JSP Uncovered: A Guide to Java Server Pages
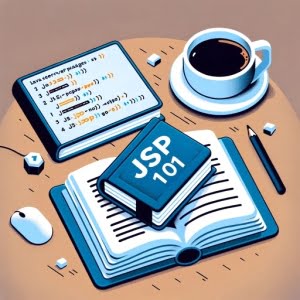
Are you finding it difficult to understand Java Server Pages (JSP)? You’re not alone. Many developers find JSP a bit complex, but it’s a crucial player in server-side web development, much like a backstage director in a theater production.
JSP, or Java Server Pages, is a technology that allows software developers to create dynamic web pages based on HTML, XML, or other types of documents. It’s an integral part of the Java technology family, designed to support application development that includes web pages, web services, and even other types of network applications.
In this guide, we’ll walk you through the basics of JSP, its usage, and advanced techniques. We’ll cover everything from setting up a JSP environment, understanding its syntax, to more advanced topics like JSP directives, scripting elements, and standard actions. We’ll also discuss alternative approaches and troubleshooting common issues.
So, let’s get started and uncover the world of Java Server Pages!
TL;DR: What is JSP?
JSP
, orJava Server Pages
, is a technology used for building web pages that support dynamic content. It leverages the power of the Java programming language and is an integral part of Java’s enterprise edition. Here’s a simple example of a JSP page:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>My First JSP Page</title>
</head>
<body>
<%= "Hello, World!" %>
</body>
</html>
In this example, we’ve created a simple JSP page that displays ‘Hello, World!’ when accessed. The code block is a JSP expression that gets evaluated and inserted into the response page.
This is just a basic introduction to JSP, but there’s much more to learn about creating dynamic web pages, using JSP directives, scripting elements, and standard actions. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Setting Up and Understanding Basic JSP
- Delving Deeper: JSP Directives, Scripting Elements, and Standard Actions
- Exploring Alternatives: Servlets, JSF, and Spring MVC
- Troubleshooting Common JSP Issues
- JSP and MVC Architecture: A Deep Dive
- JSP in Larger Web Development Projects
- Wrapping Up: Mastering JSP for Dynamic Web Development
Setting Up and Understanding Basic JSP
To start working with JSP, we first need to set up a development environment. The most common setup involves installing Java Development Kit (JDK), a server like Apache Tomcat, and an Integrated Development Environment (IDE) like Eclipse or IntelliJ IDEA.
Once you have these installed, you can create a new JSP file in your IDE and start writing your first JSP script. Here’s an example of a basic JSP file:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>My First JSP Page</title>
</head>
<body>
<%= "Hello, JSP!" %>
</body>
</html>
This is a simple JSP page that displays ‘Hello, JSP!’ when accessed. The code block is a JSP expression that gets evaluated and inserted into the response page. The first line, is a JSP directive that provides global information for the JSP page, such as the scripting language used (Java in this case), the content type, and the page encoding.
When you run this JSP page on your server, it gets compiled into a servlet (a Java class), and the server executes this servlet to generate the HTML page that gets sent to the client. This is the fundamental concept behind JSP: it’s a technology that lets you mix regular, static HTML with dynamically generated content from servlets.
Delving Deeper: JSP Directives, Scripting Elements, and Standard Actions
As you become more comfortable with JSP, you’ll start exploring its more advanced features. Among these are JSP directives, scripting elements, and standard actions.
JSP Directives
JSP directives provide instructions to the JSP engine that apply to the entire JSP page. There are three main types of directives: page
, include
, and taglib
.
The page
directive, which we saw in the basic example, is used to define attributes that apply to the entire JSP page, such as the scripting language, the error page, and the content type.
The include
directive is used to include the content of another file, usually another JSP or HTML file, in the current JSP page.
The taglib
directive is used to define a tag library that will be used in the JSP page. Tag libraries provide a way to define custom JSP tags.
Scripting Elements
JSP scripting elements let you insert Java code into your JSP pages. There are three types of scripting elements: expressions
, scriptlets
, and declarations
.
Expressions
are used to output the value of a Java expression, like we saw in the basic example.
Scriptlets
are used to insert Java code that will be executed each time the page is requested. Here’s an example:
<% int x = 10; int y = 20; int sum = x + y; %>
Sum: <%= sum %>
In this example, we define two integers, x
and y
, and calculate their sum. We then use an expression to output the sum. When you run this JSP page, you’ll see ‘Sum: 30’ displayed.
Declarations
are used to declare variables or methods that will be used later in the script. Here’s an example:
<%! int counter = 0; %>
<% counter++; %>
Counter: <%= counter %>
In this example, we declare a counter variable using a declaration, increment it using a scriptlet, and then output its value using an expression. When you run this JSP page, you’ll see ‘Counter: 1’ displayed.
Standard Actions
JSP standard actions provide functionality that can be reused in JSP pages, such as including other resources, forwarding the request to another resource, or generating HTML for a JavaBean property. Here’s an example of using the jsp:include
action to include another JSP page:
<jsp:include page="header.jsp" />
In this example, the content of header.jsp
will be included in the current JSP page each time it’s requested.
