Java FileReader Class: A Usage Guide with Examples
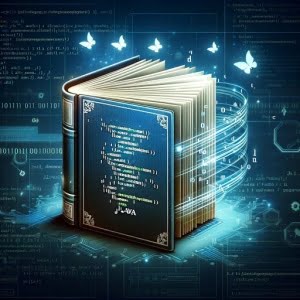
Are you finding it challenging to read files in Java? You’re not alone. Many developers find themselves in a similar situation. But just like a librarian, Java has tools to help you access and read the contents of any ‘book’ in your digital library.
This guide will walk you through the process of reading files in Java, from the basics to more advanced techniques. We’ll cover everything from using the FileReader and BufferedReader classes, to handling common issues and even exploring alternative approaches.
So, let’s dive in and start mastering file reading in Java!
TL;DR: How Do I Use the FileReader Class in Java?
You can use the
FileReader
class to read data from a file with the syntax,FileReader reader = new FileReader('file.txt');
. This approach allows you to read and process the contents of a file line by line. TheBufferedReader
class is also a viable option to use
Here’s a simple example:
FileReader reader = new FileReader('file.txt');
BufferedReader bufferedReader = new BufferedReader(reader);
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
bufferedReader.close();
# Output:
# Contents of 'file.txt'
In this example, we create a FileReader
object for ‘file.txt’. We then create a BufferedReader
object and use it to read the file line by line. Each line is printed to the console until there are no more lines to read. Finally, we close the BufferedReader
to free up system resources.
This is a basic way to read files in Java, but there’s much more to learn about handling files in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- The Basic Use of FileReader and BufferedReader in Java
- Advanced File Reading Techniques in Java
- Exploring Alternative Methods to Read Files in Java
- Handling Common Issues When Reading Files in Java
- Understanding File Handling in Java
- The Bigger Picture: File Reading in Larger Java Applications
- Wrapping Up: Mastering File Reading in Java
The Basic Use of FileReader and BufferedReader in Java
Java provides several ways to read files, but one of the most straightforward methods involves using the FileReader
and BufferedReader
classes. These classes are part of the java.io
package, which contains classes for system input and output through data streams, serialization, and file systems.
Understanding FileReader and BufferedReader
The FileReader
class is a convenience class for reading character files. It’s a bridge from byte streams to character streams: it reads bytes and decodes them into characters using a specified charset.
The BufferedReader
class, on the other hand, reads text from a character-input stream, buffering characters to provide efficient reading of characters, arrays, and lines.
Here’s a simple example of how to use these classes to read a file in Java:
try {
FileReader reader = new FileReader('file.txt');
BufferedReader bufferedReader = new BufferedReader(reader);
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
bufferedReader.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Contents of 'file.txt'
In this example, we first create a FileReader
object for ‘file.txt’. We then create a BufferedReader
object, which we use to read the file line by line. Each line is printed to the console until there are no more lines to read. Finally, we close the BufferedReader
to free up system resources.
Advantages and Potential Pitfalls
Using FileReader
and BufferedReader
to read files in Java has its advantages. It’s a simple and straightforward approach that works well for small to medium-sized files. The code is easy to understand and use, even for beginners.
However, this method may not be suitable for very large files because it reads the file line by line. If the file is too large, it can lead to performance issues. Also, it’s important to handle exceptions properly, especially FileNotFoundException
and IOException
, to prevent your program from crashing. We’ll talk more about exception handling in the ‘Troubleshooting and Considerations’ section.
Advanced File Reading Techniques in Java
As you gain more experience with Java, you’ll encounter scenarios where you need to handle more complex file reading tasks. This could involve reading large files, binary files, or using the java.nio
package for non-blocking I/O operations.
Handling Large Files in Java
For extremely large files, reading line by line might not be efficient. In this case, Java’s NIO (Non-blocking I/O) package provides the Files
and Paths
classes, which can be used to read all lines of a file at once.
Here’s an example:
import java.nio.file.*;
try {
Path path = Paths.get('largefile.txt');
List<String> lines = Files.readAllLines(path);
for (String line : lines) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Contents of 'largefile.txt'
This example reads all lines from ‘largefile.txt’ into a list of strings. Each line is then printed to the console.
