Duck Typing in Python: The Pythonic Way
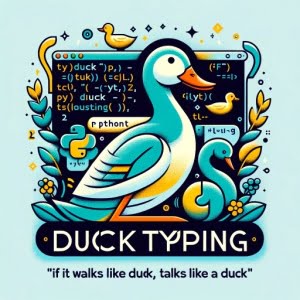
Ever scratched your head over the phrase ‘If it looks like a duck, swims like a duck, and quacks like a duck, then it probably is an unspecified variable type’? If so, you’ve probably been coding in Python quite a lot.
This phrase is more than just a quirky saying; it’s the essence of Duck Typing, a programming concept that Python embraces.
In this guide, we’ll walk you through the concept of duck typing in Python, from its basic use to advanced techniques. We’ll cover everything from the fundamentals of Duck Typing, its practical applications, as well as alternative approaches.
So, let’s dive in and start mastering Duck Typing with Python!
TL;DR: What is Duck Typing in Python?
Duck typing in Python is a programming concept where the type or the class of an object is less important than the methods it defines. When you use duck typing, you do not check types at all. Instead, you check for the presence of a given method or attribute.
Here’s a simple example:
class Duck:
def quack(self):
return 'Quack!'
class Person:
def quack(self):
return 'I'm Quacking Like a Duck!'
def in_the_forest(malard):
print(malard.quack())
donald = Duck()
john = Person()
in_the_forest(donald)
in_the_forest(john)
# Output:
# 'Quack!'
# 'I'm Quacking Like a Duck!'
In this example, we have a Duck
class and a Person
class, both with a method named quack
. In the function in_the_forest
, we don’t care about the type of the object passed in, as long as it has a quack
method. This is the essence of duck typing in Python.
But there’s much more to learn about duck typing, including its advantages, potential pitfalls, and advanced usage scenarios. Continue reading for a more detailed understanding.
Table of Contents
- Unpacking Duck Typing in Python: A Beginner’s Guide
- Duck Typing in Complex Scenarios: An Intermediate Guide
- Exploring Alternatives to Duck Typing in Python
- Troubleshooting Common Issues with Duck Typing in Python
- Python’s Dynamic Typing System and Duck Typing
- Duck Typing and Object-Oriented Programming
- Method Resolution in Python
- Duck Typing in Python: Real-World Applications
- Exploring Related Concepts
- Wrapping Up: Mastering Duck Typing in Python
Unpacking Duck Typing in Python: A Beginner’s Guide
Duck typing is a core concept in Python that revolves around an object’s behavior rather than its class or type. This means you’re more interested in what an object can do (i.e., the methods it has), rather than what it is (i.e., its class or type). It’s a unique approach that makes Python a highly flexible and dynamic language.
Let’s illustrate this with a simple example:
class Bird:
def fly(self):
return 'Flap Flap!'
class Airplane:
def fly(self):
return 'Zoom Zoom!'
def in_the_sky(flier):
print(flier.fly())
pigeon = Bird()
boeing = Airplane()
in_the_sky(pigeon)
in_the_sky(boeing)
# Output:
# 'Flap Flap!'
# 'Zoom Zoom!'
In this example, we have a Bird
class and an Airplane
class, both of which have a fly
method. The function in_the_sky
doesn’t care about the type of the object passed in; it only cares whether the object has a fly
method. This is duck typing in action!
The advantage of this approach is that it makes your code more flexible and easier to extend. You can add more classes (like Helicopter
, Superman
, etc.), and as long as they have a fly
method, they can be passed to the in_the_sky
function without any issues.
However, there are potential pitfalls to be aware of. For instance, if you pass an object that doesn’t have a fly
method to the in_the_sky
function, Python will throw an AttributeError
. This is something you’ll need to handle in your code, especially when working with objects that might not have the required methods.
Duck Typing in Complex Scenarios: An Intermediate Guide
As you become more comfortable with duck typing and start working with larger codebases, you’ll encounter more complex scenarios. For instance, you might need to implement interfaces or work with objects whose methods are dynamically added or removed.
