Pandas join() Dataframe Method Guide (With Examples)
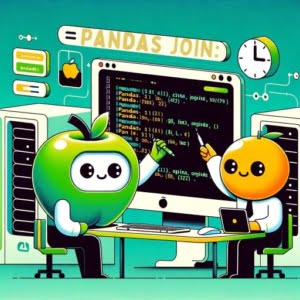
When it comes to data handling in Python, understanding the pandas join function is crucial for efficient data merging tasks. At IOFLOOD, we recognize the significance of streamlined data operations, which is why we’ve compiled this article on the pandas join function. With this info, we hope that our customers will be able to use pandas join while developing on our Dedicated bare metal servers.
This comprehensive guide will take you on a deep dive into the effective use of the pandas join function. We’ll kick off with the basics, explaining the function’s purpose and usage, and then move on to more advanced topics, including the different types of joins and handling errors.
Let’s begin our journey!
TL;DR: What is the pandas join function?
The pandas
join
function is a method in Python’s pandas library that merges the columns of two differently-indexed DataFrames into a single DataFrame. It is used with the syntax,dataframe1.join(dataframe2)
It’s a powerful tool for data manipulation, allowing you to combine data from different sources that share a common link. Here’s a simple example:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
df1.join(df2)
For more advanced methods, background, tips, and tricks, continue reading the rest of the article.
Table of Contents
Syntax Breakdown of Pandas.join()
Essentially, the pandas join function is a method that merges the columns of two differently-indexed DataFrames into a single result DataFrame.
Pandas
'join()'
can be likened to a SQL join operation, allowing you to coalesce data in a similar manner as you would combine tables in a SQL database.
The syntax for the pandas join function is as follows:
DataFrame.join(other, on=None, how='left', lsuffix='', rsuffix='', sort=False)
Let’s dissect this:
other
: This refers to the DataFrame you want to join with the original DataFrame.on
: These are the column or index level names to join on. They must be present in both DataFrames. Ifon
is None and not merging on indexes, then it merges on the common columns.how
: This defines the type of join operation. It can be one of ‘left’, ‘right’, ‘outer’, ‘inner’. We’ll delve into this in the next section.lsuffix
andrsuffix
: These are suffixes to apply to overlapping columns.sort
: This sorts the result DataFrame by the join keys in lexicographical order. It defaults to False, but if set to True, it will sort the result.
Here’s a simple example to demonstrate the use of the pandas join function:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
df1.join(df2)
This joins df1 and df2 on their index, and the result will be:
A B C D
K0 A0 B0 C0 D0
K1 A1 B1 NaN NaN
K2 A2 B2 C2 D2
As evident, the join operation merges the data from df1 and df2 into a single DataFrame.
There are several types of join operations that you can perform with pandas, including left, right, outer, and inner joins. We’ll explore these in detail in the next section. For now, understand that these options allow you to dictate the way you want to merge your data.
Advanced Parameters of join() Method
Having covered the basics, let’s explore some of the more advanced options and parameters of the pandas join function that can enhance your data manipulation capabilities.
A key parameter of the pandas join function is ‘how’. This parameter determines the type of join operation to be performed. The four options are ‘left’, ‘right’, ‘outer’, and ‘inner’.
- A ‘left’ join uses only the keys from the left DataFrame.
- A ‘right’ join uses only the keys from the right DataFrame.
- An ‘outer’ join uses the union of keys from both DataFrames.
- An ‘inner’ join uses the intersection of keys from both DataFrames.
In addition to the ‘how’ parameter, the ‘lsuffix’ and ‘rsuffix’ parameters can be used to add suffixes to overlapping column names from the left and right DataFrames, respectively.
The ‘left’ Join
The ‘left’ join includes keys from the left DataFrame only. In other words, it keeps all rows from the left DataFrame, and for matched rows in the right DataFrame, it combines columns. If there is no match in the right DataFrame, it fills with NaN.
Here’s an example of a left join operation:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
left_join_df = df1.join(df2, how='left')
print(left_join_df)
Output:
A B C D
K0 A0 B0 C0 D0
K1 A1 B1 NaN NaN
K2 A2 B2 C2 D2
In this code block, df1
is left-joined with df2
. The resultant DataFrame includes all rows from df1
. You can also see that where df1
and df2
have matched keys (K0, K2), the columns are combined. For the no-match case (i.e., K1 in df2
), df1
keeps its original row, filling in NaNs for the columns from df2
.
