How-To Use ‘Do While’ Loops | Bash Script Control Flow
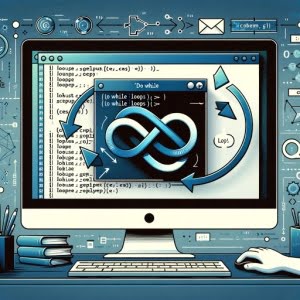
Are you finding it tough to get a grip on the ‘do while’ loop in Bash? You’re not alone. Many developers find themselves in a bind when trying to implement this fundamental control flow structure in their scripts.
Think of the ‘do while’ loop in Bash as a diligent worker, tirelessly performing a task over and over until a certain condition is met. It’s a powerful tool in your Bash scripting arsenal that can automate repetitive tasks and make your scripts more efficient.
In this guide, we’ll walk you through the process of using the ‘do while’ loop in Bash scripting, from the basics to more advanced techniques. We’ll cover everything from writing a simple ‘do while’ loop, handling multiple conditions, to troubleshooting common issues and exploring alternative approaches.
So, let’s dive in and start mastering the ‘do while’ loop in Bash!
TL;DR: How Do I Use a ‘Do While’ Loop in Bash?
In Bash, you can use a
'do while'
loop to execute a block of code repeatedly as long as a certain condition is true. Ado while
loop is made with the syntaxwhile [condition]; do...
. It’s a powerful tool for automating tasks and making your scripts more efficient.
Here’s a simple example:
#!/bin/bash
COUNTER=0
while [ $COUNTER -lt 10 ]; do
echo The counter is $COUNTER
let COUNTER=COUNTER+1
done
# Output:
# The counter is 0
# The counter is 1
# The counter is 2
# ...
# The counter is 9
In this example, we’ve created a ‘do while’ loop that prints the value of a counter variable as long as it’s less than 10. The let COUNTER=COUNTER+1
line increments the counter by one each time the loop runs. Once the counter reaches 10, the condition $COUNTER -lt 10
is no longer true, and the loop stops.
This is just a basic way to use the ‘do while’ loop in Bash, but there’s much more to learn about this powerful control flow structure. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Grasping the Basics of ‘Do While’ Loop in Bash
- Delving Deeper: Advanced ‘Do While’ Loop Usage in Bash
- Exploring Alternative Loop Structures in Bash
- Troubleshooting Common ‘Do While’ Loop Issues in Bash
- Understanding the Fundamentals of Bash Scripting and Loops
- Expanding Your Horizons: ‘Do While’ Loop in Larger Scripts and Projects
- Wrapping Up: The ‘Do While’ Loop in Bash
Grasping the Basics of ‘Do While’ Loop in Bash
The ‘do while’ loop in Bash is a control flow statement that allows code or commands to be executed repeatedly based on a given condition. The loop will always execute the block of code once, then it will repeat the loop as long as the condition is true.
Let’s break down the basic structure of a ‘do while’ loop in Bash:
while [ condition ]
do
commands
done
In this structure, the while
keyword initiates the loop, followed by the condition in square brackets. The do
keyword then precedes the commands that you want to be executed repeatedly. Finally, the done
keyword signifies the end of the loop.
Now, let’s look at a simple example of a ‘do while’ loop that prints the numbers from 1 to 5:
#!/bin/bash
num=1
while [ $num -le 5 ]
do
echo Number: $num
let num++
done
# Output:
# Number: 1
# Number: 2
# Number: 3
# Number: 4
# Number: 5
In this example, we initialize a variable num
to 1. The ‘do while’ loop then keeps printing the value of num
and incrementing it by 1 until num
is no longer less than or equal to 5. Once num
exceeds 5, the condition $num -le 5
is no longer true, and the loop terminates.
This basic use of the ‘do while’ loop in Bash demonstrates how you can automate repetitive tasks and make your scripts more efficient.
Delving Deeper: Advanced ‘Do While’ Loop Usage in Bash
Once you’ve mastered the basics of ‘do while’ loops in Bash, it’s time to explore some more advanced use cases. This includes scenarios where you might need to use nested loops or handle multiple conditions.
Bash Do While with Multiple Conditions
In Bash, you can use multiple conditions in your ‘do while’ loop. This allows you to create more complex control flow structures. Let’s look at an example where we print numbers from 1 to 10, but skip the number 5:
#!/bin/bash
num=1
while [ $num -le 10 ]
do
if [ $num -ne 5 ]
then
echo Number: $num
fi
let num++
done
# Output:
# Number: 1
# Number: 2
# Number: 3
# Number: 4
# Number: 6
# Number: 7
# Number: 8
# Number: 9
# Number: 10
In this script, we’ve added an if
statement inside the ‘do while’ loop. This checks if the current value of num
is not equal to 5. If it’s not, the script prints the number. If it is, the script skips printing and increments num
.