Let’s consider a scenario where you’re working with a Database
class that connects to a database and a MockDatabase
class used for testing. Both classes have a connect
method, but the MockDatabase
class might have additional methods for testing.
class Database:
def connect(self):
return 'Connected to the database'
class MockDatabase:
def connect(self):
return 'Connected to the mock database'
def load_fixture(self, fixture):
return f'Loaded {fixture} into the mock database'
def setup_database(db):
print(db.connect())
if hasattr(db, 'load_fixture'):
print(db.load_fixture('test_data'))
real_db = Database()
test_db = MockDatabase()
setup_database(real_db)
setup_database(test_db)
# Output:
# 'Connected to the database'
# 'Connected to the mock database'
# 'Loaded test_data into the mock database'
In this example, the setup_database
function uses duck typing to connect to a database. It doesn’t care whether it’s connecting to a real or mock database; it only cares that the db
object has a connect
method. Additionally, it checks if the db
object has a load_fixture
method before calling it. This is a more advanced use of duck typing, where we’re dynamically checking the presence of methods.
This approach allows you to write flexible code that can work with different objects, as long as they conform to the expected behavior. However, it requires careful handling and good testing practices to ensure that your code behaves correctly in all scenarios.
Exploring Alternatives to Duck Typing in Python
While duck typing is a powerful tool in Python, it’s not the only way to achieve flexible, dynamic behavior. Other methods such as using abstract base classes or explicit type checking can also be effective, depending on your specific use case.
Abstract Base Classes
Abstract base classes (ABCs) provide a way to define interfaces when other classes are expected to implement, ensuring that they adhere to a particular protocol. Here’s an example:
from abc import ABC, abstractmethod
class Flyer(ABC):
@abstractmethod
def fly(self):
pass
class Bird(Flyer):
def fly(self):
return 'Flap Flap!'
class Airplane(Flyer):
def fly(self):
return 'Zoom Zoom!'
pigeon = Bird()
boeing = Airplane()
print(pigeon.fly())
print(boeing.fly())
# Output:
# 'Flap Flap!'
# 'Zoom Zoom!'
In this example, Flyer
is an abstract base class that defines a fly
method. Any class that inherits from Flyer
must implement the fly
method, or Python will raise a TypeError
. This approach provides a clear interface and makes errors easier to catch.
Explicit Type Checking
Another alternative is explicit type checking, where you check the type of an object before using it. This approach is less flexible but can prevent errors if you’re working with a limited set of known classes.
class Bird:
def fly(self):
return 'Flap Flap!'
class Airplane:
def fly(self):
return 'Zoom Zoom!'
def in_the_sky(flier):
if isinstance(flier, (Bird, Airplane)):
print(flier.fly())
else:
raise TypeError('The object does not know how to fly')
pigeon = Bird()
boeing = Airplane()
in_the_sky(pigeon)
in_the_sky(boeing)
# Output:
# 'Flap Flap!'
# 'Zoom Zoom!'
In this example, the in_the_sky
function checks whether the flier
object is an instance of Bird
or Airplane
before calling the fly
method. If the object is not an instance of these classes, the function raises a TypeError
.
Each of these methods has its advantages and disadvantages. Duck typing is flexible and dynamic, but can lead to runtime errors. Abstract base classes provide a clear interface, but require more boilerplate code. Explicit type checking is straightforward, but less flexible. The best approach depends on your specific needs and the complexity of your project.
Troubleshooting Common Issues with Duck Typing in Python
While duck typing can make your Python code more flexible and dynamic, it can also lead to certain issues. Two of the most common issues are AttributeError
and MethodNotFound
errors. Let’s discuss these issues and provide solutions and workarounds.
Handling AttributeError
An AttributeError
occurs when you try to access or call an attribute or method that an object doesn’t have. This is a common issue when using duck typing, as you’re often calling methods without checking the object’s type.
Consider this example:
class Duck:
def quack(self):
return 'Quack!'
class Dog:
def bark(self):
return 'Woof!'
def in_the_forest(creature):
print(creature.quack())
fido = Dog()
in_the_forest(fido)
# Output:
# AttributeError: 'Dog' object has no attribute 'quack'
To prevent this error, you can use the hasattr
function to check if an object has a certain attribute or method before calling it:
def in_the_forest(creature):
if hasattr(creature, 'quack'):
print(creature.quack())
else:
print('This creature does not quack')
in_the_forest(fido)
# Output:
# 'This creature does not quack'
Dealing with MethodNotFound Errors
A MethodNotFound
error is similar to an AttributeError
, but specifically occurs when you try to call a method that doesn’t exist. This can happen when you’re using duck typing and expect an object to have a certain method, but it doesn’t.
