Java readLine() Method: Proper Usage Guide
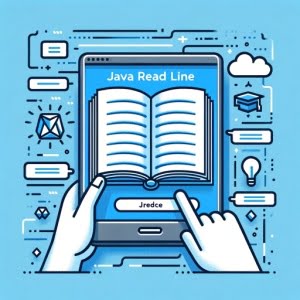
Do you find it challenging to read a line in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling this task in Java, but we’re here to help.
Think of Java as a librarian, capable of retrieving any line from the book of your program. It has built-in methods that can read lines from various sources, making it a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of reading a line in Java, from the basics to more advanced techniques. We’ll cover everything from using the BufferedReader class and the readLine() method, tcco dealing with different sources such as files, user input, and more.
Let’s get started and master reading a line in Java!
TL;DR: How Do I Utilize the readLine() Method?
The
readLine()
method must be used in conjunction with another class, such as theBufferedReader
class. Once the class is created, you can use readLine() with the syntax,String line = reader.readLine();
This combination allows you to read a line from a file or any other input source.
Here’s a simple example:
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
String line = reader.readLine();
System.out.println(line);
# Output:
# 'This is the first line of file.txt'
In this example, we create a BufferedReader
object and pass a new FileReader
object with the file name as a parameter. The readLine()
method is then used to read a line from the file, which is printed to the console.
This is a basic way to read a line in Java, but there’s much more to learn about handling different sources and advanced techniques. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
Understanding BufferedReader and readLine()
In Java, BufferedReader
is a class that makes reading characters, arrays, and lines efficient. It is wrapped around Reader
instances to buffer the input, thereby improving the reading speed.
The readLine()
method is a part of the BufferedReader
class and is used to read a line of text. This method continues to read characters until it encounters a line break (
or
), end of file (EOF), or a carriage return followed immediately by a linefeed (
).
Here’s a simple Java code example that uses BufferedReader
and readLine()
:
import java.io.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
String line = reader.readLine();
while (line != null) {
System.out.println(line);
line = reader.readLine();
}
reader.close();
}
}
# Output:
# 'This is the first line of file.txt'
# 'This is the second line of file.txt'
# 'This is the third line of file.txt'
In this example, we first import the necessary I/O classes. We then create a BufferedReader
object named ‘reader’ and initialize it with a FileReader
object. The FileReader
object is used to read the contents of a file (‘file.txt’ in this case).
We then read and print each line of the file using a while loop until there are no more lines to read (i.e., readLine()
returns null). Finally, we close the BufferedReader
using the close()
method to free up system resources.
Advantages and Potential Pitfalls
Using BufferedReader
and readLine()
is a fast and efficient way to read files in Java. It is especially useful when you need to read large files as it buffers the input, reducing the I/O operations.
However, it’s important to remember to close the BufferedReader
once you’re done to prevent memory leaks. This is done using the close()
method, as shown in the example above. If you’re using Java 7 or later, you can take advantage of the try-with-resources statement, which automatically closes the resources at the end.
Reading Lines from Different Sources
While reading from a file is a common use case, BufferedReader
and readLine()
can also handle other input sources. Let’s explore how to read lines from user input and network connections.
Reading User Input
To read lines from user input, you can use BufferedReader
with InputStreamReader
and System.in
.
import java.io.*;
public class Main {
public static void main(String[] args) throws IOException {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter a line:");
String userInput = reader.readLine();
System.out.println("You entered: " + userInput);
reader.close();
}
}
# Output:
# Enter a line:
# 'This is user input'
# You entered: This is user input
In this example, System.in
is an InputStream
which is typically connected to keyboard input of console programs. InputStreamReader
is a bridge from byte streams to character streams. It reads bytes and decodes them into characters.
Reading from Network Connections
BufferedReader
can also be used to read lines from a network connection. Here’s an example of reading from a URL connection:
import java.io.*;
import java.net.URL;
import java.net.URLConnection;
public class Main {
public static void main(String[] args) throws IOException {
URL url = new URL("https://example.com");
URLConnection connection = url.openConnection();
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
}
}
# Output:
# '<!doctype html>'
# '<html>'
# '...'
In this example, we first create a URL
object and open a connection to it. We then create a BufferedReader
object that reads from the input stream of the URL connection.
