Creating NPM Build Scripts | Node.js Developer’s Guide
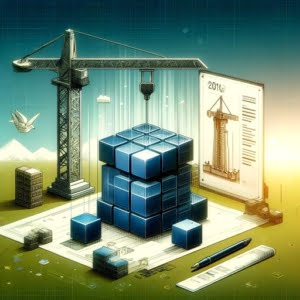
While working on Node.js servers At IOFLOOD, I’ve had to go through the tedious process of writing build scripts by hand. Over time I have been able implement optimized and automated build procedures as a common practice. To assist others facing similar challenges, I’ve crafted this comprehensive guide on npm build scripts! By delving into this guide, you’ll be able to handle tasks like compiling source code and optimizing assets with ease.
This guide will take you through the journey of mastering npm build, from understanding its basic usage to exploring advanced features and best practices. Whether you’re a beginner looking to automate your build process or an experienced developer aiming to optimize your workflow, this guide has something for everyone.
Let’s simplify project building together!
TL;DR: What is an npm Build Script?
npm build
runs a script defined in your project’spackage.json
file. You can create apackage.json
file by runningnpm init
. You can trigger your scripts with the command,npm run build
. Build scripts are typically used for compiling code or generating build artifacts.
Here’s a quick example of a build script:
"scripts": {
"build": "tsc && webpack"
}
# Output:
# Successfully compiled TypeScript files and bundled assets with Webpack.
Running npm run build
executes the defined command, compiling TypeScript files and bundling assets with Webpack.
In this example, we’ve defined a build
script in the package.json
that uses TypeScript compiler (tsc
) and Webpack for bundling. When you run npm run build
, it triggers the compilation of TypeScript files into JavaScript and then bundles them along with other assets using Webpack, preparing your project for production deployment.
Eager to master npm build and streamline your development process? Keep reading for a comprehensive guide on npm build’s capabilities and best practices.
Table of Contents
Automating Builds with npm
When you’re starting your journey in Node.js development, one of the first tools you’ll encounter is npm, the Node Package Manager. It’s not just for installing packages; it can also automate your build process, making your development workflow more efficient and reliable.
Creating a Simple Build Script
A build script in your package.json
file tells npm how to compile and prepare your project for production. Let’s create a basic script that compiles Sass files into CSS, making your styles ready for the web.
First, ensure you have a package.json
in your project. If not, create one by running npm init
and follow the prompts. Next, install the necessary package for compiling Sass:
npm install node-sass --save-dev
Now, add the following build script in your package.json
:
"scripts": {
"build-css": "node-sass --output-style compressed -o dist/css src/sass"
}
To execute this script, run:
npm run build-css
# Output:
# Compiled and compressed CSS in 'dist/css' directory.
This command compiles your Sass files located in src/sass
into compressed CSS files in the dist/css
directory. By automating this process, you save time and reduce the chance for errors, ensuring that your styles are consistently prepared for production.
The Power of npm Scripts
The beauty of npm scripts is their simplicity and flexibility. With just a few lines in your package.json
, you can automate a wide range of development tasks, from compiling code to deploying projects. This example is just the beginning. As you become more comfortable with npm, you’ll discover its full potential in streamlining your development workflow.
Elevating Your Build Process
As you become more comfortable with npm and its capabilities, it’s time to explore how you can leverage npm scripts for more complex build scenarios. This involves diving into advanced script configurations, parallel execution, and integrating npm with other powerful tools like Babel and Webpack.
Parallel Execution with npm
One way to speed up your build process is by running tasks in parallel. npm does not have built-in support for parallel execution, but you can achieve this by using the npm-run-all
package.
First, install npm-run-all
:
npm install npm-run-all --save-dev
Then, suppose you have separate scripts for building CSS and JavaScript. You can modify your package.json
to run these tasks in parallel:
"scripts": {
"build-css": "node-sass src/styles -o dist/styles",
"build-js": "webpack --config webpack.config.js",
"build": "npm-run-all --parallel build-css build-js"
}
Running npm run build
will now execute both the build-css
and build-js
scripts at the same time, significantly reducing your build time.
Environment-Specific Builds
Tailoring your build process to different environments (e.g., development, testing, production) can enhance your workflow. Here’s how you can set up environment-specific builds using npm scripts.
First, install cross-env
to easily set environment variables across platforms:
npm install cross-env --save-dev
Then, adjust your package.json
to include environment-specific build commands:
"scripts": {
"build:dev": "cross-env NODE_ENV=development webpack --config webpack.config.dev.js",
"build:prod": "cross-env NODE_ENV=production webpack --config webpack.config.prod.js"
}
By running npm run build:dev
or npm run build:prod
, you can ensure that your project is built using the appropriate settings for the target environment.
Integrating with Babel and Webpack
For projects that require transpiling ES6 or beyond, integrating Babel with your npm build process is essential. Similarly, Webpack can be used to bundle your modules and assets. Here’s a simplified setup:
First, ensure you have Babel and Webpack installed:
npm install babel-loader webpack --save-dev
Then, in your webpack.config.js
, include the Babel loader:
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['@babel/preset-env']
}
}
}
]
}
This configuration tells Webpack to use Babel for transpiling JavaScript files, ensuring your code is compatible across browsers. By integrating these tools into your npm build process, you can handle a wide range of development tasks, from transpiling and bundling to minifying and optimizing your code.