Exploring Alternatives: Servlets, JSF, and Spring MVC
While JSP is a powerful technology for building dynamic web pages, it’s not the only tool in the Java ecosystem. There are other related technologies, such as Servlets, JavaServer Faces (JSF), and Spring MVC that can accomplish the same task. Each of these technologies has its own strengths and weaknesses, and understanding these can help you make informed decisions about which tool to use for your specific needs.
Servlets
Servlets are Java classes that are run on a server to produce dynamic content. In fact, JSP pages are actually compiled into Servlets behind the scenes. Here’s an example of a simple Servlet that generates a ‘Hello, World!’ page:
import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class HelloWorldServlet extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response)
throws IOException, ServletException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html>");
out.println("<head>");
out.println("<title>Hello World!</title>");
out.println("</head>");
out.println("<body>");
out.println("<h1>Hello, World!</h1>");
out.println("</body>");
out.println("</html>");
}
}
# Output:
# 'Hello, World!'
In this example, we’re extending the HttpServlet
class and overriding the doGet
method to generate a simple HTML page. While Servlets give you a lot of control, they can also be more verbose and harder to maintain than JSP pages.
JavaServer Faces (JSF)
JSF is a Java web application framework that simplifies the development of user interfaces for Java EE applications. It provides a set of reusable UI components, a standard for web application navigation, state management, and more. Here’s an example of a JSF page that displays a ‘Hello, World!’ message:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html">
<h:head>
<title>Hello World!</title>
</h:head>
<h:body>
<h:outputText value="Hello, World!" />
</h:body>
</html>
# Output:
# 'Hello, World!'
In this example, we’re using the h:outputText
tag to output a ‘Hello, World!’ message. JSF provides a higher level of abstraction than Servlets or JSP, but it also has a steeper learning curve.
Spring MVC
Spring MVC is a part of the larger Spring Framework, which is widely used in enterprise Java development. Spring MVC provides a model-view-controller architecture that can be used to build flexible and loosely coupled web applications. Here’s an example of a Spring MVC controller that returns a ‘Hello, World!’ view:
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloWorldController {
@GetMapping("/hello")
public String hello(Model model) {
model.addAttribute("message", "Hello, World!");
return "hello";
}
}
# Output:
# 'Hello, World!'
In this example, we’re defining a controller with a hello
method that adds a ‘Hello, World!’ message to the model and returns a ‘hello’ view. Spring MVC provides a lot of flexibility and integrates well with other parts of the Spring Framework, but it can also be overkill for simpler applications.
In conclusion, while JSP is a powerful tool for building dynamic web pages, it’s not the only option. Depending on your needs, Servlets, JSF, or Spring MVC might be a better fit. Understanding the strengths and weaknesses of each of these technologies can help you make the best decision for your specific situation.
Troubleshooting Common JSP Issues
Like any technology, JSP has its fair share of common issues and obstacles. Here, we’ll discuss some of these problems, their solutions, and some best practices for optimizing your JSP code.
Error: Unable to Compile Class for JSP
One common error when working with JSP is the ‘Unable to compile class for JSP’ error. This typically happens when there’s a syntax error in your JSP code. For example:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="ISO-8859-1">
<title>My First JSP Page</title>
</head>
<body>
<%= "Hello, JSP!"
</body>
</html>
In this example, we forgot to close the JSP expression with %>
. This will cause the JSP compiler to throw an error. The solution is to fix the syntax error by closing the expression:
<%= "Hello, JSP!" %>
Error: Package javax.servlet does not exist
Another common error is the ‘package javax.servlet does not exist’ error. This happens when the Servlet API is not available to your JSP page or application. The solution is to make sure the Servlet API is included in your application’s classpath. If you’re using a build tool like Maven, you can add the Servlet API as a dependency in your pom.xml
file.
Best Practices and Optimization
When working with JSP, there are several best practices you can follow to optimize your code and avoid common pitfalls.
- Avoid Java code in JSP: While it’s possible to embed Java code in your JSP pages using scriptlets, it’s generally a bad practice. It makes your code harder to read and maintain, and it can lead to issues like spaghetti code. Instead, use JSP EL (Expression Language) and JSTL (JSP Standard Tag Library) to handle dynamic content in your JSP pages.
Use MVC architecture: JSP is best used as the view layer in an MVC (Model-View-Controller) architecture. This keeps your JSP pages focused on presentation logic, while the controllers handle the business logic and the models represent your data.
Precompile your JSP pages: By default, JSP pages are compiled on-the-fly when they’re requested for the first time. This can lead to a delay for the first user who accesses the page. To avoid this, you can precompile your JSP pages when your application starts up.
By keeping these considerations in mind, you can avoid common issues and write clean, efficient JSP code.