Whether you’re reading from a file, user input, or a network connection, the process is largely the same. The main difference lies in the source from which the BufferedReader
reads.
Exploring Alternative Methods: Scanner and Files Class
Java offers other ways to read a line beyond BufferedReader
. Let’s dive into two other methods: the Scanner
class and the Files
class from the NIO package.
Reading a Line with Scanner
Scanner
is a simple text scanner that can parse primitive types and strings using regular expressions.
import java.util.Scanner;
import java.io.File;
import java.io.FileNotFoundException;
public class Main {
public static void main(String[] args) throws FileNotFoundException {
Scanner scanner = new Scanner(new File("file.txt"));
while (scanner.hasNextLine()) {
System.out.println(scanner.nextLine());
}
scanner.close();
}
}
# Output:
# 'This is the first line of file.txt'
# 'This is the second line of file.txt'
# 'This is the third line of file.txt'
In this example, we create a Scanner
object and initialize it with a File
object. We then use the hasNextLine()
and nextLine()
methods to read and print each line of the file.
Reading a Line with Files Class
Java NIO package’s Files
class provides an easier way to read all lines of a file with the readAllLines
method.
import java.nio.file.*;
import java.io.IOException;
import java.util.List;
public class Main {
public static void main(String[] args) throws IOException {
Path path = Paths.get("file.txt");
List<String> lines = Files.readAllLines(path);
for (String line : lines) {
System.out.println(line);
}
}
}
# Output:
# 'This is the first line of file.txt'
# 'This is the second line of file.txt'
# 'This is the third line of file.txt'
Here, we use the Paths.get()
method to get the path of the file and Files.readAllLines()
to read all lines at once into a list of strings.
Comparison of Methods
Method | Advantage | Disadvantage |
---|---|---|
BufferedReader | Efficient for large files | Must handle IOException |
Scanner | Easy to use, can use delimiters | Slower for large files |
Files class | Reads all lines at once, easy to use | Not suitable for very large files |
While BufferedReader
and readLine()
are common approaches, Scanner
and Files
class offer easier usage at the cost of efficiency. Choose the right tool based on your specific needs and the size of the file.
Troubleshooting Common Issues in Java Line Reading
While reading lines in Java, you may encounter some common issues. Let’s discuss these problems and their solutions.
FileNotFoundException
When the file you’re trying to read doesn’t exist or can’t be opened, a FileNotFoundException
is thrown.
try {
BufferedReader reader = new BufferedReader(new FileReader("nonexistent.txt"));
} catch (FileNotFoundException e) {
System.out.println("File not found.");
}
# Output:
# 'File not found.'
In this example, we try to open a file that doesn’t exist. When the FileNotFoundException
is thrown, we catch it and print a simple error message.
IOException
An IOException
is more general and can be thrown for several reasons, such as when an I/O operation is failed or interrupted.
try {
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
String line = reader.readLine();
} catch (IOException e) {
System.out.println("An error occurred.");
}
# Output:
# 'An error occurred.'
In this example, if an I/O error occurs while reading the line, an IOException
is thrown, which we catch and handle by printing an error message.
Tips and Best Practices
- Handle Exceptions: Always handle or declare exceptions when dealing with I/O operations to prevent your program from crashing.
Close Resources: Don’t forget to close resources like
BufferedReader
to avoid memory leaks. Use try-with-resources statement for automatic closing.Check File Existence: Before trying to read a file, check if it exists and is readable to prevent
FileNotFoundException
.Read Documentation: When in doubt, refer to Java’s official documentation. It provides detailed information about classes and methods.
Unpacking Java’s I/O Classes and Methods
To fully grasp the concept of reading a line in Java, it’s crucial to understand the fundamental I/O classes and methods that make this possible.
Journey into Java’s I/O
Java’s I/O (Input/Output) is a part of java.io package and includes a collection of classes and interfaces that handle reading and writing data. The I/O classes can be categorized into two groups: byte-oriented classes (stream classes) and character-oriented classes (reader/writer classes).
