Java Usage Guide: What is Java Used For?
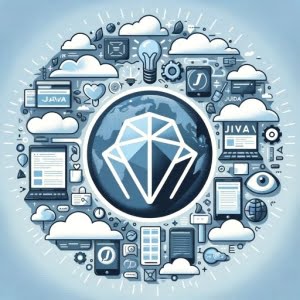
Are you curious about what Java is used for? You’re not alone. Many developers and tech enthusiasts often wonder about the applications of this popular programming language. Think of Java as a Swiss Army knife in the world of programming, with a multitude of uses that extend far beyond the basics.
Java is a versatile tool, used for everything from web development to software testing. Its wide-ranging applications make it a valuable asset for any developer’s toolkit.
In this guide, we will explore the many applications of Java, starting with the basics and moving into more advanced uses. We’ll delve into its role in web development, mobile app development, software testing, and big data analytics, among others.
So, let’s dive in and start exploring the many uses of Java!
TL;DR: What is Java Used For?
Java is a general-purpose programming language that is used for a wide range of applications. It’s used for web development, mobile app development, software testing, and big data analytics. For instance, to create a simple ‘Hello, World!’ program in Java, you would use the following code:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve created a basic Java program that prints ‘Hello, World!’ to the console. This is a simple illustration of Java’s use in software development.
But Java’s capabilities extend far beyond this. Continue reading for a more detailed exploration of the many uses of Java.
Table of Contents
- Java in Web Development
- Java in Mobile App Development
- Java in Software Testing
- Java in Big Data Analytics
- Exploring Alternatives to Java
- Overcoming Challenges in Java Applications
- Understanding Programming Languages and Java’s Role
- Java’s Unique Features
- The Future of Java and Emerging Uses
- Wrapping Up: Unveiling the Versatility of Java
Java in Web Development
Java plays a crucial role in web development, particularly in server-side scripting. Server-side scripting is a technique used in web development which involves running scripts on a web server to produce a response customized for each user’s request. Java’s robustness and scalability make it a popular choice for this purpose.
For instance, to create a simple server-side script in Java, you might use Java Servlets. A Servlet is a Java class used to extend the capabilities of servers that host applications accessed by means of a request-response model. Here’s an example of a simple Servlet that displays ‘Hello, World!’ in your web browser:
import javax.servlet.*;
import javax.servlet.http.*;
import java.io.*;
public class HelloWorld extends HttpServlet {
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
PrintWriter out = response.getWriter();
out.println("Hello, World!");
}
}
In this example, we’ve created a basic Java Servlet that, when deployed on a server and accessed via a web browser, would display ‘Hello, World!’.
Java is also used to build dynamic web pages. Dynamic web pages can change their content or appearance depending on the user’s interactions, the time of day, or other variables. JavaServer Pages (JSP) is a technology used for developing dynamic web content.
Java in Mobile App Development
Java is also widely used in mobile app development, particularly for Android apps. Android’s default programming language is Java, and most of the apps in the Google Play Store are built with Java.
For instance, to create a simple Android app that displays ‘Hello, World!’, you might write something like this:
package com.example.helloworld;
import android.os.Bundle;
import android.widget.TextView;
import android.app.Activity;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
TextView textView = new TextView(this);
textView.setText("Hello, World!");
setContentView(textView);
}
}
In this example, we’ve created a basic Android activity that, when launched, displays ‘Hello, World!’ on the screen. This illustrates Java’s role in mobile app development, specifically for Android.
Java in Software Testing
Java is a key player in software testing, providing the backbone for many automated testing tools like Selenium. Selenium is a popular open-source web-based automation tool. It provides a single interface for writing test scripts in several programming languages, including Java.
For instance, to write a simple Selenium test in Java to open a browser and navigate to a website, you might write something like this:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
public class SeleniumTest {
public static void main(String[] args) {
WebDriver driver = new FirefoxDriver();
driver.get("http://www.google.com");
driver.quit();
}
}
In this example, we’ve created a basic Selenium test that opens a Firefox browser, navigates to Google’s homepage, and then closes the browser. This illustrates Java’s role in automated software testing.
Java in Big Data Analytics
Java is also extensively used in big data analytics, particularly with tools like Hadoop. Hadoop is an open-source software framework written in Java that allows for the distributed processing of large data sets across clusters of computers.
For instance, to write a simple Hadoop MapReduce program in Java to count the frequency of words in a text file, you might write something like this:
import java.io.IOException;
import org.apache.hadoop.io.*;
import org.apache.hadoop.mapreduce.*;
public class WordCount {
public static class Map extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] words = value.toString().split(" ");
for (String str : words) {
word.set(str);
context.write(word, one);
}
}
}
public static class Reduce extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
}
In this example, we’ve created a basic Hadoop MapReduce program that counts the frequency of each word in a text file. The Map
class maps each word to the number 1, and the Reduce
class sums up these mapped values for each word. This illustrates Java’s role in big data analytics.
Exploring Alternatives to Java
While Java is a powerful and versatile language, it’s not the only tool in the shed. There are other programming languages that can be used for similar applications. Let’s take a look at some of these alternatives and compare their strengths and weaknesses to Java.
Python for Web Development
Python is another popular choice for web development, particularly for its readability and simplicity. Django and Flask are two widely-used Python frameworks for web development. Here’s a simple Flask app that displays ‘Hello, World!’:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
# Output:
# * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
# Hello, World!
This example demonstrates the simplicity and readability of Python. However, Python’s performance can be slower compared to Java, which might be a consideration for more resource-intensive web applications.
Swift for Mobile App Development
Swift is the main language used for iOS app development. Swift is known for its speed, safety features, and modern syntax. Here’s a simple Swift program that prints ‘Hello, World!’:
import Swift
print("Hello, World!")
