Python String Formatting: Methods, Tips, Examples
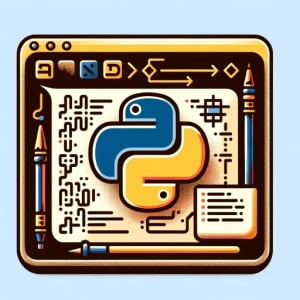
Are you struggling with string formatting in Python? It can feel like trying to tailor a suit without knowing how to thread a needle. But don’t worry, Python offers several tools to ‘tailor’ your strings to fit your needs.
In this guide, we’ll teach you how to use the tools and techniques needed to master string formatting in Python. We’ll cover Python Operators like %
, the str.format()
method, ‘f-strings’ and even some alternative approaches.
So, let’s thread the needle and start tailoring your Python strings to perfection.
TL;DR: How Do I Format Strings in Python?
Python provides several ways to format strings, but the most modern and recommended way is using
f-strings
, with the syntaxprint(f'Sample Text
). Here’s a simple example:
name = 'John'
age = 30
print(f'My name is {name} and I am {age} years old.')
# Output:
# 'My name is John and I am 30 years old.'
In this example, we’ve used an f-string to insert the variables name
and age
into a string. The f
before the string tells Python to look for variables in curly braces {}
and replace them with their values. This is a quick and readable way to format strings in Python.
For more advanced string formatting techniques, continue reading this guide. We’ll dive into other methods like the
%
operator and thestr.format()
method, and discuss when and why you might want to use them.
Table of Contents
The Basics of String Formatting
When it comes to Python string formatting, the %
operator and the str.format()
method are the first tools you need to know. They’re like the scissors and measuring tape in your Python string formatting toolkit.
The %
Operator
The %
operator is one of the oldest ways to format strings in Python. It’s a bit like a placeholder, standing in for the values you want to insert into your string. Here’s how it works:
name = 'John'
age = 30
print('My name is %s and I am %d years old.' % (name, age))
# Output:
# 'My name is John and I am 30 years old.'
In this example, %s
and %d
are placeholders for a string and a decimal (integer) respectively. The values in the parentheses after the %
operator replace these placeholders in the order they appear.
The %
operator is straightforward and easy to use, but it can get confusing if you have many variables to format or if your variables aren’t in the right order.
The str.format()
Method
The str.format()
method is a more modern way to format strings in Python. It’s more flexible and intuitive than the %
operator, especially when you’re dealing with multiple variables. Here’s how you use it:
name = 'John'
age = 30
print('My name is {} and I am {} years old.'.format(name, age))
# Output:
# 'My name is John and I am 30 years old.'
In this example, the curly braces {}
are placeholders for the variables, which are passed into the format()
method in the order they should appear in the string. If you want to specify the order of the variables, you can use positional arguments:
print('I am {1} years old. My name is {0}.'.format(name, age))
# Output:
# 'I am 30 years old. My name is John.'
The str.format()
method is more readable and flexible than the %
operator, but it can still get a bit unwieldy with many variables or complex string formats. That’s where more advanced string formatting techniques come in, which we’ll cover in the next section.
Advanced Python String Formatting
As your Python skills progress, you’ll need more advanced string formatting tools. That’s where f-strings and template strings come in. They’re like the sewing machine and serger in your Python string formatting toolkit, helping you stitch together more complex strings with ease.
F-Strings
F-strings, introduced in Python 3.6, are a modern and powerful way to format strings. They’re called f-strings because you prefix your string with the letter ‘f’. Here’s an example:
name = 'John'
age = 30
print(f'My name is {name} and I am {age} years old.')
# Output:
# 'My name is John and I am 30 years old.'
In this example, the variables name
and age
are placed directly inside the string in curly braces {}
. Python automatically replaces these with their values. This makes f-strings very readable and easy to use, even with many variables or complex string formats.
You can also perform operations inside the curly braces in f-strings, like this:
print(f'In five years, I will be {age + 5} years old.')
# Output:
# 'In five years, I will be 35 years old.'
Here, Python is adding 5 to the age variable inside the f-string. This ability to perform operations directly inside the string makes f-strings very powerful and flexible.
