3 Ways to Create Multi-Line Comments in Bash
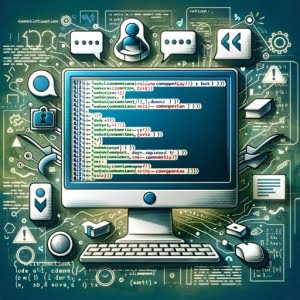
Are you finding it challenging to handle multiline comments in Bash? You’re not alone. Many developers find themselves puzzled when it comes to managing multiline comments in Bash, but we’re here to help.
Think of Bash multiline comments as a roadmap – guiding you through your Bash script, making it easier to understand and navigate. These comments can be a lifesaver when you’re dealing with complex scripts.
In this guide, we’ll walk you through the process of creating multiline comments in Bash, from the basics to more advanced techniques. We’ll cover everything from creating simple multiline comments using ‘here document’, handling different types of comments, to troubleshooting common issues.
Let’s dive in and start mastering Bash multiline comments!
TL;DR: How Do I Write a Multiline Comment in Bash?
In Bash, you can create a multiline comment by enclosing the comment in a
'here document'
. The syntax for this is: <<'END_COMMENT'
followed by your comment and thenEND_COMMENT
.
Here’s a simple example:
: <<'END_COMMENT'
This is a
multiline comment
END_COMMENT
In this example, we start with a colon :
followed by <<'END_COMMENT'
. To close the comment we write the comment name at the end END_COMMENT
. This is just a basic way to write multiline comments in Bash, but there’s much more to learn about handling comments in Bash scripts. Continue reading for more detailed instructions and advanced usage scenarios.
Table of Contents
- Basic Use of Multiline Comments in Bash
- Advanced Multiline Comments: Commenting Out Blocks of Code
- Exploring Alternative Approaches to Bash Comments
- Troubleshooting Multiline Comments in Bash
- Understanding Comments in Programming
- The Role of Comments in Bash Scripting
- The Power of Comments in Larger Bash Scripts
- Wrapping Up: Mastering Bash Multiline Comments
Basic Use of Multiline Comments in Bash
In Bash, the concept of ‘here document’ is used to create multiline comments. A ‘here document’ is a special-purpose code block that uses a form of I/O redirection to feed a command list to an interactive program or a command, such as ftp, cat, or the ex text editor.
Let’s dive into a basic example of how a ‘here document’ can be used to create a multiline comment in Bash.
#!/bin/bash
: <<'END_COMMENT'
This script prints a
welcome message
END_COMMENT
echo 'Welcome to Bash scripting!'
# Output:
# Welcome to Bash scripting!
In this example, we start the script with #!/bin/bash
which tells the system that this is a Bash script. Next, we create a multiline comment using a ‘here document’ that starts with : <<'END_COMMENT'
and ends with END_COMMENT
. This ‘here document’ acts as a multiline comment, and it won’t affect the execution of our script.
The next line after our multiline comment is a simple echo
command that prints ‘Welcome to Bash scripting!’ to the console. As you can see, the multiline comment does not interfere with the execution of the echo
command.
This method of creating multiline comments is straightforward and easy to use. However, it’s important to remember that everything between <<'END_COMMENT'
and END_COMMENT
will be treated as a comment, so make sure not to include any code that you want to execute within these boundaries.
Also, note that the word ‘END_COMMENT’ is arbitrary. You can use any word you like, but it’s a good practice to use a word that indicates the purpose of the comment.
Advanced Multiline Comments: Commenting Out Blocks of Code
As you progress with Bash scripting, you’ll often find yourself in situations where you need to comment out blocks of code. This could be for debugging purposes, or when you want to disable certain parts of your script temporarily. Thankfully, Bash multiline comments make this task effortless.
Let’s take a look at an example where we use a multiline comment to comment out a block of code.
#!/bin/bash
: <<'END_COMMENT'
echo 'This will not be printed.'
echo 'This will also not be printed.'
