Java Not Operator (!) | Basic and Advanced Uses
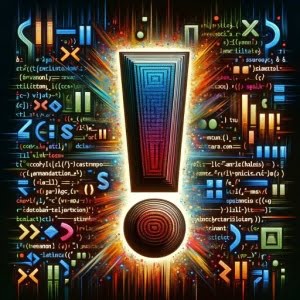
Mastering the use of the '!'
operator in Java is an important for creating conditional statements in our projects at IOFLOOD. This operator, also known as the logical complement operator, helps us reverse boolean expressions, enabling more sophisticated control flow in our programs. We plan to discuss this operator in-depth in today’s article, to help our dedicated server customers enhance their coding techniques.
In this guide, we’ll walk you through the usage of the ‘!’ operator in Java, from basic to advanced levels. We’ll cover everything from the basics of the logical complement operator to more advanced techniques, as well as alternative approaches.
Let’s get started and master the '!'
operator in Java!
TL;DR: What Does the ‘!’ Java Not Operator Do?
The
'!'
operator in Java, also known as thelogical complement operator
or thelogical NOT operator
, inverts the value of a boolean. This means it flips the boolean value from true to false, or from false to true.
Here’s a simple example:
boolean isTrue = true;
System.out.println(!isTrue);
// Output:
// false
In this example, we declare a boolean variable isTrue
and assign it the value true
. When we print the value of !isTrue
, it returns false
because the ‘!’ operator inverts the boolean value.
This is just a basic usage of the ‘!’ operator in Java. There’s much more to learn about this operator, including more complex uses and alternative approaches. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Flipping Booleans: Basic Use of ‘!’ in Java
- ‘!’ in Logical Expressions and Loops
- Alternative Ways to Invert Booleans in Java
- Troubleshooting ‘!’ Operator in Java
- Understanding Boolean Logic in Java
- Expanding the ‘!’ Operator’s Role in Larger Java Programs
- Wrapping Up: The Logical NOT (‘!’) Operator in Java
Flipping Booleans: Basic Use of ‘!’ in Java
The ‘!’ operator is a fundamental part of Java, especially when working with boolean values. Its primary function is to invert the value of a boolean. In other words, it flips true
to false
and false
to true
. This can be incredibly useful when you want to reverse a boolean condition without having to write lengthy code.
Let’s take a look at a simple example:
boolean isRaining = true;
if (!isRaining) {
System.out.println("Let's go for a picnic!");
} else {
System.out.println("We should stay indoors.");
}
// Output:
// We should stay indoors.
In the above code, we have a boolean variable isRaining
that is set to true
. The ‘if’ statement checks the inverse of isRaining
(which is false
, because ‘!’ inverts true
to false
). Therefore, the program prints ‘We should stay indoors.’
The ‘!’ operator is not just concise, but also enhances code readability and understanding. However, it’s important to remember that overusing the ‘!’ operator can sometimes lead to confusion, especially in complex conditions. It’s always a good idea to use parentheses to clearly indicate the logic when using the ‘!’ operator in more complex expressions.
‘!’ in Logical Expressions and Loops
As you become more comfortable with the ‘!’ operator in Java, you can start to use it in more complex scenarios, such as in conditional statements or loops.
Consider a scenario where you have a login system. You want to keep prompting the user for a password until they enter the correct one. Here’s how you can use the ‘!’ operator in a loop to achieve this:
String correctPassword = "secret";
String userInput;
do {
userInput = getUserInput();
} while (!userInput.equals(correctPassword));
// Output:
// (Depends on the user input)
In this code, the do-while
loop continues to ask for user input as long as the input does not match the correct password. The ‘!’ operator inverts the result of userInput.equals(correctPassword)
. If the user input matches the password, the expression becomes true
, but the ‘!’ operator flips it to false
, ending the loop.
This showcases the power of the ‘!’ operator in Java, allowing you to control the flow of your programs effectively. However, remember that clarity is key in programming. If the use of ‘!’ makes your code harder to understand, it might be better to rethink your approach.
Alternative Ways to Invert Booleans in Java
While the ‘!’ operator is a handy tool for inverting booleans in Java, it’s not the only way to achieve this. There are alternative methods that can accomplish the same task, and it’s important to understand these to make the best decision based on the context of your program.
Using Conditional Statements
One alternative is to use a conditional statement (an if-else
structure) to invert a boolean. Here’s an example:
boolean isRaining = true;
boolean isNotRaining;
if (isRaining) {
isNotRaining = false;
} else {
isNotRaining = true;
}
// Output:
// isNotRaining = false
In this code, we manually set the value of isNotRaining
based on isRaining
. If isRaining
is true
, isNotRaining
is set to false
, and vice versa. This approach provides the same end result as using the ‘!’ operator but can be more verbose and less efficient.
Using Ternary Operator
Another alternative is the ternary operator, which can be used to invert a boolean in a more concise way than an if-else
statement. Here’s how it works:
boolean isRaining = true;
boolean isNotRaining = isRaining ? false : true;
// Output:
// isNotRaining = false
The ternary operator (? :
) checks the condition isRaining
. If it’s true
, isNotRaining
is set to false
. If it’s false
, isNotRaining
is set to true
.