JSP and MVC Architecture: A Deep Dive
Java Server Pages (JSP) is a technology that forms an integral part of the Java EE web application model. To fully understand its role and how it operates, it’s essential to dive into the theory and fundamental concepts that underpin its functioning.
JSP in MVC Architecture
One of the key concepts in web application development is the Model-View-Controller (MVC) architecture. In this design pattern, an application is divided into three interconnected components:
- The Model represents the application’s data and business logic.
- The View is the visual representation of the model – essentially, the user interface.
- The Controller manages the interactions between the Model and the View.
In the context of MVC, JSP is typically used as the View component. It’s responsible for displaying the data to the user and gathering user input. The JSP pages interact with Java Servlets, which act as Controllers, managing the flow of data between the Model (usually represented by JavaBeans) and the View (JSP).
JSP and Servlet Interaction
JSP and Servlets work hand in hand in a Java EE web application. When a client sends a request to the server, it’s usually received by a Servlet. The Servlet then processes the request, interacts with the Model to retrieve or update data, and decides which JSP page to display the result to the user.
Here’s a simple example of a Servlet forwarding a request to a JSP page:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.IOException;
public class HelloWorldServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setAttribute("message", "Hello, World!");
request.getRequestDispatcher("/WEB-INF/hello.jsp").forward(request, response);
}
}
# Output:
# 'Hello, World!'
In this example, the Servlet sets an attribute message
in the request with the value ‘Hello, World!’. It then forwards the request to hello.jsp
. The JSP page can then access the message
attribute and display its value to the user.
In conclusion, understanding the role of JSP in the MVC architecture and its interaction with Servlets is crucial for effective Java EE web application development. It allows for a clear separation of concerns, with JSP handling the presentation and Servlets managing the control flow.
JSP in Larger Web Development Projects
In larger web development projects, JSP often plays a crucial role in creating dynamic, user-centric websites. It’s not uncommon to see JSP used in conjunction with other technologies such as JavaScript, CSS, and HTML to create more interactive and visually appealing websites.
For instance, you might use JSP to dynamically generate HTML content based on user input or database queries, while JavaScript is used to handle user interactions like clicks or form submissions. CSS can then be used to style the generated HTML content, creating a more engaging user experience.
Accompanying Technologies in Typical JSP Use Cases
JSP is often used in conjunction with a range of other technologies. For instance, in a typical Java EE web application, you might see JSP used alongside Servlets, JavaBeans, and a database system like MySQL or PostgreSQL. In this setup, JSP and Servlets handle the web interface, JavaBeans encapsulate the business logic, and the database system stores the data.
In more modern applications, you might see JSP used with Spring MVC or JSF, which provide a more robust framework for building web applications. These frameworks offer a range of features that can simplify and streamline the development process, such as form handling, data binding, and validation.
Further Resources for Mastering JSP
To deepen your understanding of JSP and related technologies, here are some resources that provide more in-depth information:
- Java Web Development: A Quick Overview – Dive deep into Java servlets and JSP for web development projects.
Exploring Selenium in Java – Explore how Selenium WebDriver allows developers to write online applications in Java.
JSF Overview – Learn about JavaServer Faces (JSF), a Java EE framework for building component-based user interfaces.
Oracle’s official JSP documentation offers a comprehensive usage guide to JSP.
Java EE tutorial from Oracle covers JSP as well as other Java EE technologies like Servlets, EJBs, and JSF.
Spring MVC guide offers a good introduction to the Spring MVC Framework and its features.
Remember, mastering JSP and related technologies requires practice. Don’t be afraid to experiment, make mistakes, and learn from them. Happy coding!
Wrapping Up: Mastering JSP for Dynamic Web Development
In this comprehensive guide, we’ve explored the depths of Java Server Pages (JSP), a robust technology that plays a pivotal role in server-side web development.
We embarked on our journey with the basics, learning how to set up a JSP environment and create our first JSP file. We then delved into more advanced concepts, such as JSP directives, scripting elements, and standard actions, giving you a deeper understanding of how to create dynamic web content with JSP.
Along the way, we tackled common challenges you might encounter when using JSP, such as ‘Unable to compile class for JSP’ and ‘Package javax.servlet does not exist’ errors, providing you with solutions and best practices to overcome these hurdles.
We also looked at alternative approaches to JSP, comparing it with other Java technologies like Servlets, JavaServer Faces (JSF), and Spring MVC. Here’s a quick comparison of these technologies:
Technology | Versatility | Learning Curve | Use Cases |
---|---|---|---|
JSP | High | Moderate | Dynamic web pages, small to medium applications |
Servlets | High | High | Dynamic web content, large applications |
JSF | High | High | User interfaces for Java EE applications |
Spring MVC | High | High | Flexible and loosely coupled web applications |
Whether you’re just starting out with JSP or looking to deepen your server-side web development skills, we hope this guide has given you a comprehensive understanding of JSP and its capabilities.
With its versatility and wide usage in the Java ecosystem, JSP is a powerful tool for any web developer. Now you’re well-equipped to harness its power. Happy coding!