Adding Elements to a List in Java: A How-To Guide
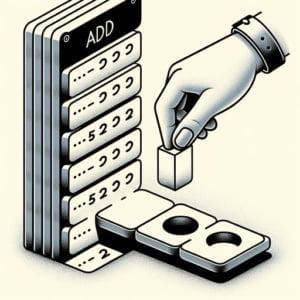
Are you finding it challenging to add elements to a list in Java? You’re not alone. Many developers find themselves puzzled when it comes to using the add()
method in Java’s List interface. Think of the add()
method as a tool – a tool that allows you to include new elements in your list, just like adding items to your shopping list.
This guide will walk you through the usage of the add()
method, from basic to advanced techniques. We’ll cover everything from the simple addition of elements to a list, to more complex scenarios like adding elements at a specific index. We’ll also explore alternative approaches and discuss common issues and their solutions.
So, let’s dive in and start mastering the add()
method in Java Lists!
TL;DR: How Do I Add an Element to a List in Java?
To add an element to a list in Java, you can use the
add()
method of the List interface, with the syntaxmyList.add(valueToAdd);
. This method allows you to include new elements in your list.
Here’s a simple example:
List<String> list = new ArrayList<>();
list.add('Hello');
// Output:
// [Hello]
In this example, we create a new ArrayList and use the add()
method to insert the string ‘Hello’ into the list. The output shows that the list now contains one element: ‘Hello’.
This is a basic way to use the
add()
method in Java, but there’s much more to learn about adding elements to a list. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Usage of add()
Method in Java Lists
The add()
method in Java is a simple yet powerful tool that allows you to add elements to your list. Let’s break down how you can use this method in your Java programs.
Step-by-Step Guide to Using add()
- First, you need to create a list. In Java, you can create a list using the ArrayList class, which implements the List interface. Here’s how you do it:
List<String> myList = new ArrayList<>();
In this line of code, we’ve created an empty list of strings named ‘myList’.
- Now, let’s add an element to our list using the
add()
method:
myList.add('Java');
// Output:
// [Java]
The add()
method takes the element you want to add as a parameter and appends it to the end of the list. The output shows that ‘Java’ has been successfully added to ‘myList’.
Advantages and Potential Pitfalls
The add()
method is straightforward to use and provides a quick way to add elements to your list. However, it’s important to note that this method always appends the element to the end of the list. If you want to insert an element at a specific index, you’ll need to use a different variant of the add()
method, which we will cover in the ‘Advanced Use’ section.
Another potential pitfall is that the add()
method does not check for duplicate elements. If you add the same element multiple times, it will appear in the list multiple times. If you want to avoid duplicates, you might want to consider using a Set instead of a List.
Inserting Elements at Specific Indices
While the basic add()
method is great for quickly appending elements to your list, Java provides a more flexible variant of this method for more complex scenarios. Specifically, you can use the add()
method to insert an element at a specific index in your list.
Code Example: Inserting Elements at Specific Indices
Let’s assume we have a list with some elements, and we want to insert a new element at the second position (index 1, as Java uses zero-based indexing). Here’s how you can do it:
List<String> myList = new ArrayList<>();
myList.add('Java');
myList.add('Python');
myList.add(1, 'C++');
System.out.println(myList);
// Output:
// [Java, C++, Python]
In this example, we first create a list and add ‘Java’ and ‘Python’ to it. Then, we use the add(int index, E element)
method to insert ‘C++’ at the second position. The output shows that ‘C++’ has been successfully inserted between ‘Java’ and ‘Python’.
Analyzing the add(int index, E element)
Method
This variant of the add()
method takes two parameters: the index at which you want to insert the new element, and the element itself. It pushes the existing elements to the right, making room for the new element. This method is extremely useful when you want to maintain a specific order in your list.
However, note that if the index you specify is out of range (either negative or greater than the size of the list), Java will throw an IndexOutOfBoundsException
. Therefore, always make sure your index is within the valid range.
Exploring Alternative Methods: addAll()
While the add()
method is a powerful tool for adding individual elements to your list, Java provides another method for adding multiple elements at once: addAll()
. This method is especially useful when you want to merge two lists.
Code Example: Merging Lists with addAll()
Let’s assume we have two lists, and we want to merge them into one. Here’s how you can do it:
List<String> list1 = new ArrayList<>(Arrays.asList('Java', 'Python'));
List<String> list2 = new ArrayList<>(Arrays.asList('C++', 'JavaScript'));
list1.addAll(list2);
System.out.println(list1);
// Output:
// [Java, Python, C++, JavaScript]
In this example, we first create two lists: ‘list1’ containing ‘Java’ and ‘Python’, and ‘list2’ containing ‘C++’ and ‘JavaScript’. We then use the addAll()
method to append all elements from ‘list2’ to ‘list1’. The output shows that ‘list1’ now contains all elements from both lists.
Analyzing the addAll()
Method
The addAll()
method takes a collection as a parameter and appends all its elements to the list. It’s a quick and easy way to merge multiple lists.
However, like the add()
method, addAll()
does not check for duplicate elements. If you add the same element multiple times, it will appear in the list multiple times. Furthermore, addAll()
will append the elements to the end of the list. If you want to insert elements at a specific index, you’ll need to use a different variant of this method: addAll(int index, Collection c)
.
As always, choose the method that best suits your needs. If you’re adding individual elements, add()
will do the trick. If you’re merging lists, addAll()
is likely your best bet.
