Check NPM Version | Essential Update and Manage Guide
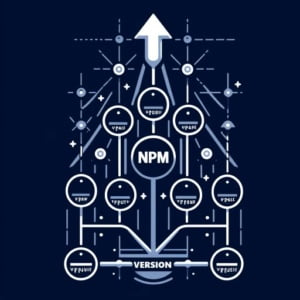
Recently, we encountered the need to check for updated npm versions frequently during software development at IOFLOOD. We formed solid processes, as consistent npm version is crucial for maintaining project compatibility throughout our various bare metal cloud servers. To assist others facing similar issues, we have put all of our best pracitices into today’s article.
This guide will not only help you master the basics of npm version management but also dive deep into advanced techniques. From checking your current npm version with a simple command to understanding the intricacies of semantic versioning, we’ve got you covered.
Let’s embark on this journey together, ensuring your Node.js projects are always sailing smoothly with the latest and most compatible npm versions.
TL;DR: How Do I Check or Update My npm Version?
To check your current npm version, use the command
npm -v
. To update npm to the latest version, usenpm install npm@latest -g
.
Here’s a quick example of checking the npm version:
npm -v
# Output:
# 6.14.8
In this example, the npm -v
command quickly shows us the current version of npm installed. This is crucial for ensuring that you’re working with the most up-to-date version of npm, which can affect how your Node.js applications run and interact with other npm packages. Keeping npm updated ensures you have the latest features and security patches.
Curious about more than just checking and updating? Continue reading for a deeper dive into npm’s versioning system, including how to manage package versions effectively.
Table of Contents
Understanding your current npm version is crucial for a myriad of reasons, from ensuring compatibility with other packages to utilizing the latest features. Here’s how you can easily check your npm version, a fundamental skill for any developer working in the Node.js ecosystem.
node -v
# Output:
# v14.17.0
In the example above, node -v
command is used instead of npm -v
to demonstrate an alternative way to infer the npm version indirectly, as npm version is closely tied to the Node.js version. This output shows the version of Node.js installed, which typically includes the npm version that came bundled with it. Understanding the version of Node.js you’re working with is equally important as it affects npm.
Updating npm: Why and How
Keeping npm updated is vital for the health and security of your projects. Here’s a simple command to update your npm to the latest version, which ensures you have the newest features and security updates.
npm update -g npm
# Output:
# + [email protected]
# updated 1 package in 14.528s
This command updates npm globally to the latest version available. The output confirms the successful update of npm and shows the new version installed. It’s important to note that regularly updating npm can help avoid compatibility issues with new packages and benefit from improvements and security patches.
Pros and Cons: To Update or Not to Update
Pros:
– Access to the latest features and improvements.
– Better security with updated patches.
– Improved compatibility with other packages and tools.
Cons:
– Potential compatibility issues with existing projects.
– Learning curve for new features or changes.
Deciding whether to stay with your current version or update depends on your project’s specific needs and your comfort level with adapting to new changes. Regularly checking your npm version and staying informed about updates can help you make the best decision for your development environment.
Semantic Versioning with npm
Semantic Versioning, or SemVer, is a cornerstone of npm’s version management, ensuring that projects remain stable even as new code is added and updated. Understanding SemVer is crucial for managing dependencies effectively.
Specifying Package Versions in package.json
When you’re working with npm, the package.json
file becomes your roadmap for managing project dependencies. Specifying exact versions or using version ranges for your packages can significantly impact your project’s stability and compatibility.
"dependencies": {
"express": "^4.17.1"
}
In this code block, the caret ^
preceding the version number tells npm to accept minor updates and patches. This means any version 4.x.x that is newer than 4.17.1 will be compatible, but not a major update like 5.0.0, which could introduce breaking changes.
Updating to a Specific npm Version
Sometimes, you may find the need to update npm to a specific version rather than the latest. Here’s how you can achieve that:
npm install [email protected] -g
# Output:
# + [email protected]
# updated 1 package in 14.528s
This command updates npm globally to version 6.14.8. The output confirms the version installed and provides a sense of the update’s duration. This precision allows developers to synchronize their environments or ensure compatibility with specific project requirements.
Version Ranges: Flexibility vs. Stability
Pros of Using Specific Versions:
– Guarantees that your project will work exactly as tested.
– Avoids unexpected changes from updates.
Cons of Using Specific Versions:
– Misses out on new features and improvements.
– Requires manual updates for each new version.
