Python Format String: Techniques and Examples
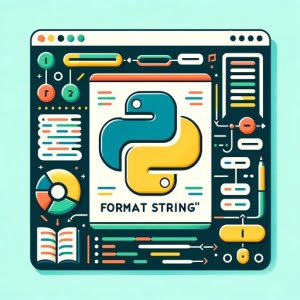
Struggling with string formatting in Python? You’re not alone. Many developers find themselves puzzled when it comes to formatting strings in Python. Luckily there are various methods available in the Python language to help generate dynamic messages, format data for output, or even debug errors.
In this guide, we’ll walk you through the process of string formatting in Python, from the basics to more advanced techniques. We’ll cover everything from the format()
function, f-strings, to even some alternative approaches that will significantly streamline your coding process.
So, Let’s dive into string formatting!
TL;DR: How Do I Use the Format() Function in Python?
Python provides several ways to format strings, but the most common method is using the
format()
function like this:'My name is {} and I am {} years old'.format('John', 30)
.
Here’s a simple example:
name = 'John'
age = 30
print('My name is {} and I am {} years old'.format(name, age))
# Output:
# 'My name is John and I am 30 years old'
In this example, we’ve used the format()
function to insert variables into a string. The curly braces {}
are placeholders where the variables get inserted. In this case, ‘John’ and ’30’ are inserted in place of the curly braces, resulting in the output ‘My name is John and I am 30 years old’.
But Python’s string formatting capabilities go far beyond this. Continue reading for more detailed examples and advanced formatting techniques.
Table of Contents
The Basics: Python format() Method
Python’s format()
function is one of the most common ways to format strings. It’s simple to use and highly versatile, making it an excellent starting point for beginners.
The format()
function works by replacing placeholders – curly braces {}
– in the string with the arguments provided in the function. Let’s look at a basic example:
name = 'Alice'
age = 25
print('My name is {} and I am {} years old'.format(name, age))
# Output:
# 'My name is Alice and I am 25 years old'
In this example, the format()
function replaces the first {}
with the variable name
(‘Alice’) and the second {}
with the variable age
(25). The result is a formatted string that includes our variables.
One of the main advantages of the format()
function is its readability. The placeholders clearly indicate where the variables will be inserted, making the code easier to understand. Additionally, the format()
function is flexible and can handle a variety of data types, including strings, integers, floats, and more.
However, there are some potential pitfalls to be aware of. For example, if you provide more arguments than there are placeholders, Python will raise an error:
print('My name is {}'.format('Alice', 25))
# Output:
# IndexError: Replacement index 1 out of range for positional args tuple
In this case, we’ve provided two arguments (‘Alice’ and 25) but only included one placeholder in the string. Python doesn’t know what to do with the extra argument, so it raises an IndexError
.
Understanding these basic principles of the format()
function is the first step towards mastering Python string formatting. In the next section, we’ll explore some more advanced techniques.
Advanced String Formatting in Python
As you become more comfortable with Python string formatting, you might find yourself needing more advanced techniques. Let’s delve into some of them.
F-Strings: A Newer, More Convenient Method
Python 3.6 introduced f-strings, a new way to format strings that’s both more readable and often faster than the format()
function. F-strings are prefixed with an ‘f’ and replace placeholders with the values of variables directly:
name = 'Alice'
age = 25
print(f'My name is {name} and I am {age} years old')
# Output:
# 'My name is Alice and I am 25 years old'
In this example, the f-string replaces {name}
and {age}
with the values of the variables name
and age
, respectively. The result is the same as our previous format()
example, but with less code and improved readability.
Formatting Specifiers: Controlling How Variables Are Displayed
Python also allows you to control how variables are displayed using formatting specifiers. For example, you can control the number of decimal places for a float, or add padding to a string. Here’s an example:
pi = 3.14159
print(f'The value of pi to 2 decimal places is {pi:.2f}')
# Output:
# 'The value of pi to 2 decimal places is 3.14'
In this case, the :.2f
inside the placeholder is a formatting specifier. It tells Python to format the variable pi
as a float with 2 decimal places.