You can handle this error in the same way as an AttributeError
, by using hasattr
to check for the method before calling it.
Remember, while duck typing in Python enhances flexibility, it’s important to handle potential issues properly to ensure your code is robust and error-free.
Python’s Dynamic Typing System and Duck Typing
To fully grasp the concept of duck typing, it’s essential to understand Python’s dynamic typing system. Unlike static-typed languages, Python is dynamically typed, meaning the type is checked during runtime. This means that you can change the type of a variable throughout your code, providing great flexibility.
Here’s an example of dynamic typing in Python:
x = 5 # x is an integer
print(type(x))
x = 'hello' # x is now a string
print(type(x))
# Output:
# <class 'int'>
# <class 'str'>
In this example, x
initially holds an integer, then is reassigned to a string. Python is perfectly okay with this, thanks to its dynamic typing system.
Duck Typing and Object-Oriented Programming
Duck typing is a concept that fits perfectly into Python’s object-oriented programming paradigm. In object-oriented programming, classes define the ‘blueprint’ of objects, and objects are instances of these classes. Each object can have attributes (characteristics) and methods (actions).
In Python, duck typing is less concerned with the class of an object and more interested in what methods the object has. This is why you can call a method on an object without knowing its class, as long as the method exists.
Consider this example:
class Duck:
def quack(self):
return 'Quack!'
class Person:
def quack(self):
return 'I can mimic a duck!'
def in_the_forest(creature):
print(creature.quack())
donald = Duck()
john = Person()
in_the_forest(donald)
in_the_forest(john)
# Output:
# 'Quack!'
# 'I can mimic a duck!'
This is the essence of duck typing: If an object quacks like a duck (has a quack
method), then it’s treated as a duck, regardless of its class.
Method Resolution in Python
Method resolution is the process Python uses to determine which method to call when there are multiple methods with the same name. Python uses a method resolution order (MRO) algorithm that starts with the object’s class and moves up the inheritance chain.
However, with duck typing, Python doesn’t need to worry about the MRO. It simply checks if the object has the method and calls it. If the method doesn’t exist, Python raises an AttributeError
.
In summary, Python’s dynamic typing system, object-oriented programming approach, and method resolution process all contribute to enabling the flexibility of duck typing.
Duck Typing in Python: Real-World Applications
Duck typing is more than just a theoretical concept; it has practical applications in real-world scenarios, especially in web development and data analysis.
Duck Typing in Web Development
In web development, duck typing can be used to handle requests and responses. For instance, you might have different classes for GET and POST requests, but as long as they both have a process
method, you can use duck typing to handle them in a uniform way.
Duck Typing in Data Analysis
In data analysis, duck typing is often used in data cleaning and transformation. For example, you might have different classes for handling CSV, Excel, and SQL data, but as long as they all have a load_data
method, you can use duck typing to load data from different sources in a consistent manner.
Exploring Related Concepts
If you’re interested in duck typing, you might also want to explore related concepts like polymorphism and dynamic typing in Python. Polymorphism is the ability of an object to take on many forms, and it’s closely related to duck typing. Dynamic typing, as we’ve discussed, is a key feature of Python that enables duck typing.
Further Resources for Mastering Duck Typing
To deepen your understanding of duck typing and related concepts, here are some resources you might find helpful:
- Python’s official documentation on dynamic typing
- Real Python’s guide on polymorphism
- A detailed article on Python’s typing system
Wrapping Up: Mastering Duck Typing in Python
In this comprehensive guide, we’ve delved into the world of duck typing in Python, a powerful programming concept that can significantly streamline your coding process.
We began with the basics, exploring what duck typing is and how it works in Python. We then moved onto more advanced territory, discussing how to use duck typing in complex scenarios and offering solutions for common issues like AttributeError
and MethodNotFound
errors. We also explored alternative approaches to duck typing, such as using abstract base classes and explicit type checking, providing you with a well-rounded understanding of how to handle dynamic behavior in Python.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Error Handling | Complexity |
---|---|---|---|
Duck Typing | High | Requires careful handling | Low to Moderate |
Abstract Base Classes | Moderate | Clear interface, easy error handling | Moderate to High |
Explicit Type Checking | Low | Straightforward error handling | Low |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of duck typing and its practical applications.
With its flexibility and dynamic nature, duck typing is a valuable tool in any Python developer’s toolkit. Happy coding!