Java Read File to String: Classes to Use for I/O Operations
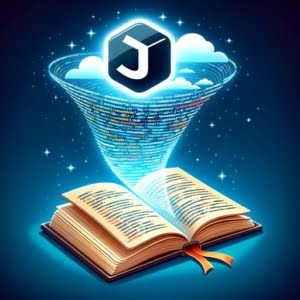
Are you finding it challenging to read a file and convert it to a string in Java? You’re not alone. Many developers find this task a bit tricky, but Java, like a librarian turning pages into knowledge, has the capability to transform file data into strings.
Java’s file handling capabilities and string data type are powerful tools that can help you manipulate and process data effectively. Understanding how to read a file and convert it to a string is a fundamental skill that can open up new possibilities for data processing and manipulation.
In this guide, we’ll walk you through the process of reading a file and converting it to a string in Java, from basic use to more advanced techniques. We’ll cover everything from using the Files
and Paths
classes, dealing with different file formats and encodings, to exploring alternative methods and troubleshooting common issues.
So, let’s dive in and start mastering how to read a file to string in Java!
TL;DR: How Do I Read a File and Convert it to a String in Java?
In Java, you can read a file and convert it to a string using the
Files
andPaths
classes. Here’s a simple example:
String content = new String(Files.readAllBytes(Paths.get("file.txt")));
# Output:
# This will read the file 'file.txt' and convert it to a string.
In this example, we use the Files.readAllBytes
method to read all bytes from the file at the path specified by Paths.get("file.txt")
. The bytes are then converted to a string using the String
constructor.
This is a basic way to read a file and convert it to a string in Java, but there’s much more to learn about file handling and string manipulation in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Java File Reading Basics: Files and Paths
- Handling File Formats and Encodings in Java
- Exploring Alternative Approaches
- Troubleshooting Common Issues in Java File Reading
- Unraveling Java’s File Handling and String Data Type
- Broadening Horizons: File Reading in Diverse Applications
- Wrapping Up: Read Files to String in Java
Java File Reading Basics: Files and Paths
Java provides several classes to interact with the file system, and two of the most fundamental ones are Files
and Paths
. These classes are part of the java.nio.file
package, which is designed for scalable and efficient file I/O operations.
Reading a File with Files and Paths
The Files
class offers a method called readAllBytes
, which reads all bytes from a file. This method is very handy when you want to read a small file into memory. The Paths
class, on the other hand, is used to get the path of the file we want to read.
Here’s a simple example of how you can use these classes to read a file and convert it to a string:
import java.nio.file.*;
public class ReadFileToString {
public static void main(String[] args) throws Exception {
String content = new String(Files.readAllBytes(Paths.get("file.txt")));
System.out.println(content);
}
}
# Output:
# [The content of 'file.txt' will be printed here]
In this code, we first import the necessary classes from the java.nio.file
package. Then, in the main
method, we read all bytes from the file ‘file.txt’ using Files.readAllBytes(Paths.get("file.txt"))
. The bytes are then converted to a string using the String
constructor, and the content of the file is printed to the console.
Advantages and Potential Pitfalls
This method is simple and convenient, but it’s not suitable for large files because it reads all bytes into memory at once, which could lead to OutOfMemoryError
if the file is too large. Also, it assumes that the file’s content is text and that the default character encoding of the Java runtime is appropriate to decode the bytes. If the file contains binary data or text in a different encoding, the result may not be what you expect.
Handling File Formats and Encodings in Java
When working with files in Java, you may encounter files of different formats and encodings. Understanding these variations is crucial to accurately reading a file and converting it to a string.
Dealing with Different Encodings
In the basic use case, we assumed that the file’s content is text and that the default character encoding of the Java runtime is appropriate to decode the bytes. However, text files can be encoded in various ways, such as UTF-8, ISO-8859-1, or Windows-1252. If you read a file with a different encoding, you need to specify that encoding when converting bytes to a string.
Here’s an example of reading a file encoded in UTF-8:
import java.nio.file.*;
import java.nio.charset.StandardCharsets;
public class ReadFileToString {
public static void main(String[] args) throws Exception {
byte[] bytes = Files.readAllBytes(Paths.get("file.txt"));
String content = new String(bytes, StandardCharsets.UTF_8);
System.out.println(content);
}
}
# Output:
# [The content of 'file.txt' encoded in UTF-8 will be printed here]
In this code, we first read all bytes from the file ‘file.txt’. Then, we convert the bytes to a string using the String
constructor, specifying StandardCharsets.UTF_8
as the character encoding. The content of the file, correctly decoded, is printed to the console.
