Dive Into Java Web Development: A Detailed Walkthrough
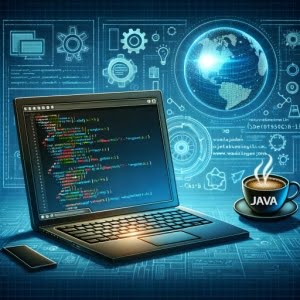
Are you ready to dive into the world of Java web development? Like a master builder, Java offers a robust set of tools for constructing sophisticated web applications. Whether you’re a novice programmer or a seasoned developer looking to add another skill to your repertoire, Java web development is a worthwhile venture.
Java, a versatile and popular programming language, is known for its efficiency and interoperability. It’s a language that can be as powerful as you need it to be, capable of handling complex web development tasks with ease.
This guide will walk you through the basics of web development using Java, covering everything from the essential tools to creating your first web application. We’ll delve into the core aspects of Java web development, explore its advanced features, and even discuss common issues and their solutions.
So, let’s embark on this exciting journey into Java web development!
TL;DR: How Do I Start with Java Web Development?
To start with Java web development, you need to understand the basics of Java, then learn about web technologies like
Servlets, JSPs, and Java frameworks like Spring and Hibernate
. Here’s a simple example of aJava Servlet
:
@WebServlet(name = 'hello', urlPatterns = {'/hello'})
public class HelloServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.getWriter().write('Hello, World!');
}
}
In this example, we define a simple Servlet that responds with ‘Hello, World!’ when accessed. The @WebServlet
annotation is used to declare the Servlet, and the doGet
method is overridden to define the response.
This is just the tip of the iceberg when it comes to Java web development. Stay tuned for a deeper dive into these concepts and more.
Table of Contents
- Java Web Development Basics
- Delving Deeper: Java Frameworks and RESTful APIs
- Exploring Alternative Approaches in Java Web Development
- Overcoming Hurdles: Troubleshooting in Java Web Development
- Understanding Web Development Principles and Java’s Role
- Java Web Development in the Real World
- Enhancing Your Java Web Development Skills
- Wrapping Up: Mastering Java Web Development
Java Web Development Basics
Before venturing into the specifics of Java web development, it’s crucial to have a solid grounding in the basics. This includes understanding Java itself and web technologies like HTML, CSS, and JavaScript.
Java Fundamentals
Java is a statically-typed, object-oriented programming language that’s known for its ‘write once, run anywhere’ philosophy. This means that Java code can run on any device that has a Java Virtual Machine (JVM).
Here’s a simple Java program that prints ‘Hello, World!’ to the console:
public class Main {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
// Output:
// Hello, World!
In this example, Main
is a public class that contains the application’s entry point, the main
method. The System.out.println
command is used to print the message to the console.
HTML, CSS, and JavaScript
HTML (HyperText Markup Language), CSS (Cascading Style Sheets), and JavaScript form the cornerstone of web development. HTML is used to structure web content, CSS is used for styling, and JavaScript is used to add interactivity.
Servlets and JSPs
Servlets and JavaServer Pages (JSPs) are key components of Java web development. Servlets are Java classes that handle requests and responses in a web application, while JSPs allow you to embed Java code in HTML.
Here’s an example of a simple web application using these technologies:
// HelloServlet.java
@WebServlet('/hello')
public class HelloServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setAttribute('message', 'Hello, World!');
request.getRequestDispatcher('/hello.jsp').forward(request, response);
}
}
// hello.jsp
<html>
<body>
<h1>${message}</h1>
</body>
</html>
In this example, the HelloServlet
class handles GET requests to the ‘/hello’ URL. It sets an attribute ‘message’ on the request and forwards the request to ‘hello.jsp’. The JSP file then accesses this attribute and displays its value in an HTML heading.
Delving Deeper: Java Frameworks and RESTful APIs
Once you’ve grasped the basics of Java web development, it’s time to explore more advanced topics. This includes Java frameworks like Spring and Hibernate, RESTful APIs, and database connectivity.
Java Frameworks: Spring and Hibernate
Java frameworks simplify the development process by providing a structured way to build applications. Spring is a comprehensive framework for developing enterprise-level Java applications. It offers features like dependency injection, transaction management, and more.
Hibernate, on the other hand, is an Object-Relational Mapping (ORM) framework. It simplifies database operations by mapping Java objects to database tables.
Here’s a simple example of a Spring application that uses Hibernate to interact with a database:
// Main.java
@SpringBootApplication
public class Main {
public static void main(String[] args) {
SpringApplication.run(Main.class, args);
}
}
// Greeting.java
@Entity
public class Greeting {
@Id
@GeneratedValue
private Long id;
private String message;
// getters and setters omitted for brevity
}
// GreetingRepository.java
public interface GreetingRepository extends JpaRepository<Greeting, Long> {
}
// GreetingController.java
@RestController
public class GreetingController {
@Autowired
private GreetingRepository greetingRepository;
@GetMapping('/greetings')
public List<Greeting> getGreetings() {
return greetingRepository.findAll();
}
}
In this example, Main
is the entry point of the application, Greeting
is an entity that corresponds to a database table, GreetingRepository
is a repository that provides database operations for Greeting
, and GreetingController
is a controller that handles HTTP requests.