The ‘right’ Join
A ‘right’ join includes keys solely from the right DataFrame. The method keeps all rows from the right DataFrame, and for matched rows in the left DataFrame, it unites columns. If no match exists in the left DataFrame, it fills with NaN.
Here’s an example of a right join operation:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
right_join_df = df1.join(df2, how='right')
print(right_join_df)
Output:
A B C D
K0 A0 B0 C0 D0
K2 A2 B2 C2 D2
K3 NaN NaN C3 D3
In the above code block, df1
is right-joined with df2
. The resulting DataFrame includes all rows from df2
.
You can see that where df1
and df2
have matched keys (K0, K2), the matching rows’ columns are combined. Moreover, the non-matching key K3 from df1
, fills NaNs for its values.
The ‘outer’ Join
The ‘outer’ join includes all keys from both left and right DataFrames. It keeps all rows from both dataframes, and for matched keys, it combines rows’ columns. If no match exists, it fills with NaN.
Below is an example of an outer join operation:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
outer_join_df = df1.join(df2, how='outer')
print(outer_join_df)
Output:
A B C D
K0 A0 B0 C0 D0
K1 A1 B1 NaN NaN
K2 A2 B2 C2 D2
K3 NaN NaN C3 D3
In this code block, an outer join is performed on df1
and df2
. As a result, all the keys from both DataFrames are included.
Rows with matched keys (K0, K2) are combined, while unmatched keys (K1 from df1
and K3 from df2
) result in rows with NaNs for the non-overlapping columns.
The ‘inner’ Join
An ‘inner’ join includes only the keys common to the left and right DataFrames. It combines rows from both DataFrames for matched keys. If no match is present, those keys are excluded.
Here is an instance of an inner join operation:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
inner_join_df = df1.join(df2, how='inner')
print(inner_join_df)
Output:
A B C D
K0 A0 B0 C0 D0
K2 A2 B2 C2 D2
In the code block, an inner join operation has been performed on df1
and df2
.
The resultant DataFrame includes only the keys that df1
and df2
have in common (K0, K2). The irrelevant keys (K1 from df1
and K3 from df2
) are excluded.
Performance Considerations
Join operations can be computationally heavy, especially when dealing with large DataFrames. A handy trick to improve performance when using the pandas join function is to sort your DataFrames by the join keys before performing the join operation.
Sorting can be done using the sort_values()
function provided by pandas. Let’s consider the following example:
import pandas as pd
# Two simple, unsorted dataframes
df1 = pd.DataFrame({'A': ['A2', 'A1', 'A3'],
'B': ['B2', 'B1', 'B3']},
index=['K2', 'K1', 'K3'])
df2 = pd.DataFrame({'C': ['C3', 'C2', 'C1'],
'D': ['D3', 'D2', 'D1']},
index=['K3', 'K2', 'K1'])
# Sorting the dataframes
df1 = df1.sort_values('A')
df2 = df2.sort_values('C')
# Joining the sorted dataframes
joined_df = df1.join(df2, how='outer')
print(joined_df)
Output:
A B C D
K1 A1 B1 C1 D1
K2 A2 B2 C2 D2
K3 A3 B3 C3 D3
In this code block, unsorted DataFrames df1
and df2
are first created. Then, each DataFrame is sorted by the values in columns ‘A’ and ‘C’ respectively using the sort_values()
function. Finally, the now sorted DataFrames are joined using pandas join function.
Sorting before joining made the actual join operation faster and more efficient. Moreover, as shown in the output, it provided a more organized result, with keys ascending from K1 to K3.
Errors and Solutions: Pandas join()
Despite its power, the pandas join function can present challenges. In this section, we’ll address some common errors you may encounter and provide solutions to help you navigate them.
ValueError: Columns Overlap but No Suffix Specified
A common error that may occur while using the pandas join function is ValueError: columns overlap but no suffix specified
. This error is seen when attempting to join two DataFrames that have one or more columns with the same name, and no suffix is provided to differentiate between the overlapping columns.
We can address this problem by using ‘lsuffix’ or ‘rsuffix’ parameters. These parameters allow us to add a suffix to the overlapping column names, thereby creating a distinction.
