Java Array Methods Explained: Your Array Utilities Guide
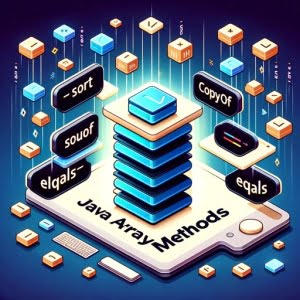
Are you finding it challenging to handle Java array methods? You’re not alone. Many developers find themselves grappling with these tools, but with a little guidance, you can master them.
Think of Java array methods as a toolbox, each method a different tool designed to manipulate arrays in unique ways. These methods are powerful and versatile, allowing you to perform various operations on arrays with ease.
This guide will walk you through the most commonly used Java array methods, from the basics to more advanced techniques. We’ll cover everything from simple methods like Arrays.sort()
, Arrays.fill()
, and Arrays.toString()
, to more complex ones like Arrays.copyOf()
, Arrays.equals()
, and Arrays.binarySearch()
. We’ll also delve into alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering Java array methods!
TL;DR: How Do I Use Array Methods in Java?
Java provides several methods within the
java.util.Arrays
class, includingArrays.sort()
,Arrays.copyOf()
,Arrays.equals()
, and more.They are static, meaning they can be called directly from the class itself, and are used to manipulate arrays.
Here’s a simple example:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
String[] fruits = {"Apple", "Cherry", "Banana", "Dragonfruit"};
Arrays.sort(fruits);
for (String fruit : fruits) {
System.out.print(fruit + " ");
}
}
}
// Output:
// `Apple Banana Cherry Dragonfruit `
In this example, we have a string array with the names of various fruits. We use the Arrays.sort()
method to sort the array in alphabetical order. Then, we print out the elements of the array, which are now in sorted order.
This is just a basic way to use Java array methods, but there’s much more to learn about manipulating arrays in Java. Continue reading for more detailed explanations and examples.
Table of Contents
Basic Array Manipulations in Java
Sorting Arrays with Arrays.sort()
One of the most common operations performed on arrays is sorting. Java provides a built-in method, Arrays.sort()
, which sorts an array into ascending numerical order or alphabetical order for strings.
Here’s an example:
int[] array = {3, 2, 1};
Arrays.sort(array);
for (int i : array) {
System.out.print(i + " ");
}
// Output:
// 1 2 3
In this example, we start with an array in descending order. After applying Arrays.sort()
, our array is rearranged into ascending order.
Filling Arrays with Arrays.fill()
The Arrays.fill()
method is used to fill an entire array with a single value. It can be useful when you want to reset all values in an array or initialize them to a specific value.
Let’s see it in action:
int[] array = new int[5];
Arrays.fill(array, 1);
for (int i : array) {
System.out.print(i + " ");
}
// Output:
// 1 1 1 1 1
In this example, we create an array of five elements and use Arrays.fill()
to fill it with the number 1.
Converting Arrays to Strings with Arrays.toString()
The Arrays.toString()
method is a simple and effective way to convert an entire array into a string format, which is useful for printing or logging purposes.
Here’s how it works:
int[] array = {1, 2, 3};
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3]
In this example, Arrays.toString()
takes an array as input and returns a string representation of the array, including brackets and commas.
Advanced Java Array Methods
Copying Arrays with Arrays.copyOf()
The Arrays.copyOf()
method allows you to create a new array that is a copy of an existing array. This method is useful when you want to manipulate an array without affecting the original data.
Here’s how to use Arrays.copyOf()
:
int[] original = {1, 2, 3};
int[] copy = Arrays.copyOf(original, original.length);
System.out.println(Arrays.toString(copy));
// Output:
// [1, 2, 3]
In this example, we create a copy of the original
array. The second parameter of the Arrays.copyOf()
method determines the length of the new array.
Comparing Arrays with Arrays.equals()
The Arrays.equals()
method is used to check if two arrays are equal, meaning their length, order, and elements are the same.
Let’s see it in action:
int[] array1 = {1, 2, 3};
int[] array2 = {1, 2, 3};
boolean isEqual = Arrays.equals(array1, array2);
System.out.println(isEqual);
// Output:
// true
In this example, Arrays.equals()
returns true
because array1
and array2
are identical.
Searching in Arrays with Arrays.binarySearch()
The Arrays.binarySearch()
method is used to search for a specific element in an array. It uses the binary search algorithm, which is more efficient than a linear search, but requires the array to be sorted first.
Here’s an example:
int[] array = {1, 2, 3, 4, 5};
int index = Arrays.binarySearch(array, 3);
System.out.println(index);
// Output:
// 2
In this example, Arrays.binarySearch()
returns 2
, which is the index of the number 3
in the array.
Exploring Alternative Approaches to Array Manipulation
Using ArrayLists for Dynamic Arrays
While Java arrays are powerful, they have a limitation: their size is fixed at the time of creation. To overcome this, we can use ArrayList
, a resizable array implementation in the Java Collections Framework.
Here’s how you can create an ArrayList and add elements to it:
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
list.add(3);
System.out.println(list);
// Output:
// [1, 2, 3]
In this example, we create an ArrayList
and add three elements to it. Unlike arrays, ArrayLists can grow and shrink dynamically as needed.
Streamlining Array Operations with Java Streams
Java Streams, introduced in Java 8, provide a powerful and flexible way to process data structures, including arrays. With Streams, you can easily perform complex data transformations using functional programming style.
