Pandas Guide: Rename Column in DataFrame
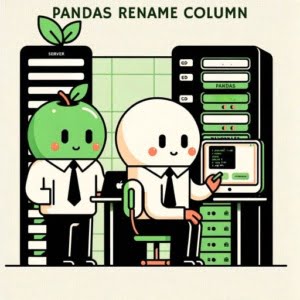
Data organization is key to successful data analysis endeavors at IOFLOOD, and the pandas rename column function is a valuable asset in this regard. This article delves into the intricacies of renaming columns in a Pandas DataFrame using pandas rename column, providing practical examples and best practices for our bare metal hosting customers and fellow developers to streamline their data management processes.
In this comprehensive guide, we’ll navigate the process of renaming columns in a Pandas DataFrame. We’ve incorporated clear, succinct examples to help you grasp the process and apply it to your own data.
So, let’s delve in and reveal the ease of renaming columns in a Pandas DataFrame.
TL;DR: How do I rename columns in a Pandas DataFrame?
You can rename columns in a Pandas DataFrame using the
rename()
function and the syntax,df.rename(columns={'OldName': 'NewName'})
.
Here’s a simple example:
df.rename(columns={'OldName': 'NewName'}, inplace=True)
For more advanced methods, background, and tips, continue reading the article.
Table of Contents
Basics of Pandas DataFrame
Before we delve into the specifics of renaming columns, it’s crucial to grasp what a Pandas DataFrame is and its significance in data analysis. Essentially, a Pandas DataFrame is a two-dimensional, size-mutable, potentially heterogeneous tabular data structure with labeled axes (rows and columns). It’s akin to an Excel spreadsheet or SQL table but with an added layer of power. DataFrames simplify the process of storing, manipulating, and analyzing data in Python.
The structure of a DataFrame is quite straightforward. It comprises rows and columns, each of which can be labeled. The labels for the columns are particularly vital as they serve as a gateway to access the data stored in them. For instance, if you have a DataFrame storing information about a group of individuals, the column labels might include ‘Name’, ‘Age’, ‘Gender’, and so on.
Creating a DataFrame is a simple process. Here’s an illustration:
import pandas as pd
data = {
'Name': ['John', 'Anna', 'Peter'],
'Age': [28, 24, 35],
'Gender': ['Male', 'Female', 'Male']
}
df = pd.DataFrame(data)
print(df)
In this code snippet, we initially import the pandas library. Subsequently, we create a dictionary where each key-value pair signifies a column and its data. This dictionary is then passed to the DataFrame constructor to create a DataFrame.
Renaming Columns with Pandas
Column names are a vital component in data analysis. They serve as a key identifier to unlock specific data points. Without them, navigating our data would be a challenging task. But what if the column names are misleading or don’t accurately represent the data they contain? That’s where the power of renaming becomes beneficial.
Comparison of methods to rename columns:
Method | Use Case | Pitfalls |
---|---|---|
rename() function | Renaming specific columns | Raises KeyError if column doesn’t exist |
Assigning to columns attribute | Renaming all columns | Raises ValueError if count of new names doesn’t match count of existing columns |
Rename() Function
One prevalent method to rename columns is by leveraging the rename()
function. This function is flexible and can be employed to rename specific columns while leaving the rest unaffected.
Let’s consider the following DataFrame:
import pandas as pd
# Creating a DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Chris'],
'Age': [21, 25, 23]
})
print(df)
Output:
Name Age
0 Alice 21
1 Bob 25
2 Chris 23
Now, let’s apply the rename()
function:
# Rename the 'Name' column to 'First Name'
df.rename(columns={'Name': 'First Name'}, inplace=True)
print(df)
Output:
First Name Age
0 Alice 21
1 Bob 25
2 Chris 23
As you can see, ‘Name’ was successfully renamed to ‘First Name’.
In this code snippet, we’re utilizing the rename()
function to alter the ‘Name’ column to ‘First Name’. The columns
parameter accepts a dictionary where the keys represent the old column names and the values are the new column names. The inplace=True
argument implements the changes in the original DataFrame.
Renaming All Columns
An alternative method to rename columns is by assigning a new list of column names to the columns
attribute of the DataFrame. This technique is beneficial when you wish to rename all the columns. However, caution is required with this method as the sequence of the new column names must align with the sequence of the existing column names.
