Python String Methods: Ultimate Functions Guide
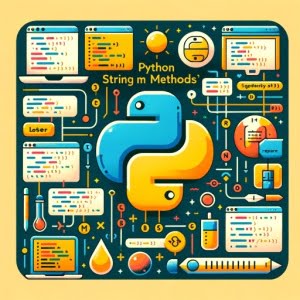
Are you finding it challenging to understand Python’s string methods? You’re not alone. Many developers, especially beginners, find themselves puzzled when it comes to manipulating and analyzing text data in Python. Think of Python’s string methods as a Swiss army knife – versatile and handy for various tasks such as, dealing with user input, parsing data from a file, or even debugging.
In this guide, we’ll walk you through the most commonly used string methods in Python, from the basics to more advanced techniques. We’ll cover everything from the lower()
, upper()
, split()
, to replace()
functions, and even some alternative approaches. So, whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you.
Let’s get started!
TL;DR: How Do I Use Python String Methods?
Python offers a variety of string methods such as
lower()
,upper()
,split()
,replace()
, and many more that allow you to manipulate and analyze text data effectively.
Here’s a simple example:
text = 'Hello, World!'
print(text.lower())
print(text.split(','))
# Output:
# 'hello, world!'
# ['Hello', ' World!']
In this example, we’ve used the lower()
method to convert all the characters in the string to lowercase, and the split()
method to divide the string into a list where each word is a separate element.
This is just the tip of the iceberg when it comes to Python’s string methods. Keep reading for a more detailed exploration and advanced usage scenarios.
Table of Contents
Python String Methods: The Basics
Python’s string methods are powerful tools that can make your coding tasks a lot simpler. Let’s start with some of the most basic yet essential string methods.
The lower()
and upper()
Methods
The lower()
method converts all uppercase characters in a string to lowercase, and the upper()
method does the opposite – it converts all lowercase characters to uppercase.
Here’s a simple example:
my_string = 'Hello, Python!'
print(my_string.lower())
print(my_string.upper())
# Output:
# 'hello, python!'
# 'HELLO, PYTHON!'
In the above example, lower()
and upper()
methods are used to change the case of the string. It’s important to note that these methods do not modify the original string but return a new one.
The split()
Method
The split()
method divides a string into a list where each word is a separate element. By default, this method splits at each space.
my_string = 'Hello, Python!'
print(my_string.split())
# Output:
# ['Hello,', 'Python!']
In the above code, split()
method is used to split the string into a list of words.
The replace()
Method
The replace()
method replaces a specified phrase with another specified phrase.
my_string = 'Hello, Python!'
print(my_string.replace('Python', 'World'))
# Output:
# 'Hello, World!'
In the above code, replace()
method is used to replace ‘Python’ with ‘World’. Again, it’s worth noting that this method doesn’t change the original string but returns a new string.
These are just a few examples of Python’s basic string methods. Each of these methods can be incredibly useful in different scenarios, from formatting text to parsing data. However, keep in mind that these methods return new strings, and the original string remains unchanged. This is because strings in Python are immutable – they can’t be changed after they’re created.
Advanced Python String Methods
After mastering the basics, it’s time to explore some of Python’s more advanced string methods. These methods provide additional functionality and can handle more complex tasks.
The join()
Method
The join()
method is a string method that concatenates all the elements in an iterable (like a list or a tuple), separated by the string it was called on.
my_list = ['Hello', 'Python']
print(' '.join(my_list))
# Output:
# 'Hello Python'
In the above example, the join()
method is used to concatenate the words in the list with a space (‘ ‘) in between.
The find()
Method
The find()
method returns the index of the first occurrence of the specified value. If the value is not found, the method returns -1.
my_string = 'Hello, Python!'
print(my_string.find('Python'))
# Output:
# 7
In the above code, find()
method is used to find the position of ‘Python’ in the string.
