Using Pandas drop() Column | DataFrame Function Guide
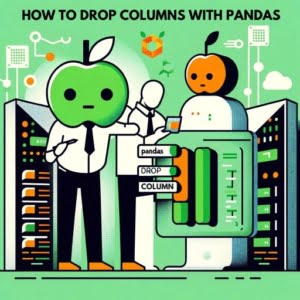
Managing and manipulating data efficiently is a top priority at IOFLOOD, especially when it comes to removing unnecessary columns from datasets. The pandas drop column function is a lifesaver in this regard, allowing for seamless data cleanup processes. In order to help our customers utilize pandas drop column on their dedicated cloud services, we have created today’s guide.
In this article, we’ll dive into the simplicity of column removal in pandas. We’ll equip you with the knowledge and skills to effectively drop columns, making your data analysis cleaner and more focused.
So, let’s get started and master the art of column removal in pandas!
TL;DR: How do I remove columns in pandas?
You can remove columns in pandas using the
drop
method on a DataFrame. For example, to remove a column named ‘A’ from a DataFramedf
, you would usedf.drop('A', axis=1)
. Remember,axis=1
is used to specify that we’re dropping a column. For more advanced methods, background, tips, and tricks, continue reading the article.
Simple Syntax example of removing a column:
df = df.drop('A', axis=1)
Here’s a more thorough example of using the drop command.
import pandas as pd
# Let's create a simple dataframe
data = {
'A': [1, 2, 3, 4, 5],
'B': [10, 20, 30, 40, 50],
'C': [100, 200, 300, 400, 500],
}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
# Now, let's remove the column 'A'
df = df.drop('A', axis=1)
print("\nDataFrame after removing 'A':")
print(df)
The output will be:
Original DataFrame:
A B C
0 1 10 100
1 2 20 200
2 3 30 300
3 4 40 400
4 5 50 500
DataFrame after removing 'A':
B C
0 10 100
1 20 200
2 30 300
3 40 400
4 50 500
As seen in the output, the column ‘A’ has been removed from the DataFrame.
Table of Contents
Basic Uses of Pandas drop() Method
In pandas, the drop
method is your tool for column removal. It’s as straightforward as it sounds. You simply specify the columns you want to eliminate from your DataFrame, making your data analysis more streamlined and focused.
Example of using the drop
method:
import pandas as pd
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
print('Original DataFrame:')
print(df)
df = df.drop('A', axis=1)
print('
DataFrame after dropping column A:')
print(df)
Let’s illustrate with a simple example. Assume you have a DataFrame df
with columns ‘A’, ‘B’, ‘C’, and ‘D’, and you wish to remove the column ‘A’. Here’s how you do it:
df = df.drop('A', axis=1)
In this line of code, ‘A’ is the column we want to remove, and axis=1
informs pandas that we’re dropping a column (not a row).
What if you need to remove multiple columns? No worries! You can pass a list of column names to the drop
method. For instance, we want to remove columns ‘B’ and ‘C’. Here’s how:
df = df.drop(['B', 'C'], axis=1)
The Inplace Parameter
You may have observed that we’re reassigning the result of the drop
method back to df
. This is because the drop
method doesn’t alter the original DataFrame by default; it returns a new one with the specified columns removed.
If you wish to modify the original DataFrame, you can use the inplace
parameter and set it to True
:
df.drop('A', axis=1, inplace=True)
Exercise caution here! Once a column is dropped with inplace=True
, it’s permanently removed from the original DataFrame.
The Axis Parameter
You might be curious about the axis
parameter we’ve been using. In pandas, axis=0
refers to rows, while axis=1
refers to columns.
When you’re using the
drop
method to remove columns, ensure to setaxis=1
.
Pandas drop(): Errors and Solutions
Even the most experienced data analysts can encounter errors when attempting to drop columns in pandas. Here we’ll go over some of the more common issues.
KeyError
For example, trying to drop a column that doesn’t exist in the DataFrame is a common error. In this case, pandas will raise a KeyError
.
