Python Exponents | How to Raise a Number to a Power
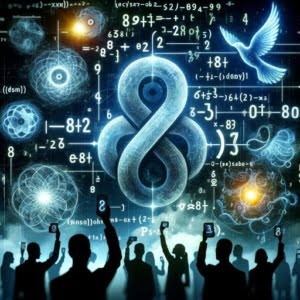
Exponentiation is a fundamental operation in many areas of programming, from data analysis to algorithm design. However, it can be a stumbling block for many coders.
Python, renowned for its readability and ease of use, offers not one, but five powerful techniques to calculate exponents. This blog post aims to simplify these methods, empowering you to harness Python’s capabilities fully. Whether you’re a seasoned programmer or a coding newbie, let’s dive into the world of Python exponentiation.
TL;DR: How can I calculate exponents in Python?
Python offers five methods to calculate exponents. The simplest way is using the double-asterisk operator like
x**n
. Other options include the built-inpow()
function, themath.pow()
function from Python’s math library,np.power()
from the NumPy library, and themath.exp()
function. For more advanced methods and techniques, continue reading the article.
x = 2
n = 3
exponent = x**n
print(exponent)
Table of Contents
Python Exponent Basics
Let’s dive into Python exponents starting with the simplest method: the double-asterisk operator (**). This operator’s simplicity and readability contribute to Python’s popularity.
Consider a scenario where you have a base number ‘x’ and you want to raise it to the power ‘n’. In Python, this operation is as simple as writing x**n
. Here, the left operand ‘x’ represents your base, and the right operand ‘n’ is the power. It’s that straightforward!
But what if ‘n’ is a negative number? Don’t worry! The double-asterisk operator is designed to handle this. When you raise a number to a negative power, you’re essentially calculating the reciprocal of the number raised to that power. So, x**-n
in Python returns 1 divided by x**n
.
x = 2
n = -3
exponent = x**n
print(exponent)
Python pow() function
You might be wondering why we need another function for exponentiation when the double-asterisk operator already does the job. Let’s delve into it.
At first glance, the pow()
function appears to do the same thing as the double-asterisk operator. You input a base ‘x’ and a power ‘n’, like so: pow(x, n)
. This raises ‘x’ to the power ‘n’, similar to x**n
. However, pow()
has a few extra tricks up its sleeve.
Importantly,
pow()
always returns a positive integer, even when ‘n’ is negative. This distinguishespow()
from the double-asterisk operator, which returns a float when ‘n’ is negative.
Example of pow() function with negative exponent:
x = 2
n = -3
exponent = pow(x, n)
print(exponent)
Secondly, pow()
introduces an optional third argument for modulus. This allows you to calculate the modulus of the exponential value in one go. The syntax is as follows: pow(x, n, m)
, where ‘m’ is the modulus. This computes (x**n) % m
in a single step, a feature not offered by the double-asterisk operator.
x = 2
n = 3
m = 2
exponent_modulus = pow(x, n, m)
print(exponent_modulus)
Depending on your specific requirements, you might find pow()
a more fitting tool for your Python exponentiation tasks.
math.pow() function
Having explored the double-asterisk operator and the built-in pow()
function, let’s now shift our focus to the math.pow()
function from Python’s math library.
The math.pow(x, n)
function, akin to its counterparts, raises ‘x’ to the power of ‘n’. Yet, it stands out due to a couple of unique characteristics.
Critically,
math.pow(x, n)
consistently returns a float. This holds true even when ‘x’ and ‘n’ are both integers, and ‘n’ is a positive number.
This contrasts with the built-in pow()
function, which returns an integer when ‘n’ is positive and ‘x’ and ‘n’ are both integers. Hence, if you’re dealing with integers but require a float result, math.pow(x, n)
is the function you should opt for.
Secondly, math.pow(x, n)
does not include the optional modulus argument that the built-in pow()
function does. This implies that you can’t compute the modulus of the exponential value in the same step as the exponentiation.
import math
x = 2
n = 3
exponent = math.pow(x, n)
print(exponent)
At first glance, one might question the utility of math.pow(x, n)
when it appears to offer less functionality than the built-in pow()
function. However, the key lies in precision. Owing to math.pow(x, n)
‘s consistent float return, it can deliver more accurate results when dealing with non-integer exponents or large numbers.