The startswith()
and endswith()
Methods
These methods are used to check if a string starts or ends with a specified value, respectively. Both methods return a boolean value.
my_string = 'Hello, Python!'
print(my_string.startswith('Hello'))
print(my_string.endswith('World'))
# Output:
# True
# False
In the above example, startswith()
method returns True because the string starts with ‘Hello’, and endswith()
method returns False because the string does not end with ‘World’.
These advanced Python string methods allow for more complex manipulations and checks on strings. They can be extremely useful in various scenarios, such as parsing more complex data, performing checks on strings, or formatting strings in more advanced ways.
Alternative Ways to Manipulate Strings
While Python’s built-in string methods are powerful and versatile, there are alternative ways to manipulate strings that can offer more flexibility or efficiency, especially when dealing with more complex tasks.
Regular Expressions
Regular expressions, or regex, is a sequence of characters that forms a search pattern. They can be used to check if a string contains a specified pattern.
import re
my_string = 'Hello, Python!'
match = re.search('Python', my_string)
if match:
print('Match found!')
else:
print('No match found.')
# Output:
# Match found!
In the above example, the re.search()
function is used to search for the pattern ‘Python’ in the string. If the pattern is found, ‘Match found!’ is printed; otherwise, ‘No match found.’ is printed.
Text Processing with Pandas
Pandas is a third-party library in Python that provides powerful data structures for data analysis and manipulation. It includes methods for string processing which can be especially useful when working with large datasets.
import pandas as pd
data = pd.Series(['Hello, Python!', 'Hello, World!'])
print(data.str.lower())
# Output:
# 0 hello, python!
# 1 hello, world!
# dtype: object
In the above example, the str.lower()
method is used to convert all characters in the Pandas Series to lowercase.
While these alternative approaches can handle more complex tasks, they can also be more complex to use and may require additional learning. Regular expressions, for example, have their own syntax that can be tricky to master. And while Pandas is a powerful tool for data manipulation, it may be overkill for simple string processing tasks. It’s important to choose the right tool for the job, considering both the task at hand and your comfort level with the tools available.
Troubleshooting String Methods
While Python string methods are incredibly useful, they can sometimes lead to unexpected issues. Here, we’ll discuss some common problems you might encounter and how to resolve them.
Handling Type Errors
Type errors can occur when you try to use a string method on a non-string data type. For instance:
num = 123
print(num.lower())
# Output:
# AttributeError: 'int' object has no attribute 'lower'
In the above example, we’re trying to use the lower()
method on an integer, which leads to a AttributeError
. To avoid this, ensure that the data type you’re working with is indeed a string.
Dealing with Index Errors
An IndexError
can occur when you try to access an index that does not exist in the string. For example:
my_string = 'Hello'
print(my_string[10])
# Output:
# IndexError: string index out of range
In this example, we’re trying to access the character at index 10 in a string that only has 5 characters, leading to an IndexError
. Always ensure that the index you’re trying to access exists within the range of the string.
Understanding Immutability
Strings in Python are immutable, meaning they can’t be changed after they’re created. This is why methods like replace()
, lower()
, and upper()
return new strings and do not modify the original string. Attempting to change a string directly can lead to a TypeError
:
my_string = 'Hello'
my_string[0] = 'h'
# Output:
# TypeError: 'str' object does not support item assignment
In this example, we’re trying to change the first character of the string, which results in a TypeError
. Instead of trying to change the string directly, use string methods to create a new string with the desired changes.
Fundamentals of Python Strings
Before diving deeper into Python’s string methods, it’s crucial to understand the fundamentals of Python strings and the concept of immutability.
What are Python Strings?
In Python, a string is a sequence of characters enclosed in single quotes, double quotes, or triple quotes. It’s one of the basic data types in Python and is used to handle text data.
my_string = 'Hello, Python!'
print(my_string)
# Output:
# 'Hello, Python!'
In the above example, ‘Hello, Python!’ is a string. We can print it out to the console using the print()
function.