Consider the following example:
import pandas as pd
# DataFrames with an overlapping column 'A'
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'A': ['A2', 'A3', 'A4'],
'C': ['C0', 'C1', 'C2']},
index=['K0', 'K2', 'K3'])
# Attempt to join without specifying suffix
try:
joined_df = df1.join(df2)
except ValueError as e:
print(e)
# Join with suffixes specified
joined_df_suffix = df1.join(df2, lsuffix='_df1', rsuffix='_df2')
print(joined_df_suffix)
Output:
columns overlap but no suffix specified: Index(['A'], dtype='object')
A_df1 B A_df2 C
K0 A0 B0 A2 C0
K1 A1 B1 NaN NaN
K2 A2 B2 A3 C1
In the initial code block, we try to join two DataFrames (df1
and df2
) that consist of overlapping column names ‘A’. Without specifying any suffix for these column names, it raises a ValueError
.
In response to this error, we specify suffixes for the ‘left’ and ‘right’ DataFrames in the second join operation using the ‘lsuffix’ and ‘rsuffix’ parameters respectively.
The ‘lsuffix’ and ‘rsuffix’ parameters add '_df1'
and '_df2'
to the overlapping column ‘A’ from df1
and df2
respectively, differentiating them in the resulting DataFrame (joined_df_suffix
).
KeyError
Another common error is a KeyError
, which occurs when you attempt to join on a key that doesn’t exist in one or both of the DataFrames.
Example of KeyError:
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']},
index=['K0', 'K1', 'K2'])
df2 = pd.DataFrame({'C': ['C0', 'C2', 'C3'],
'D': ['D0', 'D2', 'D3']},
index=['K0', 'K2', 'K3'])
# This will raise a KeyError
try:
df1.join(df2, on='non_existent_key')
except KeyError as e:
print(f'KeyError: {e}')
To fix this error, ensure that the key exists in both DataFrames:
# This will not raise a KeyError
df1.join(df2)
To rectify this error, ensure that the key you’re joining on is present in both DataFrames. If the key doesn’t exist, you’ll need to either create it or select a different key to join on.
Debugging Tips
During debugging, the info()
function can be extremely handy when dealing with the pandas join function. It provides a summary of each DataFrame, including the number of non-null values in each column, total entries, and each column’s data type. This information can be very useful for spotting issues such as missing values or incorrect data types that might be causing errors during the join process.
Let’s consider an example:
import pandas as pd
df1 = pd.DataFrame({'A': ['A0', 'A1', 'A2', None],
'B': ['B0', 'B1', 'B2', 'B3']},
index=['K0', 'K1', 'K2', 'K3'])
df2 = pd.DataFrame({'C': ['C0', 'C1', 'C2', 'C3'],
'D': ['D0', 'D1', 'D2', 'D3']},
index=['K0', 'K2', 'K3', 'K4'])
print("DataFrame 1 Information:")
df1.info()
print("\n")
print("DataFrame 2 Information:")
df2.info()
Output:
DataFrame 1 Information:
<class 'pandas.core.frame.DataFrame'>
Index: 4 entries, K0 to K3
Data columns (total 2 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 A 3 non-null object
1 B 4 non-null object
dtypes: object(2)
memory usage: 96.0+ bytes
DataFrame 2 Information:
<class 'pandas.core.frame.DataFrame'>
Index: 4 entries, K0 to K4
Data columns (total 2 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 C 4 non-null object
1 D 4 non-null object
dtypes: object(2)
memory usage: 96.0+ bytes
In the example, df1
and df2
DataFrames are created with a combination of different index values and some missing values in df1['A']
. Running info()
on both DataFrames provides us with a summary of each DataFrame. From the output, we can clearly see the number of non-null entries in each column and the data type of each column.
These insights are very helpful when joining tables as they enable you to know beforehand if you’ll require to handle any missing values or type conversion before performing the join operation.
Importance of Data Cleaning
Before performing join operations, it’s crucial to clean your data. This includes handling missing values, removing duplicates, and converting data types if necessary.
Clean data not only reduces the likelihood of errors during the join operation but also ensures that your results are accurate and meaningful.
Data Analysis using Pandas Library
While the pandas join()
function is a potent tool, it’s merely one element in the vast landscape of data analysis. In this section, we’ll broaden our perspective and explore the larger context of data analysis with pandas.
Pandas offers a wide array of functions for data analysis. For instance, the groupby()
function enables you to group your data based on certain criteria, simplifying the analysis of data subsets. The pivot_table()
function allows you to reshape your data and create pivot tables, akin to Excel. And the merge()
function, similar to the join function, facilitates combining DataFrames based on a common key.