Nested ‘Do While’ Loops in Bash
You can also nest ‘do while’ loops within each other. This is useful when you need to perform a task multiple times within another task. Here’s an example where we use a nested ‘do while’ loop to create a multiplication table:
#!/bin/bash
i=1
while [ $i -le 10 ]
do
j=1
while [ $j -le 10 ]
do
echo -n "$i * $j = $(($i*$j)) "
let j++
done
echo ""
let i++
done
# Output:
# 1 * 1 = 1 1 * 2 = 2 1 * 3 = 3 ...
# 2 * 1 = 2 2 * 2 = 4 2 * 3 = 6 ...
# ...
# 10 * 1 = 10 10 * 2 = 20 10 * 3 = 30 ...
In this script, the outer ‘do while’ loop runs 10 times, and for each iteration of the outer loop, the inner ‘do while’ loop also runs 10 times. This results in a multiplication table for the numbers 1 to 10.
These advanced uses of the ‘do while’ loop in Bash demonstrate its flexibility and power. By using multiple conditions and nested loops, you can create complex scripts to automate a wide range of tasks.
Exploring Alternative Loop Structures in Bash
While the ‘do while’ loop is a powerful tool, Bash scripting offers other types of loops that might be better suited to certain tasks. In this section, we’ll explore the ‘for’ loop and the ‘until’ loop, providing examples and discussing when to use each one.
The ‘For’ Loop in Bash
The ‘for’ loop in Bash is used to iterate over a series of words in a string, lines in a file, or elements in an array. Here’s a simple example that prints the numbers from 1 to 5:
#!/bin/bash
for num in {1..5}
do
echo Number: $num
done
# Output:
# Number: 1
# Number: 2
# Number: 3
# Number: 4
# Number: 5
In this script, the ‘for’ loop iterates over the sequence of numbers from 1 to 5, printing each one. This is a simpler and more intuitive way to perform a task a certain number of times, compared to the ‘do while’ loop.
The ‘Until’ Loop in Bash
The ‘until’ loop in Bash is similar to the ‘do while’ loop, but it keeps executing the block of code as long as the condition is false. Here’s an example that prints the numbers from 1 to 5:
#!/bin/bash
num=1
until [ $num -gt 5 ]
do
echo Number: $num
let num++
done
# Output:
# Number: 1
# Number: 2
# Number: 3
# Number: 4
# Number: 5
In this script, the ‘until’ loop keeps printing and incrementing num
until num
is greater than 5. This is a useful alternative to the ‘do while’ loop when you want to invert the condition.
In conclusion, while the ‘do while’ loop is a versatile control flow structure in Bash, the ‘for’ loop and the ‘until’ loop offer alternative approaches that might be more suitable in certain scenarios. By understanding and using these different types of loops, you can write more efficient and readable Bash scripts.
Troubleshooting Common ‘Do While’ Loop Issues in Bash
While the ‘do while’ loop in Bash is a powerful tool, it’s not without its quirks. In this section, we’ll discuss some common issues and errors you might encounter when using the ‘do while’ loop, and how to resolve them.
Infinite Loops
One common issue with ‘do while’ loops is the infinite loop, where the loop keeps running forever because the condition never becomes false. This can happen if you forget to update the variables used in the condition within the loop.
Here’s an example of an infinite loop:
#!/bin/bash
num=1
while [ $num -le 5 ]
do
echo Number: $num
done
# Output:
# Number: 1
# Number: 1
# Number: 1
# ...
In this script, the loop keeps printing 1
forever because num
is never incremented. To fix this, we need to increment num
inside the loop:
#!/bin/bash
num=1
while [ $num -le 5 ]
do
echo Number: $num
let num++
done
# Output:
# Number: 1
# Number: 2
# Number: 3
# Number: 4
# Number: 5
Syntax Errors
Another common issue is syntax errors, which can occur if you forget to use the correct keywords or punctuation. For example, forgetting to close the condition with ]
or omitting the do
keyword will result in a syntax error.
Here’s an example of a syntax error:
#!/bin/bash
num=1
while [ $num -le 5
do
echo Number: $num
let num++
done
# Output:
# ./script.sh: line 5: syntax error near unexpected token `do'
# ./script.sh: line 5: `do'
In this script, we’ve forgotten to close the condition with ]
, resulting in a syntax error. To fix this, we need to add the missing ]
:
#!/bin/bash
num=1
while [ $num -le 5 ]
do
echo Number: $num
let num++
done
# Output:
# Number: 1
# Number: 2
# Number: 3
# Number: 4
# Number: 5
By understanding and avoiding these common issues, you can use the ‘do while’ loop in Bash more effectively and write more robust scripts.
Understanding the Fundamentals of Bash Scripting and Loops
To fully grasp the ‘do while’ loop in Bash, it’s important to understand the fundamentals of Bash scripting and loops. Bash, or the Bourne-Again SHell, is a command language interpreter for the GNU operating system. It’s widely used for its ability to control job control, shell functions and scripts, and command-line editing.