To avoid this, always verify if a column exists before trying to drop it:
if 'A' in df.columns:
df.drop('A', axis=1, inplace=True)
Missing Axis
Another frequently made error is forgetting to specify the axis
parameter. Remember, axis=1
is for columns and axis=0
is for rows.
If you fail to specify
axis=1
, pandas will attempt to drop a row with the given name and likely raise aKeyError
.
Catching Errors with Try / Except
When dropping columns, consider using a try/except
block to catch any errors and handle them gracefully:
try:
df.drop('E', axis=1, inplace=True)
except KeyError:
print('Column not found')
Best Practices for drop() Method
Efficiency is crucial when dealing with large DataFrames. Here are some tips for efficient column removal:
Drop Multiple Columns Simultaneously
Drop multiple columns simultaneously by passing a list of column names to the drop
method. This is quicker than dropping one column at a time.
You can pass a list of column names to the drop
method to drop multiple columns at once.
import pandas as pd
# Create a simple dataframe
data = {'A': [1, 2, 3, 4, 5],'B': [10, 20, 30, 40, 50],'C': [100, 200, 300, 400, 500]}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
# Drop columns 'A' and 'B'
df = df.drop(['A', 'B'], axis=1)
print("\nDataFrame after removing 'A' and 'B':")
print(df)
The output will be:
Original DataFrame:
A B C
0 1 10 100
1 2 20 200
2 3 30 300
3 4 40 400
4 5 50 500
DataFrame after removing 'A' and 'B':
C
0 100
1 200
2 300
3 400
4 500
In this example, we start by creating a DataFrame with three columns: ‘A’, ‘B’, and ‘C’. We then pass a list of the columns we want to remove, [‘A’, ‘B’], to the drop
method. The result is a DataFrame with only the ‘C’ column remaining.
By dropping multiple columns at once, we can perform column removal more efficiently, particularly when working with large DataFrames.
Create a New DataFrame with Fewer Columns
If you’re dropping numerous columns, consider creating a new DataFrame with only the columns you intend to keep. This can be more efficient than dropping columns individually.
Example of creating a new DataFrame with just the columns you want to keep:
import pandas as pd
# Create a simple dataframe
data = {'A': [1, 2, 3, 4, 5],'B': [10, 20, 30, 40, 50],'C': [100, 200, 300, 400, 500]}
df = pd.DataFrame(data)
print("Original DataFrame:")
print(df)
# Create new DataFrame with only column 'C'
df_new = df[['C']]
print("\nNew DataFrame with only 'C':")
print(df_new)
The output will be:
Original DataFrame:
A B C
0 1 10 100
1 2 20 200
2 3 30 300
3 4 40 400
4 5 50 500
New DataFrame with only 'C':
C
0 100
1 200
2 300
3 400
4 500
As seen in the output, the new DataFrame contains only the ‘C’ column.
Other Methods: Pandas Data Removal
Apart from the drop
method, you can also eliminate columns from a DataFrame using the del
keyword or the pop
method:
# Here is your code.
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
print('Original DataFrame:')
print(df)
del df['A']
print('\nDataFrame after deleting column A using del keyword:')
print(df)
popped_column = df.pop('B')
print('\nDataFrame after popping column B:')
print(df)
print('\nPopped column:')
print(popped_column)
The output for your code will be:
Original DataFrame:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
DataFrame after deleting column A using del keyword:
B C
0 4 7
1 5 8
2 6 9
DataFrame after popping column B:
C
0 7
1 8
2 9
Popped column:
0 4
1 5
2 6
Name: B, dtype: int64
As you can see, first the column ‘A’ is deleted using del
keyword. After deletion, only ‘B’ and ‘C’ columns are left in the DataFrame.
Then, column ‘B’ is popped out using the pop
method which simultaneously removes it from DataFrame and returns it as a pandas series.
