Python Uppercase | String Case Conversion Guide
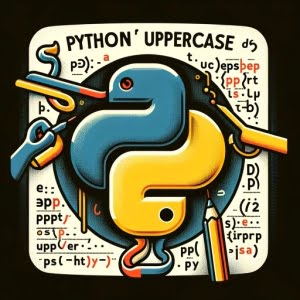
Are you finding it challenging to convert strings to uppercase in Python? You’re not alone. Many developers find themselves puzzled when it comes to this task. Think of Python’s string manipulation capabilities as a Swiss army knife – versatile and handy for various tasks.
In this guide, we’ll walk you through the process of converting strings to uppercase in Python, from the basics to more advanced techniques. We’ll cover everything from the upper()
function, dealing with special characters, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Convert a String to Uppercase in Python?
To convert a string to uppercase in Python, you can use the
upper()
function, such asprint(text.upper())
, wheretext
is a string variable. This function is a built-in method in Python that can be used on any string object to convert all the characters to uppercase.
Here’s a simple example:
text = 'hello world'
uppercase_text = text.upper()
print(uppercase_text)
# Output:
# 'HELLO WORLD'
In this example, we have a string ‘hello world’. We use the upper()
function on this string, which converts all the characters to uppercase. The result is ‘HELLO WORLD’.
This is a basic way to convert a string to uppercase in Python. However, Python’s string manipulation capabilities are vast and versatile. Continue reading for a more detailed explanation, advanced usage scenarios, and alternative approaches.
Table of Contents
- The upper() Function: Python’s Built-in Tool for Uppercase Conversion
- Tackling Special and Non-English Characters in Python Uppercase Conversion
- Exploring Alternative Approaches to Python Uppercase Conversion
- Troubleshooting Common Issues in Python Uppercase Conversion
- Python Strings and Character Encoding: The Basics
- The Power of String Manipulation in Python: Beyond Uppercase Conversion
- Wrapping Up: Mastering the Art of String Conversion in Python
The upper()
Function: Python’s Built-in Tool for Uppercase Conversion
Python’s built-in upper()
function is your go-to tool for converting any string to uppercase. This function operates on a string and returns a new string where all the lowercase letters have been converted to uppercase.
Let’s illustrate this with a simple example:
sentence = 'python is fun'
uppercase_sentence = sentence.upper()
print(uppercase_sentence)
# Output:
# 'PYTHON IS FUN'
In this example, we have a string ‘python is fun’. We use the upper()
function on this string, which converts all the characters to uppercase. The result is ‘PYTHON IS FUN’.
The upper()
function is straightforward to use and effective for transforming strings to uppercase in Python. It’s essential to remember that this function doesn’t modify the original string because strings in Python are immutable. Instead, it returns a new string.
One of the great things about the upper()
function is its simplicity and efficiency. However, it’s worth noting that it may not behave as expected with strings containing non-English characters or special characters. We’ll explore more about this in the advanced usage section.
Tackling Special and Non-English Characters in Python Uppercase Conversion
As you become more comfortable with Python’s upper()
function, you might encounter strings containing special characters or non-English characters. The upper()
function handles these cases too, but the results might not always be what you expect.
Let’s see this in action with a string that includes a special character:
special_string = 'hello, world!'
uppercase_special_string = special_string.upper()
print(uppercase_special_string)
# Output:
# 'HELLO, WORLD!'
In this example, the special character ‘!’ is unaffected by the upper()
function, as it doesn’t have an uppercase version.
Now, let’s consider a string with non-English characters:
non_english_string = 'héllo, wórld!'
uppercase_non_english_string = non_english_string.upper()
print(uppercase_non_english_string)
# Output:
# 'HÉLLO, WÓRLD!'
In the case of non-English characters, such as ‘é’ and ‘ó’, the upper()
function successfully converts them to their uppercase counterparts.
While the upper()
function is a powerful tool for string conversion in Python, it’s important to be aware of its limitations and quirks when dealing with special and non-English characters. Understanding these nuances will help you navigate string manipulation tasks more effectively.
Exploring Alternative Approaches to Python Uppercase Conversion
While the upper()
function is a reliable way to convert strings to uppercase in Python, it’s not the only tool at your disposal. Let’s explore some alternative approaches that you can use for this task.
Using the capitalize()
Function
The capitalize()
function is another built-in Python function that can be used to convert the first character of a string to uppercase. However, keep in mind that this function will make the rest of the string lowercase.
Here’s an example:
sentence = 'hello WORLD'
new_sentence = sentence.capitalize()
print(new_sentence)
# Output:
# 'Hello world'
As you can see, the capitalize()
function converted the first character of the string to uppercase and the rest of the string to lowercase.
Leveraging Third-Party Libraries
Python’s vast ecosystem of third-party libraries also offers solutions for converting strings to uppercase. For instance, the pandas
library, commonly used for data manipulation and analysis, provides a method str.upper()
for its Series objects.
Here’s how you can use it:
import pandas as pd
ser = pd.Series(['hello', 'world'])
new_ser = ser.str.upper()
print(new_ser)
# Output:
# 0 HELLO
# 1 WORLD
dtype: object
In this example, we created a pandas Series and used the str.upper()
method to convert all the strings in the Series to uppercase.
Both the capitalize()
function and the pandas
library provide effective alternatives to the upper()
function. However, they come with their own sets of advantages and disadvantages. The capitalize()
function is simple to use but only affects the first character of the string, while the pandas
method is powerful but requires understanding of the pandas library. Depending on your specific use case, one method may be more suitable than the others.