Here’s an example of using a Stream to filter and transform an array:
int[] array = {1, 2, 3, 4, 5};
int[] evenSquares = Arrays.stream(array)
.filter(n -> n % 2 == 0)
.map(n -> n * n)
.toArray();
System.out.println(Arrays.toString(evenSquares));
// Output:
// [4, 16]
In this example, we start with an array of numbers. We create a Stream from the array, filter out the odd numbers, square the remaining even numbers, and collect the results back into a new array. Streams provide a powerful and expressive way to manipulate arrays in Java.
Troubleshooting Common Issues with Java Array Methods
Handling ArrayIndexOutOfBoundsException
One common issue when working with arrays is the ArrayIndexOutOfBoundsException
. This exception is thrown to indicate that you’ve attempted to access an array with an illegal index, either negative or greater than the array’s size.
Here’s a simple example of an ArrayIndexOutOfBoundsException
:
int[] array = {1, 2, 3};
try {
System.out.println(array[3]);
} catch (ArrayIndexOutOfBoundsException e) {
e.printStackTrace();
}
// Output:
// java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
In this example, we try to access array[3]
, which doesn’t exist because our array’s length is 3 and array indices start at 0. To avoid this exception, always ensure your index is within the array’s bounds.
Dealing with Null Values in Arrays
Another common issue is dealing with null values in arrays. If you attempt to call a method on a null value, a NullPointerException
will be thrown.
Here’s an example:
Integer[] array = {1, null, 3};
try {
System.out.println(array[1].toString());
} catch (NullPointerException e) {
e.printStackTrace();
}
// Output:
// java.lang.NullPointerException
In this example, we attempt to call toString()
on a null value, which results in a NullPointerException
. To avoid this, always check if an array element is null before attempting to call methods on it.
Understanding Arrays in Java
What is an Array in Java?
An array in Java is a static data structure that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed.
Here’s an example of how to declare, instantiate, and initialize an array in Java:
int[] array = new int[3];
array[0] = 1;
array[1] = 2;
array[2] = 3;
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3]
In this example, we create an array of integers with a length of 3. We then assign the values 1, 2, and 3 to the array indices 0, 1, and 2, respectively.
How to Manipulate Arrays in Java?
Java provides a class java.util.Arrays
, which contains methods for performing operations on arrays such as sorting and searching. These methods are static, meaning they can be called directly from the class itself, without needing to create an instance of the class.
Here’s an example of using the Arrays.sort()
method to sort an array in ascending order:
int[] array = {3, 2, 1};
Arrays.sort(array);
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3]
In this example, we start with an array in descending order. After applying Arrays.sort()
, our array is rearranged into ascending order. This is just one of the many methods provided by the java.util.Arrays
class for manipulating arrays in Java.
The Relevance of Java Array Methods in Larger Projects
Java Array Methods in Data Processing
Java array methods play a crucial role in data processing tasks. For instance, sorting and searching operations powered by Arrays.sort()
and Arrays.binarySearch()
are fundamental for organizing and retrieving data efficiently.
Consider a scenario where you have a large dataset stored in an array, and you need to find specific entries. Using Arrays.binarySearch()
, you can locate these entries much faster than with a simple linear search.
Java Array Methods in Algorithm Implementation
Many algorithms, especially those related to sorting, searching, and data structures, rely heavily on array operations. For instance, the QuickSort algorithm uses the Arrays.copyOfRange()
method to partition the array into smaller arrays for recursive sorting.
Further Resources for Mastering Java Array Methods
If you’re interested in delving deeper into Java array methods and related topics, here are some resources to explore:
- IOFlood’s Java Arrays Article explores array indexing and accessing elements in Java arrays.
2D Array in Java – Explore two-dimensional arrays in Java for representing matrices and tables.
Adding Elements to Arrays in Java – Explore methods for resizing arrays and appending elements.
Oracle’s Java Tutorials covers all aspects of Java, including the Collections Framework.
Geeks for Geeks Java Programming Articles offers numerous examples and explanations of Java concepts.
Baeldung’s Guide to Java Arrays provides a deep dive into Java arrays.
These resources offer a wealth of information to help you further your understanding and mastery of Java array methods and their applications.
Wrapping Up: Java Array Methods
In this comprehensive guide, we’ve covered everything you need to know about Java array methods. From basic operations like sorting and filling arrays, to more advanced techniques such as copying arrays, comparing them, and conducting binary searches, we’ve delved into the toolbox that Java provides for array manipulation.
We began with the basics, exploring how to use simple methods like Arrays.sort()
, Arrays.fill()
, and Arrays.toString()
. We then moved on to more advanced methods such as Arrays.copyOf()
, Arrays.equals()
, and Arrays.binarySearch()
, providing code examples and detailed explanations for each.
We also discussed alternative approaches to array manipulation in Java, introducing the concept of ArrayLists and Java Streams. We highlighted common issues one might encounter when using Java array methods, such as ArrayIndexOutOfBoundsException
and issues with null values, and provided solutions to these problems.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use | Example |
---|---|---|
Arrays.sort() | Sorts an array | Arrays.sort(array); |
Arrays.fill() | Fills an array with a single value | Arrays.fill(array, 1); |
Arrays.toString() | Converts an array to a string | Arrays.toString(array); |
Arrays.copyOf() | Copies an array | Arrays.copyOf(original, length); |
Arrays.equals() | Compares two arrays | Arrays.equals(array1, array2); |
Arrays.binarySearch() | Searches an array for a specific element | Arrays.binarySearch(array, key); |
Whether you’re a beginner looking to understand the basics or a more experienced developer wanting to deepen your knowledge of Java array methods, we hope this guide has been a valuable resource. With these tools in your toolbox, you’re well-equipped to tackle array manipulation tasks in Java. Happy coding!