Best Practices for Dealing with Encodings
When dealing with text files, always be aware of the file’s encoding. If the encoding is not specified, you may get incorrect or garbled output. If you’re unsure about the encoding of a file, tools like the Unix file
command can often give you a good guess.
Exploring Alternative Approaches
While the Files
and Paths
classes are commonly used for reading files in Java, there are other methods and tools you can use. Let’s explore alternatives like the Scanner
class and third-party libraries.
Reading Files with Scanner
The Scanner
class is a simple text scanner which can parse primitive types and strings using regular expressions. It breaks its input into tokens using a delimiter pattern, which by default matches whitespace.
Here’s an example of how you can use Scanner
to read a file and convert it to a string:
import java.util.Scanner;
import java.nio.file.*;
public class ReadFileToString {
public static void main(String[] args) throws Exception {
Scanner scanner = new Scanner(Paths.get("file.txt"));
scanner.useDelimiter("\\Z");
String content = scanner.next();
System.out.println(content);
}
}
# Output:
# [The content of 'file.txt' will be printed here]
In this code, we create a Scanner
object for the file ‘file.txt’. We then set the delimiter to “\Z”, which represents the end of the input, effectively reading the entire file into a string. The content of the file is printed to the console.
Using Third-Party Libraries
There are also third-party libraries like Apache Commons IO and Google Guava which provide utilities for file I/O operations. Here’s an example of reading a file to a string using Apache Commons IO:
import org.apache.commons.io.FileUtils;
import java.io.File;
public class ReadFileToString {
public static void main(String[] args) throws Exception {
String content = FileUtils.readFileToString(new File("file.txt"), "UTF-8");
System.out.println(content);
}
}
# Output:
# [The content of 'file.txt' will be printed here]
In this code, we use FileUtils.readFileToString
to read the file ‘file.txt’ and convert it to a string. The content of the file is printed to the console.
Method | Advantage | Disadvantage |
---|---|---|
Files & Paths | Simple, Convenient | Not suitable for large files |
Scanner | Can parse types and strings | Slower for large files |
Third-Party Libraries | Provide extra utilities | Need to add external dependencies |
While Files
and Paths
are often sufficient for basic file reading tasks, Scanner
and third-party libraries offer more flexibility and functionality. However, they come with their own trade-offs, such as performance considerations and additional dependencies. Your choice of method should depend on your specific use case and requirements.
Troubleshooting Common Issues in Java File Reading
While reading a file and converting it to a string in Java can be straightforward, you might encounter some common issues. Understanding these issues and knowing how to troubleshoot them can save you a lot of time and frustration.
Handling FileNotFoundException
One of the most common issues you might face is FileNotFoundException
. This exception is thrown when a file with the specified pathname does not exist.
import java.nio.file.*;
public class ReadFileToString {
public static void main(String[] args) throws Exception {
String content = new String(Files.readAllBytes(Paths.get("nonexistent.txt")));
System.out.println(content);
}
}
# Output:
# java.nio.file.NoSuchFileException: nonexistent.txt
In this example, we’re trying to read a file that doesn’t exist, which results in a NoSuchFileException
. To handle this, you can use a try-catch
block to catch the exception and print an error message.
import java.nio.file.*;
public class ReadFileToString {
public static void main(String[] args) {
try {
String content = new String(Files.readAllBytes(Paths.get("nonexistent.txt")));
System.out.println(content);
} catch (Exception e) {
System.out.println("Error: " + e.getMessage());
}
}
}
# Output:
# Error: nonexistent.txt (No such file or directory)
Dealing with Different Encodings
As we discussed earlier, text files can be encoded in various ways. If you try to read a file with a different encoding without specifying that encoding, you may get incorrect or garbled output. Always be aware of the file’s encoding and specify it when converting bytes to a string.
Other Considerations
There are other considerations to keep in mind when reading a file in Java. For example, always close your resources after using them to prevent resource leaks. If you’re reading a large file, consider using a method that doesn’t read the entire file into memory at once to avoid OutOfMemoryError
. And remember, if you’re unsure about something, don’t hesitate to look it up or ask for help. Happy coding!
Unraveling Java’s File Handling and String Data Type
Understanding the fundamentals of Java’s file handling capabilities and string data type is crucial for effectively reading a file and converting it to a string. Let’s delve deeper into these concepts.