Reading Binary Files in Java
Binary files contain data in a format that’s not intended for interpretation as text. If you need to read binary files, you can use the FileInputStream
class.
import java.io.*;
try {
File file = new File('binaryfile.bin');
FileInputStream fis = new FileInputStream(file);
byte[] bytesArray = new byte[(int)file.length()];
fis.read(bytesArray);
for (byte b : bytesArray) {
System.out.print((char)b);
}
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Contents of 'binaryfile.bin'
In this example, we read the entire contents of ‘binaryfile.bin’ into a byte array. We then print each byte as a character to the console.
The Java NIO Package
The java.nio
package (introduced in Java 4) provides classes for non-blocking I/O operations. This can be useful for handling large files or for improving performance when working with many small files.
Here’s an example of using the java.nio
package to read a file:
import java.nio.file.*;
try {
Path path = Paths.get('file.txt');
byte[] bytes = Files.readAllBytes(path);
for (byte b : bytes) {
System.out.print((char)b);
}
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Contents of 'file.txt'
In this example, we use the Files.readAllBytes()
method to read the entire file into a byte array. We then print each byte as a character to the console.
Exploring Alternative Methods to Read Files in Java
While FileReader
and BufferedReader
are commonly used for file reading in Java, there are alternative methods that could be more suitable depending on your specific needs. Let’s explore some of these alternatives.
Using the Scanner Class
The Scanner
class is a simple text scanner which can parse primitive types and strings using regular expressions. It breaks its input into tokens using a delimiter pattern, which by default matches whitespace.
Here’s an example of using Scanner
to read a file:
import java.io.*;
import java.util.Scanner;
try {
File file = new File('file.txt');
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
# Output:
# Contents of 'file.txt'
In this example, we create a Scanner
object and use it to read the file line by line. Each line is printed to the console until there are no more lines to read.
Using Third-Party Libraries
There are also third-party libraries such as Apache Commons IO that provide utilities to assist with input/output operations. These libraries offer robust and efficient methods to read files in Java.
Here’s an example of using Apache Commons IO to read a file:
import org.apache.commons.io.FileUtils;
import java.io.File;
import java.io.IOException;
try {
File file = new File('file.txt');
String content = FileUtils.readFileToString(file, 'UTF-8');
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Contents of 'file.txt'
In this example, we use the FileUtils.readFileToString()
method to read the entire file into a string. We then print the string to the console.
Benefits, Drawbacks, and Decision-Making Considerations
The Scanner
class is useful for reading and parsing data from a file. It’s easy to use and can handle different types of input. However, it’s not the most efficient method for large files.
Third-party libraries like Apache Commons IO provide powerful and flexible utilities for file reading. They can handle large files and offer additional features not found in the standard Java libraries. However, they introduce external dependencies to your project, which might not be desirable in all cases.
When choosing a method to read files in Java, consider the size and type of the files, the complexity of the reading operation, and the specific requirements of your project.
Handling Common Issues When Reading Files in Java
When working with file reading in Java, it’s crucial to understand and handle common issues that may arise. Two of the most common exceptions you’ll encounter are FileNotFoundException
and IOException
.
Handling FileNotFoundException
A FileNotFoundException
is thrown during a file reading operation when the file with the specified pathname does not exist.
Here’s how to handle FileNotFoundException
:
import java.io.*;
try {
FileReader reader = new FileReader('nonexistentfile.txt');
} catch (FileNotFoundException e) {
System.out.println('File does not exist.');
}
# Output:
# 'File does not exist.'
In this example, we attempt to create a FileReader
for a file that does not exist. This throws a FileNotFoundException
, which we catch and handle by printing a message to the console.
Handling IOException
An IOException
is thrown when an input or output operation is failed or interrupted. When reading a file, an IOException
might be thrown if the file is unexpectedly closed or if an error occurs while reading the file.
Here’s how to handle IOException
:
import java.io.*;
try {
FileReader reader = new FileReader('file.txt');
reader.read();
reader.close();
reader.read(); // Attempt to read after closing the reader
} catch (IOException e) {
System.out.println('Error occurred while reading the file.');
}
# Output:
# 'Error occurred while reading the file.'
In this example, we attempt to read from a FileReader
after it has been closed. This throws an IOException
, which we catch and handle by printing a message to the console.
Considerations
Always close your readers and streams in a finally
block or use a try-with-resources statement to ensure they are closed even if an exception is thrown. This is important to prevent resource leaks.
Remember that handling exceptions properly is crucial for building robust and reliable Java applications. Always anticipate and plan for potential issues that might occur during file reading operations.
Understanding File Handling in Java
Java’s I/O system is robust and flexible, capable of handling a wide range of input and output scenarios. To fully grasp file reading in Java, it’s important to understand the underlying concepts that make it all possible.