RESTful APIs and Database Connectivity
RESTful APIs (Application Programming Interfaces) are a way to interact with a server. They use HTTP methods (GET, POST, PUT, DELETE) to perform operations. In the above example, the GreetingController
provides a RESTful API that allows clients to retrieve all greetings from the database.
Database connectivity in Java web development is often handled by JDBC (Java Database Connectivity) or JPA (Java Persistence API). These technologies allow Java applications to interact with relational databases. In the above example, Spring Data JPA, which is a part of the Spring framework, is used for database operations.
Exploring Alternative Approaches in Java Web Development
Java web development is not a one-size-fits-all field. There are multiple approaches and tools you can use depending on your project’s needs. Let’s explore some of these alternatives.
Different Frameworks: Struts and JSF
While Spring is a popular choice for many developers, other frameworks like Struts and JavaServer Faces (JSF) also have their strengths. Struts is a flexible, action-based framework that’s well-suited for large projects. JSF, on the other hand, is a component-based framework that simplifies UI development.
// Struts example
public class HelloWorldAction extends Action {
public ActionForward execute(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception {
HelloWorldForm helloWorldForm = (HelloWorldForm) form;
helloWorldForm.setMessage('Hello, World!');
return mapping.findForward('success');
}
}
// JSF example
@ManagedBean
public class HelloWorld {
private String message = 'Hello, World!';
// getters and setters omitted for brevity
}
In the Struts example, HelloWorldAction
is an action that sets a message on a form and returns a forward named ‘success’. In the JSF example, HelloWorld
is a managed bean that holds a message.
Microservices with Spring Boot
Microservices architecture is a design pattern where an application is made up of small, independently deployable services. Spring Boot, a project built on top of the Spring framework, makes it easy to create stand-alone, production-grade Spring applications that you can ‘just run’, making it a good fit for building microservices.
// Spring Boot application
@SpringBootApplication
public class HelloWorldApplication {
public static void main(String[] args) {
SpringApplication.run(HelloWorldApplication.class, args);
}
}
In this example, HelloWorldApplication
is a Spring Boot application. The @SpringBootApplication
annotation indicates that it’s a Spring context file and the main method delegates to Spring Boot’s SpringApplication
class.
Reactive Programming with Spring WebFlux
Reactive programming is a programming paradigm that deals with asynchronous data streams. Spring WebFlux is a reactive web framework that’s part of Spring 5+. It’s designed to fully utilize the computing resources available, making it a good choice for high-load applications.
// Spring WebFlux example
@RestController
public class HelloWorldController {
@GetMapping('/hello')
public Mono<String> hello() {
return Mono.just('Hello, World!');
}
}
In this example, HelloWorldController
is a controller that handles GET requests to the ‘/hello’ URL and returns a Mono
, a type that represents a sequence of zero or one items.
Choosing the right approach depends on a variety of factors, including the size and complexity of your project, your team’s expertise, and specific requirements like performance and scalability. Always consider the benefits and drawbacks of each option before making a decision.
Overcoming Hurdles: Troubleshooting in Java Web Development
Like any other field, Java web development comes with its own set of challenges. Let’s discuss some common errors and provide solutions to help you navigate these hurdles.
Dealing with Common Errors
One of the most common errors in Java web development is a NullPointerException
. This occurs when you try to use a null object reference. For instance:
public class Main {
public static void main(String[] args) {
String str = null;
System.out.println(str.length());
}
}
// Output:
// Exception in thread 'main' java.lang.NullPointerException
In this example, we attempt to call the length
method on a null string, resulting in a NullPointerException
. To avoid this, always check if an object is null before using it.
public class Main {
public static void main(String[] args) {
String str = null;
if (str != null) {
System.out.println(str.length());
}
}
}
// Output:
// (no output)
Best Practices and Optimization
To ensure your Java web applications run smoothly, follow best practices and consider optimization strategies. For example, always close database connections and other resources when you’re done using them to free up system resources. Use caching to improve performance, but be mindful of memory usage.
Also, consider using a profiler to identify bottlenecks in your application. This can help you focus your optimization efforts where they’ll have the most impact.
// Using a try-with-resources statement to close a database connection
try (Connection conn = DriverManager.getConnection(dbUrl, username, password)) {
// Use the connection
} catch (SQLException e) {
// Handle exception
}
In this example, we use a try-with-resources statement to automatically close a database connection when we’re done using it.
Remember, the key to successful Java web development is understanding the tools and technologies at your disposal, learning from your mistakes, and always striving to improve your skills and knowledge.
Understanding Web Development Principles and Java’s Role
To truly excel in Java web development, it’s important to understand the underlying principles of web development and how Java fits into this context.