Immutability of Python Strings
One of the key characteristics of Python strings is that they are immutable. This means that once a string is created, it cannot be changed. Any operation that appears to modify a string will instead create a new string.
my_string = 'Hello, Python!'
new_string = my_string.replace('Python', 'World')
print(my_string)
print(new_string)
# Output:
# 'Hello, Python!'
# 'Hello, World!'
In the above example, the replace()
method doesn’t change my_string
. Instead, it creates a new string new_string
where ‘Python’ is replaced with ‘World’.
This characteristic is why methods like replace()
, lower()
, and upper()
return new strings and do not modify the original string. Understanding this concept is crucial when working with Python string methods.
Use Cases of Python String Methods
Python string methods are not just useful for basic string manipulation. They are also powerful tools for more advanced tasks in data cleaning, web scraping, natural language processing (NLP), and more.
String Methods in Data Cleaning
Data cleaning is a crucial step in any data analysis process. Python string methods like strip()
, replace()
, and split()
can be used to clean and format your data, making it ready for analysis.
Web Scraping with Python String Methods
When scraping data from the web, you often end up with a large amount of text data that needs to be cleaned and formatted. Python string methods can help parse this data, extract useful information, and discard unnecessary content.
Natural Language Processing (NLP) and Python String Methods
In NLP, text data is analyzed to understand human language. Python string methods can be used to preprocess and clean this data, such as removing punctuation, converting to lowercase, and more.
Exploring Related Concepts: Regular Expressions, Unicode, and Encoding
If you’re interested in further expanding your skills, consider exploring related concepts like regular expressions for advanced pattern matching, Unicode for handling international characters, and different encoding schemes for storing strings.
Further Resources for Mastering Python String Methods
If you’re looking to dive deeper into Python string methods and related topics, here are some resources to explore:
- The Art of String Manipulation in Python: Explore the art of string manipulation in Python, learning about various methods and practices to handle textual data efficiently.
Tutorial on Python String Formatting: This tutorial by IOFlood provides a comprehensive overview of string formatting, including examples and explanations.
Guide on Python Multiline String: IOFlood’s guide explores how to work with multiline strings in Python.
Python’s official documentation on string methods: A comprehensive guide to all the string methods available in Python.
Python for Data Analysis: This book provides an in-depth look at how Python can be used for data analysis, including the use of string methods.
Natural Language Processing with Python: This online book provides a comprehensive introduction to NLP, including how to use Python string methods in the context of NLP.
Wrap Up: Python String Methods
In this comprehensive guide, we’ve delved into the world of Python string methods, exploring how to manipulate and analyze text data in Python effectively.
We began with the basics, learning how to use fundamental string methods like lower()
, upper()
, split()
, and replace()
. We then broadened our understanding, exploring more advanced methods such as join()
, find()
, startswith()
, and endswith()
. Along the way, we provided practical code examples to illustrate the usage of each method.
We tackled common challenges you might face when working with Python string methods, such as type errors and index errors, and provided solutions to these problems. We also delved into the concept of string immutability in Python, explaining why methods like replace()
, lower()
, and upper()
return new strings instead of modifying the original string.
Additionally, we explored alternative approaches to string manipulation, including the use of regular expressions and the Pandas library, providing you with a broader perspective on handling text data in Python.
Here’s a quick comparison of the methods we’ve discussed:
Method | Complexity | Use Case |
---|---|---|
Python String Methods | Low to Moderate | Basic to Intermediate String Manipulation |
Regular Expressions | High | Advanced Pattern Matching |
Pandas Library | High | Large-scale Data Analysis |
Whether you’re a beginner just starting out with Python or an experienced developer looking to refine your text manipulation skills, we hope this guide has given you a deeper understanding of Python string methods and their capabilities.
With their balance of simplicity, versatility, and power, Python string methods are an essential tool in any Python programmer’s toolkit. Happy coding!