NPM UUID Tutorial | Unique Identifiers in Node.js
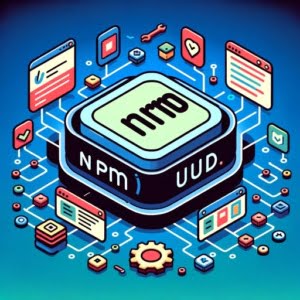
At IOFLOOD, we’ve often faced the need to generate unique identifiers in our Node.js projects. To streamline this process, we’ve put together a helpful guide on using npm to generate UUIDs. By following our simple instructions, you’ll be able to ensure unique identifiers are easily accessible whenever you need them.
Like a unique fingerprint for your data, the npm uuid package offers a robust solution for generating universally unique identifiers (UUIDs). This guide will walk you through the installation, basic usage, and advanced techniques for leveraging the uuid package in your Node.js projects.
Let’s embark on this journey to master UUID generation in Node.js using the npm uuid package!
TL;DR: How Do I Generate a UUID in Node.js Using npm?
To generate a UUID in Node.js, install the
uuid
npm package and use itsuuidv4
functions. Here’s a quick example:
const { v4: uuidv4 } = require('uuid');
console.log(uuidv4());
# Output:
# 'e13b5b4e-ea33-4a77-b9c6-2f7596b5a126'
This will output a unique UUID every time it’s run, providing a simple yet powerful way to create unique identifiers for your applications. The uuid
package supports various versions of UUIDs, allowing you to choose the one that best fits your needs.
Excited to dive deeper? Keep reading for more detailed instructions, examples, and tips on mastering UUID generation in Node.js with the npm uuid package.
Table of Contents
Getting Started with npm UUID
Installing the npm UUID Package
Before you can start generating unique identifiers in your Node.js projects, you need to install the npm uuid package. It’s a straightforward process that integrates seamlessly with your Node.js environment. Here’s how you do it:
npm install uuid
This command fetches the latest version of the uuid package from the npm registry and adds it to your project, ensuring you have all the necessary tools to generate UUIDs.
Your First UUID
Once you’ve installed the package, generating your first UUID is as simple as requiring the package in your Node.js script and calling the appropriate function. Unlike the quick example in the TL;DR section, let’s dive into generating a Version 1 UUID, which is timestamp-based.
const { v1: uuidv1 } = require('uuid');
console.log(uuidv1());
# Output:
# '6f1b92b0-cf81-11ea-9a3f-0800200c9a66'
In this example, we’ve generated a Version 1 UUID. This version of UUID uses the current timestamp, a clock sequence, and the node’s MAC address to generate a unique identifier. It’s particularly useful when you need to sort or order your UUIDs chronologically.
Understanding UUID Versions
The npm uuid package offers several versions of UUIDs, each designed for specific use cases:
- Version 1 (v1): Time-based UUID.
- Version 4 (v4): Randomly generated UUID.
These versions ensure that you can select the most appropriate type of UUID for your project’s needs. Version 4 UUIDs, for example, are ideal for scenarios where you need a completely random identifier without any chronological ordering.
Advanced Methods: UUID Generation
Specifying UUID Versions in Your Code
Once you’re comfortable generating basic UUIDs, you might find yourself needing more control over the version of UUID generated, depending on your project’s requirements. The npm uuid package provides straightforward methods to specify the version of UUID you wish to generate.
For instance, if you’ve decided that the randomness and non-sequential nature of Version 4 UUIDs are what your application needs, you can generate one like this:
const { v4: uuidv4 } = require('uuid');
const myRandomUUID = uuidv4();
console.log(myRandomUUID);
# Output:
# 'f47ac10b-58cc-4372-a567-0e02b2c3d479'
In this example, we specifically generate a Version 4 UUID. The output is a completely random UUID, showcasing the package’s ability to produce a unique identifier that’s hard to predict. This is especially useful in scenarios where security or unpredictability is a concern.
Real-World Application of UUIDs
UUIDs are not just theoretical constructs; they have practical applications in real-world Node.js projects. Whether you’re managing user sessions, ensuring the uniqueness of database entries, or tracking transactions across distributed systems, UUIDs can provide a reliable way to distinguish between entities.
Consider a scenario where you’re building a user registration system. Each user needs a unique identifier. Here’s how you might use a UUID for this purpose:
const { v4: uuidv4 } = require('uuid');
function createUser(username) {
const userId = uuidv4();
// Store the userId along with the username in your database
console.log(`User ${username} created with ID: ${userId}`);
}
createUser('johndoe');
# Output:
# 'User johndoe created with ID: 3f50a7f6-b23a-4aeb-9a2f-c212ac4fe1b8'
This example demonstrates how to integrate UUID generation into a function that creates new users. By assigning a Version 4 UUID to each new user, we ensure that every user has a unique identifier, which is crucial for database integrity and functionality.