Understanding BufferedReader
BufferedReader
is a character-oriented class in Java. It reads text from a character-input stream, buffering characters to provide efficient reading of characters, arrays, and lines.
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
In this line, a BufferedReader
object named ‘reader’ is created. The new FileReader("file.txt")
part is an instance of FileReader
, which is a convenience class for reading character files.
Decoding the FileReader
FileReader
is another character-oriented class in Java. It’s used for reading streams of characters. Here’s how you can use it:
FileReader reader = new FileReader("file.txt");
In this line, a FileReader
object named ‘reader’ is created to read ‘file.txt’.
The Role of readLine()
The readLine()
method is a member of the BufferedReader
class and is used to read a line of text. A line is considered to be terminated by any one of a line feed (‘
‘), a carriage return (‘
‘), or a carriage return followed immediately by a linefeed.
String line = reader.readLine();
In this line, the readLine()
method is called on the ‘reader’ object to read a line from the input stream.
Understanding these fundamental classes and methods is key to mastering line reading in Java.
The Power of Line Reading in Java
Reading lines in Java is not just a basic task, it’s a fundamental skill that’s integral to many applications. Its relevance extends across file handling, data processing applications, and beyond.
Line Reading in File Handling
In file handling, the ability to read lines is crucial. Whether you’re developing a text editor or processing log files, reading lines efficiently and correctly is key. With Java’s powerful I/O classes and methods, you can handle large files with ease.
Line Reading in Data Processing
Data processing often involves reading lines from a file or other sources. For instance, in a CSV file, each line usually represents a record or a data point. By reading lines, you can parse and process each record individually, making data analysis and manipulation manageable.
Exploring Related Concepts
Beyond line reading, there’s a whole world of related concepts in Java. File writing, for example, is the counterpart to file reading. Understanding how to write to a file in Java can give you more control over your data.
Stream handling is another related topic. Streams represent a sequence of data. In Java, you can use streams to read from or write to files, network connections, and more.
Further Resources for Mastering Java I/O
To deepen your understanding of Java’s I/O and related concepts, here are some additional resources:
- IOFlood’s Java File Class Guide – Explore how Java’s File class simplifies file handling tasks in your Java applications.
How to Convert File to String in Java – Master Java file reading to string conversion for handling file-based data efficiently.
Java File Creation Basics – Learn how to use Java’s file manipulation APIs like File.createNewFile() to create files.
Java I/O Tutorial by Oracle offers in-depth explanations and examples on Java’s I/O.
Console readline() Method by GeeksforGeeks presents a detailed explanation of the
readLine()
method in Java’s Console class.Readline in Java by Scaler offers an in-depth understanding of the readline method in Java.
By exploring these resources and practicing with real-world examples, you’ll be well on your way to mastering Java’s I/O and beyond.
Wrapping Up: Java readLine()
In this comprehensive guide, we’ve journeyed through the process of reading a line in Java. From understanding the basics of BufferedReader
and readLine()
to tackling more complex scenarios, we’ve covered the breadth and depth of line reading in Java.
We began with the basic use of BufferedReader
and readLine()
, explaining how these tools work in conjunction to read lines from a file. We then dove into more advanced usage, discussing how to read lines from different sources such as user input and network connections.
Along the way, we tackled common issues you might face when reading lines in Java, such as FileNotFoundException
and IOException
, providing you with solutions and workarounds for each issue. We also explored alternative approaches to line reading, comparing BufferedReader
with other methods like Scanner
and Files
class.
Here’s a quick comparison of the methods we’ve discussed:
Method | Advantage | Disadvantage |
---|---|---|
BufferedReader | Fast and efficient for large files | Must handle IOException |
Scanner | Easy to use, can use delimiters | Slower for large files |
Files class | Reads all lines at once, easy to use | Not suitable for very large files |
Whether you’re just starting out with Java line reading or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of line reading in Java and its various methods.
With its balance of speed, efficiency, and versatility, line reading in Java is a powerful tool in any developer’s arsenal. Happy coding!