After popping, only column ‘C’ is left in the DataFrame. The popped column ‘B’ is printed at the end as a pandas series.
del df['A']
will remove the column ‘A’ from the DataFrame.
df.pop('A')
will remove the column ‘A’ and return it as a Series.
Preventing Errors in DataFrames
A profound understanding of DataFrame structure can save you from numerous errors. Always be aware of the shape and structure of your DataFrame. Employ methods like head
, info
, and describe
to get a thorough sense of your DataFrame before manipulating it.
Use head
to Get a Glimpse of Your DataFrame
The head
function allows you to quickly peek at the first few rows of your DataFrame. This can give you a general idea of your data’s structure and contents.
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
print('First few rows of the DataFrame:')
print(df.head())
Output for above code snippet will be:
First few rows of the DataFrame:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
Use info
to Get Detailed Information about Your DataFrame
The info
method provides more detailed information about your DataFrame, such as the number of entries, the column names, the number of non-null entries per column, and the data types of each column.
print('Information about the DataFrame:')
print(df.info())
Output:
Information about the DataFrame:
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 3 entries, 0 to 2
Data columns (total 3 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 A 3 non-null int64
1 B 3 non-null int64
2 C 3 non-null int64
dtypes: int64(3)
memory usage: 200.0 bytes
None
Use describe
for a Statistical Summary of Your DataFrame
The describe
method gives you useful statistical information about each numerical column in your DataFrame, such as count, mean, standard deviation, minimum, maximum, and quartile values.
print('Statistical summary of the DataFrame:')
print(df.describe())
Output:
Statistical summary of the DataFrame:
A B C
count 3.0 3.0 3.0
mean 2.0 5.0 8.0
std 1.0 1.0 1.0
min 1.0 4.0 7.0
25% 1.5 4.5 7.5
50% 2.0 5.0 8.0
75% 2.5 5.5 8.5
max 3.0 6.0 9.0
As a data analyst, it’s important to have a deep understanding of your DataFrame to avoid errors and perform accurate manipulations. Using
head
,info
, anddescribe
is a good starting point to get familiar with your DataFrame.
Uses of Pandas Library: Data Analysis
Having gone over the details of dropping columns in Pandas, let’s refresh on some Pandas basics in case you need a more thorough understanding.
Pandas is a software library for Python designed to facilitate working with ‘relational’ or ‘labeled’ data. It’s a fundamental building block for practical, real-world data analysis in Python.
At the core of pandas is the DataFrame object, a two-dimensional table of data with rows and columns. It’s similar to a spreadsheet or SQL table, or a dictionary of Series objects, making pandas a commonly used and powerful tool for data manipulation and analysis.
The DataFrame: Your Data Analysis Playground
A DataFrame in pandas is a two-dimensional data structure capable of holding different types of data (like numbers, strings, and dates). It allows for flexible data manipulation with labeled axes (rows and columns). It can be thought of as a dictionary of Series structures and can be created in various ways.
Here’s an example of creating a DataFrame from a dictionary:
import pandas as pd
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
print(df)
Creating and Manipulating DataFrame Structures
Beyond creating a DataFrame, pandas provides methods for manipulating its structure. You can add columns, remove columns, rename columns, and more. This flexibility makes pandas a powerful tool for data manipulation and analysis.
For example, to add a new column to a DataFrame, you can simply assign data to a column that doesn’t exist yet:
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
print('Original DataFrame:')
print(df)
df['D'] = [10, 11, 12]
print('DataFrame after adding column D:')
print(df)
Here’s how that would look:
Original DataFrame:
A B C
0 1 4 7
1 2 5 8
2 3 6 9
DataFrame after adding column D:
A B C D
0 1 4 7 10
1 2 5 8 11
2 3 6 9 12
Sorting Dataframe by Column
Dropping columns, although common, is just one of the many operations you can perform to manipulate and analyze your data in pandas. You can also use pandas to sort data, filter data, group data, merge data, and more.