Exploring Beyond npm Build
While npm build
is a powerful tool in the Node.js ecosystem, there are scenarios where alternative tools and scripts might better suit your project’s needs. Let’s explore some of these alternatives, such as Gulp and Grunt, and understand how they can complement or replace your npm build process.
Gulp: Streamlined Workflows
Gulp is a popular task runner that uses Node.js streams for fast builds. It allows you to code your tasks in JavaScript, providing a high level of customization.
Here’s a basic example of a Gulp task to minify JavaScript files:
const gulp = require('gulp');
const uglify = require('gulp-uglify');
const rename = require('gulp-rename');
gulp.task('minify-js', function () {
return gulp.src('src/js/**/*.js')
.pipe(uglify())
.pipe(rename({ extname: '.min.js' }))
.pipe(gulp.dest('dist/js'));
});
# Output:
# Minified and renamed JS files in 'dist/js'.
This Gulp task searches for JavaScript files in src/js
, minifies them using gulp-uglify
, renames the output files to include .min.js
, and saves them in dist/js
. Gulp’s use of streams ensures this process is both fast and efficient.
Grunt: Configuration Over Code
Grunt is another task runner that emphasizes configuration over code. It uses a JSON-like file to define and manage tasks. This can make Grunt easier to grasp for those less familiar with programming.
Here’s how you could set up a Grunt task to lint JavaScript files:
module.exports = function(grunt) {
grunt.initConfig({
jshint: {
files: ['src/**/*.js'],
options: {
esversion: 6
}
}
});
grunt.loadNpmTasks('grunt-contrib-jshint');
grunt.registerTask('default', ['jshint']);
};
# Output:
# Linted JS files, with potential issues logged.
This configuration lints all JavaScript files in the src
directory, ensuring they adhere to the specified standards. It’s a simple yet powerful way to maintain code quality.
Choosing the Right Tool
Both Gulp and Grunt offer unique advantages and can be powerful alternatives or complements to npm scripts. The choice between them depends on your project’s specific needs and your comfort level with JavaScript coding versus configuration. In some cases, combining npm scripts with these tools can provide the best of both worlds, leveraging the simplicity of npm with the power and flexibility of Gulp or Grunt.
Even the most seasoned developers encounter bumps along the way when dealing with build scripts. Understanding how to troubleshoot common problems with npm build
can save you time and frustration. Let’s dive into some typical issues and how to resolve them.
Dealing with Script Failures
Script failures are a common headache. Often, they stem from missing dependencies or syntax errors in your build scripts. Here’s how you can diagnose a failing script:
npm run build
# Output:
# Error: Cannot find module 'some-module'
In this example, the build fails because a required module is missing. The solution is straightforward: install the missing module using npm.
npm install some-module --save-dev
After installing the missing dependency, running your build script should no longer throw the error. This example underscores the importance of ensuring all required modules are installed before executing your build scripts.
Solving Dependency Problems
Dependency issues can also manifest as version conflicts or corrupted package installations. If you’re encountering strange errors and suspect a dependency issue, try clearing npm’s cache and reinstalling your packages:
npm cache verify
npm install
This process will verify the cache’s integrity and reinstall all project dependencies, potentially resolving any conflicts or corruptions.
Overcoming Performance Bottlenecks
As projects grow, build times can increase, leading to productivity bottlenecks. One way to address this is by optimizing your build scripts. For instance, consider using the --parallel
flag with npm-run-all
to run multiple tasks simultaneously, as shown in the Advanced Use section.
Another approach is to minimize the work done by each build. For example, you can use incremental builds with tools like Webpack to only rebuild parts of your application that have changed since the last build:
module.exports = {
watch: true,
// Other Webpack configuration...
};
# Output:
# Webpack now rebuilds only changed modules.
This Webpack configuration enables the watch
mode, which monitors your files for changes and only rebuilds the necessary parts, significantly reducing build times for large projects.
Best Practices for Scalable Builds
To maintain scalable and efficient build scripts, adhere to the following best practices:
- Modularize your build scripts to keep them manageable as your project grows.
- Use environment variables to customize builds for different environments without changing the script.
- Regularly update dependencies to leverage performance improvements and bug fixes.
By anticipating common issues and employing best practices, you can ensure your npm build process is both robust and efficient, paving the way for smooth and successful project development.
The Bedrock of npm Build
In the vast landscape of web development, understanding the foundation upon which tools like npm build
stand is crucial. This section delves into the essentials of build processes, the pivotal role of npm in the JavaScript ecosystem, and how npm build
seamlessly integrates into the fabric of software development.