Java’s File Handling Capabilities
Java provides a rich set of APIs for file I/O operations, allowing you to create, read, update, and delete files with ease. These APIs are part of the java.io
and java.nio.file
packages, with classes like File
, Files
, Path
, and Paths
.
The Files
class, in particular, is a utility class that provides methods for file operations, such as reading all bytes or lines from a file, writing bytes or lines to a file, and more. The Paths
class is used to create Path
instances, representing file and directory paths.
Path path = Paths.get("file.txt");
byte[] bytes = Files.readAllBytes(path);
# Output:
# [bytes of 'file.txt' will be stored in the 'bytes' variable]
In this example, we create a Path
instance for ‘file.txt’ and then read all bytes from the file using Files.readAllBytes
.
Understanding the String Data Type
In Java, strings are objects of the String
class, which are immutable sequences of characters. Strings are widely used for storing text and are one of the most commonly used data types in Java.
When we read a file, we often want to convert the bytes we read into a string. This can be done using the String
constructor that takes a byte array as an argument.
String content = new String(bytes);
# Output:
# [The content of 'file.txt' will be stored in the 'content' string]
In this example, we convert the bytes from ‘file.txt’ into a string.
Grasping File Formats and Encodings
Files can come in various formats and encodings. Text files, for instance, can be encoded in UTF-8, ISO-8859-1, or Windows-1252, among others. When reading a file and converting it to a string, it’s important to know the file’s encoding to correctly decode the bytes. If the encoding is not specified, the default encoding of the Java runtime is used, which may not always be what you want.
In essence, understanding these fundamentals is key to mastering how to read a file and convert it to a string in Java. With a solid grasp of file handling, string data type, and file encodings, you’ll be able to handle a wide range of file I/O tasks with confidence.
Broadening Horizons: File Reading in Diverse Applications
The ability to read a file and convert it to a string in Java is not just a standalone skill. It’s a fundamental operation that’s relevant in a wide array of applications.
File Reading in Data Processing
In data processing, reading files is a common task. Whether you’re dealing with a simple CSV file or a complex XML document, being able to read the file and convert it to a string allows you to parse and process the data effectively.
Web Development and File Reading
In web development, you might need to read configuration files, HTML templates, or other resources. Understanding how to read a file and convert it to a string in Java can help you manage these resources effectively.
Exploring Related Concepts
Once you’ve mastered reading a file and converting it to a string, you might want to explore related concepts. For instance, you could learn how to write to files in Java, handle different file formats, or work with streams and buffers for more efficient file I/O.
Further Resources for Java File Handling Mastery
To deepen your understanding of file handling in Java, here are some resources you might find useful:
- Java File Class Complete Guide – Explore the functionalities and methods provided by Java’s File class for file I/O operations.
Reading Lines in Java – Learn Java techniques for reading text input line by line for processing or analysis.
Exploring Java FileReader – Master Java file reading operations using FileReader for efficient data retrieval.
Java Tutorials: Basic I/O: This is part of the official Java documentation and provides an overview of file I/O in Java.
Baeldung’s Guide to Java String Class covers Java’s String class, including how to create, convert, and manipulate strings.
DigitalOcean’s Java IO Tutorial covers Java’s I/O API.
Remember, mastering a programming concept involves not only understanding the concept itself but also knowing how to apply it in different contexts and being aware of related concepts. Happy learning!
Wrapping Up: Read Files to String in Java
In this comprehensive guide, we’ve delved into the process of reading a file and converting it to a string in Java. We’ve explored the use of classes, the handling of common issues, and the application of alternative strategies for this task.
We began with the basics, discussing how to use the Files
and Paths
classes to read a file and convert it to a string. We then explored the topic further, discussing how to handle different file formats and encodings. We also covered alternative approaches to reading a file and converting it to a string, such as using the Scanner
class or third-party libraries.
Along the way, we addressed common issues that you might encounter, such as FileNotFoundException
and problems with different encodings, providing solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Files & Paths | Simple, Convenient | Not suitable for large files |
Scanner | Can parse types and strings | Slower for large files |
Third-Party Libraries | Provide extra utilities | Need to add external dependencies |
Whether you’re a beginner just starting out with Java or an experienced developer looking to brush up your skills, we hope this guide has helped you understand how to read a file and convert it to a string in Java.
The ability to handle files is a fundamental skill in programming. With the knowledge you’ve gained from this guide, you’re now well-equipped to handle a wide range of file I/O tasks in Java. Happy coding!