The Role of Streams in Java
In Java, a stream can be defined as a sequence of data. There are two types of Streams: InputStream
and OutputStream
. In the context of file reading, we’re primarily concerned with InputStream
, which reads data from a source.
import java.io.*;
try {
InputStream input = new FileInputStream('file.txt');
int data = input.read();
while(data != -1) {
System.out.print((char) data);
data = input.read();
}
input.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Contents of 'file.txt'
In this example, we create an InputStream
for ‘file.txt’ and read the data byte by byte until there’s no more data to read (-1 is returned).
Character Streams vs Byte Streams
Java provides two types of streams to handle data: character streams and byte streams.
- Character Streams (
FileReader
,BufferedReader
): These are designed to handle input and output of characters, automatically handling the encoding and decoding tasks. They are ideal for text data. Byte Streams (
FileInputStream
,BufferedInputStream
): These are low-level streams that provide binary I/O operations, directly working with bytes of data. They are ideal for binary data, such as images and serialized objects.
Understanding these fundamentals of file handling in Java provides a solid foundation from which to explore more advanced topics and techniques.
The Bigger Picture: File Reading in Larger Java Applications
Understanding how to read files in Java is a fundamental skill, but it’s equally important to understand how this fits into larger Java applications. File reading is not an isolated task, but a crucial part of many Java applications.
Reading Configuration Files
Many applications store configuration settings in files. These settings can be read at runtime to modify the behavior of the application. Java’s Properties
class can be used to read properties files, which are often used for configuration.
import java.io.*;
import java.util.Properties;
try {
FileReader reader = new FileReader('config.properties');
Properties properties = new Properties();
properties.load(reader);
String property = properties.getProperty('key');
System.out.println(property);
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Value of 'key' in 'config.properties'
In this example, we read a properties file and print the value of a specific property.
Processing Large Data Sets
Java’s file reading capabilities come in handy when dealing with large data sets. For example, you might have a large CSV file that you want to parse and process in some way.
import java.io.*;
try {
BufferedReader reader = new BufferedReader(new FileReader('data.csv'));
String line;
while ((line = reader.readLine()) != null) {
String[] fields = line.split(',');
// Process fields
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
In this example, we read a CSV file line by line, splitting each line into fields based on commas.
Further Resources for Mastering File Handling in Java
If you’re interested in diving deeper into file handling in Java, here are some excellent resources to explore:
- Mastering File Handling with Java’s File Class – Dive into Java’s File class for creating, deleting, and managing files and directories.
Exploring Java BufferedReader – Master Java BufferedReader techniques for improved performance and resource management.
Java Read File to String: Basics – Learn how to read a file into a string in Java for convenient text processing.
Oracle’s Java Tutorials: Basic I/O – These official Java tutorials cover all aspects of input and output in Java.
Baeldung’s Guide to Java 8’s Files API provides a deep dive into the Files API introduced in Java 8.
Java FileReader Class by Programiz details Java’s FileReader class, with examples.
Wrapping Up: Mastering File Reading in Java
In this comprehensive guide, we’ve delved deep into the process of reading files in Java. We’ve explored the basics and advanced techniques, discussed alternative approaches, and tackled common issues that you may encounter.
We began with the basic use of FileReader
and BufferedReader
classes, which offer a simple and straightforward method to read files in Java. We then moved onto more advanced techniques, such as handling large files and binary files, and using the java.nio
package for non-blocking I/O operations.
We also explored alternative methods for file reading in Java, such as using the Scanner
class and third-party libraries like Apache Commons IO. Each method has its own benefits and potential drawbacks, and the choice between them depends on your specific needs and the requirements of your project.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
FileReader & BufferedReader | Simple, great for small to medium-sized files | Not ideal for very large files |
java.nio package | Efficient for large files | More complex to use |
Scanner class | Easy to use, great for parsing data | Not the most efficient for large files |
Apache Commons IO | Powerful, flexible, great for large files | Introduces external dependencies |
We also discussed the importance of handling exceptions like FileNotFoundException
and IOException
to prevent your program from crashing. Understanding these common issues and how to address them is crucial for building robust and reliable Java applications.
Whether you’re a beginner just starting out with file handling in Java or an experienced developer looking to brush up on your skills, we hope this guide has provided you with valuable insights and practical knowledge. Now, you’re well-equipped to handle file reading in Java with confidence. Happy coding!