This precision becomes crucial in fields like data analysis and scientific computing, where accuracy is of utmost importance.
NumPy’s np.power(x, n)
So far, we’ve been focusing on Python’s built-in tools for exponentiation. But Python’s mathematical capabilities are not limited to its built-in functions. There’s a powerful library that elevates Python’s mathematical prowess: NumPy, a library adored by data scientists and numerical programmers.
Among the many useful functions in NumPy, np.power(x, n)
is particularly noteworthy. This function, as you might have guessed, raises ‘x’ to the power of ‘n’. Like its Python counterparts, np.power(x, n)
is simple to use. But it has a unique feature: it can handle arrays.
Yes, you read that right! np.power(x, n)
is not confined to scalar values. You can input arrays for ‘x’ and ‘n’, and the function will execute element-wise exponentiation. This is especially beneficial when dealing with large datasets, as it facilitates efficient, vectorized operations.
import numpy as np
x = np.array([2, 3])
n = 3
exponent = np.power(x, n)
print(exponent)
And what about error handling? NumPy has it covered. If you attempt to raise a negative number to a non-integer power, instead of throwing an error like Python’s built-in functions, NumPy will return a NaN
(Not a Number) value.
NumPys np.power(x, n)
is a versatile and powerful tool for exponentiation, particularly when working with arrays or extensive datasets. Its capacity to handle errors gracefully and perform element-wise operations makes it a preferred tool for many Python programmers.
math.exp(x) function
There’s one more tool we need to unpack: the math.exp(x)
function. This function might not calculate exponents in the traditional sense, but its power and versatility warrant its inclusion in our guide.
The math.exp(x)
function computes the exponential value of ‘x’, which is equivalent to raising the mathematical constant ‘e’ to the power of ‘x’. While this might not appear like exponentiation in the conventional sense, it’s an essential operation in numerous areas of mathematics and science.
A significant feature of math.exp(x)
is its adaptability. It can handle positive numbers, negative numbers, and even floating-point numbers, making it a versatile tool for a wide array of mathematical calculations.
import math
x = math.pi
exponential_value = math.exp(x)
print(exponential_value)
But that’s not all. The math.exp(x)
function also works with Python’s built-in numbers, such as math.pi
and math.e
. This means you can easily calculate complex mathematical expressions, like the exponential value of pi, with a single line of code.
Further Resources for Python Math
For more insights on Python Math Functions, here are some additional resources:
- Beginner’s Guide to Python Math Functions – Master Python’s math module for performing mathematical computations.
Understanding Infinity in Python – Learn how infinity is used in Python for expressing limitless quantities.
Handling NaN Values in Python – Explore Python NaN handling techniques and considerations for data analysis tasks.
Python Arithmetic Operators Overview – Learn the basics of arithmetic operators in Python.
Introduction to Python’s Math Module – A beginner-friendly guide to understanding the math module in Python.
Official Python Math Library Documentation – Comprehensive information about the math module straight from Python’s official documentation.
As you dive deeper into these resources, your understanding and application of Python Math Functions will greatly improve.
Final thoughts
On our journey through the realm of Python exponentiation, we’ve examined five distinct methods each offering a different approach to calculating exponents in Python.
We began with the simplicity of the double-asterisk operator, a tool lauded for its directness and readability. We then delved into the built-in pow()
function, which stands out with its optional modulus argument, adding a layer of versatility.
Next, we explored the math.pow()
function, which consistently returns a float for more precise results. We then stepped into the world of NumPy with np.power()
, a function that excels in handling arrays and offers robust error handling capabilities.
Lastly, we examined the math.exp()
function, a unique tool for calculating the exponential value of a number or an inbuilt constant. This function, although unconventional in its approach to exponentiation, is a powerful tool in Python.
Each of these methods brings something unique to the table. Just as a carpenter selects the right tool for the job, you, as a programmer, need to choose the right exponentiation method based on your task.
Looking for a concise yet thorough Python guide? Our syntax cheat sheet has got you covered.
So, the next time you’re faced with an exponentiation task, remember these tools in your Python toolkit and pick the one that fits your needs the best.