Python’s ‘zfill()’ Method | Guide To Padding Numeric Strings
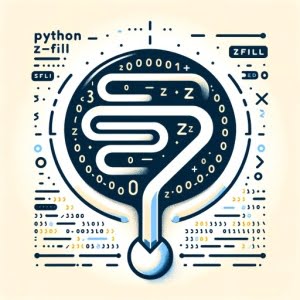
Are you finding it difficult to manage leading zeros in your Python numeric strings? You’re not alone. Many developers find themselves in a similar situation, but there’s a method in Python that can make this task a breeze.
Think of Python’s zfill method as a diligent bookkeeper, efficiently managing your numeric strings. It pads your numeric strings with leading zeros, ensuring they have the desired length.
In this guide, we’ll walk you through the ins and outs of the zfill method in Python. We’ll cover everything from basic usage to more advanced scenarios, as well as alternative approaches and common issues you might encounter.
So, let’s dive in and start mastering Python’s zfill method!
TL;DR: How Do I Use the zfill Method in Python?
The
zfill()
method in Python is used to pad a numeric string on the left with zeros, likeprint(num.zfill(3))
. It’s a handy tool when you need to ensure your numeric strings are of a certain length, regardless of the actual number they represent.
Here’s a basic example:
num = '7'
print(num.zfill(3))
# Output:
# '007'
In this example, we have a string ‘7’ that we want to pad with zeros until it’s three characters long. We use the zfill method with the argument 3, and it returns ‘007’, which is exactly what we want.
This is just a quick introduction to Python’s zfill method. Keep reading for a more in-depth look at how to use it, including advanced scenarios and alternative approaches.
Table of Contents
Python zfill: A Beginner’s Guide
Python’s zfill method is a string method that pads numeric strings on the left with zeros until they reach a desired length. It’s an essential tool for handling numeric strings in Python, especially when you need to ensure your strings are of a certain length.
Consider the following example:
num = '42'
print(num.zfill(5))
# Output:
# '00042'
In this example, we have a string ’42’ that we want to pad with zeros until it’s five characters long. We use the zfill method with the argument 5, and it returns ‘00042’. The number ’42’ is now a string of five characters with leading zeros.
This is a basic usage of the Python zfill method, but it’s a powerful tool that can make your code cleaner and more efficient. However, there are a few things you need to be aware of when using this method:
- The zfill method only works with string objects. If you try to use it with a non-string object, Python will raise a TypeError.
- If the string is already longer than the specified length, zfill will return the original string unchanged.
- The zfill method doesn’t modify the original string. Instead, it returns a new string with the padded zeros. This is because strings in Python are immutable, meaning they can’t be changed after they’re created.
Understanding these nuances can help you use the Python zfill method more effectively and avoid potential pitfalls.
Python zfill: Handling Complex Scenarios
As you become more comfortable with Python’s zfill method, you’ll find it can handle a variety of scenarios beyond simple string padding. One such case is dealing with negative numbers.
Consider the following example:
num = '-42'
print(num.zfill(6))
# Output:
# '-00042'
In this example, we have a string ‘-42’ that we want to pad with zeros until it’s six characters long. We use the zfill method with the argument 6, and it returns ‘-00042’. The number ‘-42’ is now a string of six characters with leading zeros.
Notice how the negative sign is preserved and the zeros are added after the sign. This is a key feature of the zfill method: it respects the sign of the number when adding leading zeros.
This is just one example of how Python’s zfill method can handle more complex scenarios. As you explore further, you’ll find it’s a versatile tool that can meet a variety of string padding needs.
Python String Padding: Beyond zfill
While Python’s zfill method is a powerful tool for padding numeric strings, it’s not the only solution. There are other methods you can use, such as the format
method or even creating custom functions. Let’s explore these alternatives.
Python’s Format Method for String Padding
Python’s format
method is a versatile tool that can be used for a wide range of string formatting tasks, including padding numeric strings. Here’s an example:
num = '42'
formatted_num = '{:0>5}'.format(num)
print(formatted_num)
# Output:
# '00042'
In this example, we use the format
method to pad the string ’42’ with zeros until it’s five characters long. The {:0>5}
is a format specification that tells Python to pad the string with zeros (‘0’) on the left (‘>’) until it’s five characters long.
Custom Functions for String Padding
If you need more control over your string padding, you might consider creating a custom function. Here’s an example:
def pad_string(s, length):
return '0' * (length - len(s)) + s
num = '42'
padded_num = pad_string(num, 5)
print(padded_num)
# Output:
# '00042'
In this example, we create a function pad_string
that takes a string s
and a desired length. It calculates the number of zeros needed by subtracting the length of s
from the desired length, then creates a string of that many zeros and concatenates it with s
.
Each of these methods has its advantages and disadvantages. The zfill method is simple and straightforward, but it only works with strings and doesn’t offer much flexibility. The format
method is more versatile, but its syntax can be complex for beginners. A custom function offers the most flexibility, but it requires more code and may not be as efficient as the built-in methods.
In the end, the best method depends on your specific needs and preferences. Experiment with each method to find the one that works best for you.
Python zfill: Common Issues and Solutions
While Python’s zfill method is a powerful tool, it’s not without its quirks. Here are some common issues you might encounter when using it, along with solutions and workarounds.
Dealing with Non-Numeric Strings
One common issue is trying to use the zfill method with non-numeric strings. Since zfill is designed to work with numeric strings, it might not behave as expected when used with strings that contain non-numeric characters.