Template Strings
Template strings are a less commonly used method of string formatting in Python, but they can be useful in certain situations. They’re part of the string module and provide a simpler, more limited form of string formatting, like this:
from string import Template
name = 'John'
age = 30
t = Template('My name is $name and I am $age years old.')
print(t.substitute(name=name, age=age))
# Output:
# 'My name is John and I am 30 years old.'
In this example, the $
sign is used to denote variables in the template string. The substitute()
method is then used to replace these with their values. Template strings are less flexible than other string formatting methods, but they can be useful for handling user-generated strings because they avoid potential security issues with eval()
and exec()
functions.
In the next section, we’ll cover some additional methods to format strings in Python, including using external libraries and built-in functions.
Alternate Methods for Python Strings
Python’s versatility doesn’t stop at f-strings and template strings. There are additional methods to format strings in Python, including the use of external libraries and built-in functions. These tools are like the fine details that turn a well-tailored suit into a masterpiece.
Using External Libraries for String Formatting
Python’s vast ecosystem of external libraries includes some that can help with string formatting. For instance, the popular format
library offers a powerful, flexible, and readable way to format strings. Here’s an example:
from format import format
name = 'John'
age = 30
print(format('My name is {name} and I am {age} years old.', name=name, age=age))
# Output:
# 'My name is John and I am 30 years old.'
In this example, format
works much like str.format()
, but with some added features. For instance, it supports both positional and keyword arguments, and can format complex data types like lists and dictionaries.
Built-in Functions for String Formatting
Python also has built-in functions that can help with string formatting. For instance, the join()
method is a versatile way to concatenate strings, especially when you’re dealing with lists of strings. Here’s an example:
words = ['Hello', 'World']
print(' '.join(words))
# Output:
# 'Hello World'
In this example, join()
concatenates the strings in the words
list with a space ' '
in between. This can be very useful when you’re dealing with dynamic data or large lists of strings.
Remember, Python’s string formatting tools are like a tailor’s toolkit. Each tool has its strengths and weaknesses, and the key is to choose the right tool for the job. Whether you’re a beginner or an expert, understanding these tools will help you tailor your Python strings to perfection.
Solving Errors with Python Strings
Even the best tailors encounter snags and snarls. When working with Python string formatting, you might also encounter some common issues. But don’t worry, we’ve got the solutions to help you unravel these knots.
Issue: Mixing Data Types
One common issue arises when you try to concatenate or format a string with a non-string data type. Python raises a TypeError
in this case. Here’s an example:
age = 30
print('I am ' + age + ' years old.')
# Output:
# TypeError: can only concatenate str (not "int") to str
In this example, Python raises a TypeError
because it can’t concatenate a string (‘I am ‘) with an integer (age).
Solution
The solution is to convert the non-string data type to a string using the str()
function, like this:
print('I am ' + str(age) + ' years old.')
# Output:
# 'I am 30 years old.'
Issue: Incorrect String Formatting Syntax
Another common issue is using the incorrect syntax for string formatting. This can lead to unexpected results or errors. For instance, forgetting the ‘f’ in an f-string or using the wrong type of brackets can cause problems.
Solution
The solution is to double-check your string formatting syntax. Make sure you’re using the correct type of brackets, and don’t forget the ‘f’ in f-strings.
Issue: Index Errors with Positional Formatting
When using positional formatting with the str.format()
method or the %
operator, you might encounter index errors if you have more placeholders than variables, or vice versa. Python will raise an IndexError
in this case.
Solution
The solution is to make sure you have the same number of placeholders and variables. Double-check your string and your variables to make sure everything matches up.
Remember, troubleshooting is part of the process of mastering Python string formatting. With these tips and solutions, you’ll be able to handle any snags you encounter along the way.
Understanding Strings in Python
To truly master Python string formatting, it’s essential to understand what a string is and how it’s represented in Python.
What is a String?
In Python, a string is a sequence of characters. It’s a type of data that’s used to represent text. You can create a string by enclosing characters in either single quotes ' '
, double quotes " "
, or triple quotes for multiline strings ''' '''
or """ """
. Here’s an example:
s = 'Hello, World!'
print(s)
# Output:
# 'Hello, World!'
In this example, s
is a string that contains the text ‘Hello, World!’.
