annotation to mark our test method, and the Assert
class to verify that the page title is correct.
Managing Your Project with Maven
Maven is a project management tool that can help you manage your Selenium Java projects. It can handle project build, reporting, and documentation from a central piece of information.
Here’s an example of how you might set up a Maven project for your Selenium tests:
mvn archetype:generate -DgroupId=com.mycompany.app -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command generates a new Maven project with the specified group ID and artifact ID. You can then add your Selenium tests to the src/test/java
directory, and manage your project dependencies using the pom.xml
file.
Each of these tools offers unique advantages. TestNG and JUnit provide flexible and powerful frameworks for structuring your tests, while Maven offers robust project management capabilities. Depending on your needs, you might find one or more of these tools useful in your Selenium Java testing.
Troubleshooting Selenium Java: Common Issues and Solutions
Like any tool, Selenium Java has its quirks. It’s not uncommon to encounter issues during testing. However, these issues are usually well-documented, and there are often solutions or workarounds available. Let’s explore some of the most common issues you may encounter when using Selenium Java.
Dealing with NoSuchElementException
The NoSuchElementException
is one of the most common exceptions in Selenium. It occurs when Selenium can’t find an element using the provided locator (or criteria).
Here’s an example of a test script that could throw this exception:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
public class NoSuchElementExceptionExample {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("http://www.google.com");
WebElement nonExistentElement = driver.findElement(By.id("non-existent-id"));
nonExistentElement.click();
driver.quit();
}
}
# Output:
# Exception in thread "main" org.openqa.selenium.NoSuchElementException: Unable to locate element: #non-existent-id
In this script, we’re trying to find an element with an ID of “non-existent-id”. Because no such element exists on the page, Selenium throws a NoSuchElementException
.
To avoid this exception, you can use the findElements
method, which returns a list of elements. You can then check if the list is empty before trying to interact with the element.
Handling Dynamic Elements
Dynamic elements can pose a challenge in Selenium tests. These are elements that are not immediately available and require some time to load. If you try to interact with a dynamic element before it’s fully loaded, you’ll likely encounter a StaleElementReferenceException
.
To handle this, you can use Selenium’s wait functionality. Here’s an example:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedConditions;
import org.openqa.selenium.support.ui.WebDriverWait;
public class DynamicElementExample {
public static void main(String[] args) {
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
WebDriver driver = new FirefoxDriver();
driver.get("http://www.example.com/dynamic");
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement dynamicElement = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamic-element")));
dynamicElement.click();
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.example.com/dynamic, waits for the 'dynamic-element' to load, clicks on it, and then closes.
In this script, we use a WebDriverWait
instance to wait until the dynamic element becomes visible. Once it’s visible, we click on it.
These are just a few of the common issues you may encounter when using Selenium Java. With a little patience and practice, you’ll be able to overcome these and other challenges in your web application testing journey.
Understanding Selenium Java: The Fundamentals
To effectively use Selenium Java for web application testing, it’s important to understand the fundamental concepts that underpin its operation. Let’s delve into the basics of Selenium, its WebDriver component, and Java.
What is Selenium?
Selenium is a powerful tool for controlling web browsers through the browser’s native support for automation. It’s mainly used for automating web applications for testing purposes, but it’s flexible enough to automate boring web-based administrative tasks.
// Import the WebDriver package
import org.openqa.selenium.WebDriver;
// Import the FirefoxDriver class
import org.openqa.selenium.firefox.FirefoxDriver;
public class SeleniumExample {
public static void main(String[] args) {
// Set the path to the geckodriver executable
System.setProperty("webdriver.gecko.driver", "/path/to/geckodriver");
// Create a new instance of the Firefox driver
WebDriver driver = new FirefoxDriver();
// Use the driver to visit a website
driver.get("http://www.google.com");
// Close the browser
driver.quit();
}
}
# Output:
# The Firefox browser opens, visits www.google.com, and then closes.
In this code block, we import the necessary classes, create a new WebDriver instance, use it to visit Google’s homepage, and then close the browser. This simple example demonstrates how Selenium can control a web browser programmatically.
Exploring WebDriver
WebDriver is a component of Selenium that provides an interface to create and run automation scripts. WebDriver makes direct calls to the browser using each browser’s native support for automation. It supports multiple programming languages, including Java, through bindings.
The Role of Java
Java is a popular programming language known for its ‘Write Once, Run Anywhere’ (WORA) capability. It means a programmer can develop Java software on any device, compile it into a standard bytecode and expect it to run on any device equipped with a Java Virtual Machine (JVM). This makes Java a great language for writing Selenium tests, as these tests can then be run on any platform.