Best Practices for UUID Usage
While UUIDs offer a lot of flexibility and uniqueness, it’s important to use them wisely. Here are a few best practices:
- Use the appropriate version of UUID for your needs. Consider the strengths and limitations of each version.
- Store UUIDs efficiently. UUIDs are typically stored as strings, but there are more space-efficient ways to store them if you’re working with a large number of UUIDs.
- Understand the probability of collisions. While UUID collisions are extremely unlikely, they’re not impossible. Design your systems with this in mind.
By mastering these advanced techniques and considerations, you can leverage the full power of the npm uuid package in your Node.js applications, ensuring that your projects benefit from unique and secure identifiers.
Exploring UUID Alternatives in Node.js
Beyond npm uuid: Diverse Libraries for Unique IDs
The npm uuid package is a popular choice for generating UUIDs in Node.js applications, but it’s not the only option available. As developers, understanding and evaluating alternative libraries and methods for generating unique identifiers can empower us to choose the most suitable tool for our projects.
One such alternative is the shortid
package. It generates shorter, URL-friendly unique ids, which can be particularly useful for web applications that need concise identifiers for routing or database keys.
const shortid = require('shortid');
console.log(shortid.generate());
# Output:
# 'HJIO9fWcL'
In this example, shortid.generate()
produces a shorter, URL-friendly unique id. While not a UUID in the traditional sense, the output serves a similar purpose of providing a unique identifier, with the added benefit of being more compact.
Choosing the Right ID Generation Method
When selecting an ID generation library, consider the specific requirements of your project. Here are some factors to keep in mind:
- Length and readability: If your application requires shorter or more readable IDs,
shortid
or similar libraries might be more appropriate. - Universality and uniqueness: For applications that demand globally unique identifiers, traditional UUIDs generated by the
uuid
package may be preferable. - Performance: Different methods and libraries have varying performance characteristics. Test and evaluate the impact on your application.
Expert-Level Considerations
As you delve into alternative methods for generating unique identifiers, consider the broader implications, such as:
- Collision risk: Understand the statistical likelihood of ID collisions for each method and how they might impact your application.
- Scalability: Evaluate how the ID generation method scales with your application, especially in distributed systems.
By exploring alternative libraries and methods for generating unique identifiers, and weighing them against the uuid
package, you can make informed decisions that align with your project’s needs, ensuring optimal functionality and scalability.
Troubleshooting npm UUID
Installation Hiccups
Installing the npm uuid package is usually straightforward, but occasionally, you might run into issues. If you encounter an error during installation, first ensure that your Node.js and npm versions are up to date. Outdated versions can lead to compatibility problems. Here’s how to update npm to the latest version:
npm install npm@latest -g
After updating, try installing the uuid package again. If problems persist, check your network connection and npm’s status page for any ongoing issues.
Handling UUID Collisions
UUIDs are designed to be unique, but there’s a theoretical possibility of collision, especially if you’re generating a vast number of UUIDs. To mitigate this, always use the latest version of the npm uuid package, as it adheres to the UUID standards more strictly, reducing the risk of duplicates.
const { v4: uuidv4 } = require('uuid');
let idSet = new Set();
for (let i = 0; i < 1000000; i++) {
idSet.add(uuidv4());
}
console.log(`Generated ${idSet.size} unique UUIDs.`);
# Output:
# 'Generated 1000000 unique UUIDs.'
This code block demonstrates generating a large number of UUIDs and adding them to a JavaScript Set, which automatically removes duplicates. The output confirms the uniqueness of each UUID, showcasing the package’s reliability. If you ever suspect a collision, this method can help verify the uniqueness of your UUIDs.
Optimizing UUID Storage
UUIDs are 128-bit numbers, often represented as 36-character strings (including hyphens). While this format is convenient for readability and handling in code, it might not be the most space-efficient for storage, especially in large-scale applications. Consider storing UUIDs in a binary format if your database supports it, which can significantly reduce storage requirements.
Final Considerations
When using npm uuid in your projects, remember:
- Keep your Node.js and npm versions up to date.
- Use the latest version of the uuid package to minimize collision risk.
- Consider storage optimization techniques for large-scale applications.
By addressing these common issues and considerations, you can ensure a smoother experience with the npm uuid package, making your Node.js project more robust and efficient.
Understanding UUIDs
What is a UUID?
Universally Unique Identifiers (UUIDs) are 128-bit numbers used to uniquely identify information in computer systems. The concept might seem simple, but the power of UUIDs lies in their vast number of possible combinations, making the probability of generating two identical UUIDs incredibly low.