Why Build Scripts Matter
At its core, a build process involves compiling source code into a format that can be executed by a computer. In the context of web development, this often means transforming new JavaScript syntax and features into versions compatible with a wider range of browsers, minifying code to reduce file sizes, and bundling various modules together.
npm run build
# Output:
# Bundled and optimized project files.
In this example, npm run build
might be a command that triggers a series of tasks such as transpilation, minification, and bundling. The output indicates a successful transformation of the project files into an optimized version ready for production. This process is vital for enhancing performance, ensuring compatibility, and improving the overall user experience.
npm’s Role in JavaScript Development
npm, or Node Package Manager, is more than just a tool for installing packages. It’s a cornerstone of the JavaScript community, providing a vast repository of libraries and tools, and a powerful script runner that can automate almost any task in your development workflow.
"scripts": {
"build": "webpack --mode production"
}
Here, the build
script in package.json
uses Webpack to bundle JavaScript modules for production. This is a glimpse into how npm scripts can simplify complex tasks into single commands, making your development process more efficient.
Integrating npm Build into Software Development
npm build
isn’t just a standalone command; it’s part of a larger ecosystem that includes continuous integration/continuous deployment (CI/CD) pipelines, automated testing, and more. It fits into the broader picture by providing a standardized way to execute build tasks, ensuring that software is consistently compiled and tested across different environments and stages of development.
Understanding these fundamentals not only enriches your knowledge but also empowers you to leverage npm build
and related tools to their fullest potential, streamlining your development process and elevating the quality of your projects.
Expanding npm Build’s Horizons
As developers, understanding the tools at our disposal only marks the beginning of our journey. npm build
is a powerful ally in the realm of Node.js development, but its true potential is unlocked when integrated with broader development practices such as Continuous Integration/Continuous Deployment (CI/CD) pipelines, automated testing, and sophisticated deployment strategies.
CI/CD Pipelines and npm
Continuous Integration and Continuous Deployment (CI/CD) pipelines are crucial for modern software development practices. They ensure that your code is automatically tested and deployed, making the entire software development lifecycle smoother and more efficient.
Integrating npm build
into your CI/CD pipeline can be as simple as adding a step in your pipeline configuration that executes the build script:
steps:
- label: 'Build'
command: 'npm run build'
# Output:
# Successful execution indicates your project is built and ready for the next pipeline stage.
In this example, the CI/CD pipeline executes npm run build
as part of its steps, ensuring that the code is compiled and bundled according to the project’s requirements before moving on to testing or deployment. This automation reduces manual errors and ensures consistent builds across environments.
Automated Testing with npm
Automated testing is another critical aspect of modern web development. npm build
can be configured to not only compile your code but also to run tests automatically.
For instance, integrating Mocha or Jest for testing your Node.js applications can be achieved with npm scripts:
"scripts": {
"test": "mocha || jest"
}
Running npm test
after your build process ensures that your code meets the quality standards before it’s deployed, reinforcing the reliability of your application.
Deployment Strategies
Deployment is the final step in getting your application to your users. npm can play a role here too, by scripting deployment tasks that can range from simple server restarts to complex multi-stage deployment processes.
npm run deploy
# Output:
# Application successfully deployed to production environment.
This command might internally handle various tasks such as pushing the build artifacts to a server, restarting services, or even rolling back in case of errors, making the deployment process seamless and automated.
Further Resources for Mastering npm Build
To deepen your understanding and mastery of npm build
and its ecosystem, consider exploring the following resources:
- Node.js Official Documentation: A comprehensive guide to Node.js, including npm.
- Webpack Official Guide: Learn about module bundling and how it integrates with npm scripts.
- CI/CD with GitHub Actions: Discover how to automate your build and deployment processes using GitHub Actions.
These resources provide valuable insights and practical knowledge to further enhance your development workflows, making you proficient in not just npm build, but in the broader aspects of web development and deployment strategies.
Wrapping Up: Mastering npm Build
In our comprehensive journey, we’ve demystified the npm build
command, a cornerstone in the Node.js and JavaScript development realms. This exploration has equipped you with the knowledge to leverage npm build for compiling and preparing your projects for production, akin to a master chef meticulously prepping ingredients for a gourmet dish.
We began with the basics, learning how to define and execute a simple build script in your project’s package.json
. This foundational skill paves the way for automating your build process, making your development workflow both efficient and scalable.
Venturing further, we delved into advanced configurations and techniques. From parallel execution to environment-specific builds, we explored how to optimize your build process for complex projects. Integrating with tools like Babel and Webpack, we unlocked the potential for a highly customized and powerful build setup.
Beyond the confines of npm build, we investigated alternative tools and scripts that can enhance or replace your npm build process. Understanding the strengths and use cases of Gulp, Grunt, and other build systems empowers you to choose the right tool for your project’s needs.
Tool | Use Case | Flexibility |
---|---|---|
npm build | Compiling and bundling | High |
Gulp | Task running with streams | Very High |
Grunt | Configuration-based task running | High |
As we wrap up, remember that mastering npm build and its alternatives is more than just about executing commands. It’s about crafting a build process that’s efficient, scalable, and tailored to your project’s unique requirements. The knowledge and skills you’ve gained are a solid foundation, but the landscape of web development is ever-evolving. Continue to explore, experiment, and enhance your build processes with new tools and practices.
With a well-configured build process, you’re not just preparing code for production. You’re crafting an environment where creativity and efficiency flourish, ensuring your projects stand out in the modern web development landscape. Happy building!