Let’s look at an example. Given a DataFrame like this one:
import pandas as pd
# Create a DataFrame
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Chris'],
'Age': [21, 25, 23],
'Gender': ['Female', 'Male', 'Male']
})
print(df)
Output:
Name Age Gender
0 Alice 21 Female
1 Bob 25 Male
2 Chris 23 Male
Now, let’s assign new column names:
# Assign new column names
df.columns = ['First Name', 'Years', 'Sex']
print(df)
Output:
First Name Years Sex
0 Alice 21 Female
1 Bob 25 Male
2 Chris 23 Male
So as you can see, the column ‘Name’ was renamed to ‘First Name’, ‘Age’ to ‘Years’ and ‘Gender’ to ‘Sex’. When using this method, make sure the new list of column names matches the number of columns in the DataFrame.
Solving Errors with .rename()
While the process of renaming columns is generally straightforward, you might encounter errors if you’re not vigilant.
For instance, attempting to rename a column that doesn’t exist will raise a KeyError. Also, if you’re using the second method and the count of new column names doesn’t match the count of existing columns, you’ll encounter a ValueError.
Example of a KeyError when trying to rename a column that doesn’t exist:
# Attempt to rename a non-existent column
try:
df.rename(columns={'NonExistent': 'NewName'}, inplace=True)
except KeyError as e:
print(f'KeyError: {e}')
Advanced Uses of Pandas Columns
While we’ve covered the basics of renaming columns, Pandas provides even more versatility. Let’s go a step further and explore some advanced column renaming techniques.
Some advanced techniques for renaming columns:
Technique | Description |
---|---|
Renaming multiple columns simultaneously | Use rename() with a dictionary of old and new names |
Renaming columns during data import | Use names parameter in read_csv() |
Renaming columns using a function or mapping | Pass a function to rename() |
Understanding the inplace parameter | Determines whether changes are made to original DataFrame |
We’ll go over each of these methods in turn now:
Renaming Multiple Columns Simultaneously
Renaming a single column is a breeze, but what if you’re tasked with renaming multiple columns at once? The rename()
function comes to the rescue here as well. You can input a dictionary with old and new names for as many columns as you wish to rename. Here’s an example:
df.rename(columns={'Name': 'First Name', 'Age': 'Years', 'Gender': 'Sex'}, inplace=True)
print(df)
In this code snippet, we’re renaming three columns simultaneously. Observe how the dictionary passed to the columns
parameter incorporates all the columns we intend to rename.
Renaming Columns During Data Import
There might be instances when you’d prefer to rename columns as you’re importing data. This method can save you a step in your data cleaning process. When utilizing the read_csv()
function to import data, you can employ the names
parameter to stipulate column names:
df = pd.read_csv('data.csv', names=['First Name', 'Years', 'Sex'])
In this illustration, the column names in the imported DataFrame will be set to ‘First Name’, ‘Years’, and ‘Sex’.
Renaming Columns Using a Function or Mapping
Pandas also empowers you to rename columns using a function or a mapping. This technique is beneficial when you need to apply a transformation to all column names, such as converting to lowercase, replacing spaces with underscores, or adding a prefix. Here’s how you can execute it:
df.rename(columns=str.lower, inplace=True)
This code will convert all column names to lowercase.
Understanding the Inplace Parameter
You might have noticed the inplace
parameter in the rename()
function. This parameter determines whether the renaming operation modifies the original DataFrame (inplace=True
) or returns a new DataFrame with renamed columns (inplace=False
).
If you don’t specify
inplace
, it defaults toFalse
. This parameter is pivotal when you want to preserve the original DataFrame.
Example of using inplace=False
to return a new DataFrame:
# Rename 'Name' column to 'First Name' in a new DataFrame
df_new = df.rename(columns={'Name': 'First Name'}, inplace=False)
print(df_new)
Pandas Library Overview
While renaming columns is a pivotal task, the Pandas library extends far beyond this. In reality, Pandas is a dynamo when it comes to data analysis in Python. It offers data structures and functions required to manipulate structured data, including functionalities for reading and writing data in a multitude of formats.
Pandas provides a plethora of functionalities. Apart from renaming columns, you can also utilize Pandas to manage missing data, merge and reshape datasets, execute aggregations, and much more. Here are a few critical functions and features:
Data Import and Export
Pandas supports an extensive range of formats for data import and export, including but not limited to CSV, Excel, SQL databases, and even Google BigQuery.