For example, consider the following code:
s = 'abc'
print(s.zfill(5))
# Output:
# '00abc'
In this case, the zfill method still pads the string ‘abc’ with zeros until it’s five characters long. However, since ‘abc’ is not a numeric string, the result might not be what you expected.
To avoid this issue, always ensure that the string you’re passing to the zfill method is numeric. You can do this by using the isdigit
method, which checks if a string contains only digits.
Handling Strings That Are Already the Desired Length
Another issue you might encounter is when the string is already longer than the specified length. In this case, the zfill method will return the original string unchanged.
For example:
num = '12345'
print(num.zfill(3))
# Output:
# '12345'
In this case, the string ‘12345’ is already longer than three characters, so the zfill method returns it unchanged. To avoid this issue, always ensure that the length you’re passing to the zfill method is greater than the length of the string.
By understanding these common issues and how to avoid them, you can use Python’s zfill method more effectively and write cleaner, more efficient code.
Python String Methods: Manipulation and Formatting
Python provides a robust set of string methods that allow you to manipulate and format strings in a variety of ways. These methods can be applied directly to any string object, offering a level of flexibility and power that makes Python a popular choice for text processing tasks.
One such method is zfill()
, which we’ve been exploring in detail. But to fully appreciate how zfill()
fits into Python’s string handling capabilities, it’s important to understand the fundamental concept of string methods in Python.
What are Python String Methods?
String methods are built-in functions in Python that perform specific operations on or with strings. They can do everything from changing the case of a string (upper()
, lower()
, capitalize()
) to stripping whitespace (strip()
, lstrip()
, rstrip()
), replacing substrings (replace()
), and yes, padding numeric strings with leading zeros (zfill()
).
Here’s an example of a few string methods in action:
s = ' Hello, World! '
print(s.lower())
print(s.strip())
print(s.replace('World', 'Python'))
# Output:
# ' hello, world! '
# 'Hello, World!'
# ' Hello, Python! '
In this example, we first convert the string to lowercase using the lower()
method, then remove leading and trailing whitespace with strip()
, and finally replace ‘World’ with ‘Python’ using replace()
.
How to Use Python String Methods
Python string methods are easy to use: you simply append the method to the string with a dot (.
), followed by the method name and any required arguments in parentheses. For example, s.lower()
calls the lower()
method on the string s
.
It’s important to note that string methods in Python do not change the original string. Instead, they return a new string that is the result of the operation. This is because strings in Python are immutable, meaning they can’t be changed after they’re created.
With this understanding of Python’s string methods, you can better appreciate how zfill()
fits into the picture. It’s just one of many tools in Python’s string handling toolkit, but as we’ve seen, it’s a powerful one that can make working with numeric strings much easier.
Python zfill: Beyond Numeric Strings
Python’s zfill method is a powerful tool for managing numeric strings, but its applications go beyond simple string padding. It’s also incredibly useful in areas like data processing and file handling.
Python zfill in Data Processing
In data processing, it’s common to encounter datasets where numeric values have inconsistent lengths. This can be a problem when you need to compare these values or use them as keys. Python’s zfill method can help by ensuring all numeric strings have the same length, making them easier to compare and process.
Python zfill in File Handling
When working with files, you might need to generate filenames that include numeric elements. For example, you might be generating a series of log files named ‘log1.txt’, ‘log2.txt’, etc. Using the zfill method, you can ensure these numeric elements have a consistent length, making your filenames easier to sort and manage.
Exploring Related Concepts
If you’re interested in diving deeper into Python’s string handling capabilities, there are a few related concepts you might want to explore. String formatting is a broad topic that includes not only padding strings with the zfill method, but also aligning text, controlling decimal places, and more. Regular expressions, on the other hand, offer a powerful way to search, match, and manipulate strings.
Further Resources for Python String Mastery
To help you continue your journey in mastering Python’s string handling capabilities, here are a few resources that you might find useful:
- Dive into Python Print: Core Concepts – Explore examples demonstrating the usage of the print() function for debugging and logging.
Uppercase in Python – Learn how to convert strings to uppercase in Python for uniform text representation.
Quick Guide: Joining in Python – Master Python join() functionality for efficient string construction and manipulation.
Python’s official documentation on string methods provides in-depth explanations of all string methods, including zfill.
Real Python’s guide to string formatting provides a comprehensive look at Python’s string formatting capabilities.
Python’s official documentation on Regular Expressions – If you’re ready to dive into regular expressions, this is the resource for you.
By understanding how zfill fits into Python’s broader string handling capabilities, and by exploring related concepts, you’ll be well on your way to mastering Python strings.
Wrapping Up: Mastering Python’s zfill Method
In this comprehensive guide, we’ve delved deep into the world of Python’s zfill method, a powerful tool for handling numeric strings.
We began with the basics, learning how to use the zfill method to pad numeric strings with leading zeros. We then explored more complex scenarios, such as dealing with negative numbers and non-numeric strings.
Along the way, we tackled common issues you might encounter when using the zfill method, providing you with solutions and workarounds for each issue.
We also ventured into the realm of alternative approaches, comparing Python’s zfill method with other string padding techniques, such as the format method and custom functions.
Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | Suitability for Numeric Strings |
---|---|---|---|
zfill | Low | Low | High |
format | High | Moderate | High |
Custom Function | High | High | High |
Whether you’re just starting out with Python’s zfill method or you’re looking to level up your string handling skills, we hope this guide has given you a deeper understanding of the zfill method and its capabilities.
With its simplicity and efficiency, Python’s zfill method is a powerful tool for handling numeric strings. Now, you’re well equipped to tackle any numeric string manipulation task that comes your way. Happy coding!