END_COMMENT
echo 'This will be printed.'
# Output:
# This will be printed.
In this script, we have two echo
commands within the multiline comment block. When we run this script, the lines within the multiline comment block are ignored, and only the echo 'This will be printed.'
command outside the block is executed.
This is a powerful feature of Bash multiline comments. It allows you to easily disable blocks of code without having to prepend each line with a single-line comment symbol.
However, be aware that the ‘here document’ used for multiline comments in Bash is not a comment in the traditional sense. It’s a form of I/O redirection. This means that although the ‘here document’ can serve as a multiline comment, it’s not the same as the single-line comment symbol #
, and there might be situations where the behavior of the ‘here document’ is not what you would expect from a comment. Therefore, it’s crucial to thoroughly test your scripts when using multiline comments.
Exploring Alternative Approaches to Bash Comments
While ‘here documents’ provide a handy way to create multiline comments in Bash, there are other methods you might want to consider, especially when working with smaller blocks of code or when the ‘here document’ behavior is not suitable for your needs.
Using Single-line Comments for Each Line
One such alternative is to use single-line comments for each line. This method involves prefixing each line of the comment block with the #
symbol. Let’s look at an example:
#!/bin/bash
# This is a
# multiline comment
echo 'Hello, world!'
# Output:
# Hello, world!
In this script, we have a multiline comment created by using a #
symbol at the beginning of each line. This method is simple and straightforward, but it can be tedious if you have a large block of text that you want to comment out.
Using a Command That Does Nothing
Another alternative is to use a command that does nothing, like :
or true
, and combine it with the single-line comment symbol. Here’s an example:
#!/bin/bash
: # This is a
: # multiline comment
echo 'Hello, world!'
# Output:
# Hello, world!
This method works similarly to the previous one, but it uses a command (:
) in conjunction with the #
symbol. This can be useful in certain situations, but it’s more verbose than the other methods.
In conclusion, while ‘here documents’ are a powerful tool for creating multiline comments in Bash, they are not the only option. Depending on your specific needs and the complexity of your scripts, you might find that one of these alternative methods is a better fit. As always, the key is to choose the method that makes your code as clear and understandable as possible.
Troubleshooting Multiline Comments in Bash
While Bash multiline comments are a powerful tool, they can sometimes cause unexpected issues. This is particularly true if you’re new to Bash scripting or if you’re working with complex scripts. Let’s discuss some common issues you might encounter and how to solve them.
Syntax Errors
One of the most common issues when dealing with multiline comments in Bash is syntax errors. These errors can occur if you don’t properly close the ‘here document’ or if you accidentally include code within the comment block.
Consider the following example:
#!/bin/bash
: <<'END_COMMENT'
echo 'Hello, world!'
# Output:
# bash: syntax error: unexpected end of file
In this script, we forgot to close the ‘here document’ with END_COMMENT
. As a result, the echo
command is treated as part of the comment, and the script fails with a syntax error because it reaches the end of the file without finding END_COMMENT
.
To avoid this issue, always ensure that you properly close the ‘here document’ with the same word you used to start it.
Accidentally Including Code in the Comment Block
Another common issue is accidentally including code in the comment block. Any code within the ‘here document’ will be treated as a comment and will not be executed.
Here’s an example:
#!/bin/bash
: <<'END_COMMENT'
echo 'Hello, world!'
END_COMMENT
# Output:
# (no output)
In this script, the echo
command is within the ‘here document’ and is therefore treated as a comment. As a result, the script produces no output.
To avoid this issue, always check your ‘here document’ blocks to ensure that they don’t contain any code that you want to execute.
In conclusion, while Bash multiline comments are a powerful tool, they can sometimes cause unexpected issues. By understanding these issues and knowing how to solve them, you can use multiline comments effectively and avoid common pitfalls.
Understanding Comments in Programming
Comments are an integral part of programming, regardless of the language or the complexity of the code. They serve as guideposts, providing valuable context, explanations, and insights into what the code does and why certain decisions were made.