Data Cleaning
Pandas offers numerous functions to clean and preprocess data, such as filling missing values, dropping duplicates, and replacing values.
Data Aggregation
With functions like groupby()
, pivot_table()
, and crosstab()
, you can aggregate your data in a multitude of ways.
Data Visualization
Pandas integrates seamlessly with Matplotlib, enabling you to create plots and graphs directly from DataFrames and Series.
Time Series Analysis
Pandas was initially developed for financial modeling, so it includes robust tools for working with dates, times, and time-indexed data.
These are merely a few examples of what you can accomplish with Pandas. Whether you’re a seasoned data scientist, a data analyst, or someone who frequently wrangles data, Pandas is a tool worth mastering.
Use Cases of Pandas Functions
Renaming columns is merely scratching the surface of the capabilities of the Pandas library. Pandas equips you with the tools to perform many other essential tasks, such as managing missing data, merging DataFrames, and more. Let’s take a closer look at some of these tasks.
Managing Missing Data
Missing values are a common occurrence in real-world data. Pandas equips you with several methods to handle such data, including isnull()
, notnull()
, dropna()
, and fillna()
. These methods empower you to detect, remove, or replace missing values in your DataFrame.
# Detect missing values
df.isnull()
# Remove rows with missing values
df.dropna()
# Replace missing values with a specified value
df.fillna(value)
Merging DataFrames
If you’re dealing with multiple related datasets, you might need to consolidate them into a single DataFrame. The merge()
function in Pandas enables you to do this. You can specify the columns to merge on, the type of merge (inner, outer, left, right), and more.
# Merge two DataFrames on a specified column
df = df1.merge(df2, on='column_name')
Additional Data Cleaning Tasks
Pandas also offers various functions for other data cleaning tasks, such as converting data types, replacing values, and more. For instance, you can use the astype()
function to convert the data type of a column:
# Convert the data type of a column to float
df['column_name'] = df['column_name'].astype(float)
These examples merely hint at the power and versatility of the Pandas library beyond renaming columns. The library is incredibly robust, making it an essential tool for anyone manipulating data in Python.
Further Resources for Pandas Library
If you’re interested in learning more ways to utilize the Pandas library, here are a few resources that you might find helpful:
- Mastering Data Manipulation with Pandas: Tips and Tricks by IOFlood: Enhance your data manipulation skills with Pandas by diving into this resource, which provides advanced techniques and helpful suggestions for efficient data handling.
How to Use Pandas Merge with DataFrame Objects: Our tutorial explores how to use the merge function in Pandas to combine DataFrame objects based on common columns.
Using Pandas drop() Function: A Guide: Our guide provides instructions on how to use the drop() function in Pandas to remove columns from a DataFrame in Python.
How to Rename Columns in Pandas DataFrame: This GeeksforGeeks article provides a tutorial on how to rename columns in a Pandas DataFrame using various methods in Python.
pandas.DataFrame.rename() – pandas API Reference: The official pandas documentation for the rename() function, offering a comprehensive explanation of how to use it to rename columns in a DataFrame, including different renaming strategies and examples.
Pandas Merge, Join, and Concatenate: A Comprehensive Guide: A detailed guide on combining DataFrames in Pandas using merge, join, and concatenate operations.
Recap: Columns and Pandas .rename
Renaming columns in a Pandas DataFrame is a straightforward yet vital operation in data analysis. This process can significantly enhance your data’s readability and usability, especially when the original column names are ambiguous, overly lengthy, or simply lacking in descriptiveness.
The prowess of Pandas extends well beyond renaming columns. From managing missing data and merging DataFrames to importing/exporting data and visualizing data, Pandas boasts a wide array of functionalities that make it an indispensable tool for data analysis in Python.
We’ve journeyed through the basics of renaming columns, from employing the rename()
function to directly assigning new column names. Additionally, we’ve delved into some advanced column renaming techniques, such as renaming multiple columns simultaneously, renaming columns during data import, and renaming columns using a function or mapping.
We’ve observed how the inplace
parameter in the rename()
function can dictate whether the renaming operation alters the original DataFrame or generates a new one. These techniques underscore the adaptability and power of the Pandas library in data manipulation.
Regardless of whether you’re an experienced data scientist or a novice just starting out, mastering Pandas will undeniably equip you with a potent tool in your data analysis toolkit. Here’s to effortless data wrangling!