Here’s a quick example of sorting a DataFrame by a specific column:
data = {'A': [3, 1, 2], 'B': [6, 4, 5], 'C': [9, 7, 8]}
df = pd.DataFrame(data)
print('Original DataFrame:')
print(df)
df = df.sort_values('A', ascending=False)
print('DataFrame after sorting by column A in descending order:')
print(df)
Here’s how the output would look:
Original DataFrame:
A B C
0 3 6 9
1 1 4 7
2 2 5 8
DataFrame after sorting by column A in descending order:
A B C
0 3 6 9
2 2 5 8
1 1 4 7
In this line of code, we’re sorting the DataFrame df
by the column ‘A’ in descending order.
Other Python Tools for Data Analysis
While pandas is a powerful tool for data manipulation and analysis, it’s just one of many libraries available for data analysis in Python.
NumPy
Other libraries, like NumPy for numerical computing and SciPy for scientific computing, offer additional functionalities that complement pandas. For example, NumPy’s support for multi-dimensional arrays and matrices is fundamental for numerical computations in Python.
import numpy as np
# Creating a 2D array
arr = np.array([[1, 2, 3], [4, 5, 6]])
print('2D Array:')
print(arr)
Data Visualization
Data visualization is a crucial part of data analysis. It allows you to see patterns, trends, and insights in your data that might not be obvious from looking at tables of data.
Libraries like Matplotlib and Seaborn provide a wide range of data visualization tools, from simple bar plots and line charts to complex heatmaps and interactive plots.
import matplotlib.pyplot as plt
# Data
x = ['A', 'B', 'C']
y = [1, 2, 3]
# Create bar plot
plt.bar(x, y)
plt.title('Bar Plot Example')
plt.xlabel('Categories')
plt.ylabel('Values')
plt.show()
By visualizing your data, you can gain a deeper understanding and make more informed decisions.
Further Resources for Learning in Data Analysis
The field of data analysis is vast and constantly evolving. To stay up-to-date, continuous learning is essential. There are many resources available for further learning in data analysis, from online courses and tutorials to textbooks and research papers.
Some popular platforms for learning data analysis include Coursera, edX, and Kaggle.
Further Resources for Pandas Library
If you’re interested in learning more ways to utilize the Pandas library, here are a few resources that you might find helpful:
- Python Pandas Quick Start Guide by IOFlood: This guide is intended to provide a comprehensive overview for the Pandas library.
Guide on Renaming Columns in a Pandas DataFrame: Our guide provides step-by-step instructions on how to rename columns in a Pandas DataFrame using various techniques in Python.
An In-Depth Guide to the Pandas join() Method: This guide, also provided by us, explains how to use the join() method in Pandas to merge DataFrame objects based on common columns, with examples for better understanding.
Pandas drop() Function: A Comprehensive Guide: A comprehensive guide on using the drop() function in Pandas to remove columns from a DataFrame.
How to Drop Columns in Pandas DataFrame: An article on GeeksforGeeks explaining how to drop one or multiple columns from a Pandas DataFrame using different methods.
Pandas DataFrame drop() Function: Tutorial and Examples: A tutorial on w3resource.com that demonstrates the drop() function in Pandas DataFrame, providing examples and explanations.
Wrapping Up: Pandas drop() Function
We’ve journeyed through the world of pandas and explored the art of column removal. We’ve learned how to use the drop
method to remove one or more columns from a DataFrame, discovered the importance of the axis
and inplace
parameters, and discussed the implications of column removal on data integrity.
But pandas is more than just column removal. It’s a powerful library for data manipulation and analysis, with a wide range of functionalities that make it easy to work with data in Python. From creating and manipulating DataFrames to sorting data, filtering data, and more, pandas offers the tools you need to handle any data analysis task.
As we continue to generate and collect more and more data, the demand for powerful data analysis tools like pandas will only grow. Whether you’re just starting your data analysis journey or looking to deepen your skills, mastering pandas is a valuable investment in your future. So keep exploring, keep learning, and keep analyzing data with pandas!