How is a String Represented in Python?
In Python, a string is an object of the built-in str
class. It’s represented as a sequence of Unicode characters, which means it can include any letter, number, symbol, or special character.
You can access the characters in a string by their index, like this:
s = 'Hello, World!'
print(s[0])
# Output:
# 'H'
In this example, s[0]
returns the first character in the string s
.
Understanding what a string is and how it’s represented in Python is the first step to mastering Python string formatting. With this foundation, you’ll be better equipped to understand and use the different string formatting techniques in Python.
Usage in Larger Python Projects
Python string formatting isn’t just about making your code look pretty. It’s a crucial tool for managing and manipulating data in larger Python projects. Whether you’re working with user input, file data, or API responses, knowing how to format strings effectively can save you time and headaches.
Exploring Related Concepts: String Manipulation and Regular Expressions
Once you’ve mastered string formatting, you might want to explore related concepts like string manipulation and regular expressions.
String manipulation involves altering or transforming strings. This could mean changing the case of a string, replacing parts of a string, or splitting a string into a list of substrings. Here’s an example of string manipulation in Python:
s = 'Hello, World!'
s_upper = s.upper()
print(s_upper)
# Output:
# 'HELLO, WORLD!'
In this example, the upper()
method is used to convert the string s
to uppercase.
Regular expressions, or regex, are a powerful tool for matching patterns in strings. They can be a bit complex to learn, but they’re incredibly useful for tasks like data validation, data extraction, and complex string manipulation. Here’s a simple example of using regex in Python:
import re
s = 'Hello, World!'
match = re.search('World', s)
print(match.group())
# Output:
# 'World'
In this example, the re.search()
function is used to find the word ‘World’ in the string s
.
Further Resources for Mastering Python String Formatting
Ready to dive deeper into Python string formatting? Here are a few resources to help you on your journey:
- Python Strings Explained: A Comprehensive Guide: This guide provides a thorough understanding of Python strings, covering everything from basic operations to advanced string manipulation techniques.
Guide on New Line in Python: Uses and Examples: IOFlood’s guide explores various scenarios where you might need to use new lines in Python and provides examples to demonstrate different ways of achieving new lines.
Formatting Strings in Python: This tutorial dives into the topic of string formatting in Python and provides examples and comparisons to help you understand how to format strings effectively.
A tutorial on advanced string formatting techniques: These resources offer in-depth tutorials and examples to help you master Python string formatting and related concepts. So go ahead, dive in and start tailoring your Python strings to perfection.
Python String Formatting: A Comprehensive Guide: A Real Python guide that covers various techniques and options for string formatting.
Python String Formatting: Official Documentation: The official documentation from Python that provides a detailed explanation of string formatting syntax and usage.
Recap: String Formatting Methods
Mastering Python string formatting is akin to acquiring a superpower. It’s a crucial skill that can help you manipulate and present data effectively in your Python projects.
We’ve covered a range of methods to format strings in Python, from the basic %
operator and str.format()
method to advanced techniques like f-strings and template strings. We’ve also explored alternative methods like external libraries and built-in functions, and discussed common issues and their solutions.
Here’s a quick comparison of the different string formatting methods we’ve covered:
Method | Example | Pros | Cons |
---|---|---|---|
% Operator | 'My name is %s' % name | Simple, easy to use | Can get confusing with many variables |
str.format() Method | 'My name is {}'.format(name) | Flexible, intuitive | Can get unwieldy with many variables |
F-strings | f'My name is {name}' | Readable, powerful | Requires Python 3.6 or higher |
Template Strings | Template('My name is $name').substitute(name=name) | Avoids security issues | Less flexible |
Remember, the key is to choose the right tool for the job. Each method has its strengths and weaknesses, and the best choice depends on your specific needs and the complexity of your string formatting task.
We hope this guide has given you a solid foundation in Python string formatting. With these tools in your toolkit, you’re well equipped to tailor your Python strings to perfection. Happy coding!