Each of these alternatives has its benefits and drawbacks. While using an if-else
statement or a ternary operator can be more explicit and easier to understand for some, they can also make your code more verbose. On the other hand, the ‘!’ operator is more concise but may be harder to understand for beginners. Deciding which method to use will depend on your specific situation and who will be reading your code.
Troubleshooting ‘!’ Operator in Java
While the ‘!’ operator is straightforward in its function, there can be pitfalls and common errors when using it, especially in complex logical expressions. Let’s explore a few and discuss how to solve them.
Misplacement of ‘!’ Operator
A common mistake is misplacing the ‘!’ operator, which can lead to unexpected results. Consider the following code:
boolean isRaining = true;
boolean isCold = false;
if (!isRaining && isCold) {
System.out.println("Let's go for a walk.");
} else {
System.out.println("Stay at home.");
}
// Output:
// Stay at home.
In this example, !isRaining && isCold
is false
because !isRaining
is false
and isCold
is false
, so the output is ‘Stay at home.’ But if you intended to check whether it’s not raining and not cold, the correct placement of ‘!’ should be !(isRaining && isCold)
.
Overuse of ‘!’ Operator
Overusing the ‘!’ operator can lead to code that’s difficult to read and understand. It’s often better to use positive conditions instead of negations. For example, instead of if (!isRaining && !isCold)
, consider using if (isSunny && isWarm)
.
Parentheses for Clarity
When using the ‘!’ operator with other logical operators (like &&
and ||
), use parentheses to make the logic clear. For example, !(isRaining && isCold)
is not the same as !isRaining && isCold
.
Remember, the key to using the ‘!’ operator effectively is to ensure clarity in your code. Use it when it simplifies your logic, but avoid it when it makes your code harder to understand.
Understanding Boolean Logic in Java
To fully grasp the ‘!’ operator’s functionality in Java, it’s crucial to understand boolean logic, the foundation of this operator.
In Java, a boolean is a data type that can only be either true
or false
. Boolean logic is a subset of algebra used for creating true/false statements. It’s named after George Boole, an English mathematician and logician who first defined an algebraic system of logic in the mid 19th century.
Boolean logic in Java is primarily used in control statements where a decision must be made based on a certain condition. For example:
boolean isRaining = true;
if (isRaining) {
System.out.println("Take an umbrella!");
} else {
System.out.println("Enjoy the weather!");
}
// Output:
// Take an umbrella!
In this example, the if
statement uses boolean logic to decide which statement to print. If isRaining
is true
, it prints ‘Take an umbrella!’. If isRaining
is false
, it prints ‘Enjoy the weather!’.
The ‘!’ operator plays a significant role in boolean logic by inverting the boolean value. Understanding this fundamental concept will make working with the ‘!’ operator in Java much more intuitive.
Expanding the ‘!’ Operator’s Role in Larger Java Programs
The ‘!’ operator in Java is not just for simple boolean flipping. It’s a versatile tool that can be crucial in larger Java programs, especially in control flow structures like loops and conditional statements.
Complementing Other Operators
The ‘!’ operator often works in tandem with other operators like &&
(and) and ||
(or) to form more complex logical expressions. For example, you might want to check if it’s not raining and not cold before going for a walk:
boolean isRaining = false;
boolean isCold = false;
if (!isRaining && !isCold) {
System.out.println("Perfect weather for a walk!");
}
// Output:
// Perfect weather for a walk!
In this example, the ‘!’ operator works with the &&
operator to check two conditions: it’s not raining, and it’s not cold.
Further Resources for Mastering Java Operators
To deepen your understanding of the ‘!’ operator and other Java operators, consider exploring the following resources:
- IOFlood’s Article on Java Operators explains Java’s increment and decrement operators for variable manipulation.
Java += Operator Explained – Discover how to use the “+=” operator in Java, used for additional assignment.
Java != Operator Overview – Learn about the “!=” operator in Java, used for checking if two operands are not equal.
Oracle Java Documentation – Comprehensive official documentation on Java operators.
GeeksforGeeks’ Java Operators Guide is a detailed page on Java operators with examples.
JavaTpoint’s Java Operators Tutorial includes precedence and associativity rules.
Wrapping Up: The Logical NOT (‘!’) Operator in Java
In this comprehensive guide, we’ve delved into the workings of the ‘!’ operator in Java, a key tool for inverting boolean values.
We began with the basics, understanding the primary function of the ‘!’ operator and how it can be used to flip boolean values. We then progressed to more advanced uses, exploring how the ‘!’ operator can be employed in logical expressions and loops to control the flow of your programs.
We also discussed alternative methods to invert booleans in Java, such as using conditional statements and the ternary operator, and provided a comparison to help you choose the best approach for your specific situation. Along the way, we addressed common errors and pitfalls when using the ‘!’ operator and offered solutions to help you avoid these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Conciseness | Readability | Efficiency |
---|---|---|---|
‘!’ Operator | High | Moderate | High |
Conditional Statements | Low | High | Moderate |
Ternary Operator | Moderate | High | High |
Whether you’re just starting out in Java or you’re an experienced developer looking to brush up on your skills, we hope this guide has helped you deepen your understanding of the ‘!’ operator in Java.
The ‘!’ operator is a powerful tool in Java, enabling you to write more efficient and concise code. Now, you’re well equipped to use the ‘!’ operator effectively in your Java programs. Happy coding!