The Importance of Automation Testing
Automation testing is crucial in today’s fast-paced development environments. It allows us to quickly and reliably ensure that our applications are working as expected. This is especially important when making changes to our codebase, as we can run our automated tests to catch any regressions introduced by our changes.
In the context of web applications, automation testing allows us to test our application across multiple browsers and platforms, ensuring that all our users have a consistent experience. Selenium Java, with its powerful automation capabilities, plays a key role in this process.
Selenium Java in the Real World: CI/CD and Agile Development
Selenium Java isn’t just a tool for testing web applications. It’s also a powerful ally in modern development practices such as continuous integration/continuous deployment (CI/CD) and agile development. Let’s explore how Selenium Java fits into these scenarios.
Selenium Java in Continuous Integration/Continuous Deployment
Continuous Integration and Continuous Deployment (CI/CD) is a development practice where developers integrate code into a shared repository frequently, preferably several times a day. Each integration can then be verified by an automated build and automated tests.
Selenium Java plays a crucial role in this process. It allows us to write automated tests that can be run every time code is pushed to the repository. This helps to catch issues early and ensures that our application is always in a deployable state.
Here’s an example of how you might configure a Jenkins job to run your Selenium tests whenever code is pushed to your repository:
# Jenkinsfile
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
# Output:
# The Maven project is built and the tests are run whenever code is pushed to the repository.
In this Jenkinsfile, we define a pipeline with two stages: Build and Test. In the Build stage, we run mvn clean install
to build our Maven project. In the Test stage, we run mvn test
to run our Selenium tests.
Agile Development with Selenium Java
In agile development, requirements and solutions evolve through the collaborative effort of self-organizing and cross-functional teams. Agile advocates adaptive planning, evolutionary development, early delivery, and continual improvement, and it encourages flexible responses to change.
Selenium Java fits perfectly into this methodology. It allows us to write tests that can be easily updated to reflect changing requirements. This helps to ensure that our application always meets the needs of our users, even as those needs change over time.
Exploring Related Concepts: Selenium Grid and Docker
Selenium Java is just the tip of the iceberg when it comes to web application testing. There are many related concepts and tools that you can explore to further enhance your testing capabilities.
For example, Selenium Grid allows you to run your tests on different machines against different browsers in parallel. This can significantly speed up your testing process and help you ensure that your application works correctly on all supported platforms and browsers.
Docker, on the other hand, allows you to package your application and its dependencies into a container, which can then be run on any machine that has Docker installed. This can simplify your testing setup and make it easier to run your tests in different environments.
Further Resources for Selenium Java
To deepen your understanding of Selenium Java and related concepts, you can Click Here to get started with Java web development and unleash its full potential.
Additionally, here are a few resources you might find useful:
- Java Applet Overview – Explore Java Applets, small applications that run within web browsers using Java technology.
Exploring JSP in Java – Discover how JSP simplifies the development of web applications.
Selenium HQ Documentation covers all aspects of Selenium, including WebDriver and Grid.
JUnit Documentation provides a detailed overview of the JUnit testing framework, including installation and writing tests.
Maven Getting Started Guide provides an intro to Maven, including project set up, manage dependencies, and run tests.
Wrapping Up: Selenium Java for Web Application Testing
In this comprehensive guide, we’ve navigated the ins and outs of web application testing using Selenium with Java. From the basics of setting up the environment to writing advanced test scripts, we’ve covered a wide range of topics to help you master this powerful tool.
We embarked on our journey with the basics, learning how to install Java and Selenium WebDriver, and configure an IDE to write and run test scripts. We then delved into more complex scenarios, such as testing form inputs, handling pop-up windows, and testing AJAX-based applications.
Along the way, we explored alternative approaches, introducing additional tools and libraries such as TestNG, JUnit, and Maven. We also addressed common issues you might encounter during testing, such as ‘NoSuchElementException’ and problems with handling dynamic elements, providing solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Use | Simple and easy to start with | Limited functionality |
Advanced Use | Handles complex scenarios | Requires more knowledge |
Alternative Approaches | Provides additional tools and libraries | Requires understanding of additional tools |
Whether you’re just starting out with Selenium Java or you’re looking to enhance your web application testing skills, we hope this guide has been a valuable resource.
Selenium Java is a powerful tool for automating and controlling your testing process, making your web application testing more efficient and effective. Now, you’re well equipped to tackle any web application testing challenge that comes your way. Happy testing!