UUIDs are structured according to a specific standard, which includes various versions, each designed for different use cases. The structure of a UUID is represented as a 32-character hexadecimal string, divided into five groups separated by hyphens.
const exampleUUID = '123e4567-e89b-12d3-a456-426614174000';
console.log(`Example UUID: ${exampleUUID}`);
# Output:
# 'Example UUID: 123e4567-e89b-12d3-a456-426614174000'
This code block demonstrates the typical format of a UUID. Notice the 32 hexadecimal characters (0-9 and a-f) divided into five groups. This structure allows for the immense variety and uniqueness of UUIDs, making them ideal for ensuring that each identifier is truly one of a kind.
The Importance of UUIDs in Web Development
In modern web development, ensuring the uniqueness of entities, such as users, sessions, or transactions, is crucial. UUIDs serve this purpose perfectly, providing a simple way to generate identifiers that are unique not only within the context of a single database or application but globally.
Versions of UUIDs
There are several versions of UUIDs, each designed for specific scenarios:
- Version 1 (Time-based): Generates a UUID based on the current time and the computer’s MAC address.
- Version 4 (Random): Generates a UUID using random or pseudo-random numbers.
These versions offer developers flexibility in choosing the most appropriate type of UUID for their application’s needs. For example, a time-based UUID (Version 1) might be preferred for applications where the chronological order of IDs is relevant, while a randomly generated UUID (Version 4) is a better fit for ensuring privacy and reducing predictability.
By understanding the fundamentals of UUIDs, their structure, versions, and use cases, developers can make informed decisions about generating and using unique identifiers in their projects. This knowledge is essential for building robust, scalable, and secure web applications.
Practical Usage in Tech: UUIDs
UUIDs in Distributed Systems
In the realm of distributed systems, UUIDs play a pivotal role in ensuring data consistency across different nodes. They allow for the unique identification of information without the need for a centralized authority to manage ID generation. This decentralization is crucial for systems where uptime and scalability are key.
const { v4: uuidv4 } = require('uuid');
const dataID = uuidv4();
console.log(`Data ID for distributed entry: ${dataID}`);
# Output:
# 'Data ID for distributed entry: 4e52b5a2-dcbb-4fe5-9518-fb9d76aad4e3'
This code snippet illustrates generating a unique identifier for a piece of data in a distributed system. The generated UUID ensures that each data entry can be uniquely identified across the entire system, preventing conflicts and maintaining integrity.
UUIDs in Database Design
Databases often leverage UUIDs to uniquely identify records. This practice is especially prevalent in scenarios where databases must sync across multiple locations or services, as in the case of microservices architectures. UUIDs eliminate the risk of ID collision, making data merging and replication processes smoother and more reliable.
UUIDs in Microservices Architecture
Microservices architecture, with its distributed nature, benefits greatly from using UUIDs. They serve as a straightforward solution for tracking and identifying requests, services, and data across multiple services, enhancing traceability and debuggability.
Further Resources for Mastering npm UUID
To deepen your understanding of npm UUID and its applications, here are three recommended resources:
- The Official npm UUID Documentation – Comprehensive details on installation, usage, and API reference for npm UUID.
Understanding UUIDs – Insightful view on the history, structure, and types of UUIDs.
Using UUIDs in Web Development – Practical tips and guidelines for integrating UUIDs into web applications.
By exploring these resources, you’ll gain a more thorough understanding of how UUIDs can be utilized in various aspects of software development, from ensuring uniqueness in distributed systems to enhancing database design and facilitating microservices architecture.
Wrap Up: UUID Generation with NPM
In our comprehensive exploration, we’ve uncovered the essentials of generating universally unique identifiers (UUIDs) in Node.js applications using the npm uuid package. This tool not only simplifies the process of ensuring uniqueness across your data but also brings a layer of professionalism and reliability to your projects.
We began with the basics, walking through the installation of the npm uuid package and generating our first UUID. We then advanced our skills by diving into specifying UUID versions and applying UUIDs in real-world Node.js applications, showcasing the practicality and versatility of UUIDs.
In our journey, we also explored alternative approaches for unique identifier generation, comparing the npm uuid package with other libraries. This gave us a broader perspective on the tools available and helped us understand the best scenarios for each method.
Approach | Flexibility | Ease of Use | Use Case |
---|---|---|---|
npm uuid | High | High | General Purpose |
shortid | Moderate | High | URL-friendly IDs |
Custom Methods | Low | Low | Highly Specific Needs |
Whether you’re just starting out with the npm uuid package or seeking to refine your approach to generating unique identifiers, we hope this guide has illuminated the path forward. The ability to generate unique IDs is a cornerstone of modern web development, ensuring data integrity and system reliability.
With the npm uuid package, you’re equipped with a powerful tool that’s both easy to use and highly effective. Remember to consider your project’s specific needs when choosing between UUID versions or alternative methods. Happy coding, and may your identifiers always be as unique as your ideas.