Troubleshooting Common Issues in Python Uppercase Conversion
As you gain more experience with Python’s string manipulation capabilities, you may encounter a few common issues. Let’s discuss some of these and provide solutions and workarounds.
Dealing with Mixed Case Strings
One common issue arises when dealing with mixed case strings. The upper()
function will convert all lowercase characters to uppercase, but it leaves the existing uppercase characters as they are. Let’s see this in action:
mixed_case_string = 'Hello WORLD'
uppercase_mixed_case_string = mixed_case_string.upper()
print(uppercase_mixed_case_string)
# Output:
# 'HELLO WORLD'
As you can see, the upper()
function has converted all the lowercase characters to uppercase, while the existing uppercase characters remain the same.
Handling Strings with Special Characters
Another common issue involves handling strings with special characters. As we’ve seen earlier, the upper()
function does not affect special characters as they do not have an uppercase version.
special_string = 'hello, world!'
uppercase_special_string = special_string.upper()
print(uppercase_special_string)
# Output:
# 'HELLO, WORLD!'
In this code block, the special character ‘!’ is unaffected by the upper()
function.
These are just a few of the common issues you may encounter when converting strings to uppercase in Python. Understanding these potential pitfalls can help you navigate them more effectively in your coding journey.
Python Strings and Character Encoding: The Basics
To fully grasp the process of converting strings to uppercase in Python, it’s essential to understand some fundamental concepts about Python’s string data type and character encoding.
Understanding Python Strings
In Python, a string is a sequence of characters. It’s considered an immutable data type, meaning that you can’t change an existing string. Any operation that transforms a string will instead create a new string.
Let’s take a look at a simple string declaration in Python:
simple_string = 'Hello, World!'
print(simple_string)
# Output:
# 'Hello, World!'
In this example, we’ve created a string and assigned it to the variable simple_string
. This string is a sequence of characters, including letters, a comma, and an exclamation mark.
Character Encoding and Unicode
Character encoding is a system that pairs individual characters with their binary representations. Python uses Unicode, a universal character encoding standard that provides a unique number for every character across various platforms and languages.
For example, the Unicode code point for the letter ‘A’ is U+0041. In Python, you can represent this as a string using the escape sequence ‘\u0041’:
unicode_string = '\u0041'
print(unicode_string)
# Output:
# 'A'
In this code block, we’ve created a string that represents the Unicode code point for ‘A’. When we print this string, Python converts the Unicode code point to its corresponding character.
Understanding these fundamentals of Python strings and character encoding can provide a solid foundation for mastering string manipulation tasks, such as converting strings to uppercase.
The Power of String Manipulation in Python: Beyond Uppercase Conversion
String manipulation in Python extends far beyond converting strings to uppercase. It’s a powerful tool that plays a crucial role in various areas of programming and data science.
String Manipulation in Data Processing
In data processing, string manipulation is often used to clean and prepare data. This can involve converting strings to a standard format, removing unwanted characters, or extracting specific information from strings.
Text Mining with Python
Text mining is another field where string manipulation is essential. It involves extracting high-quality information from text using patterns and trends. Tasks can include sentiment analysis, topic modeling, and text classification, all of which rely heavily on the ability to manipulate and analyze strings.
Exploring Related Concepts
If you’re interested in furthering your understanding of string manipulation in Python, there are several related concepts worth exploring. These include string formatting, which allows you to insert variables into strings, and regular expressions, a powerful tool for matching and manipulating strings.
# String formatting example
name = 'Alice'
greeting = f'Hello, {name}!'
print(greeting)
# Output:
# 'Hello, Alice!'
In this example, we use string formatting to insert the variable name
into the string greeting
. The f
before the string indicates that it’s a formatted string.
Further Resources for Mastering Python String Manipulation
To deepen your understanding of string manipulation in Python, here are some resources you might find useful:
- Python Print: Strengthening Your Output Skills – Master printing in Python by leveraging the full potential of the print() function.
Python Zfill Function – Learn how to use zfill() in Python for formatting numeric strings with fixed widths.
Print to STDERR in Python – Understand how to print error messages to stderr in Python for debugging and logging.
Official Python Documentation on String Methods – Explore Python’s official guide on string methods.
Python String Manipulation Tutorial – Learn the essentials of manipulating strings in Python with this comprehensive tutorial.
Python String Formatting Best Practices – Discover best practices for string formatting in Python.
These resources provide an in-depth look at Python’s string manipulation capabilities and can help you master this essential skill.
Wrapping Up: Mastering the Art of String Conversion in Python
In this comprehensive guide, we’ve delved into the process of converting strings to uppercase in Python. From using the built-in upper()
function to handling special and non-English characters, we’ve covered a wide range of techniques to help you master this fundamental aspect of Python string manipulation.
We began with the basics, learning how to use the upper()
function to convert strings to uppercase. We then ventured into more advanced territory, exploring how to handle strings containing special characters and non-English characters.
We also looked at alternative methods for converting strings to uppercase, such as using the capitalize()
function and leveraging third-party libraries like pandas
.
Along the way, we tackled common issues you might face when converting strings to uppercase in Python, such as dealing with mixed case strings and special characters, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
upper() function | Simple, effective | May not behave as expected with special and non-English characters |
capitalize() function | Converts first character to uppercase | Makes rest of the string lowercase |
pandas library | Powerful, versatile | Requires understanding of pandas |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of how to convert strings to uppercase in Python. Happy coding!