The groupby()
Function
The groupby()
function is a powerful feature that allows you to group your data based on defined criteria. Typically used in combination with aggregation methods, it enables you to compute various statistical measures (such as sum, mean, or count) for each group.
Example
import pandas as pd
# Setting up a sample DataFrame
df = pd.DataFrame({
"City": ["New York", "Los Angeles", "Chicago", "New York", "Chicago", "Los Angeles", "Los Angeles"],
"Temperature": [21, 24, 14, 22, 15, 25, 24],
"Humidity": [68, 58, 65, 70, 60, 55, 56]
})
grouped_df = df.groupby("City").mean()
print(grouped_df)
Output:
Temperature Humidity
City
Chicago 14.500000 62.500000
Los Angeles 24.333333 56.333333
New York 21.500000 69.000000
In this code block, a DataFrame is set up containing temperature and humidity values of three cities.
The groupby("City")
function is used to group data by city, and mean()
is used to calculate the average of temperature and humidity for each group. The output DataFrame show the average temperature and humidity for each city.
The pivot_table()
Function
Pivot tables are great tools for summarizing and analyzing large data by organizing it into a more readable format. Pandas pivot_table()
function helps you achieve exactly that.
It provides a high level of flexibility, allowing you to specify which columns to aggregate and how to aggregate them.
Example:
pivot_df = df.pivot_table(index="City", values=["Temperature", "Humidity"], aggfunc="mean")
print(pivot_df)
Output:
Humidity Temperature
City
Chicago 62.50000 14.500000
Los Angeles 56.33333 24.333333
New York 69.00000 21.500000
Here, the pivot_table()
function is used on the same DataFrame as above. The city is used as the index, the columns are “Temperature” and “Humidity”, and the aggregation function is “mean”.
The resultant pivot table thus replicates our previous groupby()
operation, however, the pivot_table()
function allows for more complex reshaping operations.
The merge()
Function
The merge()
function in pandas serves a purpose similar to join, yet it provides a more SQL-like interface for merging DataFrames.
merge()
is powerful because it allows you to join on multiple columns, perform right, left, outer, and inner joins, and even specify suffixes for overlapping column names.
Example:
df1 = pd.DataFrame({'Key': ['K0', 'K1', 'K2'],
'A': ['A0', 'A1', 'A2'],
'B': ['B0', 'B1', 'B2']})
df2 = pd.DataFrame({'Key': ['K0', 'K1', 'K2'],
'C': ['C0', 'C1', 'C2'],
'D': ['D0', 'D1', 'D2']})
merged_df = pd.merge(df1, df2, on="Key")
print(merged_df)
Output:
Key A B C D
0 K0 A0 B0 C0 D0
1 K1 A1 B1 C1 D1
2 K2 A2 B2 C2 D2
In the block above, df1
and df2
are two DataFrames with a common column ‘Key’. Using the merge()
function with ‘Key’ as the joining column results in a DataFrame that combines the corresponding rows from both DataFrames. This merge()
operation is equivalent to an ‘inner’ join, including only keys present in both ‘Key’ columns.
These functions –
groupby()
,pivot_table()
, andmerge()
– are some of the many tools pandas offers for efficient and powerful data analysis and manipulation. Each serves its distinct purpose and can be harnessed for different data analysis scenarios.
Data Analysis Workflow with Pandas
A standard data analysis workflow with pandas might look like this:
- Load your data into a pandas DataFrame using the
read_csv()
,read_excel()
, orread_sql()
function. - Cleanse your data using functions such as
dropna()
,fillna()
, andreplace()
. - Transform your data using functions like
groupby()
,pivot_table()
, andjoin()
. - Analyze your data using pandas’ statistical functions.
- Visualize your results using a library like Matplotlib or Seaborn.
Example of a typical data analysis workflow with pandas:
import pandas as pd
import matplotlib.pyplot as plt
# 1. Load data
df = pd.read_csv('data.csv')
# 2. Cleanse data
df = df.dropna()
# 3. Transform data
df_grouped = df.groupby('column1').mean()
# 4. Analyze data
mean_value = df['column2'].mean()
# 5. Visualize results
plt.plot(df['column1'], df['column2'])
plt.show()
Efficient data manipulation is the cornerstone of effective data analysis. The capability to join, reshape, and aggregate your data enables you to extract meaningful insights. And with pandas, data manipulation is not only efficient but also intuitive and user-friendly.