Bash Scripting Basics
A Bash script is a plain text file containing a series of commands. These commands are a sequence of orders for a computer system to execute. Here’s a simple Bash script that prints ‘Hello, World!’:
#!/bin/bash
echo 'Hello, World!'
# Output:
# Hello, World!
In this script, #!/bin/bash
is called a shebang, which tells the system that this script should be executed with Bash. The echo
command is used to print its arguments to the standard output, which is usually the terminal.
Looping in Bash
Looping is a fundamental concept in programming that allows you to repeat a block of commands a certain number of times. Bash supports several types of loops, including the ‘for’ loop, the ‘while’ loop, and the ‘until’ loop.
The ‘do while’ loop we’ve been discussing is a type of ‘while’ loop. It checks a condition before each iteration, and continues executing the commands as long as the condition is true. Here’s a simple ‘do while’ loop that prints the numbers from 1 to 3:
#!/bin/bash
num=1
while [ $num -le 3 ]
do
echo Number: $num
let num++
done
# Output:
# Number: 1
# Number: 2
# Number: 3
In this script, the ‘do while’ loop keeps printing and incrementing num
until num
is greater than 3. This demonstrates the basic concept of looping in Bash.
Understanding these fundamentals of Bash scripting and loops is crucial for mastering the ‘do while’ loop. With this foundation, you can write more complex scripts and automate a wide range of tasks.
Expanding Your Horizons: ‘Do While’ Loop in Larger Scripts and Projects
The ‘do while’ loop in Bash is not just for simple tasks. It can also be a powerful tool in larger scripts and projects. By using ‘do while’ loops, you can automate complex tasks, simplify your code, and make your scripts more efficient.
For instance, you could use a ‘do while’ loop to monitor system logs in real-time. With a ‘do while’ loop, you can continuously read the latest entries in a log file and perform actions based on the content of those entries.
Here’s a basic example of how you might do this:
#!/bin/bash
# Path to the log file
logfile=/var/log/syslog
# Tail the log file and pipe it into the 'do while' loop
tail -F $logfile | while read line
do
# Check if the line contains the word 'error'
if echo $line | grep -q 'error'
then
# If it does, print the line
echo $line
fi
done
# Output:
# [The output will depend on the content of your syslog file]
In this script, the ‘do while’ loop reads lines from the syslog file as they’re added. If a line contains the word ‘error’, the script prints the line. This could be a useful way to monitor your system for errors in real-time.
Exploring Related Topics in Bash
Once you’ve mastered the ‘do while’ loop in Bash, there are many related topics you might want to explore. For instance, you might want to learn about conditional statements in Bash, such as ‘if’, ‘else’, and ‘case’. These can be used in combination with ‘do while’ loops to create more complex control flow structures.
You might also want to learn about functions in Bash. Functions allow you to group commands into reusable blocks, which can simplify your scripts and make them easier to read and maintain.
Further Resources for Mastering Bash Scripting
If you’re interested in learning more about Bash scripting, here are some resources that might help:
- Advanced Bash-Scripting Guide: This is an in-depth guide to Bash scripting, covering everything from basics to advanced topics.
Bash while Loop: This Cyberciti tutorial provides a detailed explanation of the while loop in Bash scripting.
GNU Bash Manual: This is the official manual for Bash from the GNU project. It’s a comprehensive resource that covers all aspects of Bash scripting.
Wrapping Up: The ‘Do While’ Loop in Bash
In this comprehensive guide, we’ve delved into the intricacies of using the ‘do while’ loop in Bash scripting. This control flow structure, when used correctly, can automate repetitive tasks and enhance the efficiency of your scripts.
We began with the basics, learning how to write a simple ‘do while’ loop and understanding its structure. We then ventured into more advanced territory, exploring complex scenarios like handling multiple conditions and nested loops. Along the way, we tackled common challenges you might encounter when using the ‘do while’ loop, such as infinite loops and syntax errors, providing you with solutions for each issue.
We also looked at alternative approaches to looping in Bash, comparing the ‘do while’ loop with other control flow structures like the ‘for’ loop and the ‘until’ loop. Here’s a quick comparison of these loops:
Loop Type | Use Case | Complexity |
---|---|---|
‘Do While’ | Repeatedly execute a block of code as long as a condition is true | Moderate |
‘For’ | Iterate over a series of words in a string, lines in a file, or elements in an array | Low |
‘Until’ | Execute a block of code as long as a condition is false | Moderate |
Whether you’re just starting out with Bash scripting or looking to level up your skills, we hope this guide has given you a deeper understanding of the ‘do while’ loop in Bash and its capabilities.
With its balance of flexibility and power, the ‘do while’ loop is a crucial tool in any Bash scripter’s toolbox. Now, you’re well equipped to harness its benefits in your scripting journey. Happy coding!