Alignment Options: Aligning Your Text
You can also use Python’s string formatting to align text. This can be particularly useful when you’re printing tabular data. For example:
print(f'{"Left":<10} | {"Center":^10} | {"Right":>10}')
# Output:
# 'Left | Center | Right'
In this example, the <
, ^
, and >
symbols inside the placeholders specify left alignment, center alignment, and right alignment, respectively. The number following these symbols specifies the width of the field.
These advanced techniques can give you more control over your Python string formatting and make your output more readable and professional-looking. In the next section, we’ll explore some alternative approaches to string formatting.
Alternate String Formatting Methods
While the format()
function and f-strings are the most commonly used methods for string formatting in Python, there are other techniques available that can be more suitable depending on the situation. Let’s explore some of these alternative approaches.
The % Operator: Python’s Original Formatting Method
Before the format()
function and f-strings, Python used the %
operator for string formatting. This method is similar to printf-style formatting in C. Here’s an example:
name = 'Alice'
age = 25
print('My name is %s and I am %d years old' % (name, age))
# Output:
# 'My name is Alice and I am 25 years old'
In this example, %s
and %d
are placeholders for a string and an integer, respectively. The variables to be inserted are provided in a tuple after the %
operator. While this method is less common in modern Python code, it’s still fully supported and can be useful in certain scenarios.
Template Strings: A Simpler and Safer Method
Python’s string
module provides a Template
class that offers a simpler and safer way to substitute placeholders with variables. This method is particularly useful when handling format strings that are supplied by the user, as it helps prevent security vulnerabilities associated with the other methods. Here’s how it works:
from string import Template
t = Template('My name is $name and I am $age years old')
print(t.substitute(name='Alice', age=25))
# Output:
# 'My name is Alice and I am 25 years old'
In this example, the placeholders are prefixed with a $
symbol and the variables are provided as keyword arguments to the substitute()
method.
Third-Party Libraries: For More Complex Formatting Needs
There are also several third-party libraries available that can provide more advanced formatting capabilities. For example, the formatting
library offers a more powerful version of f-strings, while the tabulate
library can help with formatting tabular data. These libraries can be installed via pip and offer extensive documentation to help you get started.
As you can see, Python offers a wealth of options when it comes to string formatting. The best method to use will depend on your specific needs and the complexity of your formatting tasks. In the next section, we’ll explore some common issues encountered during string formatting and how to troubleshoot them.
Troubleshooting Issues with .format()
Even with a solid understanding of Python’s string formatting techniques, you might encounter some common issues. Let’s explore a few of these and how to resolve them.
TypeError: Not Enough Arguments
One common issue is providing fewer arguments than there are placeholders in the string. This will result in a TypeError
:
print('My name is {} and I am {} years old'.format('Alice'))
# Output:
# TypeError: not enough arguments for format string
In this example, we’ve provided only one argument (‘Alice’) but have two placeholders in the string. Python raises a TypeError
because it doesn’t have enough arguments to fill all the placeholders. To resolve this, ensure you provide an argument for each placeholder in the string.
ValueError: Single ‘}’ Encountered in Format String
Another common issue is incorrectly using the curly braces {}
in the format string. If you need to include a literal curly brace in your string, you’ll need to escape it by doubling it up {{}}
:
print('This is a {} curly brace'.format('{}'))
# Output:
# ValueError: Single '}' encountered in format string
In this example, we’ve tried to include a literal curly brace in the string using {}
. Python raises a ValueError
because it’s expecting a }
to close the placeholder. To include a literal curly brace, you need to escape it by doubling it up:
print('This is a {} curly brace'.format('{{}}'))
# Output:
# This is a {} curly brace
These are just a few examples of the issues you might encounter when formatting strings in Python. By understanding these common problems and their solutions, you can troubleshoot more effectively and write more robust code.
Fundamentals of Strings and Format
Before we delve deeper into Python string formatting, it’s crucial to understand the basics of Python strings and the concepts underlying string formatting. This will provide a solid foundation for mastering more advanced techniques.
Python Strings: The Basics
In Python, a string is a sequence of characters enclosed in quotes. You can use either single quotes ' '
or double quotes " "
to define a string. For example:
s = 'Hello, World!'
print(s)
# Output:
# 'Hello, World!'
In this example, 'Hello, World!'
is a string. Python treats the entire sequence of characters as a single unit.