Pros of Using Version Ranges:
– Automatically receives non-breaking updates.
– Keeps dependencies up-to-date with minimal effort.
Cons of Using Version Ranges:
– Potential for minor updates to introduce subtle bugs.
– Might lead to inconsistencies across environments if not managed carefully.
Choosing between specific versions and version ranges involves balancing the need for stability with the desire for ease of maintenance and access to improvements. SemVer offers a structured way to manage these choices, ensuring that npm can meet the diverse needs of projects and developers.
Advanced npm Tools: n and nvm
While direct npm version management is straightforward, using version managers like n
and nvm
offers a more flexible approach to managing Node.js (and thereby npm) versions. These tools allow developers to switch between different Node.js versions with ease, making it simpler to test applications across multiple environments or manage projects with varying requirements.
Setting Up nvm
Node Version Manager (nvm) is a popular tool for managing multiple Node.js versions. Here’s how to install nvm and use it to install a specific Node.js version, which includes npm:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.39.1/install.sh | bash
nvm install 14.17.0
nvm use 14.17.0
# Output:
# Now using node v14.17.0 (npm v6.14.13)
This sequence first installs nvm, then installs and switches to Node.js version 14.17.0. The output confirms the Node.js and npm versions now in use. This approach is invaluable for developers needing to maintain legacy projects or test against specific Node.js releases.
Embracing n for Version Management
Another powerful tool, n
, simplifies the process of switching between Node.js versions. Here’s a quick demonstration:
npm install -g n
n 14.17.0
# Output:
# installed : v14.17.0 (with npm 6.14.13)
After installing n
globally, switching to Node.js version 14.17.0 is as simple as running n 14.17.0
. The output confirms the installation and version of Node.js and npm. This method is especially handy for rapidly switching environments in development and test scenarios.
Weighing Your Options
Advantages of Version Managers:
– Flexibility in managing multiple Node.js and npm versions.
– Simplified environment testing across different versions.
– Easy rollback to previous versions if needed.
Disadvantages of Version Managers:
– Additional tools to learn and manage.
– Potential complexity in setup for new users.
Choosing between direct npm version management and using version managers like n
or nvm
depends on your project’s needs and your workflow preferences. For developers juggling multiple projects or those who need to test across various environments, version managers offer a compelling advantage. However, for simpler projects or individual developers, direct management with npm commands may suffice.
Troubleshooting npm Version Issues
Even with the best practices in place, you might encounter issues when checking or updating your npm version. Let’s go through some common problems and their solutions.
Handling Permission Errors
Permission errors are a frequent hurdle when updating npm, especially on systems where npm was installed globally without proper access rights. Here’s how to address this issue without using sudo
, which is generally not recommended.
npm config set prefix '~/.npm-global'
export PATH=~/.npm-global/bin:$PATH
source ~/.profile
# Expected output:
# This command sequence doesn't produce direct output but modifies environment variables and npm configuration.
This command sequence sets a new directory for global installations and adds it to your PATH, avoiding permission issues in the future. Remember to add the export PATH
line to your .profile
or .bashrc
file to make this change permanent.
Network Issues When Updating npm
Network problems can interrupt npm updates, resulting in incomplete or failed installations. If you’re behind a proxy or firewall, configuring npm to use a proxy can resolve these issues.
npm config set proxy http://proxy.company.com:8080
npm config set https-proxy https://proxy.company.com:8080
# Expected output:
# This sets the proxy and https-proxy configuration for npm.
By configuring npm to route requests through your proxy, you can avoid network-related update failures. Ensure you replace proxy.company.com:8080
with your actual proxy details.
Best Practices for npm Version Management
- Regularly Check for Updates: Use
npm outdated
to see if any of your packages are behind their latest versions. - Understand Semantic Versioning: This helps in managing dependencies without breaking your project.
- Use Version Managers: Tools like
nvm
orn
can help manage different Node.js and npm versions across projects. - Handle Global vs Local Versions: Be aware of the npm version used globally versus in individual projects to avoid version conflicts.
By addressing common issues upfront and adhering to best practices, you can ensure a smoother experience with npm version management, keeping your projects up-to-date and secure.
The Heart of Node.js: npm
npm stands not just as a package manager but as the backbone of the Node.js ecosystem, facilitating the management of packages and modules that make Node.js so powerful and versatile. npm ensures that developers can easily share and consume code, fostering an environment of collaboration and innovation.