# Output:
# Hello, World!
This example shows the simplicity of Swift. However, Swift is mainly used for iOS app development and isn’t as versatile as Java, which can be used for both Android and iOS app development through cross-platform frameworks like React Native.
In conclusion, while Java is a versatile and powerful language, other languages like Python and Swift can also be used for similar applications. The choice of language will depend on the specific needs and constraints of your project.
Overcoming Challenges in Java Applications
While Java is a versatile and widely-used language, developers may encounter certain challenges when using it for web development, mobile app development, software testing, and big data analytics. Let’s discuss some of these common challenges and how to overcome them.
Dealing with Performance Issues
Java applications can sometimes suffer from performance issues, such as slow execution time or high memory usage. These can be caused by various factors, such as inefficient code or inappropriate use of Java’s features.
For instance, using the ‘‘ operator to compare strings in Java can lead to unexpected results, because it compares the memory addresses of the strings, not their contents. Instead, the ‘equals()’ method should be used. Here’s an example:
String str1 = new String("Hello");
String str2 = new String("Hello");
System.out.println(str1 == str2); // false
System.out.println(str1.equals(str2)); // true
# Output:
# false
# true
In this example, ‘‘ returns false because str1 and str2 point to different memory addresses, even though their contents are the same. On the other hand, ‘equals()’ returns true because it correctly compares the contents of the strings.
Resolving Compatibility Problems
Java’s ‘write once, run anywhere’ principle means that Java code should run consistently on any platform that has a Java Runtime Environment (JRE). However, in practice, developers might encounter compatibility problems due to differences in JRE implementations or versions.
One way to mitigate these problems is to stick to the Java Standard Edition (SE) APIs as much as possible, as these are more likely to be consistent across different JREs. Additionally, testing your application on all target platforms can help catch and resolve compatibility problems early on.
In conclusion, while Java is a powerful tool for various applications, developers should be aware of these common challenges and strategies to overcome them. This knowledge can help you write more efficient and compatible Java code.
Understanding Programming Languages and Java’s Role
A programming language is a set of instructions, commands, and syntax used to create software programs. It’s a tool that developers use to instruct a computer to perform specific tasks.
Java, in particular, is a high-level, object-oriented programming language. It was designed to have the ‘write once, run anywhere’ (WORA) capability, which means that compiled Java code can run on all platforms that support Java without the need for recompilation. This makes Java a highly portable language.
Here’s a simple Java program that prints ‘Hello, World!’:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve created a basic Java program that prints ‘Hello, World!’ to the console. This is a simple illustration of Java’s use in software development.
Java’s Unique Features
Java has several features that make it suitable for various uses, including:
- Platform Independence: Java code can run on any device that has a Java Runtime Environment (JRE), making it highly portable.
Object-Oriented Programming (OOP): Java uses the OOP paradigm, which allows for the creation of modular programs and reusable code.
Robustness: Java has strong memory management and automatic garbage collection, which helps prevent errors and crashes.
Security: Java was designed with security in mind, featuring a secure runtime environment and various security features like bytecode verification.
Multithreading: Java supports multithreading, which allows for the execution of multiple parts of a program simultaneously for maximum CPU utilization.
These features make Java a versatile tool for a wide range of applications, from web development to mobile app development, software testing, and big data analytics.
The Future of Java and Emerging Uses
Java has been around for over two decades, but its journey is far from over. With continuous updates and a large active community, Java is constantly evolving to meet the needs of modern development.
Java and the Internet of Things (IoT)
One area where Java is seeing increased use is in the Internet of Things (IoT). IoT devices often need to be able to run reliably for long periods without human intervention, and Java’s robustness and security features make it a good fit for this. Moreover, Java’s platform independence means that IoT devices running Java can easily interact with each other, regardless of the specific hardware they’re running on.
Java and Cloud Computing
Cloud computing is another area where Java is playing a significant role. Java’s platform independence is a major advantage in the cloud, where applications often need to run on a variety of different hardware and operating systems. In addition, Java’s robustness and security features are particularly important in the cloud, where applications need to be reliable and secure.
Further Resources for Exploring Java Uses
To learn more about the many uses of Java, check out these resources:
- Java Syntax: Basics – Understand the syntax rules and conventions of the Java programming language.
Core Java Basics – Learn fundamental principles and techniques for Java development.
Oracle’s Java Tutorials – These official tutorials from Oracle cover a wide range of topics, from basics to advanced.
Coderanch – A great place to learn from other Java Programmer’s experiences, ask questions, and get advice from experts.
Baeldung offers a mix of free and premium content that focus on Java, Spring, and related backend technologies.
Wrapping Up: Unveiling the Versatility of Java
In this comprehensive guide, we’ve journeyed through the multifaceted world of Java, a robust and versatile programming language used in various fields of technology.
We began with the essentials, understanding what Java is used for, and exploring its basic uses in web development and mobile app development. We then delved deeper into its advanced applications in software testing and big data analytics. We also looked at alternative programming languages like Python and Swift, comparing their strengths and weaknesses to Java.
Along the way, we addressed common challenges that developers might encounter when using Java, such as performance issues and compatibility problems, providing solutions and strategies to overcome these obstacles.
Here’s a quick comparison of Java and its alternatives:
Language | Versatility | Performance | Ease of Use |
---|---|---|---|
Java | High | High | Moderate |
Python | Moderate | Moderate | High |
Swift | Low | High | Moderate |
Whether you’re just starting out with Java or you’re looking to level up your programming skills, we hope this guide has given you a deeper understanding of Java and its capabilities.
Java’s versatility and robustness make it a powerful tool in the world of programming. With its wide range of applications, from web development to big data analytics, Java continues to be a valuable asset for developers. Happy coding!