The Principles of Web Development
Web development is all about creating and maintaining websites or web applications. It involves a variety of tasks, including designing user interfaces, developing server-side components, managing databases, and optimizing for performance and scalability. Web development can be broadly divided into two areas: front-end (client-side) and back-end (server-side).
# Front-end technologies
HTML, CSS, JavaScript
# Back-end technologies
Java, Python, Ruby, PHP, .NET
As shown above, front-end technologies include HTML, CSS, and JavaScript, while back-end technologies include languages like Java, Python, Ruby, PHP, and .NET.
Java’s Role in Server-Side Programming
Java plays a crucial role in server-side programming. With its robust set of libraries and frameworks, Java allows developers to create dynamic, data-driven web applications. It’s used in a variety of contexts, from building small business websites to developing complex enterprise-level applications.
// A simple server-side Java program using Servlet
@WebServlet('/hello')
public class HelloServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.getWriter().write('Hello, World!');
}
}
In the code example above, we define a simple Servlet that responds with ‘Hello, World!’ when accessed. This is a basic example of server-side programming with Java.
The Java Ecosystem and the Evolution of Java Web Technologies
The Java ecosystem is vast and includes a variety of tools and technologies for web development. This includes Servlets and JSPs, as well as frameworks like Spring and Hibernate. Over the years, Java web technologies have evolved to become more powerful and easier to use. For instance, the introduction of annotations in Java 5 has simplified the configuration of web applications, and the Spring framework has made it easier to develop enterprise-level applications.
Java’s extensive ecosystem and continual evolution make it a powerful choice for web development. By understanding these fundamentals, you can leverage Java’s capabilities to create effective and efficient web applications.
Java Web Development in the Real World
Java web development is not just about learning the syntax or understanding the frameworks. It’s about applying these skills to solve real-world problems. Let’s explore some of these applications.
Building E-commerce Platforms
Java’s robustness and scalability make it a popular choice for building e-commerce platforms. These platforms require handling multiple user requests simultaneously, secure payment processing, and efficient data management—all of which Java can handle with ease.
// A simple e-commerce platform might have a Product class like this
public class Product {
private String name;
private double price;
// getters and setters omitted for brevity
}
In this example, we define a simple Product
class that could be part of an e-commerce platform. This is a basic example, and real-world e-commerce platforms would be much more complex.
Creating Content Management Systems
Content Management Systems (CMS) are another common use case for Java web development. A CMS allows users to manage and publish content on the web without needing to know how to code. Java’s versatility and the availability of powerful frameworks make it well-suited for such tasks.
Developing Social Networking Sites
Java is also used to develop social networking sites. These sites require handling large amounts of data, real-time updates, and millions of users, making Java’s performance and scalability crucial.
Enhancing Your Java Web Development Skills
While understanding the basics of Java web development is essential, there’s always more to learn. You might want to explore related topics like Java security, performance tuning, and deployment. These topics can help you write more secure, efficient, and production-ready code.
Further Resources for Java Web Development Mastery
To dive deeper into Java web development, consider exploring the following resources:
- Java Applications Overview – Discover Java’s versatile applications, from web development to mobile apps.
JSP Overview – Explore JavaServer Pages (JSP), a technology for developing dynamic web pages using Java.
Oracle’s Official Tutorials on Java cover a wide range of basics to advanced Java topics.
Baeldung’s Spring Guides offer in-depth information about the Spring framework for Java web development.
JavaBrains’ Java Tutorials covers a variety of Java topics, including web development, Spring, and Hibernate.
Wrapping Up: Mastering Java Web Development
In this comprehensive guide, we’ve navigated the vast ocean of Java web development, a powerful tool for creating dynamic, data-driven web applications.
We embarked on this journey with the basics, understanding the fundamentals of Java and web technologies like HTML, CSS, JavaScript, Servlets, and JSPs. We then dived deeper, exploring advanced topics like Java frameworks such as Spring and Hibernate, RESTful APIs, and database connectivity.
Our expedition didn’t stop there. We ventured into the territories of alternative approaches in Java web development, exploring different frameworks like Struts and JSF, and modern paradigms like microservices with Spring Boot and reactive programming with Spring WebFlux.
Along our journey, we confronted common challenges that developers often face in Java web development, providing solutions and best practices to overcome these hurdles. We also delved into the principles of web development and how Java fits into this landscape, offering a deeper understanding of the Java ecosystem and the evolution of Java web technologies.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Spring | Comprehensive, robust, widely used | Steeper learning curve |
Hibernate | Simplifies database operations | Requires understanding of ORM concepts |
Struts | Flexible, suitable for large projects | Less popular than Spring |
JSF | Simplifies UI development | Not as flexible as action-based frameworks |
Spring Boot | Simplifies microservices development | Might be overkill for simple applications |
Spring WebFlux | Enables reactive programming | Requires understanding of reactive concepts |
Whether you’re just starting out with Java web development or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the landscape and boosted your confidence to tackle your next project.
With its versatility and robustness, Java is a powerful tool for web development. Now, you’re well equipped to navigate this vast ocean. Happy coding!