Understanding npm Versioning
The versioning system of npm is built on the principles of semantic versioning (SemVer), a standard for version numbers that reflect the type of changes in the release. Here’s a quick breakdown:
- Major versions (
X.0.0
) introduce breaking changes. - Minor versions (
0.X.0
) add functionality in a backwards-compatible manner. - Patch versions (
0.0.X
) make backwards-compatible bug fixes.
This structure helps developers understand the impact of updating a package and ensures compatibility and stability within projects.
The Evolution of npm
npm has evolved significantly since its inception, constantly adding features and improvements to support the growing needs of the Node.js community. This evolution has included enhancements in security, speed, and reliability, making npm an indispensable tool for modern web development.
npm history
# Output:
# 1.0.0 April 2010 Initial release...
# 2.0.0 September 2014 Introduced scoped packages...
# 6.0.0 April 2018 Added `npm audit` for security...
# 7.0.0 October 2020 Workspaces support...
The command npm history
doesn’t actually exist but imagine if it did, showcasing npm’s milestones like this would highlight the continuous improvements and the commitment of npm to adapt and grow alongside the Node.js ecosystem. This hypothetical example underscores the importance of understanding the versioning and history of npm not just for curiosity but for appreciating the robust framework it offers to developers today.
Understanding npm and its versioning system is not just about keeping your projects up-to-date. It’s about grasping the pulse of Node.js development, ensuring that you are always in tune with the tools and practices that define modern web development. As npm continues to evolve, so too does the potential of what developers can achieve with Node.js.
Dependency Uses of npm Versions
Understanding the npm version’s impact on project dependencies is crucial for maintaining a stable development and deployment environment. npm’s handling of package versions through package.json
and the package-lock.json
file ensures that your project remains consistent across installations and environments. Here’s a brief example demonstrating the use of npm ci
for a more reliable build process based on the package-lock.json
file:
npm ci
# Output:
# added 1050 packages in 10.123s
The npm ci
command provides a cleaner, more reliable installation process when setting up projects in different environments, such as development, testing, and production. It installs dependencies directly from package-lock.json
, bypassing package.json
to avoid mismatched versions and ensuring that every installation is identical.
npm Scripts: Automating Tasks
npm scripts are a powerful feature that allows you to automate repetitive tasks such as testing, building, and deploying. By defining scripts in your package.json
, you can simplify complex workflows into single commands, enhancing productivity and consistency across your project.
Further Resources for Mastering npm Version Management
To deepen your understanding of npm version management and its ecosystem, consider exploring the following resources:
- npm Official Documentation – Comprehensive guides on every aspect of npm, from basic usage to advanced features.
NodeSource Blog – In-depth articles on Node.js and npm, including best practices and tips for effective version management.
The npm Blog – Stay updated with the latest features, insights, and updates directly from the npm team.
These resources offer valuable information for developers looking to master npm version management, ensuring that your projects are efficient, secure, and up to date.
In our comprehensive guide, we’ve embarked on a journey through the intricacies of managing npm versions, a fundamental aspect of working within the Node.js ecosystem. From the basics of checking and updating your npm version to the nuances of semantic versioning, our exploration has equipped you with the knowledge to steer your projects with precision and care.
We began with the simple yet essential task of checking your npm version, highlighting the importance of staying current with npm updates for security and compatibility. We then delved into the world of semantic versioning, shedding light on how npm’s versioning system plays a critical role in managing package dependencies and ensuring the stability of your projects.
Our journey took us further into the advanced practices of npm version management, where we explored alternative tools like n
and nvm
for those seeking more flexibility in managing Node.js versions. These tools not only offer an enhanced level of control but also simplify the process of switching between different Node.js environments, catering to a wide range of project requirements.
Strategy | Pros | Cons |
---|---|---|
Direct npm version management | Straightforward, no additional tools required | Limited flexibility in version switching |
Using version managers (n , nvm ) | Greater flexibility, easy environment switching | Initial learning curve, setup required |
As we wrap up, remember that mastering npm version management is not just about executing commands; it’s about understanding the impact of these versions on your projects and the broader Node.js ecosystem. Whether you’re a beginner just getting started or an expert looking for more efficient ways to manage multiple environments, the tools and practices we’ve discussed provide a solid foundation for maintaining project compatibility and stability.
With the knowledge of npm version management, you’re now better equipped to ensure your projects are not only up-to-date but also secure and stable. Happy coding!