In essence, comments are like a conversation between you and your future self, or between you and other developers who might work on your code. They help to ensure that the code remains understandable and maintainable, even as it evolves over time.
# This is a single-line comment in Python
print('Hello, world!') # This is an inline comment
# Output:
# Hello, world!
In the above Python code, we use comments to explain what the print
function does. While this is a simple example, it illustrates the fundamental role of comments in programming.
The Role of Comments in Bash Scripting
In Bash scripting, comments are just as important. They can explain the purpose of a script, provide details about the logic or algorithms used, and give information about the input and output data.
#!/bin/bash
# This script prints the number of files in the current directory
count=$(ls | wc -l)
echo "There are $count files in the current directory."
# Output:
# There are X files in the current directory.
In this Bash script, we use a comment to explain what the script does. The comment helps anyone reading the code to understand its purpose without having to decipher the commands and their parameters.
In addition to single-line comments, Bash supports multiline comments using ‘here documents’. As we’ve discussed, these comments are a powerful tool for providing more extensive explanations or for temporarily disabling blocks of code.
In conclusion, comments are a fundamental part of programming and scripting. They enhance readability, foster collaboration, and contribute significantly to the maintainability of code. Therefore, mastering the use of comments, including multiline comments in Bash, is an essential skill for any developer.
The Power of Comments in Larger Bash Scripts
When working with larger Bash scripts or projects, the use of comments, including multiline comments, becomes even more essential. As scripts grow in complexity, they can become harder to understand and maintain. Comments can help mitigate this by providing important context and explanations.
Bash Scripting Best Practices
In addition to using comments effectively, there are other best practices in Bash scripting that can help ensure your scripts are robust, efficient, and easy to understand. These include using functions to encapsulate reusable code, error handling to make your scripts more robust, and using meaningful variable names.
Code Readability and Maintenance
Comments, particularly multiline comments, play a crucial role in code readability. They allow you to provide detailed explanations and context that can make your code easier to understand. This is particularly important when working on projects with multiple developers, where clear communication through code is key.
Moreover, well-commented code is easier to maintain. If you need to revisit your code after some time, or if another developer needs to update your code, well-placed comments can save a lot of time and effort.
Further Resources for Mastering Bash Scripting
To deepen your understanding of Bash scripting and multiline comments, here are some valuable resources:
- Advanced Bash-Scripting Guide: An in-depth exploration of Bash scripting, including a detailed section on ‘here documents’.
Bash Guide for Beginners: A comprehensive guide for those starting out with Bash scripting, with easy-to-follow examples and explanations.
Bash Academy: An interactive platform to learn Bash scripting, with a focus on practical examples and exercises.
By leveraging these resources and mastering the use of comments in your Bash scripts, you can write more effective, maintainable, and robust scripts.
Wrapping Up: Mastering Bash Multiline Comments
In this comprehensive guide, we’ve explored the world of multiline comments in Bash, a powerful tool for enhancing the readability and maintainability of your scripts.
We began with the basics, learning how to create a multiline comment using a ‘here document’. We then delved into more advanced usage, demonstrating how to comment out blocks of code and providing alternative approaches for creating comments.
Along the way, we tackled common issues you might encounter when using multiline comments in Bash, such as syntax errors and accidentally including code in the comment block. We provided solutions and tips for each issue, helping you to avoid these common pitfalls.
We also looked at the broader context of comments in programming, highlighting their importance in enhancing code readability, fostering collaboration, and contributing to the maintainability of code. Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Flexibility | Suitability for Large Blocks of Text |
---|---|---|---|
‘Here Document’ | Moderate | High | High |
Single-line Comments | High | Low | Low |
Command with Single-line Comments | High | Moderate | Moderate |
Whether you’re just starting out with Bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Bash multiline comments.
With the ability to enhance the readability and maintainability of your scripts, multiline comments are a powerful tool in Bash scripting. Now, you’re well equipped to use them effectively. Happy scripting!