Libraries and Tools Similar to Pandas
While pandas is an indispensable tool for data analysis in Python, it’s not the only one. Python boasts a diverse ecosystem of libraries for data analysis, each with its unique strengths. Let’s take a brief look at some of them.
- NumPy: This is the base package for numerical computation in Python. It provides support for arrays, matrices, and high-level mathematical functions to operate on these arrays.
SciPy: Built on top of NumPy, SciPy is used for more advanced computations. It provides modules for optimization, integration, interpolation, eigenvalue problems, and more.
Matplotlib: This is a plotting library for creating static, animated, and interactive visualizations in Python.
Seaborn: Built on top of Matplotlib, Seaborn aids in creating more attractive and informative statistical graphics.
Scikit-learn: This is a machine learning library in Python. It features various classification, regression, and clustering algorithms.
Pandas can be integrated with all these tools to create a robust data analysis pipeline. For instance, you can use pandas for data manipulation, NumPy and SciPy for computation, and Matplotlib and Seaborn for visualization.
Here’s a table summarizing each library’s strengths:
Library | Main Features | Typical Use Cases |
---|---|---|
NumPy | Support for arrays and matrices, high-level mathematical functions | Numerical computation |
SciPy | Modules for optimization, integration, interpolation, etc. | Advanced computations |
Matplotlib | Creation of static, animated, and interactive visualizations | Data visualization |
Seaborn | Creation of attractive and informative statistical graphics | Advanced data visualization |
Scikit-learn | Classification, regression, and clustering algorithms | Machine learning |
Further Resources for Pandas Library
If you’re interested in learning more ways to utilize the Pandas library, here are a few resources that you might find helpful:
- Exploring Data Analysis with Pandas: A Step-by-Step Tutorial: Follow this step-by-step tutorial to learn how to perform data analysis tasks using Pandas, such as data cleaning, aggregation, and visualization.
Guide on Using the drop() Function to Remove Columns in Pandas DataFrame: Our guide provides a step-by-step explanation of how to use the drop() function in Pandas to remove columns from a DataFrame in Python.
Exploring Unique Values in a Pandas DataFrame using the unique() Function: Our tutorial demonstrates how to use the unique() function in Pandas to identify and extract unique values from a DataFrame in Python.
Joining DataFrames with Pandas: A Tutorial: A tutorial on DataCamp that covers various methods of joining DataFrames in Pandas with examples and explanations.
pandas User Guide: Merging, joining and concatenating: The official pandas user guide section on merging, joining, and concatenating DataFrames, providing comprehensive documentation and examples.
Pandas join() Function: A Comprehensive Guide: A comprehensive guide on using the join() function in Pandas to join DataFrames based on their indexes.
Recap: Join DataFrames with Pandas
In this comprehensive guide, we’ve journeyed from the fundamentals of the pandas join function to its advanced usage, discussed common troubleshooting tips, and zoomed out to understand the broader context of data analysis with pandas. Let’s take a moment to consolidate our learnings.
The pandas join function is a robust tool for merging the columns of two potentially differently-indexed DataFrames into a single result DataFrame. It’s akin to the SQL join operation but offers more flexibility and ease of use. With a firm grasp of its syntax and options, you can manipulate and analyze data like a pro.
We’ve delved into some of the advanced features of the pandas join function, such as the ‘how’, ‘lsuffix’, and ‘rsuffix’ parameters. These provide you more control over how your data is joined, enabling you to tackle complex data analysis tasks with confidence.
Of course, like any powerful tool, the pandas join function comes with its challenges. We’ve discussed common errors and their solutions, and provided tips for enhancing performance and ensuring accurate results. With these troubleshooting tips up your sleeve, you’ll be well-prepared to handle any challenges that come your way.
Finally, we’ve looked at the broader context of data analysis with pandas. The pandas library offers a plethora of functions for data manipulation and analysis, making it a versatile and powerful tool for any data analyst. And with its integration with other Python libraries, pandas is part of a rich ecosystem that can handle any data analysis task.
In conclusion, the pandas join function is an indispensable tool for any data analyst. Whether you’re a novice or an experienced analyst, understanding and mastering the pandas join function will significantly enhance your data analysis skills. So, keep practicing, keep exploring, and keep pushing the boundaries of data analysis.