Troubleshooting Common Issues with add()
While the add()
method in Java is relatively straightforward, there are a few common issues that you might encounter when using it. Here, we’ll discuss these problems and provide solutions to help you avoid them.
Issue 1: IndexOutOfBoundsException
One common issue is the IndexOutOfBoundsException
. This occurs when you try to add an element at an index that is out of range (either negative or greater than the size of the list).
List<String> myList = new ArrayList<>();
myList.add('Java');
myList.add(5, 'Python'); // This will throw an IndexOutOfBoundsException
// Output:
// Exception in thread "main" java.lang.IndexOutOfBoundsException: Index: 5, Size: 1
In this example, we attempt to add ‘Python’ at index 5. However, our list only has one element, so the valid indices are 0 and 1 (the latter for appending an element). As a result, Java throws an IndexOutOfBoundsException
.
Solution: Always ensure that the index at which you’re trying to add an element is within the valid range. In other words, it should be greater than or equal to 0 and less than or equal to the size of the list.
Issue 2: Adding Null Elements
Another potential issue is adding null elements to your list. While Java allows this, it can lead to NullPointerExceptions
later on when you attempt to use methods on these null elements.
List<String> myList = new ArrayList<>();
myList.add(null);
String element = myList.get(0);
element.length(); // This will throw a NullPointerException
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, we add a null element to our list and then attempt to call the length()
method on it. This results in a NullPointerException
.
Solution: Be cautious when adding null elements to your list. Always check if an element is null before calling methods on it.
Understanding Java’s List Interface
Before we delve deeper into the add()
method, it’s crucial to understand the basics of the List interface in Java and its core methods.
The List Interface in Java
In Java, the List interface is a part of the Java Collections Framework and extends the Collection interface. It is an ordered collection of elements that can contain duplicate values. In other words, it maintains the insertion order of the elements, meaning the first element you add will be at the first position, the second at the second position, and so on.
You can create a list in Java using various classes that implement the List interface, such as ArrayList, LinkedList, and Vector. Among these, ArrayList is the most commonly used.
List<String> myList = new ArrayList<>();
In this line of code, we’ve created an empty list of strings named ‘myList’ using the ArrayList class.
Core Methods of the List Interface
The List interface provides several methods for manipulating elements in the list. Some of the most commonly used methods include:
add(E element)
: Adds an element to the end of the list.add(int index, E element)
: Inserts an element at a specific index in the list.addAll(Collection c)
: Adds all elements in the specified collection to the end of the list.get(int index)
: Retrieves the element at the specified index in the list.remove(int index)
: Removes the element at the specified index from the list.size()
: Returns the number of elements in the list.
These methods provide the fundamental operations for manipulating lists in Java. Understanding these methods is key to mastering the add()
method and other advanced list operations.
Expanding Your Horizons: add()
in Larger Projects
The add()
method is a fundamental tool in Java, but its true power shines in larger projects. In complex applications, you often need to manage and manipulate large amounts of data. Lists, with their dynamic size and efficient methods like add()
, are perfect for this task.
Consider a project where you’re building a social media application. You might have a list of users, and whenever a new user signs up, you’d use the add()
method to add them to your list. Or imagine you’re developing a to-do list application. Every time a user creates a new task, you’d use add()
to add that task to their list.
These are just a few examples of how the add()
method can be applied in real-world projects. The possibilities are endless.
Exploring Related Concepts
Mastering the add()
method is just the beginning. Java offers a rich set of tools for list manipulation and data management. We recommend exploring the Java Collections Framework, which includes not only lists, but also sets, queues, and maps. Each of these collections has its own unique features and use cases.
Further Resources for Mastering Java Lists
To deepen your understanding of Java lists and the add()
method, Click Here for our useful guide. Additionally, here are some other resources you might find useful:
- Working with Lists of Strings in Java – Learn about adding, removing, and accessing String elements in List collections.
Java List vs ArrayList Comparison – Understand the differences between List and ArrayList interfaces in Java.
Oracle’s Official Java Documentation – A comprehensive guide to the List interface methods and usage scenarios in Java.
Baeldung’s Guide to Java Lists walks through Java lists, including how to create and manipulate them.
GeeksforGeeks’ Java Collections Framework explains Java Collections, including lists, sets, queues, and maps.
Wrapping Up:
In this comprehensive guide, we’ve delved into the usage of the add()
method in Java, an essential tool for manipulating lists.
We began with the basics, understanding how to use the add()
method to append elements to a list. We then expanded to more advanced usage, exploring how to insert elements at specific indices and how to merge lists using the addAll()
method. We also tackled common issues you might encounter when using the add()
method, such as IndexOutOfBoundsException
and NullPointerException
, and provided solutions to help you avoid these pitfalls.
We compared the add()
method with alternative approaches, such as the addAll()
method, giving you a broader perspective on how to handle adding elements to a list in Java. Here’s a quick comparison of these methods:
Method | Use Case | Pros | Cons |
---|---|---|---|
add(E element) | Adding individual elements | Simple and quick | Always appends to the end, does not check for duplicates |
add(int index, E element) | Inserting elements at specific indices | Allows precise control over element position | Can throw IndexOutOfBoundsException if index is out of range |
addAll(Collection c) | Adding multiple elements at once, merging lists | Efficient for large collections | Does not check for duplicates, always appends to the end |
Whether you’re just starting out with Java lists or you’re looking to deepen your understanding of list manipulation, we hope this guide has given you a comprehensive overview of the add()
method and its usage.
With this knowledge, you’re now equipped to handle a wide range of scenarios involving adding elements to lists in Java. Happy coding!