String Formatting: The Role of Placeholders and Escape Sequences
When formatting strings in Python, you’ll often use placeholders and escape sequences.
Placeholders
Placeholders are symbols that you insert into strings to hold the place for some other values. In Python string formatting, the curly braces {}
are used as placeholders. For example:
name = 'Alice'
print('Hello, {}!'.format(name))
# Output:
# 'Hello, Alice!'
In this example, {}
is a placeholder that gets replaced with the value of the variable name
.
Escape Sequences
Escape sequences are special characters that you can insert into strings using a backslash \
. These characters have special meaning to Python and can be used to insert things like a newline (\n
), a tab (\t
), or even a backslash itself (\\
). For example:
s = 'Hello,\nWorld!'
print(s)
# Output:
# 'Hello,
# World!'
In this example, \n
is an escape sequence that inserts a newline into the string.
Understanding these fundamental concepts is key to mastering Python string formatting. With this foundation, you can start to explore more advanced formatting techniques and their applications.
Real-World Uses: Formatting Strings
Python string formatting is not just a theoretical concept; it has practical applications in various real-world scenarios. Let’s explore some of these applications.
String Formatting in File Handling
When working with files, you often need to generate dynamic file paths, format data for output, or parse data from input. Python’s string formatting comes in handy in these scenarios. For example, you can use f-strings to generate dynamic file paths:
folder = 'documents'
file = 'report.txt'
path = f'{folder}/{file}'
print(path)
# Output:
# 'documents/report.txt'
In this example, we’ve used an f-string to generate a dynamic file path. This can be particularly useful when working with multiple files or directories.
String Formatting in Data Processing
Python string formatting is also useful in data processing tasks. For example, you might need to format data for output to a CSV file, or parse data from a JSON file. In these scenarios, you can use the format()
function or f-strings to format your data:
name = 'Alice'
age = 25
row = f'{name},{age}\n'
print(row)
# Output:
# 'Alice,25
# '
In this example, we’ve used an f-string to format data for output to a CSV file. The comma ,
is used as a delimiter, and the newline \n
is used to indicate the end of the row.
Further Resources for Python String Formatting Mastery
Mastering Python string formatting is a journey, and there’s always more to learn. Here are some resources that can help you continue your learning:
- Mastering Python Strings for Effective Coding: Enhance your coding skills with this deep dive into Python string handling, offering advanced tips and tricks for effective string manipulation.
IOFlood’s Tutorial on Python String Formatting: This tutorial dives into the topic of string formatting in Python.
IOFlood’s Guide to Python String Methods: This comprehensive guide explores various built-in string methods in Python.
Python’s official documentation on string formatting: A comprehensive guide to Python’s built-in string formatting capabilities.
Real Python’s guide to f-strings: A detailed tutorial on f-strings, one of the most powerful string formatting tools in Python.
PyBites’ article on Python string formatting: A collection of practical examples and exercises to help you master Python string formatting.
By understanding the practical applications of Python string formatting and continuing to learn and practice, you can become a Python string formatting expert.
Recap: String Formatting
In this guide, we’ve explored the ins and outs of Python string formatting, from the basics to advanced techniques.
We’ve learned how to use the format()
function and f-strings, two of the most common methods for formatting strings in Python. We’ve also delved into some alternative approaches, including the %
operator and template strings, and discussed when to use each method.
Along the way, we’ve covered some common issues you might encounter when formatting strings in Python and how to troubleshoot them. Whether it’s providing fewer arguments than there are placeholders, or incorrectly using the curly braces {}
, understanding these issues can help you write more robust code.
Finally, we’ve looked at some real-world applications of Python string formatting, from file handling to data processing. These examples demonstrate the practical value of mastering Python string formatting.
Here’s a comparison of the methods we’ve discussed:
Method | Readability | Flexibility | Security |
---|---|---|---|
format() function | High | High | Low |
F-strings | Very High | Very High | Low |
% operator | Low | Medium | Low |
Template strings | Medium | Low | High |
Each method has its strengths and weaknesses, and the best one to use will depend on your specific needs. But with a solid understanding of these techniques, you’re well on your way to becoming a Python string formatting expert.
Remember, mastering Python string formatting is a journey. Keep learning, keep practicing, and don’t be afraid to dive deeper.